Page (lv_page)¶
Overview¶
The Page consist of two Containers on each other:
a background (or base)
a top which is scrollable.
The background object can be referenced as the page itself like: lv_obj_set_width(page, 100)
.
If you create a child on the page it will be automatically moved to the scrollable container. If the scrollable container becomes larger then the background it can be *scrolled by dragging (like the lists on smartphones).
By default, the scrollable’s has LV_FIT_FILL
auto fit in all directions.
It means the scrollable size will be the same as the background’s size (minus the paddings) while the children are in the background.
But when an object is positioned out of the background the scrollable size will be increased to involve it.
Scrollbars¶
Scrollbars can be shown according to four policies:
LV_SB_MODE_OFF Never show scrollbars
LV_SB_MODE_ON Always show scrollbars
LV_SB_MODE_DRAG Show scrollbars when the page is being dragged
LV_SB_MODE_AUTO Show scrollbars when the scrollable container is large enough to be scrolled
You can set scroll bar show policy by: lv_page_set_sb_mode(page, SB_MODE)
. The default value is LV_SB_MODE_AUTO
.
Glue object¶
You can glue children to the page. In this case, you can scroll the page by dragging the child object.
It can be enabled by the lv_page_glue_obj(child, true)
.
Focus object¶
You can focus on an object on a page with lv_page_focus(page, child, LV_ANIM_ONO/FF)
.
It will move the scrollable container to show a child. The time of the animation can be set by lv_page_set_anim_time(page, anim_time)
in milliseconds.
Edge flash¶
A circle-like effect can be shown if the list reached the most top/bottom/left/right position. lv_page_set_edge_flash(list, en)
enables this feature.
Scroll propagation¶
If the list is created on an other scrollable element (like an other page) and the Page can’t be scrolled further the scrolling can be propagated to the parent to continue the scrolling on the parent.
It can be enabled with lv_page_set_scroll_propagation(list, true)
Scrollable API¶
There are functions to directly set/get the scrollable’s attributes:
lv_page_get_scrl()
lv_page_set_scrl_fit/fint2/fit4()
lv_page_set_scrl_width()
lv_page_set_scrl_height()
lv_page_set_scrl_layout()
Notes¶
The background draws its border when the scrollable is drawn. It ensures that the page always will have a closed shape even if the scrollable has the same color as the Page’s parent.
Styles¶
Use lv_page_set_style(page, LV_PAGE_STYLE_..., &style)
to set a new style for an element of the page:
LV_PAGE_STYLE_BG background’s style which uses all
style.body
properties (default:lv_style_pretty_color
)LV_PAGE_STYLE_SCRL scrollable’s style which uses all
style.body
properties (default:lv_style_pretty
)LV_PAGE_STYLE_SB scrollbar’s style which uses all
style.body
properties.padding.right/bottom
sets horizontal and vertical the scrollbars’ padding respectively and thepadding.inner
sets the scrollbar’s width. (default:lv_style_pretty_color
)
Events¶
Only the Generic events are sent by the object type.
The scrollable object has a default event callback which propagates the following events to the background object:
LV_EVENT_PRESSED
, LV_EVENT_PRESSING
, LV_EVENT_PRESS_LOST
,LV_EVENT_RELEASED
, LV_EVENT_SHORT_CLICKED
, LV_EVENT_CLICKED
, LV_EVENT_LONG_PRESSED
, LV_EVENT_LONG_PRESSED_REPEAT
Learn more about Events.
##Keys
The following Keys are processed by the Page:
LV_KEY_RIGHT/LEFT/UP/DOWN Scroll the page
Learn more about Keys.
