Calendar (lv_calendar)¶
Overview¶
The Calendar object is a classic calendar which can:
highlight the current day and week
highlight any user-defined dates
display the name of the days
go the next/previous month by button click
highlight the clicked day
To set and get dates in the calendar, the lv_calendar_date_t
type is used which is a structure with year
, month
and day
fields.
Current date¶
To set the current date (today), use the lv_calendar_set_today_date(calendar, &today_date)
function.
Shown date¶
To set the shown date, use lv_calendar_set_shown_date(calendar, &shown_date)
;
Highlighted days¶
The list of highlighted dates should be stored in a lv_calendar_date_t
array loaded by lv_calendar_set_highlighted_dates(calendar, &highlighted_dates)
.
Only the arrays pointer will be saved so the array should be a static or global variable.
Name of the days¶
The name of the days can be adjusted with lv_calendar_set_day_names(calendar, day_names)
where day_names
looks like const char * day_names[7] = {"Su", "Mo", ...};
Name of the months¶
Similarly to day_names
, the name of the month can be set with lv_calendar_set_month_names(calendar, month_names_array)
.
Styles¶
You can set the styles with lv_calendar_set_style(btn, LV_CALENDAR_STYLE_..., &style)
.
LV_CALENDAR_STYLE_BG - Style of the background using the
body
properties and the style of the date numbers using thetext
properties.body.padding.left/right/bottom
padding will be added on the edges around the date numbers.LV_CALENDAR_STYLE_HEADER - Style of the header where the current year and month is displayed.
body
andtext
properties are used.LV_CALENDAR_STYLE_HEADER_PR - Pressed header style, used when the next/prev. month button is being pressed.
text
properties are used by the arrows.LV_CALENDAR_STYLE_DAY_NAMES - Style of the day names.
text
properties are used by the ‘day’ texts andbody.padding.top
determines the space above the day names.LV_CALENDAR_STYLE_HIGHLIGHTED_DAYS -
text
properties are used to adjust the style of the highlights days.LV_CALENDAR_STYLE_INACTIVE_DAYS -
text
properties are used to adjust the style of the visible days of previous/next month.LV_CALENDAR_STYLE_WEEK_BOX -
body
properties are used to set the style of the week box.LV_CALENDAR_STYLE_TODAY_BOX -
body
andtext
properties are used to set the style of the today box.
Events¶
Besides the Generic events, the following Special events are sent by the calendars: LV_EVENT_VALUE_CHANGED is sent when the current month has changed.
In Input device related events, lv_calendar_get_pressed_date(calendar)
tells which day is currently being pressed or return NULL
if no date is pressed.
Example¶
C¶
Calendar with day select¶
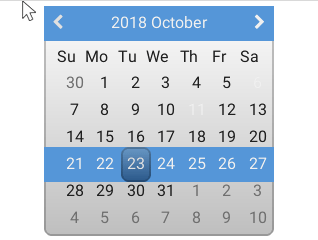
code
#include "lvgl/lvgl.h"
static void event_handler(lv_obj_t * obj, lv_event_t event)
{
if(event == LV_EVENT_CLICKED) {
lv_calendar_date_t * date = lv_calendar_get_pressed_date(obj);
if(date) {
lv_calendar_set_today_date(obj, date);
}
}
}
void lv_ex_calendar_1(void)
{
lv_obj_t * calendar = lv_calendar_create(lv_scr_act(), NULL);
lv_obj_set_size(calendar, 230, 230);
lv_obj_align(calendar, NULL, LV_ALIGN_CENTER, 0, 0);
lv_obj_set_event_cb(calendar, event_handler);
/*Set the today*/
lv_calendar_date_t today;
today.year = 2018;
today.month = 10;
today.day = 23;
lv_calendar_set_today_date(calendar, &today);
lv_calendar_set_showed_date(calendar, &today);
/*Highlight some days*/
