Drop-down list (lv_ddlist)¶
Overview¶
The drop-down list allows the user to select one value from a list. The drop-down list is closed (inactive) by default. When a drop-down list is inactive, it displays a single value. When activated (by click on the drop-down list), it displays a list of values from which the user may select one. When the user selects a new value, the drop-down list reverts to the inactive state and displays the new value.
Set options¶
The options are passed to the drop-down list as a string with lv_ddlist_set_options(ddlist, options)
. The options should be separated by \n
. For example: "First\nSecond\nThird"
.
You can select an option manually with lv_ddlist_set_selected(ddlist, id)
, where id is the index of an option.
Get selected option¶
The get the currently selected option, use lv_ddlist_get_selected(ddlist)
. It will return the index of the selected option.
lv_ddlist_get_selected_str(ddlist, buf, buf_size)
copies the name of the selected option to a buf
.
Align the options¶
To align the label horizontally, use lv_ddlist_set_align(ddlist, LV_LABEL_ALIGN_LEFT/CENTER/RIGHT)
.
Height and width¶
By default, the list’s height is adjusted automatically to show all options. The lv_ddlist_set_fix_height(ddlist, height)
sets a fixed height for the opened list. The user can put 0
to use auto height.
The width is also adjusted automatically. To prevent this, apply lv_ddlist_set_fix_width(ddlist, width)
. The user can put 0
to use auto width.
Scrollbars¶
Similarly to Page with fix height, the drop-down list supports various scrollbar display modes. It can be set by lv_ddlist_set_sb_mode(ddlist, LV_SB_MODE_...)
.
Animation time¶
The drop-down list’s open/close animation time is adjusted by lv_ddlist_set_anim_time(ddlist, anim_time)
. Zero animation time means no animation.
Decoration arrow¶
A down arrow can be added to the left side of the drop-down list with lv_ddlist_set_draw_arrow(ddlist, true)
.
Manually open/close¶
To manually open or close the drop-down list the lv_ddlist_open/close(ddlist)
function can be used.
Stay open¶
You can force the drop-down list to stay opened, when an option is selected with lv_ddlist_set_stay_open(ddlist, true)
.
Styles¶
The lv_ddlist_set_style(ddlist, LV_DDLIST_STYLE_..., &style)
set the styles of a drop-down list.
LV_DDLIST_STYLE_BG - Style of the background. All
style.body
properties are used.style.text
is used for the option’s label. Default:lv_style_pretty
.LV_DDLIST_STYLE_SEL - Style of the selected option. The
style.body
properties are used. The selected option will be recolored withtext.color
. Default:lv_style_plain_color
.LV_DDLIST_STYLE_SB - Style of the scrollbar. The
style.body
properties are used. Default:lv_style_plain_color
.
Events¶
Besides the Generic events, the following Special events are sent by the drop-down list:
LV_EVENT_VALUE_CHANGED - Sent when the new option is selected.
Learn more about Events.
Keys¶
The following Keys are processed by the Buttons:
LV_KEY_RIGHT/DOWN - Select the next option.
LV_KEY_LEFT/UP - Select the previous option.
LY_KEY_ENTER - Apply the selected option (Send
LV_EVENT_VALUE_CHANGED
event and close the drop-down list).
Example¶
C¶
Simple Drop down list¶
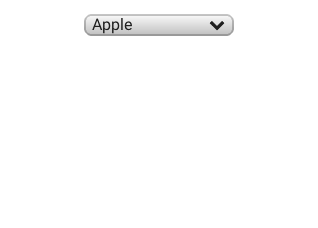
code
#include "lvgl/lvgl.h"
#include <stdio.h>
static void event_handler(lv_obj_t * obj, lv_event_t event)
{
if(event == LV_EVENT_VALUE_CHANGED) {
char buf[32];
lv_ddlist_get_selected_str(obj, buf, sizeof(buf));
printf("Option: %s\n", buf);
}
}
void lv_ex_ddlist_1(void)
{
/*Create a drop down list*/
lv_obj_t * ddlist = lv_ddlist_create(lv_scr_act(), NULL);
lv_ddlist_set_options(ddlist, "Apple\n"
"Banana\n"
"Orange\n"
"Melon\n"
"Grape\n"
"Raspberry");
lv_ddlist_set_fix_width(ddlist, 150);
lv_ddlist_set_draw_arrow(ddlist, true);
lv_obj_align(ddlist, NULL, LV_ALIGN_IN_TOP_MID, 0, 20);
lv_obj_set_event_cb(ddlist, event_handler);
}
Drop “up” list¶
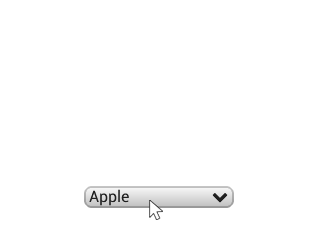
code
#include "lvgl/lvgl.h"
#include <stdio.h>
/**
* Create a drop UP list by applying auto realign
*/
void lv_ex_ddlist_2(void)
{
/*Create a drop down list*/
lv_obj_t * ddlist = lv_ddlist_create(lv_scr_act(), NULL);
lv_ddlist_set_options(ddlist, "Apple\n"
"Banana\n"
"Orange\n"
"Melon\n"
"Grape\n"
"Raspberry");
lv_ddlist_set_fix_width(ddlist, 150);
lv_ddlist_set_fix_height(ddlist, 150);
lv_ddlist_set_draw_arrow(ddlist, true);
/* Enable auto-realign when the size changes.
