Tile view (lv_tileview)¶
Overview¶
The Tileview is a container object where its elements (called tiles) can be arranged in a grid form. By swiping the user can navigate between the tiles.
If the Tileview is screen sized it gives a user interface you might have seen on the smartwatches.
Parts and Styles¶
The Tileview has the same parts as Page.
Expect LV_PAGE_PART_SCRL
because it can't be referenced and it's always transparent.
Refer the Page's documentation of details.
Usage¶
Valid positions¶
The tiles don't have to form a full grid where every element exists. There can be holes in the grid but it has to be continuous, i.e. there can't be an empty rows or columns.
With lv_tileview_set_valid_positions(tileview, valid_pos_array, array_len)
the valid positions can be set.
Scrolling will be possible only to this positions.
The 0,0
index means the top left tile.
E.g. lv_point_t valid_pos_array[] = {{0,0}, {0,1}, {1,1}, {{LV_COORD_MIN, LV_COORD_MIN}}
gives a Tile view with "L" shape.
It indicates that there is no tile in {1,1}
therefore the user can't scroll there.
In other words, the valid_pos_array
tells where the tiles are.
It can be changed on the fly to disable some positions on specific tiles.
For example, there can be a 2x2 grid where all tiles are added but the first row (y = 0) as a "main row" and the second row (y = 1) contains options for the tile above it.
Let's say horizontal scrolling is possible only in the main row and not possible between the options in the second row. In this case the valid_pos_array
needs to changed when a new main tile is selected:
for the first main tile:
{0,0}, {0,1}, {1,0}
to disable the{1,1}
option tilefor the second main tile
{0,0}, {1,0}, {1,1}
to disable the{0,1}
option tile
Set tile¶
To set the currently visible tile use lv_tileview_set_tile_act(tileview, x_id, y_id, LV_ANIM_ON/OFF)
.
Add element¶
To add elements just create an object on the Tileview and position it manually to the desired position.
lv_tileview_add_element(tielview, element)
should be used to make possible to scroll (drag) the Tileview by one its element.
For example, if there is a button on a tile, the button needs to be explicitly added to the Tileview to enable the user to scroll the Tileview with the button too.
Scroll propagation¶
The scroll propagation feature of page-like objects (like List) can be used very well here. For example, there can be a full-sized List and when it reaches the top or bottom most position the user will scroll the tile view instead.
Animation time¶
The animation time of the Tileview can be adjusted with lv_tileview_set_anim_time(tileview, anim_time)
.
Animations are applied when
a new tile is selected with
lv_tileview_set_tile_act
the current tile is scrolled a little and then released (revert the original title)
the current tile is scrolled more than half size and then released (move to the next tile)
Edge flash¶
An "edge flash" effect can be added when the tile view reached hits an invalid position or the end of tile view when scrolled.
Use lv_tileview_set_edge_flash(tileview, true)
to enable this feature.
Events¶
Besides the Generic events the following Special events are sent by the Slider:
LV_EVENT_VALUE_CHANGED Sent when a new tile loaded either with scrolling or
lv_tileview_set_act
. The event data is set ti the index of the new tile invalid_pos_array
(It's type isuint32_t *
)
Keys¶
LV_KEY_UP, LV_KEY_RIGHT Increment the slider's value by 1
LV_KEY_DOWN, LV_KEY_LEFT Decrement the slider's value by 1
Learn more about Keys.
Example¶
C¶
Tileview with content¶
code
#include "../../../lv_examples.h"
#if LV_USE_TILEVIEW
void lv_ex_tileview_1(void)
{
static lv_point_t valid_pos[] = {{0,0}, {0, 1}, {1,1}};
lv_obj_t *tileview;
tileview = lv_tileview_create(lv_scr_act(), NULL);
lv_tileview_set_valid_positions(tileview, valid_pos, 3);
lv_tileview_set_edge_flash(tileview, true);
lv_obj_t * tile1 = lv_obj_create(tileview, NULL);
lv_obj_set_size(tile1, LV_HOR_RES, LV_VER_RES);
lv_tileview_add_element(tileview, tile1);
/*Tile1: just a label*/
lv_obj_t * label = lv_label_create(tile1, NULL);
lv_label_set_text(label, "Scroll down");
lv_obj_align(label, NULL, LV_ALIGN_CENTER, 0, 0);
/*Tile2: a list*/
lv_obj_t * list = lv_list_create(tileview, NULL);
lv_obj_set_size(list, LV_HOR_RES, LV_VER_RES);
lv_obj_set_pos(list, 0, LV_VER_RES);
lv_list_set_scroll_propagation(list, true);
