Animation (lv_anim)
You can automatically change the value of a variable between a start and an end value using animations. Animation will happen by periodically calling an "animator" function with the corresponding value parameter.
The animator functions have the following prototype:
void func(void * var, lv_anim_var_t value);
This prototype is compatible with the majority of the property set functions in LVGL. For example lv_obj_set_x(widget, value) or lv_obj_set_width(widget, value)
Create an animation
To create an animation an lv_anim_t
variable has to be initialized
and configured with lv_anim_set_...()
functions.
/* INITIALIZE AN ANIMATION
*-----------------------*/
lv_anim_t a;
lv_anim_init(&a);
/* MANDATORY SETTINGS
*------------------*/
/* Set the "animator" function */
lv_anim_set_exec_cb(&a, (lv_anim_exec_xcb_t) lv_obj_set_x);
/* Set target of the animation */
lv_anim_set_var(&a, widget);
/* Length of the animation [ms] */
lv_anim_set_duration(&a, duration);
/* Set start and end values. E.g. 0, 150 */
lv_anim_set_values(&a, start, end);
/* OPTIONAL SETTINGS
*------------------*/
/* Time to wait before starting the animation [ms] */
lv_anim_set_delay(&a, delay);
/* Set path (curve). Default is linear */
lv_anim_set_path_cb(&a, lv_anim_path_ease_in);
/* Set a callback to indicate when the animation is completed. */
lv_anim_set_completed_cb(&a, completed_cb);
/* Set a callback to indicate when the animation is deleted (idle). */
lv_anim_set_deleted_cb(&a, deleted_cb);
/* Set a callback to indicate when the animation is started (after delay). */
lv_anim_set_start_cb(&a, start_cb);
/* When ready, play the animation backward with this duration. Default is 0 (disabled) [ms] */
lv_anim_set_playback_duration(&a, time);
/* Delay before playback. Default is 0 (disabled) [ms] */
lv_anim_set_playback_delay(&a, delay);
/* Number of repetitions. Default is 1. LV_ANIM_REPEAT_INFINITE for infinite repetition */
lv_anim_set_repeat_count(&a, cnt);
/* Delay before repeat. Default is 0 (disabled) [ms] */
lv_anim_set_repeat_delay(&a, delay);
/* true (default): apply the start value immediately, false: apply start value after delay when the anim. really starts. */
lv_anim_set_early_apply(&a, true/false);
/* START THE ANIMATION
*------------------*/
lv_anim_start(&a); /* Start the animation */
You can apply multiple different animations on the same variable at the
same time. For example, animate the x and y coordinates with
lv_obj_set_x()
and lv_obj_set_y()
. However, only one animation can
exist with a given variable and function pair and lv_anim_start()
will remove any existing animations for such a pair.
Animation path
You can control the path of an animation. The most simple case is linear, meaning the current value between start and end is changed with fixed steps. A path is a function which calculates the next value to set based on the current state of the animation. Currently, there are the following built-in path functions:
lv_anim_path_linear()
: linear animationlv_anim_path_step()
: change in one step at the endlv_anim_path_ease_in()
: slow at the beginninglv_anim_path_ease_out()
: slow at the endlv_anim_path_ease_in_out()
: slow at the beginning and endlv_anim_path_overshoot()
: overshoot the end valuelv_anim_path_bounce()
: bounce back a little from the end value (like hitting a wall)
Speed vs time
By default, you set the animation time directly. But in some cases, setting the animation speed is more practical.
The lv_anim_speed_to_time(speed, start, end) function calculates the
required time in milliseconds to reach the end value from a start value
with the given speed. The speed is interpreted in unit/sec dimension.
For example, lv_anim_speed_to_time(20, 0, 100) will yield 5000
milliseconds. For example, in the case of lv_obj_set_x()
unit is
pixels so 20 means 20 px/sec speed.
Delete animations
You can delete an animation with lv_anim_delete(var, func) if you provide the animated variable and its animator function.
Timeline
A timeline is a collection of multiple animations which makes it easy to create complex composite animations.
Firstly, create an animation element but don't call lv_anim_start()
.
Secondly, create an animation timeline object by calling
lv_anim_timeline_create()
.
Thirdly, add animation elements to the animation timeline by calling
lv_anim_timeline_add(at, start_time, &a). start_time
is the
start time of the animation on the timeline. Note that start_time
will override the value of delay
.
Finally, call lv_anim_timeline_start(at) to start the animation timeline.
