Arc (lv_arc)¶
Overview¶
The Arc are consists of a background and a foreground arc. Both can have start and end angles and thickness.
Parts and Styles¶
The Arc's main part is called LV_ARC_PART_MAIN
. It draws a background using the typical background style properties and an arc using the line style properties.
The arc's size and position will respect the padding style properties.
LV_ARC_PART_INDIC
is virtual part and it draws an other arc using the line style properties. It's padding values are interpreted relative to the background arc.
The radius of the indicator arc will be modified according to the greatest padding value.
LV_ARC_PART_KNOB
is virtual part and it draws on the end of the arc indicator. It uses all background properties and padding values. With zero padding the knob size is the same as the indicator's width.
Larger padding makes it larger, smaller padding makes it smaller.
Usage¶
Angles¶
To set the angles of the background, use the lv_arc_set_bg_angles(arc, start_angle, end_angle)
function or lv_arc_set_bg_start/end_angle(arc, start_angle)
.
Zero degree is at the middle right (3 o'clock) of the object and the degrees are increasing in a clockwise direction.
The angles should be in [0;360] range.
Similarly, lv_arc_set_angles(arc, start_angle, end_angle)
function or lv_arc_set_start/end_angle(arc, start_angle)
sets the angles of the indicator arc.
Rotation¶
An offset to the 0 degree position can added with lv_arc_set_rotation(arc, deg)
.
Range and values¶
Besides setting angles manually the arc can have a range and a value. To set the range use lv_arc_set_range(arc, min, max)
and to set a value use lv_arc_set_value(arc, value)
.
Using range and value the angle of the indicator will be mapped between background angles.
Note that, settings angles and values are independent. You should use either value and angle settings. Mixing the two might result unintended behavior.
Type¶
The arc can have the different "types". They are set with lv_arc_set_type
.
The following types exist:
LV_ARC_TYPE_NORMAL
indicator arc drawn clockwise (min to current)LV_ARC_TYPE_REVERSE
indicator arc drawn counter clockwise (max to current)LV_ARC_TYPE_SYMMETRIC
indicator arc drawn from the middle point to the current value.
Events¶
Besides the Generic events the following Special events are sent by the arcs:
LV_EVENT_VALUE_CHANGED sent when the arc is pressed/dragged to set a new value.
Learn more about Events.
Example¶
C¶
Simple Arc¶
code
#include "../../../lv_examples.h"
#if LV_USE_ARC
void lv_ex_arc_1(void)
{
/*Create an Arc*/
lv_obj_t * arc = lv_arc_create(lv_scr_act(), NULL);
lv_arc_set_end_angle(arc, 200);
lv_obj_set_size(arc, 150, 150);
lv_obj_align(arc, NULL, LV_ALIGN_CENTER, 0, 0);
}
#endif
Loader with Arc¶
code
#include "../../../lv_examples.h"
#if LV_USE_ARC
/**
* An `lv_task` to call periodically to set the angles of the arc
* @param t
*/
static void arc_loader(lv_task_t * t)
{
static int16_t a = 270;
a+=5;
lv_arc_set_end_angle(t->user_data, a);
if(a >= 270 + 360) {
lv_task_del(t);
return;
}
}
/**
* Create an arc which acts as a loader.
*/
void lv_ex_arc_2(void)
{
/*Create an Arc*/
lv_obj_t * arc = lv_arc_create(lv_scr_act(), NULL);
lv_arc_set_bg_angles(arc, 0, 360);
lv_arc_set_angles(arc, 270, 270);
lv_obj_align(arc, NULL, LV_ALIGN_CENTER, 0, 0);
/* Create an `lv_task` to update the arc.
* Store the `arc` in the user data*/
lv_task_create(arc_loader, 20, LV_TASK_PRIO_LOWEST, arc);
}
#endif
MicroPython¶
Simple Arc¶
Click to try in the simulator!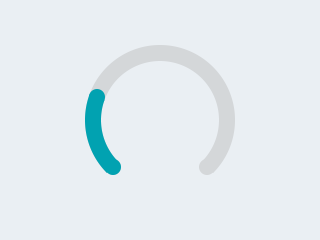
code
# create an arc
arc = lv.arc(lv.scr_act(),None)
arc.set_end_angle(200)
arc.set_size(150,150)
arc.align(None,lv.ALIGN.CENTER,0,0)
Loader with Arc¶
Click to try in the simulator!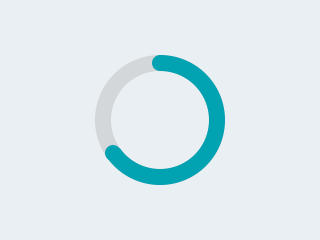
code
def arc_loader(task,arc):
angle = arc.get_value()
# print("angle: ",angle)
angle += 5
if angle > 360:
task._del()
else:
arc.set_value(angle)
# create an arc which acts as a loader
arc = lv.arc(lv.scr_act(),None)
arc.set_range(0,360)
arc.set_bg_angles(0,360)
arc.set_angles(0,360)
arc.set_rotation(270)
arc.align(None,lv.ALIGN.CENTER,0,0)
arc.set_value(10)
# Create an `lv_task` to update the arc.
task = lv.task_create_basic()
task.set_cb(lambda task: arc_loader(task, arc))
task.set_period(20)
task.set_prio(lv.TASK_PRIO.LOWEST)
API¶
Enums
Functions
-
lv_obj_t *
lv_arc_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a arc objects
- Parameters
par -- pointer to an object, it will be the parent of the new arc
copy -- pointer to a arc object, if not NULL then the new object will be copied from it
- Returns
pointer to the created arc
-
void
lv_arc_set_start_angle
(lv_obj_t *arc, uint16_t start)¶ Set the start angle of an arc. 0 deg: right, 90 bottom, etc.
