Image button (lv_imgbtn)¶
Overview¶
The Image button is very similar to the simple 'Button' object. The only difference is that, it displays user-defined images in each state instead of drawing a rectangle. Before reading this section, please read the Button section for better understanding.
Parts and Styles¶
The Image button object has only a main part called LV_IMG_BTN_PART_MAIN
from where all image style properties are used.
It's possible to recolor the image in each state with image_recolor and image_recolor_opa proeprties. For example, to make the image darker if it is pressed.
Usage¶
Image sources¶
To set the image in a state, use the lv_imgbtn_set_src(imgbtn, LV_BTN_STATE_..., &img_src)
.
The image sources works the same as described in the Image object except that, "Symbols" are not supported by the Image button.
If LV_IMGBTN_TILED
is enabled in lv_conf.h, then lv_imgbtn_set_src_tiled(imgbtn, LV_BTN_STATE_..., &img_src_left, &img_src_mid, &img_src_right)
becomes available.
Using the tiled feature the middle image will be repeated to fill the width of the object.
Therefore with LV_IMGBTN_TILED
, you can set the width of the Image button using lv_obj_set_width()
. However, without this option, the width will be always the same as the image source's width.
Button features¶
Similarly to normal Buttons lv_imgbtn_set_checkable(imgbtn, true/false)
, lv_imgbtn_toggle(imgbtn)
and lv_imgbtn_set_state(imgbtn, LV_BTN_STATE_...)
also works.
Events¶
Beside the Generic events, the following Special events are sent by the buttons:
LV_EVENT_VALUE_CHANGED - Sent when the button is toggled.
Note that, the generic input device related events (like LV_EVENT_PRESSED
) are sent in the inactive state too. You need to check the state with lv_btn_get_state(btn)
to ignore the events from inactive buttons.
Learn more about Events.
Keys¶
The following Keys are processed by the Buttons:
LV_KEY_RIGHT/UP - Go to toggled state if toggling is enabled.
LV_KEY_LEFT/DOWN - Go to non-toggled state if toggling is enabled.
Note that, as usual, the state of LV_KEY_ENTER
is translated to LV_EVENT_PRESSED/PRESSING/RELEASED
etc.
Learn more about Keys.
Example¶
C¶
Simple Image button¶
code
#include "../../../lv_examples.h"
#if LV_USE_IMGBTN
void lv_ex_imgbtn_1(void)
{
LV_IMG_DECLARE(imgbtn_green);
LV_IMG_DECLARE(imgbtn_blue);
/*Darken the button when pressed*/
static lv_style_t style;
lv_style_init(&style);
lv_style_set_image_recolor_opa(&style, LV_STATE_PRESSED, LV_OPA_30);
lv_style_set_image_recolor(&style, LV_STATE_PRESSED, LV_COLOR_BLACK);
lv_style_set_text_color(&style, LV_STATE_DEFAULT, LV_COLOR_WHITE);
/*Create an Image button*/
lv_obj_t * imgbtn1 = lv_imgbtn_create(lv_scr_act(), NULL);
lv_imgbtn_set_src(imgbtn1, LV_BTN_STATE_RELEASED, &imgbtn_green);
lv_imgbtn_set_src(imgbtn1, LV_BTN_STATE_PRESSED, &imgbtn_green);
lv_imgbtn_set_src(imgbtn1, LV_BTN_STATE_CHECKED_RELEASED, &imgbtn_blue);
lv_imgbtn_set_src(imgbtn1, LV_BTN_STATE_CHECKED_PRESSED, &imgbtn_blue);
lv_imgbtn_set_checkable(imgbtn1, true);
lv_obj_add_style(imgbtn1, LV_IMGBTN_PART_MAIN, &style);
lv_obj_align(imgbtn1, NULL, LV_ALIGN_CENTER, 0, -40);
/*Create a label on the Image button*/
lv_obj_t * label = lv_label_create(imgbtn1, NULL);
lv_label_set_text(label, "Button");
}
#endif
MicroPython¶
Simple Image button¶
Click to try in the simulator!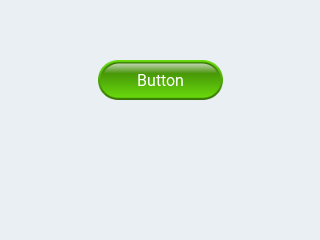
code
import usys as sys
from lv_colors import lv_colors
# create the green image data
try:
with open('../../../assets/imgbtn_green_argb.bin','rb') as f:
green_img_data = f.read()
except:
try:
with open('images/imgbtn_green_rgb565.bin','rb') as f:
green_img_data = f.read()
except:
print("Cannot open green buttom image file")
sys.exit()
# create the blue image data
try:
with open('../../../assets/imgbtn_blue_argb.bin','rb') as f:
blue_img_data = f.read()
except:
try:
with open('images/imgbtn_blue_rgb565.bin','rb') as f:
blue_img_data = f.read()
except:
print("Cannot open green buttom image file")
sys.exit()
imgbtn_green_dsc = lv.img_dsc_t(
{
"header": {"always_zero": 0, "w": 125, "h": 40, "cf": lv.img.CF.TRUE_COLOR_ALPHA},
"data": green_img_data,
"data_size": len(green_img_data),
}
)
# create the blue image data
imgbtn_blue_dsc = lv.img_dsc_t(
{
"header": {"always_zero": 0, "w": 125, "h": 40, "cf": lv.img.CF.TRUE_COLOR_ALPHA},
"data": blue_img_data,
"data_size": len(blue_img_data),
}
)
# Darken the button when pressed
style=lv.style_t()