It supports forward and backward playback of the entire animation group, using lv_anim_timeline_set_reverse(at, reverse). Note that if you want to play in reverse from the end of the timeline, you need to call lv_anim_timeline_set_progress(at, LV_ANIM_TIMELINE_PROGRESS_MAX) after adding all animations and before starting to play.
Call lv_anim_timeline_stop(at) to stop the animation timeline.
Call lv_anim_timeline_set_progress(at, progress) function to set the state of the Widget corresponding to the progress of the timeline.
Call lv_anim_timeline_get_playtime(at) function to get the total duration of the entire animation timeline.
Call lv_anim_timeline_get_reverse(at) function to get whether to reverse the animation timeline.
Call lv_anim_timeline_delete(at) function to delete the animation timeline. Note: If you need to delete a Widget during animation, be sure to delete the anim timeline before deleting the Widget. Otherwise, the program may crash or behave abnormally.
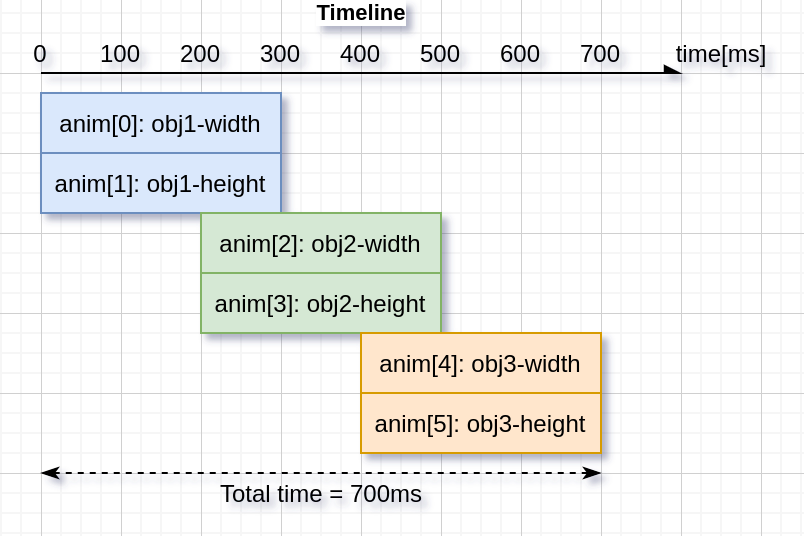
Examples
Start animation on an event
C code
View on GitHub#include "../lv_examples.h"
#if LV_BUILD_EXAMPLES && LV_USE_SWITCH
static void anim_x_cb(void * var, int32_t v)
{
lv_obj_set_x(var, v);
}
static void sw_event_cb(lv_event_t * e)
{
lv_obj_t * sw = lv_event_get_target(e);
lv_obj_t * label = lv_event_get_user_data(e);
if(lv_obj_has_state(sw, LV_STATE_CHECKED)) {
lv_anim_t a;
lv_anim_init(&a);
lv_anim_set_var(&a, label);
lv_anim_set_values(&a, lv_obj_get_x(label), 100);
lv_anim_set_duration(&a, 500);
lv_anim_set_exec_cb(&a, anim_x_cb);
lv_anim_set_path_cb(&a, lv_anim_path_overshoot);
lv_anim_start(&a);
}
else {
lv_anim_t a;
lv_anim_init(&a);
lv_anim_set_var(&a, label);
lv_anim_set_values(&a, lv_obj_get_x(label), -lv_obj_get_width(label));
lv_anim_set_duration(&a, 500);
lv_anim_set_exec_cb(&a, anim_x_cb);
lv_anim_set_path_cb(&a, lv_anim_path_ease_in);
lv_anim_start(&a);
}
}
/**
* Start animation on an event
*/
void lv_example_anim_1(void)
{
lv_obj_t * label = lv_label_create(lv_screen_active());
lv_label_set_text(label, "Hello animations!");
lv_obj_set_pos(label, 100, 10);
lv_obj_t * sw = lv_switch_create(lv_screen_active());
lv_obj_center(sw);
lv_obj_add_state(sw, LV_STATE_CHECKED);
lv_obj_add_event_cb(sw, sw_event_cb, LV_EVENT_VALUE_CHANGED, label);
}
#endif
Playback animation
C code
View on GitHub#include "../lv_examples.h"
#if LV_BUILD_EXAMPLES && LV_USE_SWITCH
static void anim_x_cb(void * var, int32_t v)
{
lv_obj_set_x(var, v);
}
static void anim_size_cb(void * var, int32_t v)
{
lv_obj_set_size(var, v, v);
}
/**
* Create a playback animation
*/
void lv_example_anim_2(void)
{
lv_obj_t * obj = lv_obj_create(lv_screen_active());
lv_obj_set_style_bg_color(obj, lv_palette_main(LV_PALETTE_RED), 0);
lv_obj_set_style_radius(obj, LV_RADIUS_CIRCLE, 0);
lv_obj_align(obj, LV_ALIGN_LEFT_MID, 10, 0);
lv_anim_t a;
lv_anim_init(&a);