* It will keep the bottom of the ddlist fixed*/
lv_obj_set_auto_realign(ddlist, true);
/*It will be called automatically when the size changes*/
lv_obj_align(ddlist, NULL, LV_ALIGN_IN_BOTTOM_MID, 0, -20);
}
MicroPython¶
Simple Drop down list¶
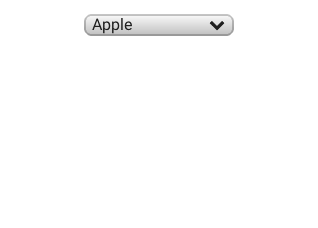
code
def event_handler(obj, event):
if event == lv.EVENT.VALUE_CHANGED:
option = " "*10 # should be large enough to store the option
obj.get_selected_str(option, len(option))
# .strip() removes trailing spaces
print("Option: \"%s\"" % option.strip())
# Create a drop down list
ddlist = lv.ddlist(lv.scr_act())
ddlist.set_options("\n".join([
"Apple",
"Banana",
"Orange",
"Melon",
"Grape",
"Raspberry"]))
ddlist.set_fix_width(150)
ddlist.set_draw_arrow(True)
ddlist.align(None, lv.ALIGN.IN_TOP_MID, 0, 20)
ddlist.set_event_cb(event_handler)
Drop “up” list¶
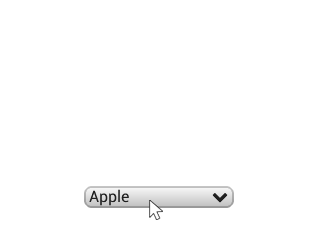
code
# Create a drop UP list by applying auto realign
# Create a drop down list
ddlist = lv.ddlist(lv.scr_act())
ddlist.set_options("\n".join([
"Apple",
"Banana",
"Orange",
"Melon",
"Grape",
"Raspberry"]))
ddlist.set_fix_width(150)
ddlist.set_fix_height(150)
ddlist.set_draw_arrow(True)
# Enable auto-realign when the size changes.
# It will keep the bottom of the ddlist fixed
ddlist.set_auto_realign(True)
# It will be called automatically when the size changes
ddlist.align(None, lv.ALIGN.IN_BOTTOM_MID, 0, -20)
API¶
Typedefs
-
typedef uint8_t
lv_ddlist_style_t
¶
Enums
Functions
-
lv_obj_t *
lv_ddlist_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a drop down list objects
- Return
pointer to the created drop down list
- Parameters
par
: pointer to an object, it will be the parent of the new drop down listcopy
: pointer to a drop down list object, if not NULL then the new object will be copied from it
-
void
lv_ddlist_set_options
(lv_obj_t *ddlist, const char *options)¶ Set the options in a drop down list from a string
- Parameters
ddlist
: pointer to drop down list objectoptions
: a string with ‘’ separated options. E.g. “One\nTwo\nThree”
-
void
lv_ddlist_set_selected
(lv_obj_t *ddlist, uint16_t sel_opt)¶ Set the selected option
- Parameters
ddlist
: pointer to drop down list objectsel_opt
: id of the selected option (0 … number of option - 1);
-
void
lv_ddlist_set_fix_height
(lv_obj_t *ddlist, lv_coord_t h)¶ Set a fix height for the drop down list If 0 then the opened ddlist will be auto. sized else the set height will be applied.
- Parameters
ddlist
: pointer to a drop down listh
: the height when the list is opened (0: auto size)
-
void
lv_ddlist_set_fix_width
(lv_obj_t *ddlist, lv_coord_t w)¶ Set a fix width for the drop down list
- Parameters
ddlist
: pointer to a drop down listw
: the width when the list is opened (0: auto size)
-
void
lv_ddlist_set_draw_arrow
(lv_obj_t *ddlist, bool en)¶ Set arrow draw in a drop down list
- Parameters
ddlist
: pointer to drop down list objecten
: enable/disable a arrow draw. E.g. “true” for draw.
