Keyboard (lv_kb)¶
Overview¶
The Keyboard object is a special Button matrix with predefined keymaps and other features to realize a virtual keyboard to write text.
Modes¶
The Keyboards have two modes:
LV_KB_MODE_TEXT - Display letters, number, and special characters.
LV_KB_MODE_NUM - Display numbers, +/- sign, and decimal dot.
To set the mode, use lv_kb_set_mode(kb, mode)
. The default is LV_KB_MODE_TEXT.
Assign Text area¶
You can assign a Text area to the Keyboard to automatically put the clicked characters there.
To assign the text area, use lv_kb_set_ta(kb, ta)
.
The assigned text area’s cursor can be managed by the keyboard: when the keyboard is assigned, the previous text area’s cursor will be hidden and the new one will be shown.
When the keyboard is closed by the Ok or Close buttons, the cursor also will be hidden. The cursor manager feature is enabled by lv_kb_set_cursor_manage(kb, true)
. The default is not managed.
New Keymap¶
You can specify a new map (layout) for the keyboard with lv_kb_set_map(kb, map)
and lv_kb_set_ctrl_map(kb, ctrl_map)
.
Learn more about the Button matrix object.
Keep in mind that, using following keywords will have the same effect as with the original map:
LV_SYMBOL_OK - Apply.
SYMBOL_CLOSE - Close.
LV_SYMBOL_LEFT - Move the cursor left.
LV_SYMBOL_RIGHT - Move the cursor right.
“ABC” - Load the uppercase map.
“abc” - Load the lower case map.
“Enter” - New line.
“Bkps” - Delete on the left.
Styles¶
The Keyboard work with 6 styles: a background and 5 button styles for each state.
You can set the styles with lv_kb_set_style(btn, LV_KB_STYLE_..., &style)
.
The background and the buttons use the style.body
properties.
The labels use the style.text
properties of the buttons’ styles.
LV_KB_STYLE_BG - Background style. Uses all
style.body
properties includingpadding
Default:lv_style_pretty
.LV_KB_STYLE_BTN_REL - Style of the released buttons. Default:
lv_style_btn_rel
.LV_KB_STYLE_BTN_PR - Style of the pressed buttons. Default:
lv_style_btn_pr
.LV_KB_STYLE_BTN_TGL_REL - Style of the toggled released buttons. Default:
lv_style_btn_tgl_rel
.LV_KB_STYLE_BTN_TGL_PR - Style of the toggled pressed buttons. Default:
lv_style_btn_tgl_pr
.LV_KB_STYLE_BTN_INA - Style of the inactive buttons. Default:
lv_style_btn_ina
.
Events¶
Besides the Generic events, the following Special events are sent by the keyboards:
LV_EVENT_VALUE_CHANGED - Sent when the button is pressed/released or repeated after long press. The event data is set to the ID of the pressed/released button.
LV_EVENT_APPLY - The Ok button is clicked.
LV_EVENT_CANCEL - The Close button is clicked.
The keyboard has a default event handler callback called lv_kb_def_event_cb
.
It handles the button pressing, map changing, the assigned text area, etc.
You can completely replace it with your custom event handler however, you can call lv_kb_def_event_cb
at the beginning of your event handler to handle the same things as before.
Learn more about Events.
Keys¶
The following Keys are processed by the buttons:
LV_KEY_RIGHT/UP/LEFT/RIGHT - To navigate among the buttons and select one.
LV_KEY_ENTER - To press/release the selected button.
Learn more about Keys.
Examples¶
C¶
Keyboard with text area¶
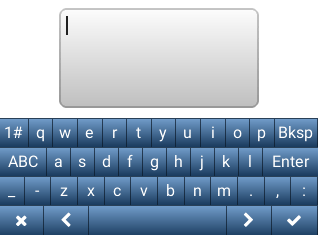
code
#include "lvgl/lvgl.h"
void lv_ex_kb_1(void)
{
/*Create styles for the keyboard*/
static lv_style_t rel_style, pr_style;
lv_style_copy(&rel_style, &lv_style_btn_rel);
rel_style.body.radius = 0;
rel_style.body.border.width = 1;
lv_style_copy(&pr_style, &lv_style_btn_pr);
pr_style.body.radius = 0;
pr_style.body.border.width = 1;
/*Create a keyboard and apply the styles*/
lv_obj_t *kb = lv_kb_create(lv_scr_act(), NULL);
lv_kb_set_cursor_manage(kb, true);
lv_kb_set_style(kb, LV_KB_STYLE_BG, &lv_style_transp_tight);
lv_kb_set_style(kb, LV_KB_STYLE_BTN_REL, &rel_style);
lv_kb_set_style(kb, LV_KB_STYLE_BTN_PR, &pr_style);
/*Create a text area. The keyboard will write here*/
lv_obj_t *ta = lv_ta_create(lv_scr_act(), NULL);
lv_obj_align(ta, NULL, LV_ALIGN_IN_TOP_MID, 0, 10);
