Tabview (lv_tabview)¶
Overview¶
The Tab view object can be used to organize content in tabs.
Adding tab¶
You can add a new tabs with lv_tabview_add_tab(tabview, "Tab name")
. It will return with a pointer to a Page object where you can add the tab’s content.
Change tab¶
To select a new tab you can:
Click on it on the header part
Slide horizontally
Use
lv_tabview_set_tab_act(tabview, id, LV_ANIM_ON/OFF)
function
The manual sliding can be disabled with lv_tabview_set_sliding(tabview, false)
.
Tab button’s position¶
By default, the tab selector buttons are placed on the top of the Tabview. It can be changed with lv_tabview_set_btns_pos(tabview, LV_TABVIEW_BTNS_POS_TOP/BOTTOM/LEFT/RIGHT)
Note that, you can’t change the tab position from top or bottom to left or right when tabs are already added.
Hide the tabs¶
The tab buttons can be hidden by lv_tabview_set_btns_hidden(tabview, true)
Animation time¶
The animation time is adjusted by lv_tabview_set_anim_time(tabview, anim_time_ms)
. It is used when the new tab is loaded.
Style usage¶
Use lv_tabview_set_style(tabview, LV_TABVIEW_STYLE_..., &style)
to set a new style for an element of the Tabview:
LV_TABVIEW_STYLE_BG main background which uses all
style.body
properties (default:lv_style_plain
)LV_TABVIEW_STYLE_INDIC a thin rectangle on indicating the current tab. Uses all
style.body
properties. Its height comes frombody.padding.inner
(default:lv_style_plain_color
)LV_TABVIEW_STYLE_BTN_BG style of the tab buttons’ background. Uses all
style.body
properties. The header height will be set automatically consideringbody.padding.top/bottom
(default:lv_style_transp
)LV_TABVIEW_STYLE_BTN_REL style of released tab buttons. Uses all
style.body
properties. (default:lv_style_tbn_rel
)LV_TABVIEW_STYLE_BTN_PR style of released tab buttons. Uses all
style.body
properties exceptpadding
. (default:lv_style_tbn_rel
)LV_TABVIEW_STYLE_BTN_TGL_REL style of selected released tab buttons. Uses all
style.body
properties exceptpadding
. (default:lv_style_tbn_rel
)LV_TABVIEW_STYLE_BTN_TGL_PR style of selected pressed tab buttons. Uses all
style.body
properties exceptpadding
. (default:lv_style_btn_tgl_pr
)
The height of the header is calculated like: font height and padding.top and padding.bottom from LV_TABVIEW_STYLE_BTN_REL + padding.top and padding bottom from LV_TABVIEW_STYLE_BTN_BG
Events¶
Besides the Generic events the following Special events are sent by the Slider:
LV_EVENT_VALUE_CHANGED Sent when a new tab is selected by sliding or clicking the tab button
Learn more about Events.
Keys¶
The following Keys are processed by the Tabview:
LV_KEY_RIGHT/LEFT Select a tab
LV_KEY_ENTER Change to the selected tab
Learn more about Keys.
Example¶
C¶
Simple Tabview¶
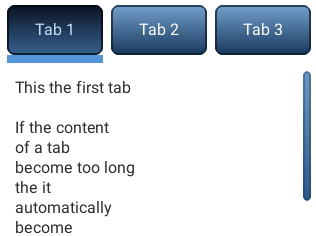
code
#include "lvgl/lvgl.h"
void lv_ex_tabview_1(void)
{
/*Create a Tab view object*/
lv_obj_t *tabview;
tabview = lv_tabview_create(lv_scr_act(), NULL);
/*Add 3 tabs (the tabs are page (lv_page) and can be scrolled*/
lv_obj_t *tab1 = lv_tabview_add_tab(tabview, "Tab 1");
lv_obj_t *tab2 = lv_tabview_add_tab(tabview, "Tab 2");
lv_obj_t *tab3 = lv_tabview_add_tab(tabview, "Tab 3");
/*Add content to the tabs*/
lv_obj_t * label = lv_label_create(tab1, NULL);
lv_label_set_text(label, "This the first tab\n\n"
"If the content\n"
"of a tab\n"
"become too long\n"
"the it \n"
"automatically\n"
"become\n"
"scrollable.");
label = lv_label_create(tab2, NULL);
lv_label_set_text(label, "Second tab");
label = lv_label_create(tab3, NULL);
lv_label_set_text(label, "Third tab");
}
MicroPython¶
Simple Tabview¶
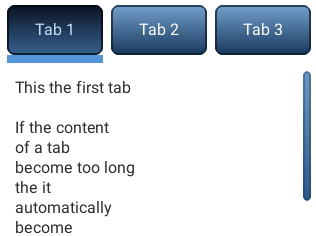
code
# Create a Tab view object
tabview = lv.tabview(lv.scr_act())
# Add 3 tabs (the tabs are page (lv_page) and can be scrolled
tab1 = tabview.add_tab("Tab 1")
tab2 = tabview.add_tab("Tab 2")
tab3 = tabview.add_tab("Tab 3")
# Add content to the tabs
label = lv.label(tab1)
label.set_text("""This the first tab
If the content
of a tab
become too long
the it
automatically
become
scrollable.""")
