Container (lv_cont)¶
Overview¶
The containers are essentially a basic object with some special features.
Layout¶
You can apply a layout on the containers to automatically order their children. The layout spacing comes from style.body.padding. ...
properties. The possible layout options:
LV_LAYOUT_OFF - Do not align the children.
LV_LAYOUT_CENTER - Align children to the center in column and keep
padding.inner
space between them.LV_LAYOUT_COL_L - Align children in a left-justified column. Keep
padding.left
space on the left,pad.top
space on the top andpadding.inner
space between the children.LV_LAYOUT_COL_M - Align children in centered column. Keep
padding.top
space on the top andpadding.inner
space between the children.LV_LAYOUT_COL_R - Align children in a right-justified column. Keep
padding.right
space on the right,padding.top
space on the top andpadding.inner
space between the children.LV_LAYOUT_ROW_T - Align children in a top justified row. Keep
padding.left
space on the left,padding.top
space on the top andpadding.inner
space between the children.LV_LAYOUT_ROW_M - Align children in centered row. Keep
padding.left
space on the left andpadding.inner
space between the children.LV_LAYOUT_ROW_B - Align children in a bottom justified row. Keep
padding.left
space on the left,padding.bottom
space on the bottom andpadding.inner
space between the children.LV_LAYOUT_PRETTY - Put as many objects as possible in a row (with at least
padding.inner
space andpadding.left/right
space on the sides). Divide the space in each line equally between the children. Keeppadding.top
space on the top andpad.inner
space between the lines.LV_LAYOUT_GRID - Similar to
LV_LAYOUT_PRETTY
but not divide horizontal space equally just letpadding.left/right
on the edges andpadding.inner
space between the elements.
Autofit¶
Container have an autofit feature which can automatically change the size of the container according to its children and/or parent. The following options exist:
LV_FIT_NONE - Do not change the size automatically.
LV_FIT_TIGHT - Shrink-wrap the container around all of its children, while keeping
padding.top/bottom/left/right
space on the edges.LV_FIT_FLOOD - Set the size to the parent’s size minus
padding.top/bottom/left/right
(from the parent’s style) space.LV_FIT_FILL - Use
LV_FIT_FLOOD
while smaller than the parent andLV_FIT_TIGHT
when larger. It will ensure that the container is, at minimum, the size of its parent.
To set the auto fit mode for all directions, use lv_cont_set_fit(cont, LV_FIT_...)
.
To use different auto fit horizontally and vertically, use lv_cont_set_fit2(cont, hor_fit_type, ver_fit_type)
.
To use different auto fit in all 4 directions, use lv_cont_set_fit4(cont, left_fit_type, right_fit_type, top_fit_type, bottom_fit_type)
.
Styles¶
You can set the styles with lv_cont_set_style(btn, LV_CONT_STYLE_MAIN, &style)
.
style.body properties are used.
Example¶
C¶
Container with auto-fit¶
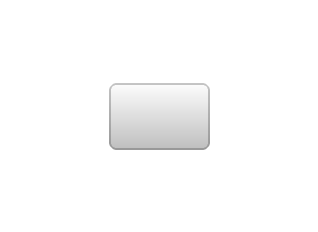
code
#include "lvgl/lvgl.h"
void lv_ex_cont_1(void)
{
lv_obj_t * cont;
cont = lv_cont_create(lv_scr_act(), NULL);
lv_obj_set_auto_realign(cont, true); /*Auto realign when the size changes*/
lv_obj_align_origo(cont, NULL, LV_ALIGN_CENTER, 0, 0); /*This parametrs will be sued when realigned*/
lv_cont_set_fit(cont, LV_FIT_TIGHT);
lv_cont_set_layout(cont, LV_LAYOUT_COL_M);
lv_obj_t * label;
label = lv_label_create(cont, NULL);
lv_label_set_text(label, "Short text");
label = lv_label_create(cont, NULL);
lv_label_set_text(label, "It is a long text");
label = lv_label_create(cont, NULL);
lv_label_set_text(label, "Here is an even longer text");
}
MicroPython¶
Container with auto-fit¶
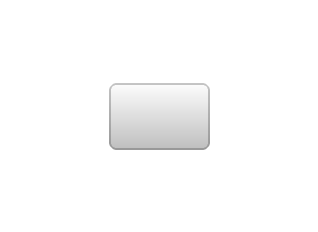
code
cont = lv.cont(lv.scr_act())
cont.set_auto_realign(True) # Auto realign when the size changes
cont.align_origo(None, lv.ALIGN.CENTER, 0, 0) # This parametrs will be sued when realigned
cont.set_fit(lv.FIT.TIGHT)
cont.set_layout(lv.LAYOUT.COL_M)
label = lv.label(cont)
label.set_text("Short text")
label = lv.label(cont)
label.set_text("It is a long text")
label = lv.label(cont)
label.set_text("Here is an even longer text")
API¶
Enums
-
enum [anonymous]¶
Container layout options
Values:
-
enumerator
LV_LAYOUT_OFF
= 0¶ No layout
-
enumerator
LV_LAYOUT_CENTER
¶ Center objects
-
enumerator
LV_LAYOUT_COL_L
¶ Column left align
-
enumerator
LV_LAYOUT_COL_M
¶ Column middle align
-
enumerator
LV_LAYOUT_COL_R
¶ Column right align
-
enumerator
LV_LAYOUT_ROW_T
¶ Row top align
-
enumerator
LV_LAYOUT_ROW_M
¶ Row middle align
-
enumerator
LV_LAYOUT_ROW_B
¶ Row bottom align
-
enumerator
LV_LAYOUT_PRETTY
¶ Put as many object as possible in row and begin a new row
-
enumerator
LV_LAYOUT_GRID
¶ Align same-sized object into a grid
-
enumerator
_LV_LAYOUT_NUM
¶
-
enumerator
-
enum [anonymous]¶
How to resize the container around the children.