static lv_calendar_date_t highlihted_days[3]; /*Only it's pointer will be saved so should be static*/
highlihted_days[0].year = 2018;
highlihted_days[0].month = 10;
highlihted_days[0].day = 6;
highlihted_days[1].year = 2018;
highlihted_days[1].month = 10;
highlihted_days[1].day = 11;
highlihted_days[2].year = 2018;
highlihted_days[2].month = 11;
highlihted_days[2].day = 22;
lv_calendar_set_highlighted_dates(calendar, highlihted_days, 3);
}
MicroPython¶
Calendar with day select¶
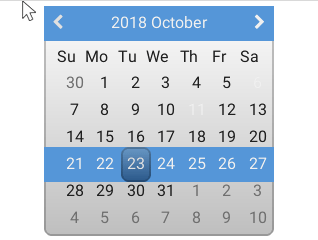
code
def event_handler(obj, event):
if event == lv.EVENT.CLICKED:
date = obj.get_pressed_date()
if date is not None:
obj.set_today_date(date)
calendar = lv.calendar(lv.scr_act())
calendar.set_size(230, 230)
calendar.align(None, lv.ALIGN.CENTER, 0, 0)
calendar.set_event_cb(event_handler)
# Set the today
today = lv.calendar_date_t()
today.year = 2018
today.month = 10
today.day = 23
calendar.set_today_date(today)
calendar.set_showed_date(today)
highlihted_days = [
lv.calendar_date_t({'year':2018, 'month':10, 'day':6}),
lv.calendar_date_t({'year':2018, 'month':10, 'day':11}),
lv.calendar_date_t({'year':2018, 'month':11, 'day':22})
]
calendar.set_highlighted_dates(highlihted_days, len(highlihted_days))
API¶
Typedefs
-
typedef uint8_t
lv_calendar_style_t
¶
Enums
-
enum [anonymous]¶
Calendar styles
Values:
-
enumerator
LV_CALENDAR_STYLE_BG
¶ Background and “normal” date numbers style
-
enumerator
LV_CALENDAR_STYLE_HEADER
¶
-
enumerator
LV_CALENDAR_STYLE_HEADER_PR
¶ Calendar header style
-
enumerator
LV_CALENDAR_STYLE_DAY_NAMES
¶ Calendar header style (when pressed)
-
enumerator
LV_CALENDAR_STYLE_HIGHLIGHTED_DAYS
¶ Day name style
-
enumerator
LV_CALENDAR_STYLE_INACTIVE_DAYS
¶ Highlighted day style
-
enumerator
LV_CALENDAR_STYLE_WEEK_BOX
¶ Inactive day style
-
enumerator
LV_CALENDAR_STYLE_TODAY_BOX
¶ Week highlight style
-
enumerator
Functions
-
lv_obj_t *
lv_calendar_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a calendar objects
- Return
pointer to the created calendar
- Parameters
par
: pointer to an object, it will be the parent of the new calendarcopy
: pointer to a calendar object, if not NULL then the new object will be copied from it
-
void
lv_calendar_set_today_date
(lv_obj_t *calendar, lv_calendar_date_t *today)¶ Set the today’s date
- Parameters
calendar
: pointer to a calendar objecttoday
: pointer to anlv_calendar_date_t
variable containing the date of today. The value will be saved it can be local variable too.
-
void
lv_calendar_set_showed_date
(lv_obj_t *calendar, lv_calendar_date_t *showed)¶ Set the currently showed
- Parameters
calendar
: pointer to a calendar objectshowed
: pointer to anlv_calendar_date_t
variable containing the date to show. The value will be saved it can be local variable too.
-
void
lv_calendar_set_highlighted_dates
(lv_obj_t *calendar, lv_calendar_date_t highlighted[], uint16_t date_num)¶ Set the the highlighted dates
- Parameters
calendar
: pointer to a calendar objecthighlighted
: pointer to anlv_calendar_date_t
array containing the dates. ONLY A POINTER WILL BE SAVED! CAN’T BE LOCAL ARRAY.date_num
: number of dates in the array
-
void
lv_calendar_set_day_names
(lv_obj_t *calendar, const char **day_names)¶ Set the name of the days
- Parameters
calendar
: pointer to a calendar objectday_names
: pointer to an array with the names. E.g.const char * days[7] = {"Sun", "Mon", ...}
Only the pointer will be saved so this variable can’t be local which will be destroyed later.