label = lv.label(tab2)
label.set_text("Second tab")
label = lv.label(tab3)
label.set_text("Third tab")
API¶
Enums
Functions
-
lv_obj_t *
lv_tabview_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a Tab view object
- Return
pointer to the created tab
- Parameters
par
: pointer to an object, it will be the parent of the new tabcopy
: pointer to a tab object, if not NULL then the new object will be copied from it
-
void
lv_tabview_clean
(lv_obj_t *tabview)¶ Delete all children of the scrl object, without deleting scrl child.
- Parameters
tabview
: pointer to an object
-
lv_obj_t *
lv_tabview_add_tab
(lv_obj_t *tabview, const char *name)¶ Add a new tab with the given name
- Return
pointer to the created page object (lv_page). You can create your content here
- Parameters
tabview
: pointer to Tab view object where to ass the new tabname
: the text on the tab button
-
void
lv_tabview_set_tab_act
(lv_obj_t *tabview, uint16_t id, lv_anim_enable_t anim)¶ Set a new tab
- Parameters
tabview
: pointer to Tab view objectid
: index of a tab to loadanim
: LV_ANIM_ON: set the value with an animation; LV_ANIM_OFF: change the value immediately
-
void
lv_tabview_set_sliding
(lv_obj_t *tabview, bool en)¶ Enable horizontal sliding with touch pad
- Parameters
tabview
: pointer to Tab view objecten
: true: enable sliding; false: disable sliding
-
void
lv_tabview_set_anim_time
(lv_obj_t *tabview, uint16_t anim_time)¶ Set the animation time of tab view when a new tab is loaded
- Parameters
tabview
: pointer to Tab view objectanim_time
: time of animation in milliseconds
-
void
lv_tabview_set_style
(lv_obj_t *tabview, lv_tabview_style_t type, const lv_style_t *style)¶ Set the style of a tab view
- Parameters
tabview
: pointer to a tan view objecttype
: which style should be setstyle
: pointer to the new style
-
void
lv_tabview_set_btns_pos
(lv_obj_t *tabview, lv_tabview_btns_pos_t btns_pos)¶ Set the position of tab select buttons
- Parameters
tabview
: pointer to a tab view objectbtns_pos
: which button position
Set whether tab buttons are hidden
- Parameters
tabview
: pointer to a tab view objecten
: whether tab buttons are hidden
-
uint16_t
lv_tabview_get_tab_act
(const lv_obj_t *tabview)¶ Get the index of the currently active tab
- Return
the active tab index
- Parameters
tabview
: pointer to Tab view object
-
uint16_t
lv_tabview_get_tab_count
(const lv_obj_t *tabview)¶ Get the number of tabs
- Return
tab count
- Parameters
tabview
: pointer to Tab view object
-
lv_obj_t *
lv_tabview_get_tab
(const lv_obj_t *tabview, uint16_t id)¶ Get the page (content area) of a tab
- Return
pointer to page (lv_page) object
- Parameters
tabview
: pointer to Tab view objectid
: index of the tab (>= 0)
-
bool
lv_tabview_get_sliding
(const lv_obj_t *tabview)¶ Get horizontal sliding is enabled or not
- Return
true: enable sliding; false: disable sliding
- Parameters
tabview
: pointer to Tab view object
-
uint16_t
lv_tabview_get_anim_time
(const lv_obj_t *tabview)¶ Get the animation time of tab view when a new tab is loaded
- Return
time of animation in milliseconds
- Parameters
tabview
: pointer to Tab view object
-
const lv_style_t *
lv_tabview_get_style
(const lv_obj_t *tabview, lv_tabview_style_t type)¶ Get a style of a tab view
- Return
style pointer to a style
- Parameters
tabview
: pointer to a ab view objecttype
: which style should be get
-
lv_tabview_btns_pos_t
lv_tabview_get_btns_pos
(const lv_obj_t *tabview)¶ Get position of tab select buttons
- Parameters
tabview
: pointer to a ab view object
Get whether tab buttons are hidden
- Return
whether tab buttons are hidden
- Parameters
tabview
: pointer to a tab view object
-
struct
lv_tabview_ext_t
¶