lv_anim_set_var(&a, obj);
lv_anim_set_values(&a, 10, 50);
lv_anim_set_duration(&a, 1000);
lv_anim_set_playback_delay(&a, 100);
lv_anim_set_playback_duration(&a, 300);
lv_anim_set_repeat_delay(&a, 500);
lv_anim_set_repeat_count(&a, LV_ANIM_REPEAT_INFINITE);
lv_anim_set_path_cb(&a, lv_anim_path_ease_in_out);
lv_anim_set_exec_cb(&a, anim_size_cb);
lv_anim_start(&a);
lv_anim_set_exec_cb(&a, anim_x_cb);
lv_anim_set_values(&a, 10, 240);
lv_anim_start(&a);
}
#endif
Cubic Bezier animation
C code
View on GitHub#include "../lv_examples.h"
#if LV_BUILD_EXAMPLES && LV_USE_SLIDER && LV_USE_CHART && LV_USE_BUTTON && LV_USE_GRID
/**
* the example show the use of cubic-bezier3 in animation.
* the control point P1,P2 of cubic-bezier3 can be adjusted by slider.
* and the chart shows the cubic-bezier3 in real time. you can click
* run button see animation in current point of cubic-bezier3.
*/
#define CHART_POINTS_NUM 256
struct {
lv_obj_t * anim_obj;
lv_obj_t * chart;
lv_chart_series_t * ser1;
lv_obj_t * p1_slider;
lv_obj_t * p1_label;
lv_obj_t * p2_slider;
lv_obj_t * p2_label;
lv_obj_t * run_btn;
uint16_t p1;
uint16_t p2;
lv_anim_t a;
} ginfo;
static int32_t anim_path_bezier3_cb(const lv_anim_t * a);
static void refer_chart_cubic_bezier(void);
static void run_button_event_handler(lv_event_t * e);
static void slider_event_cb(lv_event_t * e);
static void page_obj_init(lv_obj_t * par);
static void anim_x_cb(void * var, int32_t v);
/**
* create an animation
*/
void lv_example_anim_3(void)
{
static int32_t col_dsc[] = {LV_GRID_FR(1), 200, LV_GRID_FR(1), LV_GRID_TEMPLATE_LAST};
static int32_t row_dsc[] = {30, 10, 10, LV_GRID_FR(1), LV_GRID_TEMPLATE_LAST};
/*Create a container with grid*/
lv_obj_t * cont = lv_obj_create(lv_screen_active());
lv_obj_set_style_pad_all(cont, 2, LV_PART_MAIN);
lv_obj_set_style_pad_column(cont, 10, LV_PART_MAIN);
lv_obj_set_style_pad_row(cont, 10, LV_PART_MAIN);
lv_obj_set_grid_dsc_array(cont, col_dsc, row_dsc);
lv_obj_set_size(cont, 320, 240);
lv_obj_center(cont);
page_obj_init(cont);
lv_anim_init(&ginfo.a);
lv_anim_set_var(&ginfo.a, ginfo.anim_obj);
int32_t end = lv_obj_get_style_width(cont, LV_PART_MAIN) -
lv_obj_get_style_width(ginfo.anim_obj, LV_PART_MAIN) - 10;
lv_anim_set_values(&ginfo.a, 5, end);
lv_anim_set_duration(&ginfo.a, 2000);
lv_anim_set_exec_cb(&ginfo.a, anim_x_cb);
lv_anim_set_path_cb(&ginfo.a, anim_path_bezier3_cb);
refer_chart_cubic_bezier();
}
static int32_t anim_path_bezier3_cb(const lv_anim_t * a)
{
uint32_t t = lv_map(a->act_time, 0, a->duration, 0, 1024);
int32_t step = lv_bezier3(t, 0, ginfo.p1, ginfo.p2, 1024);
int32_t new_value;
new_value = step * (a->end_value - a->start_value);
new_value = new_value >> 10;
new_value += a->start_value;
return new_value;
}
static void refer_chart_cubic_bezier(void)
{
for(uint16_t i = 0; i <= CHART_POINTS_NUM; i ++) {
uint32_t t = i * (1024 / CHART_POINTS_NUM);
