Scale (lv_scale)
Overview
Scale allows you to have a linear scale with ranges and sections with custom styling.
Parts and Styles
The scale widget is divided in the following three parts:
LV_PART_MAIN
Main line. See blue line in the example image.LV_PART_ITEMS
Minor ticks. See red minor ticks in the example image.LV_PART_INDICATOR
Major ticks and its labels (if enabled). See pink labels and green major ticks in the example image.
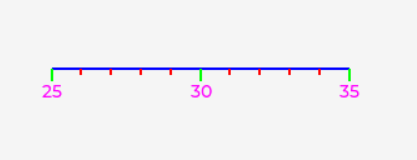
Usage
Set ranges
The minor and major range (values of each tick) are configured with lv_scale_set_range(scale, minor_range, major_range).
Tick drawing order
You can set the drawing of the ticks on top of the main line with lv_scale_set_draw_ticks_on_top(scale, true). The default drawing order is below the main line.
This is a scale with the ticks being drawn below of the main line (default):
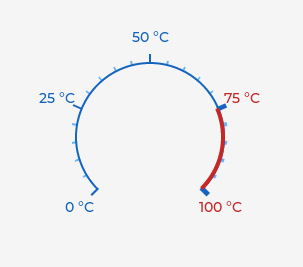
This is an scale with the ticks being drawn at the top of the main line:
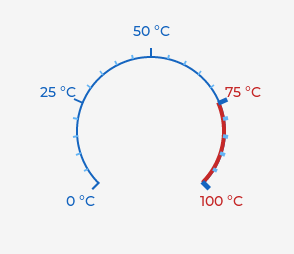
Configure ticks
Set the number of total ticks with lv_scale_set_total_tick_count(scale, total_tick_count) and then configure the major tick being every Nth ticks with lv_scale_set_major_tick_every(scale, nth_tick).
Labels on major ticks can be configured with lv_scale_set_label_show(scale, show_label),
set show_label to true if labels should be drawn, false to hide them.
If instead of a numerical value in the major ticks a text is required they can be set
with lv_scale_set_text_src(scale, custom_labels) using NULL
as the last element,
i.e. static char * custom_labels[3] = {"One", "Two", NULL};.
<strong> NOTE: </strong> The major tick value is calculated with the lv_map API (when not setting the custom labels), this calculation takes into consideration the total tick number and the scale range, so the label drawn can present rounding errors when the calculated value is a float number.
The length of the ticks can be configured with the length style property on the LV_PART_INDICATOR
for major ticks and LV_PART_ITEMS
for minor ticks, for example with local style:
lv_obj_set_style_length(scale, 5, LV_PART_INDICATOR); for major ticks
and lv_obj_set_style_length(scale, 5, LV_PART_ITEMS); for minor ticks.
Sections
A section is the space between a minor and a major range. They can be created with lv_scale_add_section(scale)
and it handles back an lv_scale_section_t
pointer.
The range of the section is configured with lv_scale_section_set_range(section, minor_range, major_range).
The style of each of the three parts of the scale section can be set with
lv_scale_section_set_style(section, PART, style_pointer), where PART can be
LV_PART_MAIN
, LV_PART_ITEMS
or LV_PART_INDICATOR
,
style_pointer should point to a global or static lv_style_t
variable.
For labels the following properties can be configured:
lv_style_set_text_font()
, lv_style_set_text_color()
,
lv_style_set_text_letter_space()
, lv_style_set_text_opa()
.
For lines (main line, major and minor ticks) the following properties can be configured:
lv_style_set_line_color()
, lv_style_set_line_width()
.
Events
No events supported by this widget.
Keys
No keys supported by this widget.
