Window (lv_win)¶
Overview¶
The windows are one of the most complex container-like objects. They are built from two main parts:
Title¶
On the header, there is a title which can be modified by: lv_win_set_title(win, "New title")
. The title always inherits the style of the header.
Control buttons¶
You can add control buttons to the right side of the header with: lv_win_add_btn(win, LV_SYMBOL_CLOSE)
.
The second parameter is an Image source.
lv_win_close_event_cb
can be used as an event callback to close the Window.
You can modify the size of the control buttons with the lv_win_set_btn_size(win, new_size)
function.
Scrollbars¶
The scrollbar behavior can be set by lv_win_set_sb_mode(win, LV_SB_MODE_...)
. See Page for details.
Manual scroll and focus¶
To scroll the Window directly you can use lv_win_scroll_hor(win, dist_px)
or lv_win_scroll_ver(win, dist_px)
.
To make the Window show an object on it use lv_win_focus(win, child, LV_ANIM_ON/OFF)
.
The time of scroll and focus animations can be adjusted with lv_win_set_anim_time(win, anim_time_ms)
Style usage¶
Use lv_win_set_style(win, LV_WIN_STYLE_..., &style)
to set a new style for an element of the Window:
LV_WIN_STYE_BG main background which uses all
style.body
properties (header and content page are placed on it) (default:lv_style_plain
)LV_WIN_STYLE_CONTENT content page’s scrollable part which uses all
style.body
properties (default:lv_style_transp
)LV_WIN_STYLE_SB scroll bar’s style which uses all
style.body
properties.left/top
padding sets the scrollbars’ padding respectively and the inner padding sets the scrollbar’s width. (default:lv_style_pretty_color
)LV_WIN_STYLE_HEADER header’s style which uses all
style.body
properties (default:lv_style_plain_color
)LV_WIN_STYLE_BTN_REL released button’s style (on header) which uses all
style.body
properties (default:lv_style_btn_rel
)LV_WIN_STYLE_BTN_PR released button’s style (on header) which uses all
style.body
properties (default:lv_style_btn_pr
)
The height of the header is set to the greater value from buttons’ height (set by lv_win_set_btn_size
) and title height (comes from header_style.text.font
) plus the body.padding.top
and body.padding.bottom
of the header style.
Keys¶
The following Keys are processed by the Page:
LV_KEY_RIGHT/LEFT/UP/DOWN Scroll the page
Learn more about Keys.
Example¶
C¶
Simple window¶
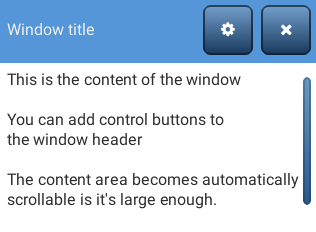
code
#include "lvgl/lvgl.h"
void lv_ex_win_1(void)
{
/*Create a window*/
lv_obj_t * win = lv_win_create(lv_scr_act(), NULL);
lv_win_set_title(win, "Window title"); /*Set the title*/
/*Add control button to the header*/
lv_obj_t * close_btn = lv_win_add_btn(win, LV_SYMBOL_CLOSE); /*Add close button and use built-in close action*/
lv_obj_set_event_cb(close_btn, lv_win_close_event_cb);
lv_win_add_btn(win, LV_SYMBOL_SETTINGS); /*Add a setup button*/
/*Add some dummy content*/
lv_obj_t * txt = lv_label_create(win, NULL);
lv_label_set_text(txt, "This is the content of the window\n\n"
"You can add control buttons to\n"
"the window header\n\n"
"The content area becomes automatically\n"
"scrollable is it's large enough.\n\n"
" You can scroll the content\n"
"See the scroll bar on the right!");
}
MicroPython¶
Simple window¶
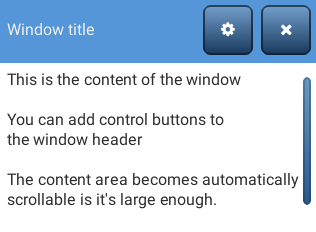
code
# Create a window
win = lv.win(lv.scr_act())
win.set_title("Window title") # Set the title
# Add control button to the header
close_btn = win.add_btn(lv.SYMBOL.CLOSE) # Add close button and use built-in close action
close_btn.set_event_cb(lv.win.close_event_cb)
win.add_btn(lv.SYMBOL.SETTINGS) # Add a setup button
# Add some dummy content
txt = lv.label(win)
txt.set_text(
"""This is the content of the window
You can add control buttons to
the window header
The content area becomes automatically
scrollable is it's large enough.
You can scroll the content
See the scroll bar on the right!"""
)
API¶
Typedefs
-
typedef uint8_t
lv_win_style_t
¶
Enums
-
enum [anonymous]¶
Window styles.
Values:
-
enumerator
LV_WIN_STYLE_BG
¶ Window object background style.
-
enumerator
LV_WIN_STYLE_CONTENT
¶ Window content style.
-
enumerator
LV_WIN_STYLE_SB
¶ Window scrollbar style.
-
enumerator
LV_WIN_STYLE_HEADER
¶ Window titlebar background style.
-
enumerator
LV_WIN_STYLE_BTN_REL
¶ Same meaning as ordinary button styles.
