LED (lv_led)¶
Overview¶
The LEDs are rectangle-like (or circle) object.
Brightness¶
You can set their brightness with lv_led_set_bright(led, bright)
. The brightness should be between 0 (darkest) and 255 (lightest).
Toggle¶
Use lv_led_on(led)
and lv_led_off(led)
to set the brightness to a predefined ON or OFF value. The lv_led_toggle(led)
toggles between the ON and OFF state.
Styles¶
The LED uses one style which can be set by lv_led_set_style(led, LV_LED_STYLE_MAIN, &style)
.
To determine the appearance, the style.body
properties are used.
The colors are darkened and shadow width is reduced at a lower brightness and gains its original value at brightness 255 to show a lighting effect.
The default style is: lv_style_pretty_color
. Note that, the LED doesn’t look like a LED with the default style so you should create your style. See the example below.
Example¶
C¶
LED with custom style¶
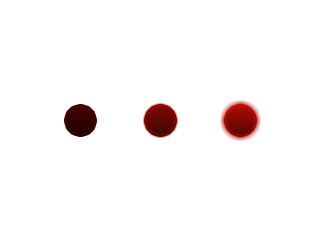
code
#include "lvgl/lvgl.h"
void lv_ex_led_1(void)
{
/*Create a style for the LED*/
static lv_style_t style_led;
lv_style_copy(&style_led, &lv_style_pretty_color);
style_led.body.radius = LV_RADIUS_CIRCLE;
style_led.body.main_color = LV_COLOR_MAKE(0xb5, 0x0f, 0x04);
style_led.body.grad_color = LV_COLOR_MAKE(0x50, 0x07, 0x02);
style_led.body.border.color = LV_COLOR_MAKE(0xfa, 0x0f, 0x00);
style_led.body.border.width = 3;
style_led.body.border.opa = LV_OPA_30;
style_led.body.shadow.color = LV_COLOR_MAKE(0xb5, 0x0f, 0x04);
style_led.body.shadow.width = 5;
/*Create a LED and switch it OFF*/
lv_obj_t * led1 = lv_led_create(lv_scr_act(), NULL);
lv_led_set_style(led1, LV_LED_STYLE_MAIN, &style_led);
lv_obj_align(led1, NULL, LV_ALIGN_CENTER, -80, 0);
lv_led_off(led1);
/*Copy the previous LED and set a brightness*/
lv_obj_t * led2 = lv_led_create(lv_scr_act(), led1);
lv_obj_align(led2, NULL, LV_ALIGN_CENTER, 0, 0);
lv_led_set_bright(led2, 190);
/*Copy the previous LED and switch it ON*/
lv_obj_t * led3 = lv_led_create(lv_scr_act(), led1);
lv_obj_align(led3, NULL, LV_ALIGN_CENTER, 80, 0);
lv_led_on(led3);
}
MicroPython¶
LED with custom style¶
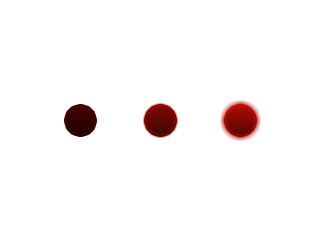
code
# Create a style for the LED
style_led = lv.style_t()
lv.style_copy(style_led, lv.style_pretty_color)
style_led.body.radius = 800 # large enough to draw a circle
style_led.body.main_color = lv.color_make(0xb5, 0x0f, 0x04)
style_led.body.grad_color = lv.color_make(0x50, 0x07, 0x02)
style_led.body.border.color = lv.color_make(0xfa, 0x0f, 0x00)
style_led.body.border.width = 3
style_led.body.border.opa = lv.OPA._30
style_led.body.shadow.color = lv.color_make(0xb5, 0x0f, 0x04)
style_led.body.shadow.width = 5
# Create a LED and switch it OFF
led1 = lv.led(lv.scr_act())
led1.set_style(lv.led.STYLE.MAIN, style_led)
led1.align(None, lv.ALIGN.CENTER, -80, 0)
led1.off()
# Copy the previous LED and set a brightness
led2 = lv.led(lv.scr_act(), led1)
led2.align(None, lv.ALIGN.CENTER, 0, 0)
led2.set_bright(190)
# Copy the previous LED and switch it ON
led3 = lv.led(lv.scr_act(), led1)
led3.align(None, lv.ALIGN.CENTER, 80, 0)
led3.on()
API¶
Typedefs
-
typedef uint8_t
lv_led_style_t
¶
Functions
-
lv_obj_t *
lv_led_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a led objects
- Return
pointer to the created led
- Parameters
par
: pointer to an object, it will be the parent of the new ledcopy
: pointer to a led object, if not NULL then the new object will be copied from it
-
void
lv_led_set_bright
(lv_obj_t *led, uint8_t bright)¶ Set the brightness of a LED object
- Parameters
led
: pointer to a LED objectbright
: 0 (max. dark) … 255 (max. light)
-
void
lv_led_toggle
(lv_obj_t *led)¶ Toggle the state of a LED
- Parameters
led
: pointer to a LED object
-
void
lv_led_set_style
(lv_obj_t *led, lv_led_style_t type, const lv_style_t *style)¶ Set the style of a led
- Parameters
led
: pointer to a led objecttype
: which style should be set (can be onlyLV_LED_STYLE_MAIN
)style
: pointer to a style
-
uint8_t
lv_led_get_bright
(const lv_obj_t *led)¶ Get the brightness of a LEd object
- Return
bright 0 (max. dark) … 255 (max. light)
- Parameters
led
: pointer to LED object
-
const lv_style_t *
lv_led_get_style
(const lv_obj_t *led, lv_led_style_t type)¶ Get the style of an led object
- Return
pointer to the led’s style
- Parameters
led
: pointer to an led objecttype
: which style should be get (can be onlyLV_CHART_STYLE_MAIN
)