Switch (lv_sw)¶
Overview¶
The Switch can be used to turn on/off something. The look like a little slider.
Change state¶
The state of the switch can be changed by
Clicking on it
Sliding it
Using
lv_sw_on(sw, LV_ANIM_ON/OFF)
,lv_sw_off(sw, LV_ANIM_ON/OFF)
orlv_sw_toggle(sw, LV_ANOM_ON/OFF)
functions
Animation time¶
The time of animations, when the switch changes state, can be adjusted with lv_sw_set_anim_time(sw, anim_time)
.
Styles¶
You can modify the Switch’s styles with lv_sw_set_style(sw, LV_SW_STYLE_..., &style)
.
LV_SW_STYLE_BG Style of the background. All
style.body
properties are used. Thepadding
values make the Switch smaller than the knob. (negative value makes is larger)LV_SW_STYLE_INDIC Style of the indicator. All
style.body
properties are used. Thepadding
values make the indicator smaller than the background.LV_SW_STYLE_KNOB_OFF Style of the knob when the switch is off. The
style.body
properties are used except padding.LV_SW_STYLE_KNOB_ON Style of the knob when the switch is on. The
style.body
properties are used except padding.
Events¶
Besides the Generic events the following Special events are sent by the Switch:
LV_EVENT_VALUE_CHANGED Sent when the switch changes state.
Keys¶
LV_KEY_UP, LV_KEY_RIGHT Turn on the slider
LV_KEY_DOWN, LV_KEY_LEFT Turn off the slider
Learn more about Keys.
Example¶
C¶
Simple Switch¶
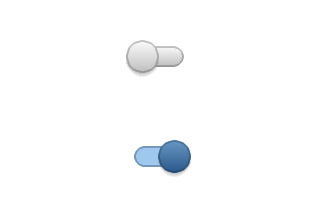
code
#include "lvgl/lvgl.h"
#include <stdio.h>
static void event_handler(lv_obj_t * obj, lv_event_t event)
{
if(event == LV_EVENT_VALUE_CHANGED) {
printf("State: %s\n", lv_sw_get_state(obj) ? "On" : "Off");
}
}
void lv_ex_sw_1(void)
{
/*Create styles for the switch*/
static lv_style_t bg_style;
static lv_style_t indic_style;
static lv_style_t knob_on_style;
static lv_style_t knob_off_style;
lv_style_copy(&bg_style, &lv_style_pretty);
bg_style.body.radius = LV_RADIUS_CIRCLE;
bg_style.body.padding.top = 6;
bg_style.body.padding.bottom = 6;
lv_style_copy(&indic_style, &lv_style_pretty_color);
indic_style.body.radius = LV_RADIUS_CIRCLE;
indic_style.body.main_color = lv_color_hex(0x9fc8ef);
indic_style.body.grad_color = lv_color_hex(0x9fc8ef);
indic_style.body.padding.left = 0;
indic_style.body.padding.right = 0;
indic_style.body.padding.top = 0;
indic_style.body.padding.bottom = 0;
lv_style_copy(&knob_off_style, &lv_style_pretty);
knob_off_style.body.radius = LV_RADIUS_CIRCLE;
knob_off_style.body.shadow.width = 4;
knob_off_style.body.shadow.type = LV_SHADOW_BOTTOM;
lv_style_copy(&knob_on_style, &lv_style_pretty_color);
knob_on_style.body.radius = LV_RADIUS_CIRCLE;
knob_on_style.body.shadow.width = 4;
knob_on_style.body.shadow.type = LV_SHADOW_BOTTOM;
/*Create a switch and apply the styles*/
lv_obj_t *sw1 = lv_sw_create(lv_scr_act(), NULL);
lv_sw_set_style(sw1, LV_SW_STYLE_BG, &bg_style);
lv_sw_set_style(sw1, LV_SW_STYLE_INDIC, &indic_style);
lv_sw_set_style(sw1, LV_SW_STYLE_KNOB_ON, &knob_on_style);
lv_sw_set_style(sw1, LV_SW_STYLE_KNOB_OFF, &knob_off_style);
lv_obj_align(sw1, NULL, LV_ALIGN_CENTER, 0, -50);
lv_obj_set_event_cb(sw1, event_handler);
/*Copy the first switch and turn it ON*/
lv_obj_t *sw2 = lv_sw_create(lv_scr_act(), sw1);
lv_sw_on(sw2, LV_ANIM_ON);
lv_obj_align(sw2, NULL, LV_ALIGN_CENTER, 0, 50);
}
MicroPython¶
Simple Switch¶
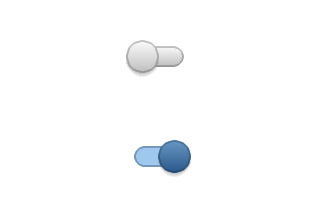
code
def event_handler(obj, event):
if event == lv.EVENT.VALUE_CHANGED:
