Table (lv_table)¶
Overview¶
Tables, as usual, are built from rows, columns, and cells containing texts.
The Table object is very light weighted because only the texts are stored. No real objects are created for cells but they are just drawn on the fly.
Parts and Styles¶
The main part of the Table is called LV_TABLE_PART_BG
. It's a rectangle like background and uses all the typical background style properties.
For the cells there are 4 virtual parts. Every cell has type (1, 2, ... 16) which tells which part's styles to apply on them. The cell parts can be referenced by LV_TABLE_PART_CELL1 + x
where x
is between 0..15
.
The number of cell types can be adjusted in lv_conf.h
by LV_TABLE_CELL_STYLE_CNT
. By default it's 4. The default 4 cell types' part be referenced with dedicated names too:
LV_TABLE_PART_CELL1
LV_TABLE_PART_CELL2
LV_TABLE_PART_CELL3
LV_TABLE_PART_CELL4
The cells also use all the typical background style properties. If there is a line break (\n
) in a cell's content then a horizontal division line will drawn after the line break using the line style properties.
The style of texts in the cells are inherited from the cell parts or the background part.
Usage¶
Rows and Columns¶
To set number of rows and columns use lv_table_set_row_cnt(table, row_cnt)
and lv_table_set_col_cnt(table, col_cnt)
Width and Height¶
The width of the columns can be set with lv_table_set_col_width(table, col_id, width)
. The overall width of the Table object will be set to the sum of columns widths.
The height is calculated automatically from the cell styles (font, padding etc) and the number of rows.
Set cell value¶
The cells can store only texts so numbers needs to be converted to text before displaying them in a table.
lv_table_set_cell_value(table, row, col, "Content")
. The text is saved by the table so it can be even a local variable.
Line break can be used in the text like "Value\n60.3"
.
Align¶
The text alignment in cells can be adjusted individually with lv_table_set_cell_align(table, row, col, LV_LABEL_ALIGN_LEFT/CENTER/RIGHT)
.
Cell type¶
You can use 4 different cell types. Each has its own style.
Cell types can be used to add different style for example to:
table header
first column
highlight a cell
etc
The type can be selected with lv_table_set_cell_type(table, row, col, type)
type
can be 1, 2, 3 or 4.
Merge cells¶
Cells can be merged horizontally with lv_table_set_cell_merge_right(table, col, row, true)
. To merge more adjacent cells apply this function for each cell.
Crop text¶
By default, the texts are word-wrapped to fit into the width of the cell and the height of the cell is set automatically.
To disable this and keep the text as it is enable lv_table_set_cell_crop(table, row, col, true)
.
Example¶
C¶
Simple table¶
code
#include "../../../lv_examples.h"
#if LV_USE_TABLE
void lv_ex_table_1(void)
{
lv_obj_t * table = lv_table_create(lv_scr_act(), NULL);
lv_table_set_col_cnt(table, 2);
lv_table_set_row_cnt(table, 4);
lv_obj_align(table, NULL, LV_ALIGN_CENTER, 0, 0);
/*Make the cells of the first row center aligned */
lv_table_set_cell_align(table, 0, 0, LV_LABEL_ALIGN_CENTER);
lv_table_set_cell_align(table, 0, 1, LV_LABEL_ALIGN_CENTER);
/*Align the price values to the right in the 2nd column*/
lv_table_set_cell_align(table, 1, 1, LV_LABEL_ALIGN_RIGHT);
lv_table_set_cell_align(table, 2, 1, LV_LABEL_ALIGN_RIGHT);
lv_table_set_cell_align(table, 3, 1, LV_LABEL_ALIGN_RIGHT);
lv_table_set_cell_type(table, 0, 0, 2);
lv_table_set_cell_type(table, 0, 1, 2);
/*Fill the first column*/
lv_table_set_cell_value(table, 0, 0, "Name");
