Image button (lv_imgbtn)¶
Overview¶
The Image button is very similar to the simple ‘Button’ object. The only difference is that, it displays user-defined images in each state instead of drawing a button. Before reading this section, please read the Button section for better understanding.
Image sources¶
To set the image in a state, use the lv_imgbtn_set_src(imgbtn, LV_BTN_STATE_..., &img_src)
. The image sources works the same as described in the Image object except that, “Symbols” are not supported by the Image button.
If LV_IMGBTN_TILED
is enabled in lv_conf.h, then three sources can be set for each state:
left
center
right
The center image will be repeated to fill the width of the object. Therefore with LV_IMGBTN_TILED
, you can set the width of the Image button. However, without this option, the width will be always the same as the image source’s width.
States¶
The states also work like with Button object. It can be set with lv_imgbtn_set_state(imgbtn, LV_BTN_STATE_...)
.
Toggle¶
The toggle feature can be enabled with lv_imgbtn_set_toggle(imgbtn, true)
Style usage¶
Similar to normal Buttons, Image buttons also have 5 independent styles for the 5 state. You can set them via: lv_imgbtn_set_style(btn, LV_IMGBTN_STYLE_..., &style)
. The styles use the style.image
properties.
LV_IMGBTN_STYLE_REL - Style of the released state. Default:
lv_style_btn_rel
.LV_IMGBTN_STYLE_PR - Style of the pressed state. Default:
lv_style_btn_pr
.LV_IMGBTN_STYLE_TGL_REL - Style of the toggled released state. Default:
lv_style_btn_tgl_rel
.LV_IMGBTN_STYLE_TGL_PR - Style of the toggled pressed state. Default:
lv_style_btn_tgl_pr
.LV_IMGBTN_STYLE_INA - Style of the inactive state. Default:
lv_style_btn_ina
.
When labels are created on a button, it’s a good practice to set the image button’s style.text
properties too. Because labels have style = NULL
, by default, they inherit the parent’s (image button) style.
Hence you don’t need to create a new style for the label.
Events¶
Beside the Generic events, the following Special events are sent by the buttons:
LV_EVENT_VALUE_CHANGED - Sent when the button is toggled.
Note that, the generic input device related events (like LV_EVENT_PRESSED
) are sent in the inactive state too. You need to check the state with lv_btn_get_state(btn)
to ignore the events from inactive buttons.
Learn more about Events.
Keys¶
The following Keys are processed by the Buttons:
LV_KEY_RIGHT/UP - Go to toggled state if toggling is enabled.
LV_KEY_LEFT/DOWN - Go to non-toggled state if toggling is enabled.
Note that, as usual, the state of LV_KEY_ENTER
is translated to LV_EVENT_PRESSED/PRESSING/RELEASED
etc.
Learn more about Keys.
Example¶
C¶
Simple Image button¶
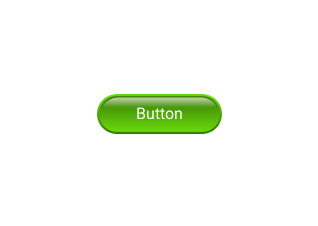
code
#include "lvgl/lvgl.h"
void lv_ex_imgbtn_1(void)
{
static lv_style_t style_pr;
lv_style_copy(&style_pr, &lv_style_plain);
style_pr.image.color = LV_COLOR_BLACK;
style_pr.image.intense = LV_OPA_50;
style_pr.text.color = lv_color_hex3(0xaaa);
LV_IMG_DECLARE(imgbtn_green);
LV_IMG_DECLARE(imgbtn_blue);
/*Create an Image button*/
lv_obj_t * imgbtn1 = lv_imgbtn_create(lv_scr_act(), NULL);
lv_imgbtn_set_src(imgbtn1, LV_BTN_STATE_REL, &imgbtn_green);
lv_imgbtn_set_src(imgbtn1, LV_BTN_STATE_PR, &imgbtn_green);
lv_imgbtn_set_src(imgbtn1, LV_BTN_STATE_TGL_REL, &imgbtn_blue);
lv_imgbtn_set_src(imgbtn1, LV_BTN_STATE_TGL_PR, &imgbtn_blue);
lv_imgbtn_set_style(imgbtn1, LV_BTN_STATE_PR, &style_pr); /*Use the darker style in the pressed state*/
lv_imgbtn_set_style(imgbtn1, LV_BTN_STATE_TGL_PR, &style_pr);
lv_imgbtn_set_toggle(imgbtn1, true);
lv_obj_align(imgbtn1, NULL, LV_ALIGN_CENTER, 0, -40);
/*Create a label on the Image button*/
lv_obj_t * label = lv_label_create(imgbtn1, NULL);
lv_label_set_text(label, "Button");
}
MicroPython¶
No examples yet.
