Preloader (lv_preload)¶
Overview¶
The preloader object is a spinning arc over a border.
Arc length¶
The length of the arc can be adjusted by lv_preload_set_arc_length(preload, deg)
.
Spinning speed¶
The speed of the spinning can be adjusted by lv_preload_set_spin_time(preload, time_ms)
.
Spin types¶
You can choose from more spin types:
LV_PRELOAD_TYPE_SPINNING_ARC spin the arc, slow down on the top
LV_PRELOAD_TYPE_FILLSPIN_ARC spin the arc, slow down on the top but also stretch the arc
To apply one if them use lv_preload_set_type(preload, LV_PRELOAD_TYPE_...)
Spin direction¶
The direction of spinning can be changed with lv_preload_set_dir(preload, LV_PRELOAD_DIR_FORWARD/BACKWARD)
.
Styles¶
You can set the styles with lv_preload_set_style(btn, LV_PRELOAD_STYLE_MAIN, &style)
. It describes both the arc and the border style:
arc is described by the
line
propertiesborder is described by the
body.border
properties includingbody.padding.left/top
(the smaller is used) to give a smaller radius for the border.
Events¶
Only the Generic events are sent by the object type.
Example¶
C¶
Preloader with custom style¶
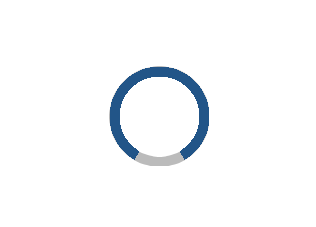
code
#include "lvgl/lvgl.h"
void lv_ex_preload_1(void)
{
/*Create a style for the Preloader*/
static lv_style_t style;
lv_style_copy(&style, &lv_style_plain);
style.line.width = 10; /*10 px thick arc*/
style.line.color = lv_color_hex3(0x258); /*Blueish arc color*/
style.body.border.color = lv_color_hex3(0xBBB); /*Gray background color*/
style.body.border.width = 10;
style.body.padding.left = 0;
/*Create a Preloader object*/
lv_obj_t * preload = lv_preload_create(lv_scr_act(), NULL);
lv_obj_set_size(preload, 100, 100);
lv_obj_align(preload, NULL, LV_ALIGN_CENTER, 0, 0);
lv_preload_set_style(preload, LV_PRELOAD_STYLE_MAIN, &style);
}
MicroPython¶
Preloader with custom style¶
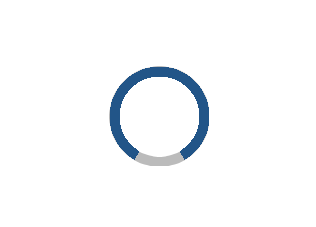
code
# Create a style for the Preloader
style = lv.style_t()
lv.style_copy(style, lv.style_plain)
style.line.width = 10 # 10 px thick arc
style.line.color = lv.color_hex3(0x258) # Blueish arc color
style.body.border.color = lv.color_hex3(0xBBB) # Gray background color
style.body.border.width = 10
style.body.padding.left = 0
# Create a Preloader object
preload = lv.preload(lv.scr_act())
preload.set_size(100, 100)
preload.align(None, lv.ALIGN.CENTER, 0, 0)
preload.set_style(lv.preload.STYLE.MAIN, style)
MicroPython¶
No examples yet.
API¶
Typedefs
-
typedef uint8_t
lv_preload_type_t
¶
-
typedef uint8_t
lv_preload_dir_t
¶
-
typedef uint8_t
lv_preload_style_t
¶
Enums
-
enum [anonymous]¶
Type of preloader.
Values:
-
enumerator
LV_PRELOAD_TYPE_SPINNING_ARC
¶
-
enumerator
LV_PRELOAD_TYPE_FILLSPIN_ARC
¶
-
enumerator
LV_PRELOAD_TYPE_CONSTANT_ARC
¶
-
enumerator
Functions
-
lv_obj_t *
lv_preload_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a pre loader objects
- Return
pointer to the created pre loader
- Parameters
par
: pointer to an object, it will be the parent of the new pre loadercopy
: pointer to a pre loader object, if not NULL then the new object will be copied from it
-
void
lv_preload_set_arc_length
(lv_obj_t *preload, lv_anim_value_t deg)¶ Set the length of the spinning arc in degrees
- Parameters
preload
: pointer to a preload objectdeg
: length of the arc
-
void
lv_preload_set_spin_time
(lv_obj_t *preload, uint16_t time)¶ Set the spin time of the arc
- Parameters
preload
: pointer to a preload objecttime
: time of one round in milliseconds
-
void
lv_preload_set_style
(lv_obj_t *preload, lv_preload_style_t type, const lv_style_t *style)¶ Set a style of a pre loader.
- Parameters
preload
: pointer to pre loader objecttype
: which style should be setstyle
: pointer to a style
-
void
lv_preload_set_type
(lv_obj_t *preload, lv_preload_type_t type)¶ Set the animation type of a preloader.
- Parameters
preload
: pointer to pre loader objecttype
: animation type of the preload
-
void
lv_preload_set_dir
(lv_obj_t *preload, lv_preload_dir_t dir)¶ Set the animation direction of a preloader
- Parameters
preload
: pointer to pre loader objectdirection
: animation direction of the preload
-
lv_anim_value_t
lv_preload_get_arc_length
(const lv_obj_t *preload)¶ Get the arc length [degree] of the a pre loader
- Parameters
preload
: pointer to a pre loader object
-
uint16_t
lv_preload_get_spin_time
(const lv_obj_t *preload)¶ Get the spin time of the arc
- Parameters
preload
: pointer to a pre loader object [milliseconds]
-
const lv_style_t *
lv_preload_get_style
(const lv_obj_t *preload, lv_preload_style_t type)¶ Get style of a pre loader.
- Return
style pointer to the style
- Parameters
preload
: pointer to pre loader objecttype
: which style should be get
-
lv_preload_type_t
lv_preload_get_type
(lv_obj_t *preload)¶ Get the animation type of a preloader.
- Return
animation type
- Parameters
preload
: pointer to pre loader object
-
lv_preload_dir_t
lv_preload_get_dir
(lv_obj_t *preload)¶ Get the animation direction of a preloader
- Return
animation direction
- Parameters
preload
: pointer to pre loader object
-
void
lv_preload_spinner_anim
(void *ptr, lv_anim_value_t val)¶ Animator function (exec_cb) to rotate the arc of spinner.
- Parameters
ptr
: pointer to preloaderval
: the current desired value [0..360]
-
struct
lv_preload_ext_t
¶ Public Members
-
lv_arc_ext_t
arc
¶
-
lv_anim_value_t
arc_length
¶
-
uint16_t
time
¶
-
lv_preload_type_t
anim_type
¶
-
lv_preload_dir_t
anim_dir
¶
-
lv_arc_ext_t