Example
C code
View on GitHub#include "../../lv_examples.h"
#if LV_USE_SCALE && LV_BUILD_EXAMPLES
/**
* A simple horizontal scale
*/
void lv_example_scale_1(void)
{
lv_obj_t * scale = lv_scale_create(lv_screen_active());
lv_obj_set_size(scale, lv_pct(80), 100);
lv_scale_set_mode(scale, LV_SCALE_MODE_HORIZONTAL_BOTTOM);
lv_obj_center(scale);
lv_scale_set_label_show(scale, true);
lv_scale_set_total_tick_count(scale, 31);
lv_scale_set_major_tick_every(scale, 5);
lv_obj_set_style_length(scale, 5, LV_PART_ITEMS);
lv_obj_set_style_length(scale, 10, LV_PART_INDICATOR);
lv_scale_set_range(scale, 10, 40);
}
#endif
C code
View on GitHub#include "../../lv_examples.h"
#if LV_USE_SCALE && LV_BUILD_EXAMPLES
/**
* An vertical scale with section and custom styling
*/
void lv_example_scale_2(void)
{
lv_obj_t * scale = lv_scale_create(lv_screen_active());
lv_obj_set_size(scale, 60, 200);
lv_scale_set_label_show(scale, true);
lv_scale_set_mode(scale, LV_SCALE_MODE_VERTICAL_RIGHT);
lv_obj_center(scale);
lv_scale_set_total_tick_count(scale, 21);
lv_scale_set_major_tick_every(scale, 5);
lv_obj_set_style_length(scale, 10, LV_PART_INDICATOR);
lv_obj_set_style_length(scale, 5, LV_PART_ITEMS);
lv_scale_set_range(scale, 0, 100);
static const char * custom_labels[] = {"0 °C", "25 °C", "50 °C", "75 °C", "100 °C", NULL};
lv_scale_set_text_src(scale, custom_labels);
static lv_style_t indicator_style;
lv_style_init(&indicator_style);
/* Label style properties */
lv_style_set_text_font(&indicator_style, LV_FONT_DEFAULT);
lv_style_set_text_color(&indicator_style, lv_palette_darken(LV_PALETTE_BLUE, 3));
/* Major tick properties */
lv_style_set_line_color(&indicator_style, lv_palette_darken(LV_PALETTE_BLUE, 3));
lv_style_set_width(&indicator_style, 10U); /*Tick length*/
lv_style_set_line_width(&indicator_style, 2U); /*Tick width*/
lv_obj_add_style(scale, &indicator_style, LV_PART_INDICATOR);
static lv_style_t minor_ticks_style;
lv_style_init(&minor_ticks_style);
lv_style_set_line_color(&minor_ticks_style, lv_palette_lighten(LV_PALETTE_BLUE, 2));
lv_style_set_width(&minor_ticks_style, 5U); /*Tick length*/
lv_style_set_line_width(&minor_ticks_style, 2U); /*Tick width*/
lv_obj_add_style(scale, &minor_ticks_style, LV_PART_ITEMS);
static lv_style_t main_line_style;
lv_style_init(&main_line_style);
/* Main line properties */
lv_style_set_line_color(&main_line_style, lv_palette_darken(LV_PALETTE_BLUE, 3));
lv_style_set_line_width(&main_line_style, 2U); // Tick width
lv_obj_add_style(scale, &main_line_style, LV_PART_MAIN);
/* Add a section */
static lv_style_t section_minor_tick_style;
static lv_style_t section_label_style;
static lv_style_t section_main_line_style;
lv_style_init(§ion_label_style);
lv_style_init(§ion_minor_tick_style);
lv_style_init(§ion_main_line_style);
/* Label style properties */
lv_style_set_text_font(§ion_label_style, LV_FONT_DEFAULT);
lv_style_set_text_color(§ion_label_style, lv_palette_darken(LV_PALETTE_RED, 3));
lv_style_set_line_color(§ion_label_style, lv_palette_darken(LV_PALETTE_RED, 3));
lv_style_set_line_width(§ion_label_style, 5U); /*Tick width*/
lv_style_set_line_color(§ion_minor_tick_style, lv_palette_lighten(LV_PALETTE_RED, 2));
lv_style_set_line_width(§ion_minor_tick_style, 4U); /*Tick width*/
/* Main line properties */
lv_style_set_line_color(§ion_main_line_style, lv_palette_darken(LV_PALETTE_RED, 3));
lv_style_set_line_width(§ion_main_line_style, 4U); /*Tick width*/
/* Configure section styles */
lv_scale_section_t * section = lv_scale_add_section(scale);