-
void
lv_ddlist_set_stay_open
(lv_obj_t *ddlist, bool en)¶ Leave the list opened when a new value is selected
- Parameters
ddlist
: pointer to drop down list objecten
: enable/disable “stay open” feature
-
void
lv_ddlist_set_sb_mode
(lv_obj_t *ddlist, lv_sb_mode_t mode)¶ Set the scroll bar mode of a drop down list
- Parameters
ddlist
: pointer to a drop down list objectsb_mode
: the new mode from ‘lv_page_sb_mode_t’ enum
-
void
lv_ddlist_set_anim_time
(lv_obj_t *ddlist, uint16_t anim_time)¶ Set the open/close animation time.
- Parameters
ddlist
: pointer to a drop down listanim_time
: open/close animation time [ms]
-
void
lv_ddlist_set_style
(lv_obj_t *ddlist, lv_ddlist_style_t type, const lv_style_t *style)¶ Set a style of a drop down list
- Parameters
ddlist
: pointer to a drop down list objecttype
: which style should be setstyle
: pointer to a style
-
void
lv_ddlist_set_align
(lv_obj_t *ddlist, lv_label_align_t align)¶ Set the alignment of the labels in a drop down list
- Parameters
ddlist
: pointer to a drop down list objectalign
: alignment of labels
-
const char *
lv_ddlist_get_options
(const lv_obj_t *ddlist)¶ Get the options of a drop down list
- Return
the options separated by ‘
’-s (E.g. “Option1\nOption2\nOption3”)
- Parameters
ddlist
: pointer to drop down list object
-
uint16_t
lv_ddlist_get_selected
(const lv_obj_t *ddlist)¶ Get the selected option
- Return
id of the selected option (0 … number of option - 1);
- Parameters
ddlist
: pointer to drop down list object
-
void
lv_ddlist_get_selected_str
(const lv_obj_t *ddlist, char *buf, uint16_t buf_size)¶ Get the current selected option as a string
- Parameters
ddlist
: pointer to ddlist objectbuf
: pointer to an array to store the stringbuf_size
: size ofbuf
in bytes. 0: to ignore it.
-
lv_coord_t
lv_ddlist_get_fix_height
(const lv_obj_t *ddlist)¶ Get the fix height value.
- Return
the height if the ddlist is opened (0: auto size)
- Parameters
ddlist
: pointer to a drop down list object
-
bool
lv_ddlist_get_draw_arrow
(lv_obj_t *ddlist)¶ Get arrow draw in a drop down list
- Parameters
ddlist
: pointer to drop down list object
-
bool
lv_ddlist_get_stay_open
(lv_obj_t *ddlist)¶ Get whether the drop down list stay open after selecting a value or not
- Parameters
ddlist
: pointer to drop down list object
-
lv_sb_mode_t
lv_ddlist_get_sb_mode
(const lv_obj_t *ddlist)¶ Get the scroll bar mode of a drop down list
- Return
scrollbar mode from ‘lv_page_sb_mode_t’ enum
- Parameters
ddlist
: pointer to a drop down list object
-
uint16_t
lv_ddlist_get_anim_time
(const lv_obj_t *ddlist)¶ Get the open/close animation time.
- Return
open/close animation time [ms]
- Parameters
ddlist
: pointer to a drop down list
-
const lv_style_t *
lv_ddlist_get_style
(const lv_obj_t *ddlist, lv_ddlist_style_t type)¶ Get a style of a drop down list
- Return
style pointer to a style
- Parameters
ddlist
: pointer to a drop down list objecttype
: which style should be get
-
lv_label_align_t
lv_ddlist_get_align
(const lv_obj_t *ddlist)¶ Get the alignment of the labels in a drop down list
- Return
alignment of labels
- Parameters
ddlist
: pointer to a drop down list object
-
void
lv_ddlist_open
(lv_obj_t *ddlist, lv_anim_enable_t anim)¶ Open the drop down list with or without animation
- Parameters
ddlist
: pointer to drop down list objectanim_en
: LV_ANIM_ON: use animation; LV_ANOM_OFF: not use animations
-
void
lv_ddlist_close
(lv_obj_t *ddlist, lv_anim_enable_t anim)¶ Close (Collapse) the drop down list
- Parameters
ddlist
: pointer to drop down list objectanim_en
: LV_ANIM_ON: use animation; LV_ANOM_OFF: not use animations
-
struct
lv_ddlist_ext_t
¶