Example¶
C¶
Page with scrollbar¶
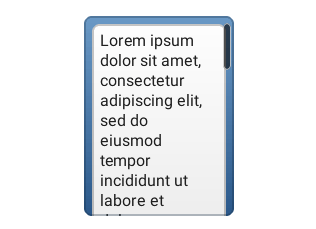
code
#include "lvgl/lvgl.h"
void lv_ex_page_1(void)
{
/*Create a scroll bar style*/
static lv_style_t style_sb;
lv_style_copy(&style_sb, &lv_style_plain);
style_sb.body.main_color = LV_COLOR_BLACK;
style_sb.body.grad_color = LV_COLOR_BLACK;
style_sb.body.border.color = LV_COLOR_WHITE;
style_sb.body.border.width = 1;
style_sb.body.border.opa = LV_OPA_70;
style_sb.body.radius = LV_RADIUS_CIRCLE;
style_sb.body.opa = LV_OPA_60;
style_sb.body.padding.right = 3;
style_sb.body.padding.bottom = 3;
style_sb.body.padding.inner = 8; /*Scrollbar width*/
/*Create a page*/
lv_obj_t * page = lv_page_create(lv_scr_act(), NULL);
lv_obj_set_size(page, 150, 200);
lv_obj_align(page, NULL, LV_ALIGN_CENTER, 0, 0);
lv_page_set_style(page, LV_PAGE_STYLE_SB, &style_sb); /*Set the scrollbar style*/
/*Create a label on the page*/
lv_obj_t * label = lv_label_create(page, NULL);
lv_label_set_long_mode(label, LV_LABEL_LONG_BREAK); /*Automatically break long lines*/
lv_obj_set_width(label, lv_page_get_fit_width(page)); /*Set the label width to max value to not show hor. scroll bars*/
lv_label_set_text(label, "Lorem ipsum dolor sit amet, consectetur adipiscing elit,\n"
"sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.\n"
"Ut enim ad minim veniam, quis nostrud exercitation ullamco\n"
"laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure\n"
"dolor in reprehenderit in voluptate velit esse cillum dolore\n"
"eu fugiat nulla pariatur.\n"
"Excepteur sint occaecat cupidatat non proident, sunt in culpa\n"
"qui officia deserunt mollit anim id est laborum.");
}
MicroPython¶
Page with scrollbar¶
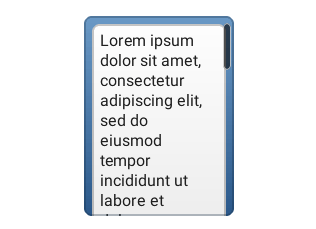
code
# Create a scroll bar style
style_sb = lv.style_t()
lv.style_copy(style_sb, lv.style_plain)
style_sb.body.main_color = lv.color_make(0,0,0)
style_sb.body.grad_color = lv.color_make(0,0,0)
style_sb.body.border.color = lv.color_make(0xff,0xff,0xff)
style_sb.body.border.width = 1
style_sb.body.border.opa = lv.OPA._70
style_sb.body.radius = 800 # large enough to make a circle
style_sb.body.opa = lv.OPA._60
style_sb.body.padding.right = 3
style_sb.body.padding.bottom = 3
style_sb.body.padding.inner = 8 # Scrollbar width
# Create a page
page = lv.page(lv.scr_act())
page.set_size(150, 200)
page.align(None, lv.ALIGN.CENTER, 0, 0)
page.set_style(lv.page.STYLE.SB, style_sb) # Set the scrollbar style
# Create a label on the page
label = lv.label(page)
label.set_long_mode(lv.label.LONG.BREAK) # Automatically break long lines
label.set_width(page.get_fit_width()) # Set the label width to max value to not show hor. scroll bars
label.set_text("""Lorem ipsum dolor sit amet, consectetur adipiscing elit,
sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.
Ut enim ad minim veniam, quis nostrud exercitation ullamco
laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure
dolor in reprehenderit in voluptate velit esse cillum dolore
eu fugiat nulla pariatur.
Excepteur sint occaecat cupidatat non proident, sunt in culpa
qui officia deserunt mollit anim id est laborum.""")
API¶
Typedefs
-
typedef uint8_t
lv_sb_mode_t
¶
-
typedef uint8_t
lv_page_edge_t
¶
-
typedef uint8_t
lv_page_style_t
¶
Enums
-
enum [anonymous]¶
Scrollbar modes: shows when should the scrollbars be visible
Values:
-
enumerator
LV_SB_MODE_OFF
= 0x0¶ Never show scrollbars
-
enumerator
LV_SB_MODE_ON
= 0x1¶ Always show scrollbars
-
enumerator
LV_SB_MODE_DRAG
= 0x2¶ Show scrollbars when page is being dragged
-
enumerator
LV_SB_MODE_AUTO
= 0x3¶ Show scrollbars when the scrollable container is large enough to be scrolled
-
enumerator
LV_SB_MODE_HIDE
= 0x4¶ Hide the scroll bar temporally
-
enumerator
LV_SB_MODE_UNHIDE
= 0x5¶ Unhide the previously hidden scrollbar. Recover it’s type too
-
enumerator
Functions
-
lv_obj_t *
lv_page_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a page objects
- Return
pointer to the created page
- Parameters
par
: pointer to an object, it will be the parent of the new pagecopy
: pointer to a page object, if not NULL then the new object will be copied from it
-
void
lv_page_clean
(lv_obj_t *page)¶ Delete all children of the scrl object, without deleting scrl child.