lv_scale_section_set_range(section, 75, 100);
lv_scale_section_set_style(section, LV_PART_INDICATOR, §ion_label_style);
lv_scale_section_set_style(section, LV_PART_ITEMS, §ion_minor_tick_style);
lv_scale_section_set_style(section, LV_PART_MAIN, §ion_main_line_style);
lv_obj_set_style_bg_color(scale, lv_palette_main(LV_PALETTE_BLUE_GREY), 0);
lv_obj_set_style_bg_opa(scale, LV_OPA_50, 0);
lv_obj_set_style_pad_left(scale, 8, 0);
lv_obj_set_style_radius(scale, 8, 0);
lv_obj_set_style_pad_ver(scale, 20, 0);
}
#endif
C code
View on GitHub#include "../../lv_examples.h"
#if LV_USE_SCALE && LV_BUILD_EXAMPLES
LV_IMAGE_DECLARE(img_hand);
lv_obj_t * needle_line;
lv_obj_t * needle_img;
static void set_needle_line_value(void * obj, int32_t v)
{
lv_scale_set_line_needle_value(obj, needle_line, 60, v);
}
static void set_needle_img_value(void * obj, int32_t v)
{
lv_scale_set_image_needle_value(obj, needle_img, v);
}
/**
* A simple round scale
*/
void lv_example_scale_3(void)
{
lv_obj_t * scale_line = lv_scale_create(lv_screen_active());
lv_obj_set_size(scale_line, 150, 150);
lv_scale_set_mode(scale_line, LV_SCALE_MODE_ROUND_INNER);
lv_obj_set_style_bg_opa(scale_line, LV_OPA_COVER, 0);
lv_obj_set_style_bg_color(scale_line, lv_palette_lighten(LV_PALETTE_RED, 5), 0);
lv_obj_set_style_radius(scale_line, LV_RADIUS_CIRCLE, 0);
lv_obj_set_style_clip_corner(scale_line, true, 0);
lv_obj_align(scale_line, LV_ALIGN_LEFT_MID, LV_PCT(2), 0);
lv_scale_set_label_show(scale_line, true);
lv_scale_set_total_tick_count(scale_line, 31);
lv_scale_set_major_tick_every(scale_line, 5);
lv_obj_set_style_length(scale_line, 5, LV_PART_ITEMS);
lv_obj_set_style_length(scale_line, 10, LV_PART_INDICATOR);
lv_scale_set_range(scale_line, 10, 40);
lv_scale_set_angle_range(scale_line, 270);
lv_scale_set_rotation(scale_line, 135);
needle_line = lv_line_create(scale_line);
lv_obj_set_style_line_width(needle_line, 6, LV_PART_MAIN);
lv_obj_set_style_line_rounded(needle_line, true, LV_PART_MAIN);
lv_anim_t anim_scale_line;
lv_anim_init(&anim_scale_line);
lv_anim_set_var(&anim_scale_line, scale_line);
lv_anim_set_exec_cb(&anim_scale_line, set_needle_line_value);
lv_anim_set_duration(&anim_scale_line, 1000);
lv_anim_set_repeat_count(&anim_scale_line, LV_ANIM_REPEAT_INFINITE);
lv_anim_set_playback_duration(&anim_scale_line, 1000);
lv_anim_set_values(&anim_scale_line, 10, 40);
lv_anim_start(&anim_scale_line);
lv_obj_t * scale_img = lv_scale_create(lv_screen_active());
lv_obj_set_size(scale_img, 150, 150);
lv_scale_set_mode(scale_img, LV_SCALE_MODE_ROUND_INNER);
lv_obj_set_style_bg_opa(scale_img, LV_OPA_COVER, 0);
lv_obj_set_style_bg_color(scale_img, lv_palette_lighten(LV_PALETTE_RED, 5), 0);
lv_obj_set_style_radius(scale_img, LV_RADIUS_CIRCLE, 0);
lv_obj_set_style_clip_corner(scale_img, true, 0);
lv_obj_align(scale_img, LV_ALIGN_RIGHT_MID, LV_PCT(-2), 0);
lv_scale_set_label_show(scale_img, true);
lv_scale_set_total_tick_count(scale_img, 31);
lv_scale_set_major_tick_every(scale_img, 5);
lv_obj_set_style_length(scale_img, 5, LV_PART_ITEMS);
lv_obj_set_style_length(scale_img, 10, LV_PART_INDICATOR);
lv_scale_set_range(scale_img, 10, 40);
lv_scale_set_angle_range(scale_img, 270);
lv_scale_set_rotation(scale_img, 135);
/* image must point to the right. E.g. -O------>*/
needle_img = lv_image_create(scale_img);
lv_image_set_src(needle_img, &img_hand);
lv_obj_align(needle_img, LV_ALIGN_CENTER, 47, -2);
lv_image_set_pivot(needle_img, 3, 4);
lv_anim_t anim_scale_img;
lv_anim_init(&anim_scale_img);
lv_anim_set_var(&anim_scale_img, scale_img);