int32_t step = lv_bezier3(t, 0, ginfo.p1, ginfo.p2, 1024);
lv_chart_set_value_by_id2(ginfo.chart, ginfo.ser1, i, t, step);
}
lv_chart_refresh(ginfo.chart);
}
static void anim_x_cb(void * var, int32_t v)
{
lv_obj_set_style_translate_x(var, v, LV_PART_MAIN);
}
static void run_button_event_handler(lv_event_t * e)
{
lv_event_code_t code = lv_event_get_code(e);
if(code == LV_EVENT_CLICKED) {
lv_anim_start(&ginfo.a);
}
}
static void slider_event_cb(lv_event_t * e)
{
char buf[16];
lv_obj_t * label;
lv_obj_t * slider = lv_event_get_target(e);
if(slider == ginfo.p1_slider) {
label = ginfo.p1_label;
ginfo.p1 = lv_slider_get_value(slider);
lv_snprintf(buf, sizeof(buf), "p1:%d", ginfo.p1);
}
else {
label = ginfo.p2_label;
ginfo.p2 = lv_slider_get_value(slider);
lv_snprintf(buf, sizeof(buf), "p2:%d", ginfo.p2);
}
lv_label_set_text(label, buf);
refer_chart_cubic_bezier();
}
static void page_obj_init(lv_obj_t * par)
{
ginfo.anim_obj = lv_obj_create(par);
lv_obj_set_size(ginfo.anim_obj, 30, 30);
lv_obj_set_align(ginfo.anim_obj, LV_ALIGN_TOP_LEFT);
lv_obj_remove_flag(ginfo.anim_obj, LV_OBJ_FLAG_SCROLLABLE);
lv_obj_set_style_bg_color(ginfo.anim_obj, lv_palette_main(LV_PALETTE_RED), LV_PART_MAIN);
lv_obj_set_grid_cell(ginfo.anim_obj, LV_GRID_ALIGN_START, 0, 1, LV_GRID_ALIGN_START, 0, 1);
ginfo.p1_label = lv_label_create(par);
ginfo.p2_label = lv_label_create(par);
lv_label_set_text(ginfo.p1_label, "p1:0");
lv_label_set_text(ginfo.p2_label, "p2:0");
lv_obj_set_grid_cell(ginfo.p1_label, LV_GRID_ALIGN_START, 0, 1, LV_GRID_ALIGN_START, 1, 1);
lv_obj_set_grid_cell(ginfo.p2_label, LV_GRID_ALIGN_START, 0, 1, LV_GRID_ALIGN_START, 2, 1);
ginfo.p1_slider = lv_slider_create(par);
ginfo.p2_slider = lv_slider_create(par);
lv_slider_set_range(ginfo.p1_slider, 0, 1024);
lv_slider_set_range(ginfo.p2_slider, 0, 1024);
lv_obj_set_style_pad_all(ginfo.p1_slider, 2, LV_PART_KNOB);
lv_obj_set_style_pad_all(ginfo.p2_slider, 2, LV_PART_KNOB);
lv_obj_add_event_cb(ginfo.p1_slider, slider_event_cb, LV_EVENT_VALUE_CHANGED, NULL);
lv_obj_add_event_cb(ginfo.p2_slider, slider_event_cb, LV_EVENT_VALUE_CHANGED, NULL);
lv_obj_set_grid_cell(ginfo.p1_slider, LV_GRID_ALIGN_STRETCH, 1, 1, LV_GRID_ALIGN_START, 1, 1);
lv_obj_set_grid_cell(ginfo.p2_slider, LV_GRID_ALIGN_STRETCH, 1, 1, LV_GRID_ALIGN_START, 2, 1);
ginfo.run_btn = lv_button_create(par);
lv_obj_add_event_cb(ginfo.run_btn, run_button_event_handler, LV_EVENT_CLICKED, NULL);
lv_obj_t * btn_label = lv_label_create(ginfo.run_btn);
lv_label_set_text(btn_label, LV_SYMBOL_PLAY);
lv_obj_center(btn_label);
lv_obj_set_grid_cell(ginfo.run_btn, LV_GRID_ALIGN_STRETCH, 2, 1, LV_GRID_ALIGN_STRETCH, 1, 2);
ginfo.chart = lv_chart_create(par);
lv_obj_set_style_pad_all(ginfo.chart, 0, LV_PART_MAIN);
lv_obj_set_style_size(ginfo.chart, 0, 0, LV_PART_INDICATOR);
lv_chart_set_type(ginfo.chart, LV_CHART_TYPE_SCATTER);