-
void
lv_calendar_set_month_names
(lv_obj_t *calendar, const char **month_names)¶ Set the name of the month
- Parameters
calendar
: pointer to a calendar objectmonth_names
: pointer to an array with the names. E.g.const char * days[12] = {"Jan", "Feb", ...}
Only the pointer will be saved so this variable can’t be local which will be destroyed later.
-
void
lv_calendar_set_style
(lv_obj_t *calendar, lv_calendar_style_t type, const lv_style_t *style)¶ Set a style of a calendar.
- Parameters
calendar
: pointer to calendar objecttype
: which style should be setstyle
: pointer to a style
-
lv_calendar_date_t *
lv_calendar_get_today_date
(const lv_obj_t *calendar)¶ Get the today’s date
- Return
return pointer to an
lv_calendar_date_t
variable containing the date of today.- Parameters
calendar
: pointer to a calendar object
-
lv_calendar_date_t *
lv_calendar_get_showed_date
(const lv_obj_t *calendar)¶ Get the currently showed
- Return
pointer to an
lv_calendar_date_t
variable containing the date is being shown.- Parameters
calendar
: pointer to a calendar object
-
lv_calendar_date_t *
lv_calendar_get_pressed_date
(const lv_obj_t *calendar)¶ Get the the pressed date.
- Return
pointer to an
lv_calendar_date_t
variable containing the pressed date.NULL
if not date pressed (e.g. the header)- Parameters
calendar
: pointer to a calendar object
-
lv_calendar_date_t *
lv_calendar_get_highlighted_dates
(const lv_obj_t *calendar)¶ Get the the highlighted dates
- Return
pointer to an
lv_calendar_date_t
array containing the dates.- Parameters
calendar
: pointer to a calendar object
-
uint16_t
lv_calendar_get_highlighted_dates_num
(const lv_obj_t *calendar)¶ Get the number of the highlighted dates
- Return
number of highlighted days
- Parameters
calendar
: pointer to a calendar object
-
const char **
lv_calendar_get_day_names
(const lv_obj_t *calendar)¶ Get the name of the days
- Return
pointer to the array of day names
- Parameters
calendar
: pointer to a calendar object
-
const char **
lv_calendar_get_month_names
(const lv_obj_t *calendar)¶ Get the name of the month
- Return
pointer to the array of month names
- Parameters
calendar
: pointer to a calendar object
-
const lv_style_t *
lv_calendar_get_style
(const lv_obj_t *calendar, lv_calendar_style_t type)¶ Get style of a calendar.
- Return
style pointer to the style
- Parameters
calendar
: pointer to calendar objecttype
: which style should be get
-
struct
lv_calendar_date_t
¶ - #include <lv_calendar.h>
Represents a date on the calendar object (platform-agnostic).
-
struct
lv_calendar_ext_t
¶ Public Members
-
lv_calendar_date_t
today
¶
-
lv_calendar_date_t
showed_date
¶
-
lv_calendar_date_t *
highlighted_dates
¶
-
int8_t
btn_pressing
¶
-
uint16_t
highlighted_dates_num
¶
-
lv_calendar_date_t
pressed_date
¶
-
const char **
day_names
¶
-
const char **
month_names
¶
-
const lv_style_t *
style_header
¶
-
const lv_style_t *
style_header_pr
¶
-
const lv_style_t *
style_day_names
¶
-
const lv_style_t *
style_highlighted_days
¶
-
const lv_style_t *
style_inactive_days
¶
-
const lv_style_t *
style_week_box
¶
-
const lv_style_t *
style_today_box
¶
-
lv_calendar_date_t