lv_anim_set_exec_cb(&anim_scale_img, set_needle_img_value);
lv_anim_set_duration(&anim_scale_img, 1000);
lv_anim_set_repeat_count(&anim_scale_img, LV_ANIM_REPEAT_INFINITE);
lv_anim_set_playback_duration(&anim_scale_img, 1000);
lv_anim_set_values(&anim_scale_img, 10, 40);
lv_anim_start(&anim_scale_img);
}
#endif
C code
View on GitHub#include "../../lv_examples.h"
#if LV_USE_SCALE && LV_BUILD_EXAMPLES
/**
* A round scale with section and custom styling
*/
void lv_example_scale_4(void)
{
lv_obj_t * scale = lv_scale_create(lv_screen_active());
lv_obj_set_size(scale, 150, 150);
lv_scale_set_label_show(scale, true);
lv_scale_set_mode(scale, LV_SCALE_MODE_ROUND_OUTER);
lv_obj_center(scale);
lv_scale_set_total_tick_count(scale, 21);
lv_scale_set_major_tick_every(scale, 5);
lv_obj_set_style_length(scale, 5, LV_PART_ITEMS);
lv_obj_set_style_length(scale, 10, LV_PART_INDICATOR);
lv_scale_set_range(scale, 0, 100);
static const char * custom_labels[] = {"0 °C", "25 °C", "50 °C", "75 °C", "100 °C", NULL};
lv_scale_set_text_src(scale, custom_labels);
static lv_style_t indicator_style;
lv_style_init(&indicator_style);
/* Label style properties */
lv_style_set_text_font(&indicator_style, LV_FONT_DEFAULT);
lv_style_set_text_color(&indicator_style, lv_palette_darken(LV_PALETTE_BLUE, 3));
/* Major tick properties */
lv_style_set_line_color(&indicator_style, lv_palette_darken(LV_PALETTE_BLUE, 3));
lv_style_set_width(&indicator_style, 10U); /*Tick length*/
lv_style_set_line_width(&indicator_style, 2U); /*Tick width*/
lv_obj_add_style(scale, &indicator_style, LV_PART_INDICATOR);
static lv_style_t minor_ticks_style;
lv_style_init(&minor_ticks_style);
lv_style_set_line_color(&minor_ticks_style, lv_palette_lighten(LV_PALETTE_BLUE, 2));
lv_style_set_width(&minor_ticks_style, 5U); /*Tick length*/
lv_style_set_line_width(&minor_ticks_style, 2U); /*Tick width*/
lv_obj_add_style(scale, &minor_ticks_style, LV_PART_ITEMS);
static lv_style_t main_line_style;
lv_style_init(&main_line_style);
/* Main line properties */
lv_style_set_arc_color(&main_line_style, lv_palette_darken(LV_PALETTE_BLUE, 3));
lv_style_set_arc_width(&main_line_style, 2U); /*Tick width*/
lv_obj_add_style(scale, &main_line_style, LV_PART_MAIN);
/* Add a section */
static lv_style_t section_minor_tick_style;
static lv_style_t section_label_style;
static lv_style_t section_main_line_style;
lv_style_init(§ion_label_style);
lv_style_init(§ion_minor_tick_style);
lv_style_init(§ion_main_line_style);
/* Label style properties */
lv_style_set_text_font(§ion_label_style, LV_FONT_DEFAULT);
lv_style_set_text_color(§ion_label_style, lv_palette_darken(LV_PALETTE_RED, 3));
lv_style_set_line_color(§ion_label_style, lv_palette_darken(LV_PALETTE_RED, 3));
lv_style_set_line_width(§ion_label_style, 5U); /*Tick width*/
lv_style_set_line_color(§ion_minor_tick_style, lv_palette_lighten(LV_PALETTE_RED, 2));
lv_style_set_line_width(§ion_minor_tick_style, 4U); /*Tick width*/
/* Main line properties */
lv_style_set_arc_color(§ion_main_line_style, lv_palette_darken(LV_PALETTE_RED, 3));
lv_style_set_arc_width(§ion_main_line_style, 4U); /*Tick width*/
/* Configure section styles */
lv_scale_section_t * section = lv_scale_add_section(scale);
lv_scale_section_set_range(section, 75, 100);
lv_scale_section_set_style(section, LV_PART_INDICATOR, §ion_label_style);
lv_scale_section_set_style(section, LV_PART_ITEMS, §ion_minor_tick_style);
lv_scale_section_set_style(section, LV_PART_MAIN, §ion_main_line_style);
}
#endif
C code
View on GitHub#include "../../lv_examples.h"