ginfo.ser1 = lv_chart_add_series(ginfo.chart, lv_palette_main(LV_PALETTE_RED), LV_CHART_AXIS_PRIMARY_Y);
lv_chart_set_range(ginfo.chart, LV_CHART_AXIS_PRIMARY_Y, 0, 1024);
lv_chart_set_range(ginfo.chart, LV_CHART_AXIS_PRIMARY_X, 0, 1024);
lv_chart_set_point_count(ginfo.chart, CHART_POINTS_NUM);
lv_obj_set_grid_cell(ginfo.chart, LV_GRID_ALIGN_STRETCH, 0, 3, LV_GRID_ALIGN_STRETCH, 3, 1);
}
#endif
Animation timeline
C code
View on GitHub#include "../lv_examples.h"
#if LV_USE_FLEX && LV_BUILD_EXAMPLES
static const int32_t obj_width = 90;
static const int32_t obj_height = 70;
static void set_width(lv_anim_t * var, int32_t v)
{
lv_obj_set_width(var->var, v);
}
static void set_height(lv_anim_t * var, int32_t v)
{
lv_obj_set_height(var->var, v);
}
static void set_slider_value(lv_anim_t * var, int32_t v)
{
lv_slider_set_value(var->var, v, LV_ANIM_OFF);
}
static void btn_start_event_handler(lv_event_t * e)
{
lv_obj_t * btn = lv_event_get_current_target_obj(e);
lv_anim_timeline_t * anim_timeline = lv_event_get_user_data(e);
bool reverse = lv_obj_has_state(btn, LV_STATE_CHECKED);
lv_anim_timeline_set_reverse(anim_timeline, reverse);
lv_anim_timeline_start(anim_timeline);
}
static void btn_pause_event_handler(lv_event_t * e)
{
lv_anim_timeline_t * anim_timeline = lv_event_get_user_data(e);
lv_anim_timeline_pause(anim_timeline);
}
static void slider_prg_event_handler(lv_event_t * e)
{
lv_obj_t * slider = lv_event_get_current_target_obj(e);
lv_anim_timeline_t * anim_timeline = lv_event_get_user_data(e);
int32_t progress = lv_slider_get_value(slider);
lv_anim_timeline_set_progress(anim_timeline, progress);
}
/**
* Create an animation timeline
*/
void lv_example_anim_timeline_1(void)
{
/* Create anim timeline */
lv_anim_timeline_t * anim_timeline = lv_anim_timeline_create();
lv_obj_t * par = lv_screen_active();
lv_obj_set_flex_flow(par, LV_FLEX_FLOW_ROW);
lv_obj_set_flex_align(par, LV_FLEX_ALIGN_SPACE_AROUND, LV_FLEX_ALIGN_CENTER, LV_FLEX_ALIGN_CENTER);
/* create btn_start */
lv_obj_t * btn_start = lv_button_create(par);
lv_obj_add_event_cb(btn_start, btn_start_event_handler, LV_EVENT_VALUE_CHANGED, anim_timeline);
lv_obj_add_flag(btn_start, LV_OBJ_FLAG_IGNORE_LAYOUT);
lv_obj_add_flag(btn_start, LV_OBJ_FLAG_CHECKABLE);
lv_obj_align(btn_start, LV_ALIGN_TOP_MID, -100, 20);
lv_obj_t * label_start = lv_label_create(btn_start);
lv_label_set_text(label_start, "Start");
lv_obj_center(label_start);
/* create btn_pause */
lv_obj_t * btn_pause = lv_button_create(par);
lv_obj_add_event_cb(btn_pause, btn_pause_event_handler, LV_EVENT_CLICKED, anim_timeline);
lv_obj_add_flag(btn_pause, LV_OBJ_FLAG_IGNORE_LAYOUT);
lv_obj_align(btn_pause, LV_ALIGN_TOP_MID, 100, 20);
lv_obj_t * label_pause = lv_label_create(btn_pause);
lv_label_set_text(label_pause, "Pause");
lv_obj_center(label_pause);
/* create slider_prg */
lv_obj_t * slider_prg = lv_slider_create(par);
