Table (lv_table)¶
Overview¶
Tables, as usual, are built from rows, columns, and cells containing texts.
The Table object is very light weighted because only the texts are stored. No real objects are created for cells but they are just drawn on the fly.
Rows and Columns¶
To set number of rows and columns use lv_table_set_row_cnt(table, row_cnt)
and lv_table_set_col_cnt(table, col_cnt)
Width and Height¶
The width of the columns can be set with lv_table_set_col_width(table, col_id, width)
. The overall width of the Table object will be set to the sum of columns widths.
The height is calculated automatically from the cell styles (font, padding etc) and the number of rows.
Set cell value¶
The cells can store on texts so need to convert numbers to text before displaying them in a table.
lv_table_set_cell_value(table, row, col, "Content")
. The text is saved by the table so it can be even a local variable.
Line break can be used in the text like "Value\n60.3"
.
Align¶
The text alignment in cells can be adjusted individually with lv_table_set_cell_align(table, row, col, LV_LABEL_ALIGN_LEFT/CENTER/RIGHT)
.
Cell type¶
You can use 4 different cell types. Each has its own style.
Cell types can be used to add different style for example to:
table header
first column
highlight a cell
etc
The type can be selected with lv_table_set_cell_type(table, row, col, type)
type
can be 1, 2, 3 or 4.
Merge cells¶
Cells can be merged horizontally with lv_table_set_cell_merge_right(table, col, row, true)
. To merge more adjacent cells apply this function for each cell.
Crop text¶
By default, the texts are word-wrapped to fit into the width of the cell and the height of the cell is set automatically. To disable this and keep the text as it is enable lv_table_set_cell_crop(table, row, col, true)
.
Styles¶
Use lv_table_set_style(page, LV_TABLE_STYLE_..., &style)
to set a new style for an element of the page:
LV_PAGE_STYLE_BG background’s style which uses all
style.body
properties (default:lv_style_plain_color
)LV_PAGE_STYLE_CELL1/2/3/4 4 for styles for the 4 cell types. All
style.body
properties are used. (default:lv_style_plain
)
Example¶
C¶
Simple table¶
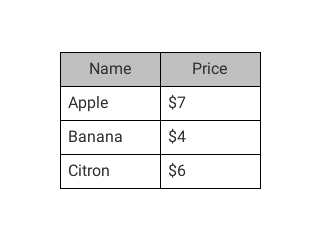
code
#include "lvgl/lvgl.h"
void lv_ex_table_1(void)
{
/*Create a normal cell style*/
static lv_style_t style_cell1;
lv_style_copy(&style_cell1, &lv_style_plain);
style_cell1.body.border.width = 1;
style_cell1.body.border.color = LV_COLOR_BLACK;
/*Crealte a header cell style*/
static lv_style_t style_cell2;
lv_style_copy(&style_cell2, &lv_style_plain);
style_cell2.body.border.width = 1;
style_cell2.body.border.color = LV_COLOR_BLACK;
style_cell2.body.main_color = LV_COLOR_SILVER;
style_cell2.body.grad_color = LV_COLOR_SILVER;
lv_obj_t * table = lv_table_create(lv_scr_act(), NULL);
lv_table_set_style(table, LV_TABLE_STYLE_CELL1, &style_cell1);
lv_table_set_style(table, LV_TABLE_STYLE_CELL2, &style_cell2);
lv_table_set_style(table, LV_TABLE_STYLE_BG, &lv_style_transp_tight);
lv_table_set_col_cnt(table, 2);
lv_table_set_row_cnt(table, 4);
lv_obj_align(table, NULL, LV_ALIGN_CENTER, 0, 0);
/*Make the cells of the first row center aligned */
lv_table_set_cell_align(table, 0, 0, LV_LABEL_ALIGN_CENTER);
lv_table_set_cell_align(table, 0, 1, LV_LABEL_ALIGN_CENTER);
/*Make the cells of the first row TYPE = 2 (use `style_cell2`) */
lv_table_set_cell_type(table, 0, 0, 2);
lv_table_set_cell_type(table, 0, 1, 2);
/*Fill the first column*/
lv_table_set_cell_value(table, 0, 0, "Name");
lv_table_set_cell_value(table, 1, 0, "Apple");