#if LV_USE_SCALE && LV_BUILD_EXAMPLES
/**
* An scale with section and custom styling
*/
void lv_example_scale_5(void)
{
lv_obj_t * scale = lv_scale_create(lv_screen_active());
lv_obj_set_size(scale, lv_display_get_horizontal_resolution(NULL) / 2, lv_display_get_vertical_resolution(NULL) / 2);
lv_scale_set_label_show(scale, true);
lv_scale_set_total_tick_count(scale, 10);
lv_scale_set_major_tick_every(scale, 5);
lv_obj_set_style_length(scale, 5, LV_PART_ITEMS);
lv_obj_set_style_length(scale, 10, LV_PART_INDICATOR);
lv_scale_set_range(scale, 25, 35);
static const char * custom_labels[3] = {"One", "Two", NULL};
lv_scale_set_text_src(scale, custom_labels);
static lv_style_t indicator_style;
lv_style_init(&indicator_style);
/* Label style properties */
lv_style_set_text_font(&indicator_style, LV_FONT_DEFAULT);
lv_style_set_text_color(&indicator_style, lv_color_hex(0xff00ff));
/* Major tick properties */
lv_style_set_line_color(&indicator_style, lv_color_hex(0x00ff00));
lv_style_set_width(&indicator_style, 10U); // Tick length
lv_style_set_line_width(&indicator_style, 2U); // Tick width
lv_obj_add_style(scale, &indicator_style, LV_PART_INDICATOR);
static lv_style_t minor_ticks_style;
lv_style_init(&minor_ticks_style);
lv_style_set_line_color(&minor_ticks_style, lv_color_hex(0xff0000));
lv_style_set_width(&minor_ticks_style, 5U); // Tick length
lv_style_set_line_width(&minor_ticks_style, 2U); // Tick width
lv_obj_add_style(scale, &minor_ticks_style, LV_PART_ITEMS);
static lv_style_t main_line_style;
lv_style_init(&main_line_style);
/* Main line properties */
lv_style_set_line_color(&main_line_style, lv_color_hex(0x0000ff));
lv_style_set_line_width(&main_line_style, 2U); // Tick width
lv_obj_add_style(scale, &main_line_style, LV_PART_MAIN);
lv_obj_center(scale);
/* Add a section */
static lv_style_t section_minor_tick_style;
static lv_style_t section_label_style;
lv_style_init(§ion_label_style);
lv_style_init(§ion_minor_tick_style);
/* Label style properties */
lv_style_set_text_font(§ion_label_style, LV_FONT_DEFAULT);
lv_style_set_text_color(§ion_label_style, lv_color_hex(0xff0000));
lv_style_set_text_letter_space(§ion_label_style, 10);
lv_style_set_text_opa(§ion_label_style, LV_OPA_50);
lv_style_set_line_color(§ion_label_style, lv_color_hex(0xff0000));
// lv_style_set_width(§ion_label_style, 20U); // Tick length
lv_style_set_line_width(§ion_label_style, 5U); // Tick width
lv_style_set_line_color(§ion_minor_tick_style, lv_color_hex(0x0000ff));
// lv_style_set_width(§ion_label_style, 20U); // Tick length
lv_style_set_line_width(§ion_minor_tick_style, 4U); // Tick width
/* Configure section styles */
lv_scale_section_t * section = lv_scale_add_section(scale);
lv_scale_section_set_range(section, 25, 30);
lv_scale_section_set_style(section, LV_PART_INDICATOR, §ion_label_style);
lv_scale_section_set_style(section, LV_PART_ITEMS, §ion_minor_tick_style);
}
#endif
C code
View on GitHub#include "../../lv_examples.h"
#if LV_USE_SCALE && LV_BUILD_EXAMPLES
#if LV_USE_FLOAT
#define my_PRIprecise "f"
#else
#define my_PRIprecise LV_PRId32
#endif
static lv_obj_t * scale;
static lv_obj_t * minute_hand;
static lv_obj_t * hour_hand;
static lv_point_precise_t minute_hand_points[2];
static int32_t hour;
static int32_t minute;
static void timer_cb(lv_timer_t * timer)
{
LV_UNUSED(timer);
minute++;
if(minute > 59) {
minute = 0;
hour++;
if(hour > 11) {
hour = 0;
}
}
/**
* the scale will store the needle line points in the existing
* point array if one was set with `lv_line_set_points_mutable`.