lv_table_set_cell_value(table, 1, 0, "Apple");
lv_table_set_cell_value(table, 2, 0, "Banana");
lv_table_set_cell_value(table, 3, 0, "Citron");
/*Fill the second column*/
lv_table_set_cell_value(table, 0, 1, "Price");
lv_table_set_cell_value(table, 1, 1, "$7");
lv_table_set_cell_value(table, 2, 1, "$4");
lv_table_set_cell_value(table, 3, 1, "$6");
lv_table_ext_t * ext = lv_obj_get_ext_attr(table);
ext->row_h[0] = 20;
}
#endif
MicroPython¶
Simple table¶
Click to try in the simulator!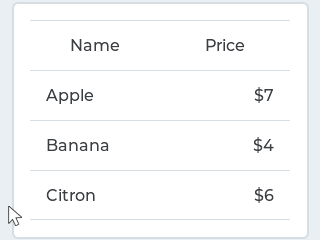
code
table = lv.table(lv.scr_act(), None)
table.set_col_cnt(2)
table.set_row_cnt(4)
table.set_size(200,200)
table.align(lv.scr_act(), lv.ALIGN.CENTER, -15, 0)
# Make the cells of the first row center aligned
table.set_col_width(0,100)
table.set_cell_align(0, 0, lv.label.ALIGN.CENTER)
table.set_cell_align(0, 1, lv.label.ALIGN.CENTER)
# Align the price values to the right in the 2nd column
table.set_col_width(1,100)
table.set_cell_align(1, 1, lv.label.ALIGN.RIGHT)
table.set_cell_align(2, 1, lv.label.ALIGN.RIGHT)
table.set_cell_align(3, 1, lv.label.ALIGN.RIGHT)
table.set_cell_type(0, 0, 2)
table.set_cell_type(0, 1, 2)
# Fill the first column
table.set_cell_value(0, 0, "Name")
table.set_cell_value(1, 0, "Apple")
table.set_cell_value(2, 0, "Banana")
table.set_cell_value(3, 0, "Citron")
# Fill the second column
table.set_cell_value(0, 1, "Price")
table.set_cell_value(1, 1, "$7")
table.set_cell_value(2, 1, "$4")
table.set_cell_value(3, 1, "$6")
MicroPython¶
No examples yet.
API¶
Enums
Functions
-
lv_obj_t *
lv_table_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a table object
- Parameters
par -- pointer to an object, it will be the parent of the new table
copy -- pointer to a table object, if not NULL then the new object will be copied from it
- Returns
pointer to the created table
-
void
lv_table_set_cell_value
(lv_obj_t *table, uint16_t row, uint16_t col, const char *txt)¶ Set the value of a cell.
- Parameters
table -- pointer to a Table object
row -- id of the row [0 .. row_cnt -1]
col -- id of the column [0 .. col_cnt -1]
txt -- text to display in the cell. It will be copied and saved so this variable is not required after this function call.
-
void
lv_table_set_cell_value_fmt
(lv_obj_t *table, uint16_t row, uint16_t col, const char *fmt, ...)¶ Set the value of a cell. Memory will be allocated to store the text by the table.
- Parameters
table -- pointer to a Table object
row -- id of the row [0 .. row_cnt -1]
col -- id of the column [0 .. col_cnt -1]
fmt --
printf
-like format
-
void
lv_table_set_row_cnt
(lv_obj_t *table, uint16_t row_cnt)¶ Set the number of rows
- Parameters
table -- table pointer to a Table object
row_cnt -- number of rows
-
void
lv_table_set_col_cnt
(lv_obj_t *table, uint16_t col_cnt)¶ Set the number of columns
- Parameters
table -- table pointer to a Table object
col_cnt -- number of columns. Must be < LV_TABLE_COL_MAX
-
void
lv_table_set_col_width
(lv_obj_t *table, uint16_t col_id, lv_coord_t w)¶ Set the width of a column
- Parameters
table -- table pointer to a Table object
col_id -- id of the column [0 .. LV_TABLE_COL_MAX -1]
w -- width of the column
-
void
lv_table_set_cell_align
(lv_obj_t *table, uint16_t row, uint16_t col, lv_label_align_t align)¶ Set the text align in a cell
- Parameters
table -- pointer to a Table object
row -- id of the row [0 .. row_cnt -1]
col -- id of the column [0 .. col_cnt -1]
align -- LV_LABEL_ALIGN_LEFT or LV_LABEL_ALIGN_CENTER or LV_LABEL_ALIGN_RIGHT
-
void
lv_table_set_cell_type
(lv_obj_t *table, uint16_t row, uint16_t col, uint8_t type)¶ Set the type of a cell.
