Arc (lv_arc)¶
Overview¶
The Arc object draws an arc within start and end angles and with a given thickness.
Angles¶
To set the angles, use the lv_arc_set_angles(arc, start_angle, end_angle)
function. The zero degree is at the bottom of the object and the degrees are increasing in a counter-clockwise direction.
The angles should be in [0;360] range.
Notes¶
The width and height of the Arc should be the same.
Currently, the Arc object does not support anti-aliasing.
Styles¶
To set the style of an Arc object, use lv_arc_set_style(arc, LV_ARC_STYLE_MAIN, &style)
line.rounded - make the endpoints rounded (opacity won’t work properly if set to 1)
line.width - the thickness of the arc
line.color - the color of the arc.
body.opa - opacity of the arc.
Example¶
C¶
Simple Arc¶
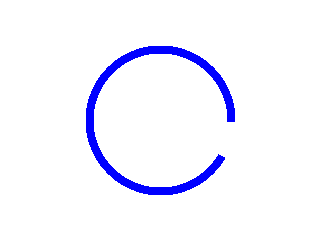
code
#include "lvgl/lvgl.h"
void lv_ex_arc_1(void)
{
/*Create style for the Arcs*/
static lv_style_t style;
lv_style_copy(&style, &lv_style_plain);
style.line.color = LV_COLOR_BLUE; /*Arc color*/
style.line.width = 8; /*Arc width*/
/*Create an Arc*/
lv_obj_t * arc = lv_arc_create(lv_scr_act(), NULL);
lv_arc_set_style(arc, LV_ARC_STYLE_MAIN, &style); /*Use the new style*/
lv_arc_set_angles(arc, 90, 60);
lv_obj_set_size(arc, 150, 150);
lv_obj_align(arc, NULL, LV_ALIGN_CENTER, 0, 0);
}
Loader with Arc¶
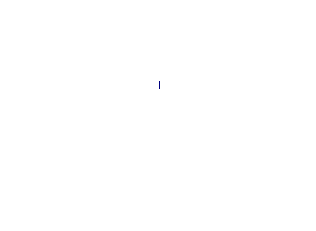
code
#include "lvgl/lvgl.h"
/**
* An `lv_task` to call periodically to set the angles of the arc
* @param t
*/
static void arc_loader(lv_task_t * t)
{
static int16_t a = 0;
a+=5;
if(a >= 359) a = 359;
if(a < 180) lv_arc_set_angles(t->user_data, 180-a ,180);
else lv_arc_set_angles(t->user_data, 540-a ,180);
if(a == 359) {
lv_task_del(t);
return;
}
}
/**
* Create an arc which acts as a loader.
*/
void lv_ex_arc_2(void)
{
/*Create style for the Arcs*/
static lv_style_t style;
lv_style_copy(&style, &lv_style_plain);
style.line.color = LV_COLOR_NAVY; /*Arc color*/
style.line.width = 8; /*Arc width*/
/*Create an Arc*/
lv_obj_t * arc = lv_arc_create(lv_scr_act(), NULL);
lv_arc_set_angles(arc, 180, 180);
lv_arc_set_style(arc, LV_ARC_STYLE_MAIN, &style);
lv_obj_align(arc, NULL, LV_ALIGN_CENTER, 0, 0);
/* Create an `lv_task` to update the arc.
* Store the `arc` in the user data*/
lv_task_create(arc_loader, 20, LV_TASK_PRIO_LOWEST, arc);
}
MicroPython¶
Simple Arc¶
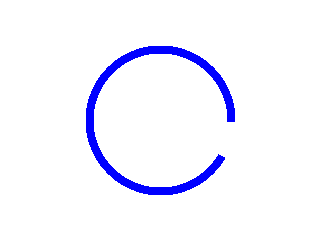
code
# Create style for the Arcs
style = lv.style_t()
lv.style_copy(style, lv.style_plain)
style.line.color = lv.color_make(0,0,255) # Arc color
style.line.width = 8 # Arc width
# Create an Arc
arc = lv.arc(lv.scr_act())
arc.set_style(lv.arc.STYLE.MAIN, style) # Use the new style
arc.set_angles(90, 60)
arc.set_size(150, 150)
arc.align(None, lv.ALIGN.CENTER, 0, 0)
Loader with Arc¶
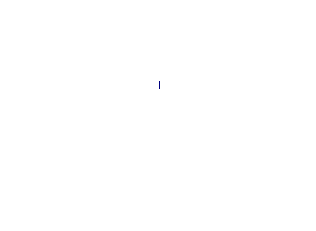
code
# Create an arc which acts as a loader.
class loader_arc(lv.arc):
def __init__(self, parent, color=lv.color_hex(0x000080),
width=8, style=lv.style_plain, rate=20):
super().__init__(parent)
self.a = 0
self.rate = rate
# Create style for the Arcs
self.style = lv.style_t()
lv.style_copy(self.style, style)
self.style.line.color = color
self.style.line.width = width
# Create an Arc
self.set_angles(180, 180);
self.set_style(self.STYLE.MAIN, self.style);
# Spin the Arc
self.spin()
def spin(self):
# Create an `lv_task` to update the arc.
lv.task_create(self.task_cb, self.rate, lv.TASK_PRIO.LOWEST, {})
# An `lv_task` to call periodically to set the angles of the arc
def task_cb(self, task):
self.a+=5;
if self.a >= 359: self.a = 359
if self.a < 180: self.set_angles(180-self.a, 180)
else: self.set_angles(540-self.a, 180)
if self.a == 359:
self.a = 0
lv.task_del(task)
# Create a loader arc
loader_arc = loader_arc(lv.scr_act())
loader_arc.align(None, lv.ALIGN.CENTER, 0, 0)
API¶
Typedefs
-
typedef uint8_t
lv_arc_style_t
¶
Functions
-
lv_obj_t *
lv_arc_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a arc objects
- Return
pointer to the created arc
- Parameters
par
: pointer to an object, it will be the parent of the new arccopy
: pointer to a arc object, if not NULL then the new object will be copied from it
-
void
lv_arc_set_angles
(lv_obj_t *arc, uint16_t start, uint16_t end)¶ Set the start and end angles of an arc. 0 deg: bottom, 90 deg: right etc.
- Parameters
arc
: pointer to an arc objectstart
: the start angle [0..360]end
: the end angle [0..360]
-
void
lv_arc_set_style
(lv_obj_t *arc, lv_arc_style_t type, const lv_style_t *style)¶ Set a style of a arc.
- Parameters
arc
: pointer to arc objecttype
: which style should be setstyle
: pointer to a style
-
uint16_t
lv_arc_get_angle_start
(lv_obj_t *arc)¶ Get the start angle of an arc.
- Return
the start angle [0..360]
- Parameters
arc
: pointer to an arc object
-
uint16_t
lv_arc_get_angle_end
(lv_obj_t *arc)¶ Get the end angle of an arc.
- Return
the end angle [0..360]
- Parameters
arc
: pointer to an arc object
-
const lv_style_t *
lv_arc_get_style
(const lv_obj_t *arc, lv_arc_style_t type)¶ Get style of a arc.
- Return
style pointer to the style
- Parameters
arc
: pointer to arc objecttype
: which style should be get
-
struct
lv_arc_ext_t
¶