* Otherwise, it will allocate the needle line points.
*/
/* the scale will store the minute hand line points in `minute_hand_points` */
lv_scale_set_line_needle_value(scale, minute_hand, 60, minute);
/* log the points that were stored in the array */
LV_LOG_USER(
"minute hand points - "
"0: (%" my_PRIprecise ", %" my_PRIprecise "), "
"1: (%" my_PRIprecise ", %" my_PRIprecise ")",
minute_hand_points[0].x, minute_hand_points[0].y,
minute_hand_points[1].x, minute_hand_points[1].y
);
/* the scale will allocate the hour hand line points */
lv_scale_set_line_needle_value(scale, hour_hand, 40, hour * 5 + (minute / 12));
}
/**
* A round scale with multiple needles, resembling a clock
*/
void lv_example_scale_6(void)
{
scale = lv_scale_create(lv_screen_active());
lv_obj_set_size(scale, 150, 150);
lv_scale_set_mode(scale, LV_SCALE_MODE_ROUND_INNER);
lv_obj_set_style_bg_opa(scale, LV_OPA_60, 0);
lv_obj_set_style_bg_color(scale, lv_color_black(), 0);
lv_obj_set_style_radius(scale, LV_RADIUS_CIRCLE, 0);
lv_obj_set_style_clip_corner(scale, true, 0);
lv_obj_center(scale);
lv_scale_set_label_show(scale, true);
lv_scale_set_total_tick_count(scale, 61);
lv_scale_set_major_tick_every(scale, 5);
static const char * hour_ticks[] = {"12", "1", "2", "3", "4", "5", "6", "7", "8", "9", "10", "11", NULL};
lv_scale_set_text_src(scale, hour_ticks);
static lv_style_t indicator_style;
lv_style_init(&indicator_style);
/* Label style properties */
lv_style_set_text_font(&indicator_style, LV_FONT_DEFAULT);
lv_style_set_text_color(&indicator_style, lv_palette_main(LV_PALETTE_YELLOW));
/* Major tick properties */
lv_style_set_line_color(&indicator_style, lv_palette_main(LV_PALETTE_YELLOW));
lv_style_set_length(&indicator_style, 8); /* tick length */
lv_style_set_line_width(&indicator_style, 2); /* tick width */
lv_obj_add_style(scale, &indicator_style, LV_PART_INDICATOR);
/* Minor tick properties */
static lv_style_t minor_ticks_style;
lv_style_init(&minor_ticks_style);
lv_style_set_line_color(&minor_ticks_style, lv_palette_main(LV_PALETTE_YELLOW));
lv_style_set_length(&minor_ticks_style, 6); /* tick length */
lv_style_set_line_width(&minor_ticks_style, 2); /* tick width */
lv_obj_add_style(scale, &minor_ticks_style, LV_PART_ITEMS);
/* Main line properties */
static lv_style_t main_line_style;
lv_style_init(&main_line_style);
lv_style_set_arc_color(&main_line_style, lv_color_black());
lv_style_set_arc_width(&main_line_style, 5);
lv_obj_add_style(scale, &main_line_style, LV_PART_MAIN);
lv_scale_set_range(scale, 0, 60);
lv_scale_set_angle_range(scale, 360);
lv_scale_set_rotation(scale, 270);
minute_hand = lv_line_create(scale);
lv_line_set_points_mutable(minute_hand, minute_hand_points, 2);
lv_obj_set_style_line_width(minute_hand, 3, 0);
lv_obj_set_style_line_rounded(minute_hand, true, 0);
lv_obj_set_style_line_color(minute_hand, lv_color_white(), 0);
