Checkbox (lv_cb)¶
Overview¶
The Checkbox objects are built from a Button background which contains an also Button bullet and a Label to realize a classical checkbox.
Text¶
The text can be modified by the lv_cb_set_text(cb, "New text")
function. It will dynamically allocate the text.
To set a static text, use lv_cb_set_static_text(cb, txt)
. This way, only a pointer of txt
will be stored and it shouldn’t be deallocated while the checkbox exists.
Check/Uncheck¶
You can manually check / un-check the Checkbox via lv_cb_set_checked(cb, true/false)
. Setting true
will check the checkbox and false
will un-check the checkbox.
Inactive¶
To make the Checkbox inactive, use lv_cb_set_inactive(cb, true)
.
Styles¶
The Checkbox styles can be modified with lv_cb_set_style(cb, LV_CB_STYLE_..., &style)
.
LV_CB_STYLE_BG - Background style. Uses all
style.body
properties. The label’s style comes fromstyle.text
. Default:lv_style_transp
LV_CB_STYLE_BOX_REL - Style of the released box. Uses the
style.body
properties. Default:lv_style_btn_rel
LV_CB_STYLE_BOX_PR - Style of the pressed box. Uses the
style.body
properties. Default:lv_style_btn_pr
LV_CB_STYLE_BOX_TGL_REL - Style of the checked released box. Uses the
style.body
properties. Default:lv_style_btn_tgl_rel
LV_CB_STYLE_BOX_TGL_PR - Style of the checked released box. Uses the
style.body
properties. Default:lv_style_btn_tgl_pr
LV_CB_STYLE_BOX_INA - Style of the inactive box. Uses the
style.body
properties. Default:lv_style_btn_ina
Events¶
Besides the Generic events the following Special events are sent by the Checkboxes:
LV_EVENT_VALUE_CHANGED - sent when the checkbox is toggled.
Note that, the generic input device-related events (like LV_EVENT_PRESSED
) are sent in the inactive state too. You need to check the state with lv_cb_is_inactive(cb)
to ignore the events from inactive Checkboxes.
Learn more about Events.
Keys¶
The following Keys are processed by the ‘Buttons’:
LV_KEY_RIGHT/UP - Go to toggled state if toggling is enabled
LV_KEY_LEFT/DOWN - Go to non-toggled state if toggling is enabled
Note that, as usual, the state of LV_KEY_ENTER
is translated to LV_EVENT_PRESSED/PRESSING/RELEASED
etc.
Learn more about Keys.
Example¶
C¶
Simple Checkbox¶
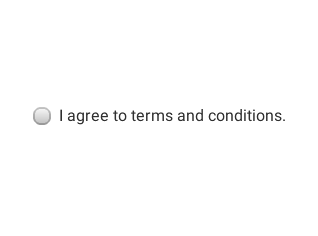
code
#include "lvgl/lvgl.h"
#include <stdio.h>
static void event_handler(lv_obj_t * obj, lv_event_t event)
{
if(event == LV_EVENT_VALUE_CHANGED) {
printf("State: %s\n", lv_cb_is_checked(obj) ? "Checked" : "Unchecked");
}
}
void lv_ex_cb_1(void)
{
lv_obj_t * cb = lv_cb_create(lv_scr_act(), NULL);
lv_cb_set_text(cb, "I agree to terms and conditions.");
lv_obj_align(cb, NULL, LV_ALIGN_CENTER, 0, 0);
lv_obj_set_event_cb(cb, event_handler);
}
MicroPython¶
Simple Checkbox¶
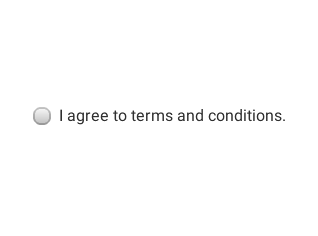
code
def event_handler(obj, event):
if event == lv.EVENT.VALUE_CHANGED:
print("State: %s" % ("Checked" if obj.is_checked() else "Unchecked"))
cb = lv.cb(lv.scr_act())
cb.set_text("I agree to terms and conditions.")
cb.align(None, lv.ALIGN.CENTER, 0, 0)
cb.set_event_cb(event_handler)
API¶
Typedefs
-
typedef uint8_t
lv_cb_style_t
¶
Enums
-
enum [anonymous]¶
Checkbox styles.
Values:
-
enumerator
LV_CB_STYLE_BG
¶ Style of object background.
-
enumerator
LV_CB_STYLE_BOX_REL
¶ Style of box (released).
-
enumerator
LV_CB_STYLE_BOX_PR
¶ Style of box (pressed).
-
enumerator
LV_CB_STYLE_BOX_TGL_REL
¶ Style of box (released but checked).
-
enumerator
LV_CB_STYLE_BOX_TGL_PR
¶ Style of box (pressed and checked).
-
enumerator
LV_CB_STYLE_BOX_INA
¶ Style of disabled box
-
enumerator
Functions
-
lv_obj_t *
lv_cb_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a check box objects
- Return
pointer to the created check box
- Parameters
par
: pointer to an object, it will be the parent of the new check boxcopy
: pointer to a check box object, if not NULL then the new object will be copied from it
-
void
lv_cb_set_text
(lv_obj_t *cb, const char *txt)¶ Set the text of a check box.
txt
will be copied and may be deallocated after this function returns.- Parameters
cb
: pointer to a check boxtxt
: the text of the check box. NULL to refresh with the current text.
-
void
lv_cb_set_static_text
(lv_obj_t *cb, const char *txt)¶ Set the text of a check box.
txt
must not be deallocated during the life of this checkbox.- Parameters
cb
: pointer to a check boxtxt
: the text of the check box. NULL to refresh with the current text.
-
void
lv_cb_set_checked
(lv_obj_t *cb, bool checked)¶ Set the state of the check box
- Parameters
cb
: pointer to a check box objectchecked
: true: make the check box checked; false: make it unchecked
-
void
lv_cb_set_inactive
(lv_obj_t *cb)¶ Make the check box inactive (disabled)
- Parameters
cb
: pointer to a check box object
-
void
lv_cb_set_style
(lv_obj_t *cb, lv_cb_style_t type, const lv_style_t *style)¶ Set a style of a check box
- Parameters
cb
: pointer to check box objecttype
: which style should be setstyle
: pointer to a style
-
const char *
lv_cb_get_text
(const lv_obj_t *cb)¶ Get the text of a check box
- Return
pointer to the text of the check box
- Parameters
cb
: pointer to check box object
-
bool
lv_cb_is_checked
(const lv_obj_t *cb)¶ Get the current state of the check box
- Return
true: checked; false: not checked
- Parameters
cb
: pointer to a check box object
-
bool
lv_cb_is_inactive
(const lv_obj_t *cb)¶ Get whether the check box is inactive or not.
- Return
true: inactive; false: not inactive
- Parameters
cb
: pointer to a check box object
-
const lv_style_t *
lv_cb_get_style
(const lv_obj_t *cb, lv_cb_style_t type)¶ Get a style of a button
- Return
style pointer to the style
- Parameters
cb
: pointer to check box objecttype
: which style should be get
-
struct
lv_cb_ext_t
¶