Spinner (lv_spinner)¶
Overview¶
The Spinner object is a spinning arc over a border.
Parts and Styles¶
The Spinner uses the the following parts:
LV_SPINNER_PART_BG
: main partLV_SPINNER_PART_INDIC
: the spinning arc (virtual part)
The parts and style works the same as in case of Arc. Read its documentation for a details description.
Usage¶
Arc length¶
The length of the arc can be adjusted by lv_spinner_set_arc_length(spinner, deg)
.
Spinning speed¶
The speed of the spinning can be adjusted by lv_spinner_set_spin_time(preload, time_ms)
.
Spin types¶
You can choose from more spin types:
LV_SPINNER_TYPE_SPINNING_ARC spin the arc, slow down on the top
LV_SPINNER_TYPE_FILLSPIN_ARC spin the arc, slow down on the top but also stretch the arc
LV_SPINNER_TYPE_CONSTANT_ARC spin the arc at a constant speed
To apply one if them use lv_spinner_set_type(preload, LV_SPINNER_TYPE_...)
Spin direction¶
The direction of spinning can be changed with lv_spinner_set_dir(preload, LV_SPINNER_DIR_FORWARD/BACKWARD)
.
Events¶
Only the Generic events are sent by the object type.
Example¶
C¶
Simple spinner¶
code
#include "../../../lv_examples.h"
#if LV_USE_SPINNER
void lv_ex_spinner_1(void)
{
/*Create a Preloader object*/
lv_obj_t * preload = lv_spinner_create(lv_scr_act(), NULL);
lv_obj_set_size(preload, 100, 100);
lv_obj_align(preload, NULL, LV_ALIGN_CENTER, 0, 0);
}
#endif
MicroPython¶
Simple spinner¶
Click to try in the simulator!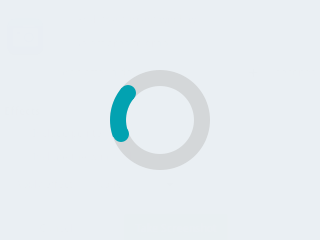
code
# create a Preloader object
preload = lv.spinner(lv.scr_act(), None)
preload.set_size(100, 100)
preload.align(None, lv.ALIGN.CENTER, 0, 0)
MicroPython¶
No examples yet.
API¶
Typedefs
-
typedef uint8_t
lv_spinner_type_t
¶
-
typedef uint8_t
lv_spinner_dir_t
¶
-
typedef uint8_t
lv_spinner_style_t
¶
Enums
-
enum [anonymous]¶
Type of spinner.
Values:
-
enumerator
LV_SPINNER_TYPE_SPINNING_ARC
¶
-
enumerator
LV_SPINNER_TYPE_FILLSPIN_ARC
¶
-
enumerator
LV_SPINNER_TYPE_CONSTANT_ARC
¶
-
enumerator
Functions
-
lv_obj_t *
lv_spinner_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a spinner object
- Parameters
par -- pointer to an object, it will be the parent of the new spinner
copy -- pointer to a spinner object, if not NULL then the new object will be copied from it
- Returns
pointer to the created spinner
-
void
lv_spinner_set_arc_length
(lv_obj_t *spinner, lv_anim_value_t deg)¶ Set the length of the spinning arc in degrees
- Parameters
spinner -- pointer to a spinner object
deg -- length of the arc
-
void
lv_spinner_set_spin_time
(lv_obj_t *spinner, uint16_t time)¶ Set the spin time of the arc
- Parameters
spinner -- pointer to a spinner object
time -- time of one round in milliseconds
-
void
lv_spinner_set_type
(lv_obj_t *spinner, lv_spinner_type_t type)¶ Set the animation type of a spinner.
- Parameters
spinner -- pointer to spinner object
type -- animation type of the spinner
-
void
lv_spinner_set_dir
(lv_obj_t *spinner, lv_spinner_dir_t dir)¶ Set the animation direction of a spinner
- Parameters
spinner -- pointer to spinner object
direction -- animation direction of the spinner
-
lv_anim_value_t
lv_spinner_get_arc_length
(const lv_obj_t *spinner)¶ Get the arc length [degree] of the a spinner
- Parameters
spinner -- pointer to a spinner object
-
uint16_t
lv_spinner_get_spin_time
(const lv_obj_t *spinner)¶ Get the spin time of the arc
- Parameters
spinner -- pointer to a spinner object [milliseconds]
-
lv_spinner_type_t
lv_spinner_get_type
(lv_obj_t *spinner)¶ Get the animation type of a spinner.
- Parameters
spinner -- pointer to spinner object
- Returns
animation type
-
lv_spinner_dir_t
lv_spinner_get_dir
(lv_obj_t *spinner)¶ Get the animation direction of a spinner
- Parameters
spinner -- pointer to spinner object
- Returns
animation direction
-
void
lv_spinner_anim_cb
(void *ptr, lv_anim_value_t val)¶ Animator function (exec_cb) to rotate the arc of spinner.
- Parameters
ptr -- pointer to spinner
val -- the current desired value [0..360]
-
struct
lv_spinner_ext_t
¶ Public Members
-
lv_arc_ext_t
arc
¶
-
lv_anim_value_t
arc_length
¶
-
uint16_t
time
¶
-
lv_spinner_type_t
anim_type
¶
-
lv_spinner_dir_t
anim_dir
¶
-
lv_arc_ext_t