- Parameters
arc -- pointer to an arc object
start -- the start angle
-
void
lv_arc_set_end_angle
(lv_obj_t *arc, uint16_t end)¶ Set the start angle of an arc. 0 deg: right, 90 bottom, etc.
- Parameters
arc -- pointer to an arc object
end -- the end angle
-
void
lv_arc_set_angles
(lv_obj_t *arc, uint16_t start, uint16_t end)¶ Set the start and end angles
- Parameters
arc -- pointer to an arc object
start -- the start angle
end -- the end angle
-
void
lv_arc_set_bg_start_angle
(lv_obj_t *arc, uint16_t start)¶ Set the start angle of an arc background. 0 deg: right, 90 bottom, etc.
- Parameters
arc -- pointer to an arc object
start -- the start angle
-
void
lv_arc_set_bg_end_angle
(lv_obj_t *arc, uint16_t end)¶ Set the start angle of an arc background. 0 deg: right, 90 bottom etc.
- Parameters
arc -- pointer to an arc object
end -- the end angle
-
void
lv_arc_set_bg_angles
(lv_obj_t *arc, uint16_t start, uint16_t end)¶ Set the start and end angles of the arc background
- Parameters
arc -- pointer to an arc object
start -- the start angle
end -- the end angle
-
void
lv_arc_set_rotation
(lv_obj_t *arc, uint16_t rotation_angle)¶ Set the rotation for the whole arc
- Parameters
arc -- pointer to an arc object
rotation_angle -- rotation angle
-
void
lv_arc_set_type
(lv_obj_t *arc, lv_arc_type_t type)¶ Set the type of arc.
- Parameters
arc -- pointer to arc object
type -- arc type
-
void
lv_arc_set_value
(lv_obj_t *arc, int16_t value)¶ Set a new value on the arc
- Parameters
arc -- pointer to a arc object
value -- new value
-
void
lv_arc_set_range
(lv_obj_t *arc, int16_t min, int16_t max)¶ Set minimum and the maximum values of a arc
- Parameters
arc -- pointer to the arc object
min -- minimum value
max -- maximum value
-
void
lv_arc_set_chg_rate
(lv_obj_t *arc, uint16_t threshold)¶ Set the threshold of arc knob increments position.
- Parameters
arc -- pointer to a arc object
threshold -- increment threshold
-
void
lv_arc_set_adjustable
(lv_obj_t *arc, bool adjustable)¶ Set whether the arc is adjustable.
- Parameters
arc -- pointer to a arc object
adjustable -- whether the arc has a knob that can be dragged
-
uint16_t
lv_arc_get_angle_start
(lv_obj_t *arc)¶ Get the start angle of an arc.
- Parameters
arc -- pointer to an arc object
- Returns
the start angle [0..360]
-
uint16_t
lv_arc_get_angle_end
(lv_obj_t *arc)¶ Get the end angle of an arc.
- Parameters
arc -- pointer to an arc object
- Returns
the end angle [0..360]
-
uint16_t
lv_arc_get_bg_angle_start
(lv_obj_t *arc)¶ Get the start angle of an arc background.
- Parameters
arc -- pointer to an arc object
- Returns
the start angle [0..360]
-
uint16_t
lv_arc_get_bg_angle_end
(lv_obj_t *arc)¶ Get the end angle of an arc background.
- Parameters
arc -- pointer to an arc object
- Returns
the end angle [0..360]
-
lv_arc_type_t
lv_arc_get_type
(const lv_obj_t *arc)¶ Get whether the arc is type or not.
- Parameters
arc -- pointer to a arc object
- Returns
arc type
-
int16_t
lv_arc_get_value
(const lv_obj_t *arc)¶ Get the value of the of a arc
- Parameters
arc -- pointer to a arc object
- Returns
the value of the of the arc
-
int16_t
lv_arc_get_min_value
(const lv_obj_t *arc)¶ Get the minimum value of a arc
- Parameters
arc -- pointer to a arc object
- Returns
the minimum value of the arc
-
int16_t
lv_arc_get_max_value
(const lv_obj_t *arc)¶ Get the maximum value of a arc
- Parameters
arc -- pointer to a arc object
- Returns
the maximum value of the arc
-
struct
lv_arc_ext_t
¶ Public Members
-
uint16_t
rotation_angle
¶
-
uint16_t
arc_angle_start
¶
-
uint16_t
arc_angle_end
¶
-
uint16_t
bg_angle_start
¶
-
uint16_t
bg_angle_end
¶
-
lv_style_list_t
style_arc
¶
-
lv_style_list_t
style_knob
¶
-
int16_t
cur_value
¶
-
int16_t
min_value
¶
-
int16_t
max_value
¶
-
uint16_t
dragging
¶
-
uint16_t
type
¶
-
uint16_t
adjustable
¶
-
uint16_t
min_close
¶
-
uint16_t
chg_rate
¶
-
uint32_t
last_tick
¶
-
int16_t
last_angle
¶
-
uint16_t