lv_obj_add_event_cb(slider_prg, slider_prg_event_handler, LV_EVENT_VALUE_CHANGED, anim_timeline);
lv_obj_add_flag(slider_prg, LV_OBJ_FLAG_IGNORE_LAYOUT);
lv_obj_align(slider_prg, LV_ALIGN_BOTTOM_MID, 0, -20);
lv_slider_set_range(slider_prg, 0, LV_ANIM_TIMELINE_PROGRESS_MAX);
/* create 3 objects */
lv_obj_t * obj1 = lv_obj_create(par);
lv_obj_set_size(obj1, obj_width, obj_height);
lv_obj_set_scrollbar_mode(obj1, LV_SCROLLBAR_MODE_OFF);
lv_obj_t * obj2 = lv_obj_create(par);
lv_obj_set_size(obj2, obj_width, obj_height);
lv_obj_set_scrollbar_mode(obj2, LV_SCROLLBAR_MODE_OFF);
lv_obj_t * obj3 = lv_obj_create(par);
lv_obj_set_size(obj3, obj_width, obj_height);
lv_obj_set_scrollbar_mode(obj3, LV_SCROLLBAR_MODE_OFF);
/* anim-slider */
lv_anim_t a_slider;
lv_anim_init(&a_slider);
lv_anim_set_var(&a_slider, slider_prg);
lv_anim_set_values(&a_slider, 0, LV_ANIM_TIMELINE_PROGRESS_MAX);
lv_anim_set_custom_exec_cb(&a_slider, set_slider_value);
lv_anim_set_path_cb(&a_slider, lv_anim_path_linear);
lv_anim_set_duration(&a_slider, 700);
/* anim-obj1 */
lv_anim_t a1;
lv_anim_init(&a1);
lv_anim_set_var(&a1, obj1);
lv_anim_set_values(&a1, 0, obj_width);
lv_anim_set_custom_exec_cb(&a1, set_width);
lv_anim_set_path_cb(&a1, lv_anim_path_overshoot);
lv_anim_set_duration(&a1, 300);
lv_anim_t a2;
lv_anim_init(&a2);
lv_anim_set_var(&a2, obj1);
lv_anim_set_values(&a2, 0, obj_height);
lv_anim_set_custom_exec_cb(&a2, set_height);
lv_anim_set_path_cb(&a2, lv_anim_path_ease_out);
lv_anim_set_duration(&a2, 300);
/* anim-obj2 */
lv_anim_t a3;
lv_anim_init(&a3);
lv_anim_set_var(&a3, obj2);
lv_anim_set_values(&a3, 0, obj_width);
lv_anim_set_custom_exec_cb(&a3, set_width);
lv_anim_set_path_cb(&a3, lv_anim_path_overshoot);
lv_anim_set_duration(&a3, 300);
lv_anim_t a4;
lv_anim_init(&a4);
lv_anim_set_var(&a4, obj2);
lv_anim_set_values(&a4, 0, obj_height);
lv_anim_set_custom_exec_cb(&a4, set_height);
lv_anim_set_path_cb(&a4, lv_anim_path_ease_out);
lv_anim_set_duration(&a4, 300);
/* anim-obj3 */
lv_anim_t a5;
lv_anim_init(&a5);
lv_anim_set_var(&a5, obj3);
lv_anim_set_values(&a5, 0, obj_width);
lv_anim_set_custom_exec_cb(&a5, set_width);
lv_anim_set_path_cb(&a5, lv_anim_path_overshoot);
lv_anim_set_duration(&a5, 300);
lv_anim_t a6;
lv_anim_init(&a6);
lv_anim_set_var(&a6, obj3);
lv_anim_set_values(&a6, 0, obj_height);
lv_anim_set_custom_exec_cb(&a6, set_height);
lv_anim_set_path_cb(&a6, lv_anim_path_ease_out);
lv_anim_set_duration(&a6, 300);
/* add animations to timeline */
lv_anim_timeline_add(anim_timeline, 0, &a_slider);
lv_anim_timeline_add(anim_timeline, 0, &a1);
lv_anim_timeline_add(anim_timeline, 0, &a2);
lv_anim_timeline_add(anim_timeline, 200, &a3);
lv_anim_timeline_add(anim_timeline, 200, &a4);
lv_anim_timeline_add(anim_timeline, 400, &a5);
lv_anim_timeline_add(anim_timeline, 400, &a6);
lv_anim_timeline_set_progress(anim_timeline, LV_ANIM_TIMELINE_PROGRESS_MAX);
}
#endif