Slider (lv_slider)¶
Overview¶
The Slider object looks like a Bar supplemented with a knob. The knob can be dragged to set a value. The Slider also can be vertical or horizontal.
Value and range¶
To set an initial value use lv_slider_set_value(slider, new_value, LV_ANIM_ON/OFF)
.
lv_slider_set_anim_time(slider, anim_time)
sets the animation time in milliseconds.
To specify the range (min, max values) the lv_slider_set_range(slider, min , max)
can be used.
Symmetrical¶
The slider can be drawn symmetrical to zero (drawn from zero, left to right), if it’s enabled with lv_slider_set_sym(slider, true)
Knob placement¶
The knob can be placed in two ways:
inside the background
on the edges on min/max values
Use the lv_slider_set_knob_in(slider, true/false)
to choose between the modes. (knob_in = false is the default)
Styles¶
You can modify the slider’s styles with lv_slider_set_style(slider, LV_SLIDER_STYLE_..., &style)
.
LV_SLIDER_STYLE_BG Style of the background. All
style.body
properties are used. Thepadding
values make the knob larger than the background. (negative value makes is larger)LV_SLIDER_STYLE_INDIC Style of the indicator. All
style.body
properties are used. Thepadding
values make the indicator smaller than the background.LV_SLIDER_STYLE_KNOB Style of the knob. All
style.body
properties are used exceptpadding
.
Events¶
Besides the Generic events the following Special events are sent by the Slider:
LV_EVENT_VALUE_CHANGED Sent while the slider is being dragged or changed with keys.
Keys¶
LV_KEY_UP, LV_KEY_RIGHT Increment the slider’s value by 1
LV_KEY_DOWN, LV_KEY_LEFT Decrement the slider’s value by 1
Learn more about Keys.
Example¶
C¶
Slider with custo mstyle¶
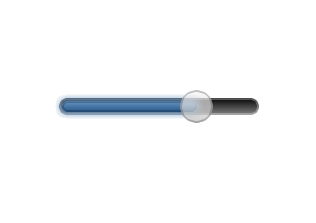
code
#include "lvgl/lvgl.h"
#include <stdio.h>
static void event_handler(lv_obj_t * obj, lv_event_t event)
{
if(event == LV_EVENT_VALUE_CHANGED) {
printf("Value: %d\n", lv_slider_get_value(obj));
}
}
void lv_ex_slider_1(void)
{
/*Create styles*/
static lv_style_t style_bg;
static lv_style_t style_indic;
static lv_style_t style_knob;
lv_style_copy(&style_bg, &lv_style_pretty);
style_bg.body.main_color = LV_COLOR_BLACK;
style_bg.body.grad_color = LV_COLOR_GRAY;
style_bg.body.radius = LV_RADIUS_CIRCLE;
style_bg.body.border.color = LV_COLOR_WHITE;
lv_style_copy(&style_indic, &lv_style_pretty_color);
style_indic.body.radius = LV_RADIUS_CIRCLE;
style_indic.body.shadow.width = 8;
style_indic.body.shadow.color = style_indic.body.main_color;
style_indic.body.padding.left = 3;
style_indic.body.padding.right = 3;
style_indic.body.padding.top = 3;
style_indic.body.padding.bottom = 3;
lv_style_copy(&style_knob, &lv_style_pretty);
style_knob.body.radius = LV_RADIUS_CIRCLE;
style_knob.body.opa = LV_OPA_70;
style_knob.body.padding.top = 10 ;
style_knob.body.padding.bottom = 10 ;
/*Create a slider*/
lv_obj_t * slider = lv_slider_create(lv_scr_act(), NULL);
lv_slider_set_style(slider, LV_SLIDER_STYLE_BG, &style_bg);
lv_slider_set_style(slider, LV_SLIDER_STYLE_INDIC,&style_indic);
lv_slider_set_style(slider, LV_SLIDER_STYLE_KNOB, &style_knob);
lv_obj_align(slider, NULL, LV_ALIGN_CENTER, 0, 0);
lv_obj_set_event_cb(slider, event_handler);
}
Set value with slider¶
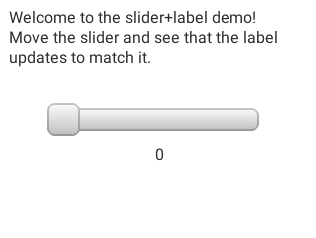
code
/**
* @file lv_ex_slider_2.c
*
*/
/*********************
* INCLUDES
*********************/
#include "lvgl/lvgl.h"
#include <stdio.h>
/*********************
* DEFINES
*********************/
/**********************
* TYPEDEFS
**********************/
/**********************
