Line meter (lv_lmeter)¶
Overview¶
The Line Meter object consists of some radial lines which draw a scale.
Set value¶
When setting a new value with lv_lmeter_set_value(lmeter, new_value)
the proportional part of the scale will be recolored.
Range and Angles¶
The lv_lmeter_set_range(lmeter, min, max)
function sets the range of the line meter.
You can set the angle of the scale and the number of the lines by: lv_lmeter_set_scale(lmeter, angle, line_num)
.
The default angle is 240 and the default line number is 21.
Angle offset¶
By default the scale angle is interpreted symmetrically to the y axis. It results in “standing” line meter. With lv_lmeter_set_angle_offset
an offset can be added the scale angle.
It can used e.g to put a quarter line meter into a corner or a half line meter to the right or left side.
Styles¶
The line meter uses one style which can be set by lv_lmeter_set_style(lmeter, LV_LMETER_STYLE_MAIN, &style)
. The line meter’s properties are derived from the following style attributes:
line.color “inactive line’s” color which are greater then the current value
body.main_color “active line’s” color at the beginning of the scale
body.grad_color “active line’s” color at the end of the scale (gradient with main color)
body.padding.hor line length
line.width line width
The default style is lv_style_pretty_color
.
Example¶
C¶
Simple Line meter¶
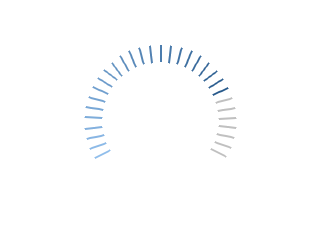
code
#include "lvgl/lvgl.h"
void lv_ex_lmeter_1(void)
{
/*Create a style for the line meter*/
static lv_style_t style_lmeter;
lv_style_copy(&style_lmeter, &lv_style_pretty_color);
style_lmeter.line.width = 2;
style_lmeter.line.color = LV_COLOR_SILVER;
style_lmeter.body.main_color = lv_color_hex(0x91bfed); /*Light blue*/
style_lmeter.body.grad_color = lv_color_hex(0x04386c); /*Dark blue*/
style_lmeter.body.padding.left = 16; /*Line length*/
/*Create a line meter */
lv_obj_t * lmeter;
lmeter = lv_lmeter_create(lv_scr_act(), NULL);
lv_lmeter_set_range(lmeter, 0, 100); /*Set the range*/
lv_lmeter_set_value(lmeter, 80); /*Set the current value*/
lv_lmeter_set_scale(lmeter, 240, 31); /*Set the angle and number of lines*/
lv_lmeter_set_style(lmeter, LV_LMETER_STYLE_MAIN, &style_lmeter); /*Apply the new style*/
lv_obj_set_size(lmeter, 150, 150);
lv_obj_align(lmeter, NULL, LV_ALIGN_CENTER, 0, 0);
}
MicroPython¶
Simple Line meter¶
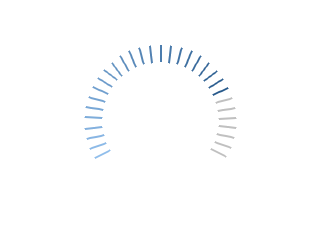
code
# Create a style for the line meter
style_lmeter = lv.style_t()
lv.style_copy(style_lmeter, lv.style_pretty_color)
style_lmeter.line.width = 2
style_lmeter.line.color = lv.color_hex(0xc0c0c0) # Silver
style_lmeter.body.main_color = lv.color_hex(0x91bfed) # Light blue
style_lmeter.body.grad_color = lv.color_hex(0x04386c) # Dark blue
style_lmeter.body.padding.left = 16 # Line length
# Create a line meter
lmeter = lv.lmeter(lv.scr_act())
lmeter.set_range(0, 100) # Set the range
lmeter.set_value(80) # Set the current value
lmeter.set_scale(240, 31) # Set the angle and number of lines
lmeter.set_style(lv.lmeter.STYLE.MAIN, style_lmeter) # Apply the new style
lmeter.set_size(150, 150)
lmeter.align(None, lv.ALIGN.CENTER, 0, 0)
API¶
Typedefs
-
typedef uint8_t
lv_lmeter_style_t
¶
Functions
-
lv_obj_t *
lv_lmeter_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a line meter objects
- Return
pointer to the created line meter
- Parameters
par
: pointer to an object, it will be the parent of the new line metercopy
: pointer to a line meter object, if not NULL then the new object will be copied from it
-
void
lv_lmeter_set_value
(lv_obj_t *lmeter, int16_t value)¶ Set a new value on the line meter
- Parameters
lmeter
: pointer to a line meter objectvalue
: new value
-
void
lv_lmeter_set_range
(lv_obj_t *lmeter, int16_t min, int16_t max)¶ Set minimum and the maximum values of a line meter
- Parameters
lmeter
: pointer to he line meter objectmin
: minimum valuemax
: maximum value
-
void
lv_lmeter_set_scale
(lv_obj_t *lmeter, uint16_t angle, uint16_t line_cnt)¶ Set the scale settings of a line meter
- Parameters
lmeter
: pointer to a line meter objectangle
: angle of the scale (0..360)line_cnt
: number of lines
-
void
lv_lmeter_set_angle_offset
(lv_obj_t *lmeter, uint16_t angle)¶ Set the set an offset for the line meter’s angles to rotate it.
- Parameters
lmeter
: pointer to a line meter objectangle
: angle offset (0..360), rotates clockwise
-
void
lv_lmeter_set_style
(lv_obj_t *lmeter, lv_lmeter_style_t type, lv_style_t *style)¶ Set the styles of a line meter
- Parameters
lmeter
: pointer to a line meter objecttype
: which style should be set (can be onlyLV_LMETER_STYLE_MAIN
)style
: set the style of the line meter
-
int16_t
lv_lmeter_get_value
(const lv_obj_t *lmeter)¶ Get the value of a line meter
- Return
the value of the line meter
- Parameters
lmeter
: pointer to a line meter object
-
int16_t
lv_lmeter_get_min_value
(const lv_obj_t *lmeter)¶ Get the minimum value of a line meter
- Return
the minimum value of the line meter
- Parameters
lmeter
: pointer to a line meter object
-
int16_t
lv_lmeter_get_max_value
(const lv_obj_t *lmeter)¶ Get the maximum value of a line meter
- Return
the maximum value of the line meter
- Parameters
lmeter
: pointer to a line meter object
-
uint16_t
lv_lmeter_get_line_count
(const lv_obj_t *lmeter)¶ Get the scale number of a line meter
- Return
number of the scale units
- Parameters
lmeter
: pointer to a line meter object
-
uint16_t
lv_lmeter_get_scale_angle
(const lv_obj_t *lmeter)¶ Get the scale angle of a line meter
- Return
angle of the scale
- Parameters
lmeter
: pointer to a line meter object
-
uint16_t
lv_lmeter_get_angle_offset
(lv_obj_t *lmeter)¶ get the set an offset for the line meter.
- Return
angle offset (0..360)
- Parameters
lmeter
: pointer to a line meter object
-
const lv_style_t *
lv_lmeter_get_style
(const lv_obj_t *lmeter, lv_lmeter_style_t type)¶ Get the style of a line meter
- Return
pointer to the line meter’s style
- Parameters
lmeter
: pointer to a line meter objecttype
: which style should be get (can be onlyLV_LMETER_STYLE_MAIN
)
-
struct
lv_lmeter_ext_t
¶