hour_hand = lv_line_create(scale);
lv_obj_set_style_line_width(hour_hand, 5, 0);
lv_obj_set_style_line_rounded(hour_hand, true, 0);
lv_obj_set_style_line_color(hour_hand, lv_palette_main(LV_PALETTE_RED), 0);
hour = 11;
minute = 5;
lv_timer_t * timer = lv_timer_create(timer_cb, 250, NULL);
lv_timer_ready(timer);
}
#endif
C code
View on GitHub#include "../../lv_examples.h"
#if LV_USE_SCALE && LV_BUILD_EXAMPLES
#include "../../../src/lvgl_private.h" //To expose the fields of lv_draw_task_t
static void draw_event_cb(lv_event_t * e)
{
lv_obj_t * obj = lv_event_get_target(e);
lv_draw_task_t * draw_task = lv_event_get_draw_task(e);
lv_draw_dsc_base_t * base_dsc = lv_draw_task_get_draw_dsc(draw_task);
lv_draw_label_dsc_t * label_draw_dsc = lv_draw_task_get_label_dsc(draw_task);
if(base_dsc->part == LV_PART_INDICATOR) {
if(label_draw_dsc) {
const lv_color_t color_idx[7] = {
lv_palette_main(LV_PALETTE_RED),
lv_palette_main(LV_PALETTE_ORANGE),
lv_palette_main(LV_PALETTE_YELLOW),
lv_palette_main(LV_PALETTE_GREEN),
lv_palette_main(LV_PALETTE_CYAN),
lv_palette_main(LV_PALETTE_BLUE),
lv_palette_main(LV_PALETTE_PURPLE),
};
uint8_t major_tick = lv_scale_get_major_tick_every(obj);
label_draw_dsc->color = color_idx[base_dsc->id1 / major_tick];
/*Free the previously allocated text if needed*/
if(label_draw_dsc->text_local) lv_free((void *)label_draw_dsc->text);
/*Malloc the text and set text_local as 1 to make LVGL automatically free the text.
* (Local texts are malloc'd internally by LVGL. Mimic this behavior here too)*/
char tmp_buffer[20] = {0}; /* Big enough buffer */
lv_snprintf(tmp_buffer, sizeof(tmp_buffer), "%.1f", base_dsc->id2 * 1.0f);
label_draw_dsc->text = lv_strdup(tmp_buffer);
label_draw_dsc->text_local = 1;
lv_point_t size;
lv_text_get_size(&size, label_draw_dsc->text, label_draw_dsc->font, 0, 0, 1000, LV_TEXT_FLAG_NONE);
int32_t new_w = size.x;
int32_t old_w = lv_area_get_width(&draw_task->area);
/* Distribute the new size equally on both sides */
draw_task->area.x1 -= (new_w - old_w) / 2;
draw_task->area.x2 += ((new_w - old_w) + 1) / 2; /* +1 for rounding */
}
}
}
/**
* Customizing scale major tick label color with `LV_EVENT_DRAW_TASK_ADDED` event
*/
void lv_example_scale_7(void)
{
lv_obj_t * scale = lv_scale_create(lv_screen_active());
lv_obj_set_size(scale, lv_pct(80), 100);
lv_scale_set_mode(scale, LV_SCALE_MODE_HORIZONTAL_BOTTOM);
lv_obj_center(scale);
lv_scale_set_label_show(scale, true);
lv_scale_set_total_tick_count(scale, 31);
lv_scale_set_major_tick_every(scale, 5);
lv_obj_set_style_length(scale, 5, LV_PART_ITEMS);
lv_obj_set_style_length(scale, 10, LV_PART_INDICATOR);
lv_scale_set_range(scale, 10, 40);
lv_obj_add_event_cb(scale, draw_event_cb, LV_EVENT_DRAW_TASK_ADDED, NULL);
lv_obj_add_flag(scale, LV_OBJ_FLAG_SEND_DRAW_TASK_EVENTS);
}
#endif
API
lv_scale.h .. Autogenerated