lv_table_set_cell_value(table, 2, 0, "Banana");
lv_table_set_cell_value(table, 3, 0, "Citron");
/*Fill the second column*/
lv_table_set_cell_value(table, 0, 1, "Price");
lv_table_set_cell_value(table, 1, 1, "$7");
lv_table_set_cell_value(table, 2, 1, "$4");
lv_table_set_cell_value(table, 3, 1, "$6");
}
MicroPython¶
Simple table¶
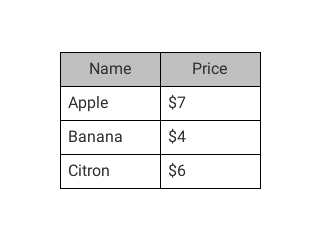
code
# Create a normal cell style
style_cell1 = lv.style_t()
lv.style_copy(style_cell1, lv.style_plain)
style_cell1.body.border.width = 1
style_cell1.body.border.color = lv.color_make(0,0,0)
# Crealte a header cell style
style_cell2 = lv.style_t()
lv.style_copy(style_cell2, lv.style_plain)
style_cell2.body.border.width = 1
style_cell2.body.border.color = lv.color_make(0,0,0)
style_cell2.body.main_color = lv.color_make(0xC0, 0xC0, 0xC0)
style_cell2.body.grad_color = lv.color_make(0xC0, 0xC0, 0xC0)
table = lv.table(lv.scr_act())
table.set_style(lv.table.STYLE.CELL1, style_cell1)
table.set_style(lv.table.STYLE.CELL2, style_cell2)
table.set_style(lv.table.STYLE.BG, lv.style_transp_tight)
table.set_col_cnt(2)
table.set_row_cnt(4)
table.align(None, lv.ALIGN.CENTER, 0, 0)
# Make the cells of the first row center aligned
table.set_cell_align(0, 0, lv.label.ALIGN.CENTER)
table.set_cell_align(0, 1, lv.label.ALIGN.CENTER)
# Make the cells of the first row TYPE = 2 (use `style_cell2`)
table.set_cell_type(0, 0, 2)
table.set_cell_type(0, 1, 2)
# Fill the first column
table.set_cell_value(0, 0, "Name")
table.set_cell_value(1, 0, "Apple")
table.set_cell_value(2, 0, "Banana")
table.set_cell_value(3, 0, "Citron")
# Fill the second column
table.set_cell_value(0, 1, "Price")
table.set_cell_value(1, 1, "$7")
table.set_cell_value(2, 1, "$4")
table.set_cell_value(3, 1, "$6")
MicroPython¶
No examples yet.
API¶
Typedefs
-
typedef uint8_t
lv_table_style_t
¶
Enums
Functions
-
lv_obj_t *
lv_table_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a table object
- Return
pointer to the created table
- Parameters
par
: pointer to an object, it will be the parent of the new tablecopy
: pointer to a table object, if not NULL then the new object will be copied from it
-
void
lv_table_set_cell_value
(lv_obj_t *table, uint16_t row, uint16_t col, const char *txt)¶ Set the value of a cell.
- Parameters
table
: pointer to a Table objectrow
: id of the row [0 .. row_cnt -1]col
: id of the column [0 .. col_cnt -1]txt
: text to display in the cell. It will be copied and saved so this variable is not required after this function call.
-
void
lv_table_set_row_cnt
(lv_obj_t *table, uint16_t row_cnt)¶ Set the number of rows
- Parameters
table
: table pointer to a Table objectrow_cnt
: number of rows
-
void
lv_table_set_col_cnt
(lv_obj_t *table, uint16_t col_cnt)¶ Set the number of columns
- Parameters
table
: table pointer to a Table objectcol_cnt
: number of columns. Must be < LV_TABLE_COL_MAX
-
void
lv_table_set_col_width
(lv_obj_t *table, uint16_t col_id, lv_coord_t w)¶ Set the width of a column
- Parameters
table
: table pointer to a Table objectcol_id
: id of the column [0 .. LV_TABLE_COL_MAX -1]w
: width of the column
-
void
lv_table_set_cell_align
(lv_obj_t *table, uint16_t row, uint16_t col, lv_label_align_t align)¶ Set the text align in a cell
- Parameters
table
: pointer to a Table objectrow
: id of the row [0 .. row_cnt -1]col
: id of the column [0 .. col_cnt -1]align
: LV_LABEL_ALIGN_LEFT or LV_LABEL_ALIGN_CENTER or LV_LABEL_ALIGN_RIGHT
-
void
lv_table_set_cell_type
(lv_obj_t *table, uint16_t row, uint16_t col, uint8_t type)¶ Set the type of a cell.