* STATIC PROTOTYPES
**********************/
static void slider_event_cb(lv_obj_t * slider, lv_event_t event);
/**********************
* STATIC VARIABLES
**********************/
static lv_obj_t * slider_label;
/**********************
* MACROS
**********************/
/**********************
* GLOBAL FUNCTIONS
**********************/
void lv_ex_slider_2(void)
{
/* Create a slider in the center of the display */
lv_obj_t * slider = lv_slider_create(lv_scr_act(), NULL);
lv_obj_set_width(slider, LV_DPI * 2);
lv_obj_align(slider, NULL, LV_ALIGN_CENTER, 0, 0);
lv_obj_set_event_cb(slider, slider_event_cb);
lv_slider_set_range(slider, 0, 100);
/* Create a label below the slider */
slider_label = lv_label_create(lv_scr_act(), NULL);
lv_label_set_text(slider_label, "0");
lv_obj_set_auto_realign(slider_label, true);
lv_obj_align(slider_label, slider, LV_ALIGN_OUT_BOTTOM_MID, 0, 10);
/* Create an informative label */
lv_obj_t * info = lv_label_create(lv_scr_act(), NULL);
lv_label_set_text(info, "Welcome to the slider+label demo!\n"
"Move the slider and see that the label\n"
"updates to match it.");
lv_obj_align(info, NULL, LV_ALIGN_IN_TOP_LEFT, 10, 10);
}
/**********************
* STATIC FUNCTIONS
**********************/
static void slider_event_cb(lv_obj_t * slider, lv_event_t event)
{
if(event == LV_EVENT_VALUE_CHANGED) {
static char buf[4]; /* max 3 bytes for number plus 1 null terminating byte */
snprintf(buf, 4, "%u", lv_slider_get_value(slider));
lv_label_set_text(slider_label, buf);
}
}
MicroPython¶
Slider with custo mstyle¶
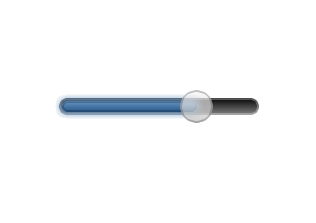
code
def event_handler(obj, event):
if event == lv.EVENT.VALUE_CHANGED:
print("Value: %d" % obj.get_value())
# Create styles
style_bg = lv.style_t()
style_indic = lv.style_t()
style_knob = lv.style_t()
lv.style_copy(style_bg, lv.style_pretty)
style_bg.body.main_color = lv.color_make(0,0,0)
style_bg.body.grad_color = lv.color_make(0x80, 0x80, 0x80)
style_bg.body.radius = 800 # large enough to make a circle
style_bg.body.border.color = lv.color_make(0xff,0xff,0xff)
lv.style_copy(style_indic, lv.style_pretty_color)
style_indic.body.radius = 800
style_indic.body.shadow.width = 8
style_indic.body.shadow.color = style_indic.body.main_color
style_indic.body.padding.left = 3
style_indic.body.padding.right = 3
style_indic.body.padding.top = 3
style_indic.body.padding.bottom = 3
lv.style_copy(style_knob, lv.style_pretty)
style_knob.body.radius = 800
style_knob.body.opa = lv.OPA._70
style_knob.body.padding.top = 10
style_knob.body.padding.bottom = 10
# Create a slider
slider = lv.slider(lv.scr_act())
slider.set_style(lv.slider.STYLE.BG, style_bg)
slider.set_style(lv.slider.STYLE.INDIC, style_indic)
slider.set_style(lv.slider.STYLE.KNOB, style_knob)
slider.align(None, lv.ALIGN.CENTER, 0, 0)
slider.set_event_cb(event_handler)
Set value with slider¶
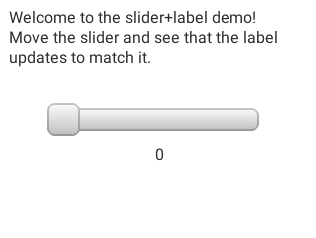
code
def slider_event_cb(slider, event):
if event == lv.EVENT.VALUE_CHANGED:
slider_label.set_text("%u" % slider.get_value())
# Create a slider in the center of the display
slider = lv.slider(lv.scr_act())
slider.set_width(200)
slider.align(None, lv.ALIGN.CENTER, 0, 0)
slider.set_event_cb(slider_event_cb)
slider.set_range(0, 100)
# Create a label below the slider
slider_label = lv.label(lv.scr_act())
slider_label.set_text("0")
slider_label.set_auto_realign(True)
slider_label.align(slider, lv.ALIGN.OUT_BOTTOM_MID, 0, 10)
# Create an informative label
info = lv.label(lv.scr_act())
info.set_text("""Welcome to the slider+label demo!