print("State: %s" % ("On" if obj.get_state() else "Off"))
# Create styles for the switch
bg_style = lv.style_t()
indic_style = lv.style_t()
knob_on_style = lv.style_t()
knob_off_style = lv.style_t()
lv.style_copy(bg_style, lv.style_pretty)
bg_style.body.radius = 800
bg_style.body.padding.top = 6
bg_style.body.padding.bottom = 6
lv.style_copy(indic_style, lv.style_pretty_color)
indic_style.body.radius = 800
indic_style.body.main_color = lv.color_hex(0x9fc8ef)
indic_style.body.grad_color = lv.color_hex(0x9fc8ef)
indic_style.body.padding.left = 0
indic_style.body.padding.right = 0
indic_style.body.padding.top = 0
indic_style.body.padding.bottom = 0
lv.style_copy(knob_off_style, lv.style_pretty)
knob_off_style.body.radius = 800
knob_off_style.body.shadow.width = 4
knob_off_style.body.shadow.type = lv.SHADOW.BOTTOM
lv.style_copy(knob_on_style, lv.style_pretty_color)
knob_on_style.body.radius = 800
knob_on_style.body.shadow.width = 4
knob_on_style.body.shadow.type = lv.SHADOW.BOTTOM
# Create a switch and apply the styles
sw1 = lv.sw(lv.scr_act())
sw1.set_style(lv.sw.STYLE.BG, bg_style)
sw1.set_style(lv.sw.STYLE.INDIC, indic_style)
sw1.set_style(lv.sw.STYLE.KNOB_ON, knob_on_style)
sw1.set_style(lv.sw.STYLE.KNOB_OFF, knob_off_style)
sw1.align(None, lv.ALIGN.CENTER, 0, -50)
sw1.set_event_cb(event_handler)
# Copy the first switch and turn it ON
sw2 = lv.sw(lv.scr_act(), sw1)
sw2.on(lv.ANIM.ON)
sw2.align(None, lv.ALIGN.CENTER, 0, 50)
sw2.set_event_cb(lambda o,e: None)
API¶
Typedefs
-
typedef uint8_t
lv_sw_style_t
¶
Enums
Functions
-
lv_obj_t *
lv_sw_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a switch objects
- Return
pointer to the created switch
- Parameters
par
: pointer to an object, it will be the parent of the new switchcopy
: pointer to a switch object, if not NULL then the new object will be copied from it
-
void
lv_sw_on
(lv_obj_t *sw, lv_anim_enable_t anim)¶ Turn ON the switch
- Parameters
sw
: pointer to a switch objectanim
: LV_ANIM_ON: set the value with an animation; LV_ANIM_OFF: change the value immediately
-
void
lv_sw_off
(lv_obj_t *sw, lv_anim_enable_t anim)¶ Turn OFF the switch
- Parameters
sw
: pointer to a switch objectanim
: LV_ANIM_ON: set the value with an animation; LV_ANIM_OFF: change the value immediately
-
bool
lv_sw_toggle
(lv_obj_t *sw, lv_anim_enable_t anim)¶ Toggle the position of the switch
- Return
resulting state of the switch.
- Parameters
sw
: pointer to a switch objectanim
: LV_ANIM_ON: set the value with an animation; LV_ANIM_OFF: change the value immediately
-
void
lv_sw_set_style
(lv_obj_t *sw, lv_sw_style_t type, const lv_style_t *style)¶ Set a style of a switch
- Parameters
sw
: pointer to a switch objecttype
: which style should be setstyle
: pointer to a style
-
void
lv_sw_set_anim_time
(lv_obj_t *sw, uint16_t anim_time)¶ Set the animation time of the switch
- Return
style pointer to a style
- Parameters
sw
: pointer to a switch objectanim_time
: animation time
-
bool
lv_sw_get_state
(const lv_obj_t *sw)¶ Get the state of a switch
- Return
false: OFF; true: ON
- Parameters
sw
: pointer to a switch object
-
const lv_style_t *
lv_sw_get_style
(const lv_obj_t *sw, lv_sw_style_t type)¶ Get a style of a switch
- Return
style pointer to a style
- Parameters
sw
: pointer to a switch objecttype
: which style should be get
-
struct
lv_sw_ext_t
¶