- Parameters
table
: pointer to a Table objectrow
: id of the row [0 .. row_cnt -1]col
: id of the column [0 .. col_cnt -1]type
: 1,2,3 or 4. The cell style will be chosen accordingly.
-
void
lv_table_set_cell_crop
(lv_obj_t *table, uint16_t row, uint16_t col, bool crop)¶ Set the cell crop. (Don’t adjust the height of the cell according to its content)
- Parameters
table
: pointer to a Table objectrow
: id of the row [0 .. row_cnt -1]col
: id of the column [0 .. col_cnt -1]crop
: true: crop the cell content; false: set the cell height to the content.
-
void
lv_table_set_cell_merge_right
(lv_obj_t *table, uint16_t row, uint16_t col, bool en)¶ Merge a cell with the right neighbor. The value of the cell to the right won’t be displayed.
- Parameters
table
: table pointer to a Table objectrow
: id of the row [0 .. row_cnt -1]col
: id of the column [0 .. col_cnt -1]en
: true: merge right; false: don’t merge right
-
void
lv_table_set_style
(lv_obj_t *table, lv_table_style_t type, const lv_style_t *style)¶ Set a style of a table.
- Parameters
table
: pointer to table objecttype
: which style should be setstyle
: pointer to a style
-
const char *
lv_table_get_cell_value
(lv_obj_t *table, uint16_t row, uint16_t col)¶ Get the value of a cell.
- Return
text in the cell
- Parameters
table
: pointer to a Table objectrow
: id of the row [0 .. row_cnt -1]col
: id of the column [0 .. col_cnt -1]
-
uint16_t
lv_table_get_row_cnt
(lv_obj_t *table)¶ Get the number of rows.
- Return
number of rows.
- Parameters
table
: table pointer to a Table object
-
uint16_t
lv_table_get_col_cnt
(lv_obj_t *table)¶ Get the number of columns.
- Return
number of columns.
- Parameters
table
: table pointer to a Table object
-
lv_coord_t
lv_table_get_col_width
(lv_obj_t *table, uint16_t col_id)¶ Get the width of a column
- Return
width of the column
- Parameters
table
: table pointer to a Table objectcol_id
: id of the column [0 .. LV_TABLE_COL_MAX -1]
-
lv_label_align_t
lv_table_get_cell_align
(lv_obj_t *table, uint16_t row, uint16_t col)¶ Get the text align of a cell
- Return
LV_LABEL_ALIGN_LEFT (default in case of error) or LV_LABEL_ALIGN_CENTER or LV_LABEL_ALIGN_RIGHT
- Parameters
table
: pointer to a Table objectrow
: id of the row [0 .. row_cnt -1]col
: id of the column [0 .. col_cnt -1]
-
lv_label_align_t
lv_table_get_cell_type
(lv_obj_t *table, uint16_t row, uint16_t col)¶ Get the type of a cell
- Return
1,2,3 or 4
- Parameters
table
: pointer to a Table objectrow
: id of the row [0 .. row_cnt -1]col
: id of the column [0 .. col_cnt -1]
-
lv_label_align_t
lv_table_get_cell_crop
(lv_obj_t *table, uint16_t row, uint16_t col)¶ Get the crop property of a cell
- Return
true: text crop enabled; false: disabled
- Parameters
table
: pointer to a Table objectrow
: id of the row [0 .. row_cnt -1]col
: id of the column [0 .. col_cnt -1]
-
bool
lv_table_get_cell_merge_right
(lv_obj_t *table, uint16_t row, uint16_t col)¶ Get the cell merge attribute.
- Return
true: merge right; false: don’t merge right
- Parameters
table
: table pointer to a Table objectrow
: id of the row [0 .. row_cnt -1]col
: id of the column [0 .. col_cnt -1]
-
const lv_style_t *
lv_table_get_style
(const lv_obj_t *table, lv_table_style_t type)¶ Get style of a table.
- Return
style pointer to the style
- Parameters
table
: pointer to table objecttype
: which style should be get
-
union
lv_table_cell_format_t
¶ - #include <lv_table.h>
Internal table cell format structure.
Use the
lv_table
APIs instead.
-
struct
lv_table_ext_t
¶