-
enumerator
LV_WIN_STYLE_BTN_PR
¶
-
enumerator
Functions
-
lv_obj_t *
lv_win_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a window objects
- Return
pointer to the created window
- Parameters
par
: pointer to an object, it will be the parent of the new windowcopy
: pointer to a window object, if not NULL then the new object will be copied from it
-
void
lv_win_clean
(lv_obj_t *win)¶ Delete all children of the scrl object, without deleting scrl child.
- Parameters
win
: pointer to an object
-
lv_obj_t *
lv_win_add_btn
(lv_obj_t *win, const void *img_src)¶ Add control button to the header of the window
- Return
pointer to the created button object
- Parameters
win
: pointer to a window objectimg_src
: an image source (‘lv_img_t’ variable, path to file or a symbol)
-
void
lv_win_close_event_cb
(lv_obj_t *btn, lv_event_t event)¶ Can be assigned to a window control button to close the window
- Parameters
btn
: pointer to the control button on teh widows headerevet
: the event type
-
void
lv_win_set_title
(lv_obj_t *win, const char *title)¶ Set the title of a window
- Parameters
win
: pointer to a window objecttitle
: string of the new title
-
void
lv_win_set_btn_size
(lv_obj_t *win, lv_coord_t size)¶ Set the control button size of a window
- Return
control button size
- Parameters
win
: pointer to a window object
-
void
lv_win_set_content_size
(lv_obj_t *win, lv_coord_t w, lv_coord_t h)¶ Set the size of the content area.
- Parameters
win
: pointer to a window objectw
: widthh
: height (the window will be higher with the height of the header)
-
void
lv_win_set_layout
(lv_obj_t *win, lv_layout_t layout)¶ Set the layout of the window
- Parameters
win
: pointer to a window objectlayout
: the layout from ‘lv_layout_t’
-
void
lv_win_set_sb_mode
(lv_obj_t *win, lv_sb_mode_t sb_mode)¶ Set the scroll bar mode of a window
- Parameters
win
: pointer to a window objectsb_mode
: the new scroll bar mode from ‘lv_sb_mode_t’
-
void
lv_win_set_anim_time
(lv_obj_t *win, uint16_t anim_time)¶ Set focus animation duration on
lv_win_focus()
- Parameters
win
: pointer to a window objectanim_time
: duration of animation [ms]
-
void
lv_win_set_style
(lv_obj_t *win, lv_win_style_t type, const lv_style_t *style)¶ Set a style of a window
- Parameters
win
: pointer to a window objecttype
: which style should be setstyle
: pointer to a style
-
void
lv_win_set_drag
(lv_obj_t *win, bool en)¶ Set drag status of a window. If set to ‘true’ window can be dragged like on a PC.
- Parameters
win
: pointer to a window objecten
: whether dragging is enabled
-
const char *
lv_win_get_title
(const lv_obj_t *win)¶ Get the title of a window
- Return
title string of the window
- Parameters
win
: pointer to a window object
-
lv_obj_t *
lv_win_get_content
(const lv_obj_t *win)¶ Get the content holder object of window (
lv_page
) to allow additional customization- Return
the Page object where the window’s content is
- Parameters
win
: pointer to a window object
-
lv_coord_t
lv_win_get_btn_size
(const lv_obj_t *win)¶ Get the control button size of a window
- Return
control button size
- Parameters
win
: pointer to a window object
-
lv_obj_t *
lv_win_get_from_btn
(const lv_obj_t *ctrl_btn)¶ Get the pointer of a widow from one of its control button. It is useful in the action of the control buttons where only button is known.
- Return
pointer to the window of ‘ctrl_btn’
- Parameters
ctrl_btn
: pointer to a control button of a window
-
lv_layout_t
lv_win_get_layout
(lv_obj_t *win)¶ Get the layout of a window
- Return
the layout of the window (from ‘lv_layout_t’)
- Parameters
win
: pointer to a window object
-
lv_sb_mode_t
lv_win_get_sb_mode
(lv_obj_t *win)¶ Get the scroll bar mode of a window
- Return
the scroll bar mode of the window (from ‘lv_sb_mode_t’)
- Parameters
win
: pointer to a window object
-
uint16_t
lv_win_get_anim_time
(const lv_obj_t *win)¶ Get focus animation duration
- Return
duration of animation [ms]
- Parameters
win
: pointer to a window object
-
lv_coord_t
lv_win_get_width
(lv_obj_t *win)¶ Get width of the content area (page scrollable) of the window
- Return
the width of the content area
- Parameters
win
: pointer to a window object
-
const lv_style_t *
lv_win_get_style
(const lv_obj_t *win, lv_win_style_t type)¶ Get a style of a window
- Return
style pointer to a style
- Parameters
win
: pointer to a button objecttype
: which style window be get
-
bool
lv_win_get_drag
(const lv_obj_t *win)¶ Get drag status of a window. If set to ‘true’ window can be dragged like on a PC.
- Return
whether window is draggable
- Parameters
win
: pointer to a window object
-
void
lv_win_focus
(lv_obj_t *win, lv_obj_t *obj, lv_anim_enable_t anim_en)¶ Focus on an object. It ensures that the object will be visible in the window.
- Parameters
win
: pointer to a window objectobj
: pointer to an object to focus (must be in the window)anim_en
: LV_ANIM_ON focus with an animation; LV_ANIM_OFF focus without animation
-
struct
lv_win_ext_t
¶