Move the slider and see that the label
updates to match it.""")
info.align(None, lv.ALIGN.IN_TOP_LEFT, 10, 10)
API¶
Typedefs
-
typedef uint8_t
lv_slider_style_t
¶
Enums
Functions
-
lv_obj_t *
lv_slider_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a slider objects
- Return
pointer to the created slider
- Parameters
par
: pointer to an object, it will be the parent of the new slidercopy
: pointer to a slider object, if not NULL then the new object will be copied from it
-
void
lv_slider_set_value
(lv_obj_t *slider, int16_t value, lv_anim_enable_t anim)¶ Set a new value on the slider
- Parameters
slider
: pointer to a slider objectvalue
: new valueanim
: LV_ANIM_ON: set the value with an animation; LV_ANIM_OFF: change the value immediately
-
void
lv_slider_set_range
(lv_obj_t *slider, int16_t min, int16_t max)¶ Set minimum and the maximum values of a bar
- Parameters
slider
: pointer to the slider objectmin
: minimum valuemax
: maximum value
-
void
lv_slider_set_anim_time
(lv_obj_t *slider, uint16_t anim_time)¶ Make the slider symmetric to zero. The indicator will grow from zero instead of the minimum position.
- Parameters
slider
: pointer to a slider objecten
: true: enable disable symmetric behavior; false: disable
-
void
lv_slider_set_sym
(lv_obj_t *slider, bool en)¶ Set the animation time of the slider
- Parameters
slider
: pointer to a bar objectanim_time
: the animation time in milliseconds.
-
void
lv_slider_set_knob_in
(lv_obj_t *slider, bool in)¶ Set the ‘knob in’ attribute of a slider
- Parameters
slider
: pointer to slider objectin
: true: the knob is drawn always in the slider; false: the knob can be out on the edges
-
void
lv_slider_set_style
(lv_obj_t *slider, lv_slider_style_t type, const lv_style_t *style)¶ Set a style of a slider
- Parameters
slider
: pointer to a slider objecttype
: which style should be setstyle
: pointer to a style
-
int16_t
lv_slider_get_value
(const lv_obj_t *slider)¶ Get the value of a slider
- Return
the value of the slider
- Parameters
slider
: pointer to a slider object
-
int16_t
lv_slider_get_min_value
(const lv_obj_t *slider)¶ Get the minimum value of a slider
- Return
the minimum value of the slider
- Parameters
slider
: pointer to a slider object
-
int16_t
lv_slider_get_max_value
(const lv_obj_t *slider)¶ Get the maximum value of a slider
- Return
the maximum value of the slider
- Parameters
slider
: pointer to a slider object
-
bool
lv_slider_is_dragged
(const lv_obj_t *slider)¶ Give the slider is being dragged or not
- Return
true: drag in progress false: not dragged
- Parameters
slider
: pointer to a slider object
-
uint16_t
lv_slider_get_anim_time
(lv_obj_t *slider)¶ Get the animation time of the slider
- Return
the animation time in milliseconds.
- Parameters
slider
: pointer to a slider object
-
bool
lv_slider_get_sym
(lv_obj_t *slider)¶ Get whether the slider is symmetric or not.
- Return
true: symmetric is enabled; false: disable
- Parameters
slider
: pointer to a bar object
-
bool
lv_slider_get_knob_in
(const lv_obj_t *slider)¶ Get the ‘knob in’ attribute of a slider
- Return
true: the knob is drawn always in the slider; false: the knob can be out on the edges
- Parameters
slider
: pointer to slider object
-
const lv_style_t *
lv_slider_get_style
(const lv_obj_t *slider, lv_slider_style_t type)¶ Get a style of a slider
- Return
style pointer to a style
- Parameters
slider
: pointer to a slider objecttype
: which style should be get
-
struct
lv_slider_ext_t
¶