Roller (lv_roller)¶
Overview¶
Roller allows you to simply select one option from more with scrolling. Its functionalities are similar to Drop down list.
Set options¶
The options are passed to the Roller as a string with lv_roller_set_options(roller, options, LV_ROLLER_MODE_NORMAL/INFINITE)
. The options should be separated by \n
. For example: "First\nSecond\nThird"
.
LV_ROLLER_MODE_INIFINITE
make the roller circular.
You can select an option manually with lv_roller_set_selected(roller, id)
, where id is the index of an option.
Get selected option¶
The get the currently selected option use lv_roller_get_selected(roller)
it will return the index of the selected option.
lv_roller_get_selected_str(roller, buf, buf_size)
copy the name of the selected option to buf
.
Align the options¶
To align the label horizontally use lv_roller_set_align(roller, LV_LABEL_ALIGN_LEFT/CENTER/RIGHT)
.
Height and width¶
You can set the number of visible rows with lv_roller_set_visible_row_count(roller, num)
The width is adjusted automatically according to the width of the options. To prevent this apply lv_roller_set_fix_width(roller, width)
. 0
means to use auto width.
Animation time¶
When the Roller is scrolled and doesn’t stop exactly on an option it will scroll to the nearest valid option automatically.
The time of this scroll animation can be changed by lv_roller_set_anim_time(roller, anim_time)
. Zero animation time means no animation.
Styles¶
The lv_roller_set_style(roller, LV_ROLLER_STYLE_..., &style)
set the styles of a Roller.
LV_ROLLER_STYLE_BG Style of the background. All
style.body
properties are used.style.text
is used for the option’s label. Default:lv_style_pretty
LV_ROLLER_STYLE_SEL Style of the selected option. The
style.body
properties are used. The selected option will be recolored withtext.color
. Default:lv_style_plain_color
Events¶
Besides, the Generic events the following Special events are sent by the Drop down lists:
LV_EVENT_VALUE_CHANGED sent when a new option is selected
Learn more about Events.
Keys¶
The following Keys are processed by the Buttons:
LV_KEY_RIGHT/DOWN Select the next option
LV_KEY_LEFT/UP Select the previous option
LY_KEY_ENTER Apply the selected option (Send
LV_EVENT_VALUE_CHANGED
event)
Example¶
C¶
Simple Roller¶
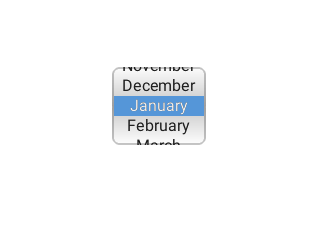
code
#include "lvgl/lvgl.h"
#include <stdio.h>
static void event_handler(lv_obj_t * obj, lv_event_t event)
{
if(event == LV_EVENT_VALUE_CHANGED) {
char buf[32];
lv_roller_get_selected_str(obj, buf, sizeof(buf));
printf("Selected month: %s\n", buf);
}
}
void lv_ex_roller_1(void)
{
lv_obj_t *roller1 = lv_roller_create(lv_scr_act(), NULL);
lv_roller_set_options(roller1,
"January\n"
"February\n"
"March\n"
"April\n"
"May\n"
"June\n"
"July\n"
"August\n"
"September\n"
"October\n"
"November\n"
"December",
LV_ROLLER_MODE_INIFINITE);
lv_roller_set_visible_row_count(roller1, 4);
lv_obj_align(roller1, NULL, LV_ALIGN_CENTER, 0, 0);
lv_obj_set_event_cb(roller1, event_handler);
}
MicroPython¶
Simple Roller¶
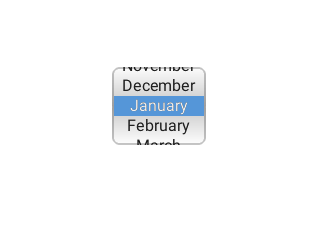
code
def event_handler(obj, event):
if event == lv.EVENT.VALUE_CHANGED:
option = " "*10
obj.get_selected_str(option, len(option))
print("Selected month: %s" % option.strip())
roller1 = lv.roller(lv.scr_act())
roller1.set_options("\n".join([
"January",
"February",
"March",
"April",
"May",
"June",
"July",
"August",
"September",
"October",
"November",
"December"]), lv.roller.MODE.INIFINITE)
roller1.set_visible_row_count(4)
roller1.align(None, lv.ALIGN.CENTER, 0, 0)
roller1.set_event_cb(event_handler)
API¶
Enums
Functions
-