- Parameters
table -- pointer to a Table object
row -- id of the row [0 .. row_cnt -1]
col -- id of the column [0 .. col_cnt -1]
type -- 1,2,3 or 4. The cell style will be chosen accordingly.
-
void
lv_table_set_cell_crop
(lv_obj_t *table, uint16_t row, uint16_t col, bool crop)¶ Set the cell crop. (Don't adjust the height of the cell according to its content)
- Parameters
table -- pointer to a Table object
row -- id of the row [0 .. row_cnt -1]
col -- id of the column [0 .. col_cnt -1]
crop -- true: crop the cell content; false: set the cell height to the content.
-
void
lv_table_set_cell_merge_right
(lv_obj_t *table, uint16_t row, uint16_t col, bool en)¶ Merge a cell with the right neighbor. The value of the cell to the right won't be displayed.
- Parameters
table -- table pointer to a Table object
row -- id of the row [0 .. row_cnt -1]
col -- id of the column [0 .. col_cnt -1]
en -- true: merge right; false: don't merge right
-
const char *
lv_table_get_cell_value
(lv_obj_t *table, uint16_t row, uint16_t col)¶ Get the value of a cell.
- Parameters
table -- pointer to a Table object
row -- id of the row [0 .. row_cnt -1]
col -- id of the column [0 .. col_cnt -1]
- Returns
text in the cell
-
uint16_t
lv_table_get_row_cnt
(lv_obj_t *table)¶ Get the number of rows.
- Parameters
table -- table pointer to a Table object
- Returns
number of rows.
-
uint16_t
lv_table_get_col_cnt
(lv_obj_t *table)¶ Get the number of columns.
- Parameters
table -- table pointer to a Table object
- Returns
number of columns.
-
lv_coord_t
lv_table_get_col_width
(lv_obj_t *table, uint16_t col_id)¶ Get the width of a column
- Parameters
table -- table pointer to a Table object
col_id -- id of the column [0 .. LV_TABLE_COL_MAX -1]
- Returns
width of the column
-
lv_label_align_t
lv_table_get_cell_align
(lv_obj_t *table, uint16_t row, uint16_t col)¶ Get the text align of a cell
- Parameters
table -- pointer to a Table object
row -- id of the row [0 .. row_cnt -1]
col -- id of the column [0 .. col_cnt -1]
- Returns
LV_LABEL_ALIGN_LEFT (default in case of error) or LV_LABEL_ALIGN_CENTER or LV_LABEL_ALIGN_RIGHT
-
lv_label_align_t
lv_table_get_cell_type
(lv_obj_t *table, uint16_t row, uint16_t col)¶ Get the type of a cell
- Parameters
table -- pointer to a Table object
row -- id of the row [0 .. row_cnt -1]
col -- id of the column [0 .. col_cnt -1]
- Returns
1,2,3 or 4
-
lv_label_align_t
lv_table_get_cell_crop
(lv_obj_t *table, uint16_t row, uint16_t col)¶ Get the crop property of a cell
- Parameters
table -- pointer to a Table object
row -- id of the row [0 .. row_cnt -1]
col -- id of the column [0 .. col_cnt -1]
- Returns
true: text crop enabled; false: disabled
-
bool
lv_table_get_cell_merge_right
(lv_obj_t *table, uint16_t row, uint16_t col)¶ Get the cell merge attribute.
- Parameters
table -- table pointer to a Table object
row -- id of the row [0 .. row_cnt -1]
col -- id of the column [0 .. col_cnt -1]
- Returns
true: merge right; false: don't merge right
-
lv_res_t
lv_table_get_pressed_cell
(lv_obj_t *table, uint16_t *row, uint16_t *col)¶ Get the last pressed or being pressed cell
- Parameters
table -- pointer to a table object
row -- pointer to variable to store the pressed row
col -- pointer to variable to store the pressed column
- Returns
LV_RES_OK: a valid pressed cell was found, LV_RES_INV: no valid cell is pressed
-
union
lv_table_cell_format_t
¶ - #include <lv_table.h>
Internal table cell format structure.
Use the
lv_table
APIs instead.
-
struct
lv_table_ext_t
¶