lv_list_set_scrollbar_mode(list, LV_SCROLLBAR_MODE_OFF);
lv_list_add_btn(list, NULL, "One");
lv_list_add_btn(list, NULL, "Two");
lv_list_add_btn(list, NULL, "Three");
lv_list_add_btn(list, NULL, "Four");
lv_list_add_btn(list, NULL, "Five");
lv_list_add_btn(list, NULL, "Six");
lv_list_add_btn(list, NULL, "Seven");
lv_list_add_btn(list, NULL, "Eight");
/*Tile3: a button*/
lv_obj_t * tile3 = lv_obj_create(tileview, tile1);
lv_obj_set_pos(tile3, LV_HOR_RES, LV_VER_RES);
lv_tileview_add_element(tileview, tile3);
lv_obj_t * btn = lv_btn_create(tile3, NULL);
lv_obj_align(btn, NULL, LV_ALIGN_CENTER, 0, 0);
lv_tileview_add_element(tileview, btn);
label = lv_label_create(btn, NULL);
lv_label_set_text(label, "No scroll up");
}
#endif
MicroPython¶
Tileview with content¶
Click to try in the simulator!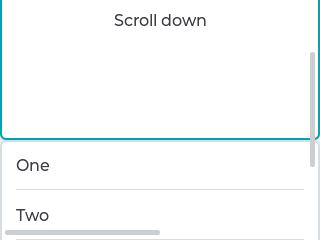
code
LV_HOR_RES=240
LV_VER_RES=240
# create a tileview
valid_pos = [{"x":0, "y": 0}, {"x": 0, "y": 1}, {"x": 1,"y": 1}]
tileview = lv.tileview(lv.scr_act())
tileview.set_valid_positions(valid_pos, len(valid_pos))
tileview.set_edge_flash(True)
tile1 = lv.obj(tileview)
tile1.set_size(LV_HOR_RES, LV_VER_RES)
tileview.add_element(tile1)
# Tile1: just a label
label = lv.label(tile1)
label.set_text("Scroll Down")
label.align(None, lv.ALIGN.CENTER, 0, 0)
# Tile2: a list
lst = lv.list(tileview)
lst.set_size(LV_HOR_RES, LV_VER_RES)
lst.set_pos(0, LV_VER_RES)
lst.set_scroll_propagation(True)
lst.set_scrollbar_mode(lv.SCROLLBAR_MODE.OFF)
tileview.add_element(lst)
list_btn = lst.add_btn(None, "One")
tileview.add_element(list_btn)
list_btn = lst.add_btn(None, "Two")
tileview.add_element(list_btn)
list_btn = lst.add_btn(None, "Three")
tileview.add_element(list_btn)
list_btn = lst.add_btn(None, "Four")
tileview.add_element(list_btn)
list_btn = lst.add_btn(None, "Five")
tileview.add_element(list_btn)
list_btn = lst.add_btn(None, "Six")
tileview.add_element(list_btn)
list_btn = lst.add_btn(None, "Seven")
tileview.add_element(list_btn)
list_btn = lst.add_btn(None, "Eight")
tileview.add_element(list_btn)
# Tile3: a button
tile3 = lv.obj(tileview, tile1)
tile3.set_pos(LV_HOR_RES, LV_VER_RES)
tileview.add_element(tile3)
btn = lv.btn(tile3)
btn.align(None, lv.ALIGN.CENTER, 0, 0)
label = lv.label(btn)
label.set_text("No scroll up")
API¶
Enums
Functions
-
lv_obj_t *
lv_tileview_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a tileview objects
- Parameters
par -- pointer to an object, it will be the parent of the new tileview
copy -- pointer to a tileview object, if not NULL then the new object will be copied from it
- Returns
pointer to the created tileview
-
void
lv_tileview_add_element
(lv_obj_t *tileview, lv_obj_t *element)¶ Register an object on the tileview. The register object will able to slide the tileview
- Parameters
tileview -- pointer to a Tileview object
element -- pointer to an object
-
void
lv_tileview_set_valid_positions
(lv_obj_t *tileview, const lv_point_t valid_pos[], uint16_t valid_pos_cnt)¶ Set the valid position's indices. The scrolling will be possible only to these positions.
- Parameters
tileview -- pointer to a Tileview object
valid_pos -- array width the indices. E.g.
lv_point_t p[] = {{0,0}, {1,0}, {1,1}
. Only the pointer is saved so can't be a local variable.valid_pos_cnt -- number of elements in
valid_pos
array
-
void
lv_tileview_set_tile_act
(lv_obj_t *tileview, lv_coord_t x, lv_coord_t y, lv_anim_enable_t anim)¶ Set the tile to be shown
- Parameters
tileview -- pointer to a tileview object
x -- column id (0, 1, 2...)
y -- line id (0, 1, 2...)
anim -- LV_ANIM_ON: set the value with an animation; LV_ANIM_OFF: change the value immediately
-
static inline void
lv_tileview_set_edge_flash
(lv_obj_t *tileview, bool en)¶ Enable the edge flash effect. (Show an arc when the an edge is reached)
- Parameters
tileview -- pointer to a Tileview
en -- true or false to enable/disable end flash
-
static inline void
lv_tileview_set_anim_time
(lv_obj_t *tileview, uint16_t anim_time)¶ Set the animation time for the Tile view
- Parameters
tileview -- pointer to a page object
anim_time -- animation time in milliseconds
-
void
lv_tileview_get_tile_act
(lv_obj_t *tileview, lv_coord_t *x, lv_coord_t *y)¶ Get the tile to be shown
- Parameters
tileview -- pointer to a tileview object
x -- column id (0, 1, 2...)
y -- line id (0, 1, 2...)
-
struct
lv_tileview_ext_t
¶