- Parameters
page
: pointer to an object
-
lv_obj_t *
lv_page_get_scrl
(const lv_obj_t *page)¶ Get the scrollable object of a page
- Return
pointer to a container which is the scrollable part of the page
- Parameters
page
: pointer to a page object
-
uint16_t
lv_page_get_anim_time
(const lv_obj_t *page)¶ Get the animation time
- Return
the animation time in milliseconds
- Parameters
page
: pointer to a page object
-
void
lv_page_set_sb_mode
(lv_obj_t *page, lv_sb_mode_t sb_mode)¶ Set the scroll bar mode on a page
- Parameters
page
: pointer to a page objectsb_mode
: the new mode from ‘lv_page_sb.mode_t’ enum
-
void
lv_page_set_anim_time
(lv_obj_t *page, uint16_t anim_time)¶ Set the animation time for the page
- Parameters
page
: pointer to a page objectanim_time
: animation time in milliseconds
-
void
lv_page_set_scroll_propagation
(lv_obj_t *page, bool en)¶ Enable the scroll propagation feature. If enabled then the page will move its parent if there is no more space to scroll.
- Parameters
page
: pointer to a Pageen
: true or false to enable/disable scroll propagation
-
void
lv_page_set_edge_flash
(lv_obj_t *page, bool en)¶ Enable the edge flash effect. (Show an arc when the an edge is reached)
- Parameters
page
: pointer to a Pageen
: true or false to enable/disable end flash
-
void
lv_page_set_scrl_fit4
(lv_obj_t *page, lv_fit_t left, lv_fit_t right, lv_fit_t top, lv_fit_t bottom)¶ Set the fit policy in all 4 directions separately. It tell how to change the page size automatically.
- Parameters
page
: pointer to a page objectleft
: left fit policy fromlv_fit_t
right
: right fit policy fromlv_fit_t
top
: bottom fit policy fromlv_fit_t
bottom
: bottom fit policy fromlv_fit_t
-
void
lv_page_set_scrl_fit2
(lv_obj_t *page, lv_fit_t hor, lv_fit_t ver)¶ Set the fit policy horizontally and vertically separately. It tell how to change the page size automatically.
- Parameters
page
: pointer to a page objecthot
: horizontal fit policy fromlv_fit_t
ver
: vertical fit policy fromlv_fit_t
-
void
lv_page_set_scrl_fit
(lv_obj_t *page, lv_fit_t fit)¶ Set the fit policyin all 4 direction at once. It tell how to change the page size automatically.
- Parameters
page
: pointer to a button objectfit
: fit policy fromlv_fit_t
-
void
lv_page_set_scrl_width
(lv_obj_t *page, lv_coord_t w)¶ Set width of the scrollable part of a page
- Parameters
page
: pointer to a page objectw
: the new width of the scrollable (it ha no effect is horizontal fit is enabled)
-
void
lv_page_set_scrl_height
(lv_obj_t *page, lv_coord_t h)¶ Set height of the scrollable part of a page
- Parameters
page
: pointer to a page objecth
: the new height of the scrollable (it ha no effect is vertical fit is enabled)
-
void
lv_page_set_scrl_layout
(lv_obj_t *page, lv_layout_t layout)¶ Set the layout of the scrollable part of the page
- Parameters
page
: pointer to a page objectlayout
: a layout from ‘lv_cont_layout_t’
-
void
lv_page_set_style
(lv_obj_t *page, lv_page_style_t type, const lv_style_t *style)¶ Set a style of a page
- Parameters
page
: pointer to a page objecttype
: which style should be setstyle
: pointer to a style
-
lv_sb_mode_t
lv_page_get_sb_mode
(const lv_obj_t *page)¶ Set the scroll bar mode on a page
- Return
the mode from ‘lv_page_sb.mode_t’ enum
- Parameters
page
: pointer to a page object
-
bool
lv_page_get_scroll_propagation
(lv_obj_t *page)¶ Get the scroll propagation property
- Return
true or false
- Parameters
page
: pointer to a Page
-
bool
lv_page_get_edge_flash
(lv_obj_t *page)¶ Get the edge flash effect property.