lv_obj_t *
lv_roller_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a roller object
- Return
pointer to the created roller
- Parameters
par
: pointer to an object, it will be the parent of the new rollercopy
: pointer to a roller object, if not NULL then the new object will be copied from it
-
void
lv_roller_set_options
(lv_obj_t *roller, const char *options, lv_roller_mode_t mode)¶ Set the options on a roller
- Parameters
roller
: pointer to roller objectoptions
: a string with ‘’ separated options. E.g. “One\nTwo\nThree”
mode
:LV_ROLLER_MODE_NORMAL
orLV_ROLLER_MODE_INFINITE
-
void
lv_roller_set_align
(lv_obj_t *roller, lv_label_align_t align)¶ Set the align of the roller’s options (left, right or center[default])
- Parameters
roller
: - pointer to a roller objectalign
: - one of lv_label_align_t values (left, right, center)
-
void
lv_roller_set_selected
(lv_obj_t *roller, uint16_t sel_opt, lv_anim_enable_t anim)¶ Set the selected option
- Parameters
roller
: pointer to a roller objectsel_opt
: id of the selected option (0 … number of option - 1);anim
: LV_ANOM_ON: set with animation; LV_ANIM_OFF set immediately
-
void
lv_roller_set_visible_row_count
(lv_obj_t *roller, uint8_t row_cnt)¶ Set the height to show the given number of rows (options)
- Parameters
roller
: pointer to a roller objectrow_cnt
: number of desired visible rows
-
void
lv_roller_set_fix_width
(lv_obj_t *roller, lv_coord_t w)¶ Set a fix width for the drop down list
- Parameters
roller
: pointer to a roller obejctw
: the width when the list is opened (0: auto size)
-
void
lv_roller_set_anim_time
(lv_obj_t *roller, uint16_t anim_time)¶ Set the open/close animation time.
- Parameters
roller
: pointer to a roller objectanim_time
: open/close animation time [ms]
-
void
lv_roller_set_style
(lv_obj_t *roller, lv_roller_style_t type, const lv_style_t *style)¶ Set a style of a roller
- Parameters
roller
: pointer to a roller objecttype
: which style should be setstyle
: pointer to a style
-
uint16_t
lv_roller_get_selected
(const lv_obj_t *roller)¶ Get the id of the selected option
- Return
id of the selected option (0 … number of option - 1);
- Parameters
roller
: pointer to a roller object
-
void
lv_roller_get_selected_str
(const lv_obj_t *roller, char *buf, uint16_t buf_size)¶ Get the current selected option as a string
- Parameters
roller
: pointer to roller objectbuf
: pointer to an array to store the stringbuf_size
: size ofbuf
in bytes. 0: to ignore it.
-
lv_label_align_t
lv_roller_get_align
(const lv_obj_t *roller)¶ Get the align attribute. Default alignment after _create is LV_LABEL_ALIGN_CENTER
- Return
LV_LABEL_ALIGN_LEFT, LV_LABEL_ALIGN_RIGHT or LV_LABEL_ALIGN_CENTER
- Parameters
roller
: pointer to a roller object
-
const char *
lv_roller_get_options
(const lv_obj_t *roller)¶ Get the options of a roller
- Return
the options separated by ‘
’-s (E.g. “Option1\nOption2\nOption3”)
- Parameters
roller
: pointer to roller object
-
uint16_t
lv_roller_get_anim_time
(const lv_obj_t *roller)¶ Get the open/close animation time.
- Return
open/close animation time [ms]
- Parameters
roller
: pointer to a roller
-
bool
lv_roller_get_hor_fit
(const lv_obj_t *roller)¶ Get the auto width set attribute
- Return
true: auto size enabled; false: manual width settings enabled
- Parameters
roller
: pointer to a roller object
-
const lv_style_t *
lv_roller_get_style
(const lv_obj_t *roller, lv_roller_style_t type)¶ Get a style of a roller
- Return
style pointer to a style
- Parameters
roller
: pointer to a roller objecttype
: which style should be get
-
struct
lv_roller_ext_t
¶