lv_ta_set_text(ta, "");
/*Assign the text area to the keyboard*/
lv_kb_set_ta(kb, ta);
}
MicroPython¶
Keyboard with text area¶
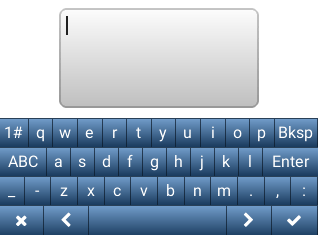
code
# Create styles for the keyboard
rel_style = lv.style_t()
pr_style = lv.style_t()
lv.style_copy(rel_style, lv.style_btn_rel)
rel_style.body.radius = 0
rel_style.body.border.width = 1
lv.style_copy(pr_style, lv.style_btn_pr)
pr_style.body.radius = 0
pr_style.body.border.width = 1
# Create a keyboard and apply the styles
kb = lv.kb(lv.scr_act())
kb.set_cursor_manage(True)
kb.set_style(lv.kb.STYLE.BG, lv.style_transp_tight)
kb.set_style(lv.kb.STYLE.BTN_REL, rel_style)
kb.set_style(lv.kb.STYLE.BTN_PR, pr_style)
# Create a text area. The keyboard will write here
ta = lv.ta(lv.scr_act())
ta.align(None, lv.ALIGN.IN_TOP_MID, 0, 10)
ta.set_text("")
# Assign the text area to the keyboard
kb.set_ta(ta)
API¶
Enums
Functions
-
lv_obj_t *
lv_kb_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a keyboard objects
- Return
pointer to the created keyboard
- Parameters
par
: pointer to an object, it will be the parent of the new keyboardcopy
: pointer to a keyboard object, if not NULL then the new object will be copied from it
-
void
lv_kb_set_ta
(lv_obj_t *kb, lv_obj_t *ta)¶ Assign a Text Area to the Keyboard. The pressed characters will be put there.
- Parameters
kb
: pointer to a Keyboard objectta
: pointer to a Text Area object to write there
-
void
lv_kb_set_mode
(lv_obj_t *kb, lv_kb_mode_t mode)¶ Set a new a mode (text or number map)
- Parameters
kb
: pointer to a Keyboard objectmode
: the mode from ‘lv_kb_mode_t’
-
void
lv_kb_set_cursor_manage
(lv_obj_t *kb, bool en)¶ Automatically hide or show the cursor of the current Text Area
- Parameters
kb
: pointer to a Keyboard objecten
: true: show cursor on the current text area, false: hide cursor
-
void
lv_kb_set_map
(lv_obj_t *kb, const char *map[])¶ Set a new map for the keyboard
- Parameters
kb
: pointer to a Keyboard objectmap
: pointer to a string array to describe the map. See ‘lv_btnm_set_map()’ for more info.
-
void
lv_kb_set_ctrl_map
(lv_obj_t *kb, const lv_btnm_ctrl_t ctrl_map[])¶ Set the button control map (hidden, disabled etc.) for the keyboard. The control map array will be copied and so may be deallocated after this function returns.
- Parameters
kb
: pointer to a keyboard objectctrl_map
: pointer to an array oflv_btn_ctrl_t
control bytes. See:lv_btnm_set_ctrl_map
for more details.
-
void
lv_kb_set_style
(lv_obj_t *kb, lv_kb_style_t type, const lv_style_t *style)¶ Set a style of a keyboard
- Parameters
kb
: pointer to a keyboard objecttype
: which style should be setstyle
: pointer to a style
-
lv_obj_t *
lv_kb_get_ta
(const lv_obj_t *kb)¶ Assign a Text Area to the Keyboard. The pressed characters will be put there.
- Return
pointer to the assigned Text Area object
- Parameters
kb
: pointer to a Keyboard object
-
lv_kb_mode_t
lv_kb_get_mode
(const lv_obj_t *kb)¶ Set a new a mode (text or number map)
- Return
the current mode from ‘lv_kb_mode_t’
- Parameters
kb
: pointer to a Keyboard object
-
bool
lv_kb_get_cursor_manage
(const lv_obj_t *kb)¶ Get the current cursor manage mode.
- Return
true: show cursor on the current text area, false: hide cursor
- Parameters
kb
: pointer to a Keyboard object
-
const char **
lv_kb_get_map_array
(const lv_obj_t *kb)¶ Get the current map of a keyboard
- Return
the current map
- Parameters
kb
: pointer to a keyboard object
-
const lv_style_t *
lv_kb_get_style
(const lv_obj_t *kb, lv_kb_style_t type)¶ Get a style of a keyboard
- Return
style pointer to a style
- Parameters
kb
: pointer to a keyboard objecttype
: which style should be get
-
void
lv_kb_def_event_cb
(lv_obj_t *kb, lv_event_t event)¶ Default keyboard event to add characters to the Text area and change the map. If a custom
event_cb
is added to the keyboard this function be called from it to handle the button clicks- Parameters
kb
: pointer to a keyboardevent
: the triggering event
-
struct
lv_kb_ext_t
¶