API¶
Typedefs
-
typedef uint8_t
lv_imgbtn_style_t
¶
Enums
Functions
-
lv_obj_t *
lv_imgbtn_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a image button objects
- Return
pointer to the created image button
- Parameters
par
: pointer to an object, it will be the parent of the new image buttoncopy
: pointer to a image button object, if not NULL then the new object will be copied from it
-
void
lv_imgbtn_set_src
(lv_obj_t *imgbtn, lv_btn_state_t state, const void *src)¶ Set images for a state of the image button
- Parameters
imgbtn
: pointer to an image button objectstate
: for which state set the new image (fromlv_btn_state_t
) `src
: pointer to an image source (a C array or path to a file)
-
void
lv_imgbtn_set_src
(lv_obj_t *imgbtn, lv_btn_state_t state, const void *src_left, const void *src_mid, const void *src_right)¶ Set images for a state of the image button
- Parameters
imgbtn
: pointer to an image button objectstate
: for which state set the new image (fromlv_btn_state_t
) `src_left
: pointer to an image source for the left side of the button (a C array or path to a file)src_mid
: pointer to an image source for the middle of the button (ideally 1px wide) (a C array or path to a file)src_right
: pointer to an image source for the right side of the button (a C array or path to a file)
-
void
lv_imgbtn_set_toggle
(lv_obj_t *imgbtn, bool tgl)¶ Enable the toggled states. On release the button will change from/to toggled state.
- Parameters
imgbtn
: pointer to an image button objecttgl
: true: enable toggled states, false: disable
-
void
lv_imgbtn_set_state
(lv_obj_t *imgbtn, lv_btn_state_t state)¶ Set the state of the image button
- Parameters
imgbtn
: pointer to an image button objectstate
: the new state of the button (from lv_btn_state_t enum)
-
void
lv_imgbtn_toggle
(lv_obj_t *imgbtn)¶ Toggle the state of the image button (ON->OFF, OFF->ON)
- Parameters
imgbtn
: pointer to a image button object
-
void
lv_imgbtn_set_style
(lv_obj_t *imgbtn, lv_imgbtn_style_t type, const lv_style_t *style)¶ Set a style of a image button.
- Parameters
imgbtn
: pointer to image button objecttype
: which style should be setstyle
: pointer to a style
-
const void *
lv_imgbtn_get_src
(lv_obj_t *imgbtn, lv_btn_state_t state)¶ Get the images in a given state
- Return
pointer to an image source (a C array or path to a file)
- Parameters
imgbtn
: pointer to an image button objectstate
: the state where to get the image (fromlv_btn_state_t
) `
-
const void *
lv_imgbtn_get_src_left
(lv_obj_t *imgbtn, lv_btn_state_t state)¶ Get the left image in a given state
- Return
pointer to the left image source (a C array or path to a file)
- Parameters
imgbtn
: pointer to an image button objectstate
: the state where to get the image (fromlv_btn_state_t
) `
-
const void *
lv_imgbtn_get_src_middle
(lv_obj_t *imgbtn, lv_btn_state_t state)¶ Get the middle image in a given state
- Return
pointer to the middle image source (a C array or path to a file)
- Parameters
imgbtn
: pointer to an image button objectstate
: the state where to get the image (fromlv_btn_state_t
) `
-
const void *
lv_imgbtn_get_src_right
(lv_obj_t *imgbtn, lv_btn_state_t state)¶ Get the right image in a given state
- Return
pointer to the left image source (a C array or path to a file)
- Parameters
imgbtn
: pointer to an image button objectstate
: the state where to get the image (fromlv_btn_state_t
) `
-
lv_btn_state_t
lv_imgbtn_get_state
(const lv_obj_t *imgbtn)¶ Get the current state of the image button
- Return
the state of the button (from lv_btn_state_t enum)
- Parameters
imgbtn
: pointer to a image button object
-
bool
lv_imgbtn_get_toggle
(const lv_obj_t *imgbtn)¶ Get the toggle enable attribute of the image button
- Return
ture: toggle enabled, false: disabled
- Parameters
imgbtn
: pointer to a image button object
-
const lv_style_t *
lv_imgbtn_get_style
(const lv_obj_t *imgbtn, lv_imgbtn_style_t type)¶ Get style of a image button.
- Return
style pointer to the style
- Parameters
imgbtn
: pointer to image button objecttype
: which style should be get
-
struct
lv_imgbtn_ext_t
¶ Public Members
-
lv_btn_ext_t
btn
¶
-
const void *
img_src
[_LV_BTN_STATE_NUM
]¶
-
const void *
img_src_left
[_LV_BTN_STATE_NUM
]¶
-
const void *
img_src_mid
[_LV_BTN_STATE_NUM
]¶
-
const void *
img_src_right
[_LV_BTN_STATE_NUM
]¶
-
lv_img_cf_t
act_cf
¶
-
lv_btn_ext_t