Values:
-
enumerator
LV_FIT_NONE
¶ Do not change the size automatically
-
enumerator
LV_FIT_TIGHT
¶ Shrink wrap around the children
-
enumerator
LV_FIT_FLOOD
¶ Align the size to the parent’s edge
-
enumerator
LV_FIT_FILL
¶ Align the size to the parent’s edge first but if there is an object out of it then get larger
-
enumerator
_LV_FIT_NUM
¶
-
enumerator
Functions
-
lv_obj_t *
lv_cont_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a container objects
- Return
pointer to the created container
- Parameters
par
: pointer to an object, it will be the parent of the new containercopy
: pointer to a container object, if not NULL then the new object will be copied from it
-
void
lv_cont_set_layout
(lv_obj_t *cont, lv_layout_t layout)¶ Set a layout on a container
- Parameters
cont
: pointer to a container objectlayout
: a layout from ‘lv_cont_layout_t’
-
void
lv_cont_set_fit4
(lv_obj_t *cont, lv_fit_t left, lv_fit_t right, lv_fit_t top, lv_fit_t bottom)¶ Set the fit policy in all 4 directions separately. It tell how to change the container’s size automatically.
- Parameters
cont
: pointer to a container objectleft
: left fit policy fromlv_fit_t
right
: right fit policy fromlv_fit_t
top
: top fit policy fromlv_fit_t
bottom
: bottom fit policy fromlv_fit_t
-
void
lv_cont_set_fit2
(lv_obj_t *cont, lv_fit_t hor, lv_fit_t ver)¶ Set the fit policy horizontally and vertically separately. It tells how to change the container’s size automatically.
- Parameters
cont
: pointer to a container objecthor
: horizontal fit policy fromlv_fit_t
ver
: vertical fit policy fromlv_fit_t
-
void
lv_cont_set_fit
(lv_obj_t *cont, lv_fit_t fit)¶ Set the fit policy in all 4 direction at once. It tells how to change the container’s size automatically.
- Parameters
cont
: pointer to a container objectfit
: fit policy fromlv_fit_t
-
void
lv_cont_set_style
(lv_obj_t *cont, lv_cont_style_t type, const lv_style_t *style)¶ Set the style of a container
- Parameters
cont
: pointer to a container objecttype
: which style should be set (can be onlyLV_CONT_STYLE_MAIN
)style
: pointer to the new style
-
lv_layout_t
lv_cont_get_layout
(const lv_obj_t *cont)¶ Get the layout of a container
- Return
the layout from ‘lv_cont_layout_t’
- Parameters
cont
: pointer to container object
-
lv_fit_t
lv_cont_get_fit_left
(const lv_obj_t *cont)¶ Get left fit mode of a container
- Return
an element of
lv_fit_t
- Parameters
cont
: pointer to a container object
-
lv_fit_t
lv_cont_get_fit_right
(const lv_obj_t *cont)¶ Get right fit mode of a container
- Return
an element of
lv_fit_t
- Parameters
cont
: pointer to a container object
-
lv_fit_t
lv_cont_get_fit_top
(const lv_obj_t *cont)¶ Get top fit mode of a container
- Return
an element of
lv_fit_t
- Parameters
cont
: pointer to a container object
-
lv_fit_t
lv_cont_get_fit_bottom
(const lv_obj_t *cont)¶ Get bottom fit mode of a container
- Return
an element of
lv_fit_t
- Parameters
cont
: pointer to a container object
-
const lv_style_t *
lv_cont_get_style
(const lv_obj_t *cont, lv_cont_style_t type)¶ Get the style of a container
- Return
pointer to the container’s style
- Parameters
cont
: pointer to a container objecttype
: which style should be get (can be onlyLV_CONT_STYLE_MAIN
)
-
struct
lv_cont_ext_t
¶