- Parameters
page
: pointer to a Page return true or false
-
lv_coord_t
lv_page_get_fit_width
(lv_obj_t *page)¶ Get that width which can be set to the children to still not cause overflow (show scrollbars)
- Return
the width which still fits into the page
- Parameters
page
: pointer to a page object
-
lv_coord_t
lv_page_get_fit_height
(lv_obj_t *page)¶ Get that height which can be set to the children to still not cause overflow (show scrollbars)
- Return
the height which still fits into the page
- Parameters
page
: pointer to a page object
-
lv_coord_t
lv_page_get_scrl_width
(const lv_obj_t *page)¶ Get width of the scrollable part of a page
- Return
the width of the scrollable
- Parameters
page
: pointer to a page object
-
lv_coord_t
lv_page_get_scrl_height
(const lv_obj_t *page)¶ Get height of the scrollable part of a page
- Return
the height of the scrollable
- Parameters
page
: pointer to a page object
-
lv_layout_t
lv_page_get_scrl_layout
(const lv_obj_t *page)¶ Get the layout of the scrollable part of a page
- Return
the layout from ‘lv_cont_layout_t’
- Parameters
page
: pointer to page object
-
lv_fit_t
lv_page_get_scrl_fit_left
(const lv_obj_t *page)¶ Get the left fit mode
- Return
an element of
lv_fit_t
- Parameters
page
: pointer to a page object
-
lv_fit_t
lv_page_get_scrl_fit_right
(const lv_obj_t *page)¶ Get the right fit mode
- Return
an element of
lv_fit_t
- Parameters
page
: pointer to a page object
-
lv_fit_t
lv_page_get_scrl_fit_top
(const lv_obj_t *page)¶ Get the top fit mode
- Return
an element of
lv_fit_t
- Parameters
page
: pointer to a page object
-
lv_fit_t
lv_page_get_scrl_fit_bottom
(const lv_obj_t *page)¶ Get the bottom fit mode
- Return
an element of
lv_fit_t
- Parameters
page
: pointer to a page object
-
const lv_style_t *
lv_page_get_style
(const lv_obj_t *page, lv_page_style_t type)¶ Get a style of a page
- Return
style pointer to a style
- Parameters
page
: pointer to page objecttype
: which style should be get
-
bool
lv_page_on_edge
(lv_obj_t *page, lv_page_edge_t edge)¶ Find whether the page has been scrolled to a certain edge.
- Return
true if the page is on the specified edge
- Parameters
page
: Page objectedge
: Edge to check
-
void
lv_page_glue_obj
(lv_obj_t *obj, bool glue)¶ Glue the object to the page. After it the page can be moved (dragged) with this object too.
- Parameters
obj
: pointer to an object on a pageglue
: true: enable glue, false: disable glue
-
void
lv_page_focus
(lv_obj_t *page, const lv_obj_t *obj, lv_anim_enable_t anim_en)¶ Focus on an object. It ensures that the object will be visible on the page.
- Parameters
page
: pointer to a page objectobj
: pointer to an object to focus (must be on the page)anim_en
: LV_ANIM_ON to focus with animation; LV_ANIM_OFF to focus without animation
-
void
lv_page_scroll_hor
(lv_obj_t *page, lv_coord_t dist)¶ Scroll the page horizontally
- Parameters
page
: pointer to a page objectdist
: the distance to scroll (< 0: scroll left; > 0 scroll right)
-
struct
lv_page_ext_t
¶ Public Members
-
const lv_style_t *
style
¶
-
lv_area_t
hor_area
¶
-
lv_area_t
ver_area
¶
-
uint8_t
hor_draw
¶
-
uint8_t
ver_draw
¶
-
lv_sb_mode_t
mode
¶
-
struct lv_page_ext_t::[anonymous]
sb
¶
-
lv_anim_value_t
state
¶
-
uint8_t
enabled
¶
-
uint8_t
top_ip
¶
-
uint8_t
bottom_ip
¶
-
uint8_t
right_ip
¶
-
uint8_t
left_ip
¶
-
struct lv_page_ext_t::[anonymous]
edge_flash
¶
-
uint16_t
anim_time
¶
-
uint8_t
scroll_prop
¶
-
uint8_t
scroll_prop_ip
¶
-
const lv_style_t *