style.init()
style.set_image_recolor_opa(lv.STATE.PRESSED, lv.OPA._30)
style.set_image_recolor(lv.STATE.PRESSED, lv_colors.BLACK)
style.set_text_color(lv.STATE.DEFAULT, lv_colors.WHITE)
# Create an Image button
imgbtn1 = lv.imgbtn(lv.scr_act(), None)
imgbtn1.set_src(lv.btn.STATE.RELEASED, imgbtn_green_dsc)
imgbtn1.set_src(lv.btn.STATE.PRESSED, imgbtn_green_dsc)
imgbtn1.set_src(lv.btn.STATE.CHECKED_RELEASED, imgbtn_blue_dsc)
imgbtn1.set_src(lv.btn.STATE.CHECKED_PRESSED, imgbtn_blue_dsc)
imgbtn1.set_checkable(True)
imgbtn1.add_style(lv.imgbtn.PART.MAIN, style)
imgbtn1.align(None, lv.ALIGN.CENTER, 0, -40)
# Create a label on the Image button
label = lv.label(imgbtn1, None)
label.set_text("Button")
API¶
Typedefs
-
typedef uint8_t
lv_imgbtn_part_t
¶
Functions
-
lv_obj_t *
lv_imgbtn_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a image button objects
- Parameters
par -- pointer to an object, it will be the parent of the new image button
copy -- pointer to a image button object, if not NULL then the new object will be copied from it
- Returns
pointer to the created image button
-
void
lv_imgbtn_set_src
(lv_obj_t *imgbtn, lv_btn_state_t state, const void *src)¶ Set images for a state of the image button
- Parameters
imgbtn -- pointer to an image button object
state -- for which state set the new image (from
lv_btn_state_t
) `src -- pointer to an image source (a C array or path to a file)
-
void
lv_imgbtn_set_src_tiled
(lv_obj_t *imgbtn, lv_btn_state_t state, const void *src_left, const void *src_mid, const void *src_right)¶ Set images for a state of the image button
- Parameters
imgbtn -- pointer to an image button object
state -- for which state set the new image (from
lv_btn_state_t
) `src_left -- pointer to an image source for the left side of the button (a C array or path to a file)
src_mid -- pointer to an image source for the middle of the button (ideally 1px wide) (a C array or path to a file)
src_right -- pointer to an image source for the right side of the button (a C array or path to a file)
-
void
lv_imgbtn_set_state
(lv_obj_t *imgbtn, lv_btn_state_t state)¶ Set the state of the image button
- Parameters
imgbtn -- pointer to an image button object
state -- the new state of the button (from lv_btn_state_t enum)
-
void
lv_imgbtn_toggle
(lv_obj_t *imgbtn)¶ Toggle the state of the image button (ON->OFF, OFF->ON)
- Parameters
imgbtn -- pointer to a image button object
-
static inline void
lv_imgbtn_set_checkable
(lv_obj_t *imgbtn, bool tgl)¶ Enable the toggled states. On release the button will change from/to toggled state.
- Parameters
imgbtn -- pointer to an image button object
tgl -- true: enable toggled states, false: disable
-
const void *
lv_imgbtn_get_src
(lv_obj_t *imgbtn, lv_btn_state_t state)¶ Get the images in a given state
- Parameters
imgbtn -- pointer to an image button object
state -- the state where to get the image (from
lv_btn_state_t
) `
- Returns
pointer to an image source (a C array or path to a file)
-
const void *
lv_imgbtn_get_src_left
(lv_obj_t *imgbtn, lv_btn_state_t state)¶ Get the left image in a given state
- Parameters
imgbtn -- pointer to an image button object
state -- the state where to get the image (from
lv_btn_state_t
) `
- Returns
pointer to the left image source (a C array or path to a file)
-
const void *
lv_imgbtn_get_src_middle
(lv_obj_t *imgbtn, lv_btn_state_t state)¶ Get the middle image in a given state
- Parameters
imgbtn -- pointer to an image button object
state -- the state where to get the image (from
lv_btn_state_t
) `
- Returns
pointer to the middle image source (a C array or path to a file)
-
const void *
lv_imgbtn_get_src_right
(lv_obj_t *imgbtn, lv_btn_state_t state)¶ Get the right image in a given state
- Parameters
imgbtn -- pointer to an image button object
state -- the state where to get the image (from
lv_btn_state_t
) `
- Returns
pointer to the left image source (a C array or path to a file)
-
static inline lv_btn_state_t
lv_imgbtn_get_state
(const lv_obj_t *imgbtn)¶ Get the current state of the image button
- Parameters
imgbtn -- pointer to a image button object
- Returns
the state of the button (from lv_btn_state_t enum)
-
struct
lv_imgbtn_ext_t
¶ Public Members
-
lv_btn_ext_t
btn
¶
-
const void *
img_src_mid
[_LV_BTN_STATE_LAST
]¶
-
const void *
img_src_left
[_LV_BTN_STATE_LAST
]¶
-
const void *
img_src_right
[_LV_BTN_STATE_LAST
]¶
-
lv_img_cf_t
act_cf
¶
-
uint8_t
tiled
¶
-
lv_btn_ext_t