Page (lv_page)¶
Parts and Styles¶
The Page's main part is called LV_PAGE_PART_BG
which is the background of the Page. It uses all the typical background style properties. Using padding will add space on the sides.
The scrollable object can be referenced via the LV_PAGE_PART_SCRL
part. It also uses all the typical background style properties and padding to add space on the sides.
LV_LIST_PART_SCROLLBAR
is a virtual part of the background to draw the scroll bars. Uses all the typical background style properties, size to set the width of the scroll bars, and pad_right and pad_bottom to set the spacing.
LV_LIST_PART_EDGE_FLASH
is also a virtual part of the background to draw a semicircle on the sides when the list can not be scrolled in that direction further. Uses all the typical background properties.
Usage¶
The background object can be referenced as the page itself like. E.g. to set the page's width: lv_obj_set_width(page, 100)
.
If a child is created on the page it will be automatically moved to the scrollable container. If the scrollable container becomes larger then the background it can be scrolled by dragging (like the lists on smartphones).
By default, the scrollable's has LV_FIT_MAX
fit in all directions.
It means the scrollable size will be the same as the background's size (minus the padding) while the children are in the background.
But when an object is positioned out of the background the scrollable size will be increased to involve it.
Scrollbars¶
Scrollbars can be shown according to four policies:
LV_SCRLBAR_MODE_OFF
Never show scroll barsLV_SCRLBAR_MODE_ON
Always show scroll barsLV_SCRLBAR_MODE_DRAG
Show scroll bars when the page is being draggedLV_SCRLBAR_MODE_AUTO
Show scroll bars when the scrollable container is large enough to be scrolledLV_SCRLBAR_MODE_HIDE
Hide the scroll bar temporallyLV_SCRLBAR_MODE_UNHIDE
Unhide the previously hidden scrollbar. Recover the original mode too
The scroll bar show policy can be changed by: lv_page_set_scrlbar_mode(page, SB_MODE)
. The default value is LV_SCRLBAR_MODE_AUTO
.
Glue object¶
A children can be "glued" to the page. In this case, if the page can be scrolled by dragging that object.
It can be enabled by the lv_page_glue_obj(child, true)
.
Focus object¶
An object on a page can be focused with lv_page_focus(page, child, LV_ANIM_ONO/FF)
.
It will move the scrollable container to show a child. The time of the animation can be set by lv_page_set_anim_time(page, anim_time)
in milliseconds.
child
doesn't have to be a direct child of the page. This is it works if the scrollable object is the grandparent of the object too.
Edge flash¶
A circle-like effect can be shown if the list reached the most top/bottom/left/right position. lv_page_set_edge_flash(list, en)
enables this feature.
Scroll propagation¶
If the list is created on an other scrollable element (like an other page) and the Page can't be scrolled further the scrolling can be propagated to the parent to continue the scrolling on the parent.
It can be enabled with lv_page_set_scroll_propagation(list, true)
Clean the page¶
All the object created on the page can be clean with lv_page_clean(page)
. Note that lv_obj_clean(page)
doesn't work here because it would delete the scrollable object too.
Scrollable API¶
There are functions to directly set/get the scrollable's attributes:
lv_page_get_scrl()
lv_page_set_scrl_fit/fint2/fit4()
lv_page_set_scrl_width()
lv_page_set_scrl_height()
lv_page_set_scrl_fit_width()
lv_page_set_scrl_fit_height()
lv_page_set_scrl_layout()
Events¶
Only the Generic events are sent by the object type.
The scrollable object has a default event callback which propagates the following events to the background object:
LV_EVENT_PRESSED
, LV_EVENT_PRESSING
, LV_EVENT_PRESS_LOST
,LV_EVENT_RELEASED
, LV_EVENT_SHORT_CLICKED
, LV_EVENT_CLICKED
, LV_EVENT_LONG_PRESSED
, LV_EVENT_LONG_PRESSED_REPEAT
Learn more about Events.
##Keys
The following Keys are processed by the Page:
LV_KEY_RIGHT/LEFT/UP/DOWN Scroll the page
Learn more about Keys.
Example¶
C¶
Page with scrollbar¶
code
#include "../../../lv_examples.h"
#if LV_USE_PAGE
void lv_ex_page_1(void)
{
/*Create a page*/
lv_obj_t * page = lv_page_create(lv_scr_act(), NULL);
lv_obj_set_size(page, 150, 200);
lv_obj_align(page, NULL, LV_ALIGN_CENTER, 0, 0);
/*Create a label on the page*/
lv_obj_t * label = lv_label_create(page, NULL);
lv_label_set_long_mode(label, LV_LABEL_LONG_BREAK); /*Automatically break long lines*/
lv_obj_set_width(label, lv_page_get_width_fit(page)); /*Set the label width to max value to not show hor. scroll bars*/
lv_label_set_text(label, "Lorem ipsum dolor sit amet, consectetur adipiscing elit,\n"
"sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.\n"
"Ut enim ad minim veniam, quis nostrud exercitation ullamco\n"
"laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure\n"
"dolor in reprehenderit in voluptate velit esse cillum dolore\n"
"eu fugiat nulla pariatur.\n"
"Excepteur sint occaecat cupidatat non proident, sunt in culpa\n"
"qui officia deserunt mollit anim id est laborum.");
}
#endif
MicroPython¶
Page with scrollbar¶
Click to try in the simulator!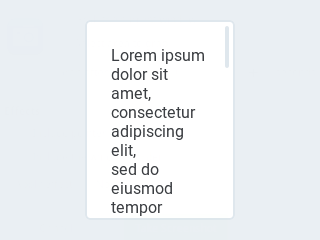
code
# create a page
page = lv.page(lv.scr_act(),None)
page.set_size(150,200)
page.align(None,lv.ALIGN.CENTER,0,0)
# page.set_scrollbar_mode(lv.SCROLLBAR_MODE.OFF)
# Create a label on the page
label = lv.label(page, None);
label.set_long_mode(lv.label.LONG.BREAK); # Automatically break long lines
label.set_width(lv.page.get_width_fit(page)); # Set the label width to max value to not show hor. scroll bars*/
label.set_text("Lorem ipsum dolor sit amet, consectetur adipiscing elit,\n"
"sed do eiusmod tempor incididunt ut labore et dolore magna aliqua.\n"
"Ut enim ad minim veniam, quis nostrud exercitation ullamco\n"
"laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure\n"
"dolor in reprehenderit in voluptate velit esse cillum dolore\n"
"eu fugiat nulla pariatur.\n"
"Excepteur sint occaecat cupidatat non proident, sunt in culpa\n"
"qui officia deserunt mollit anim id est laborum.")
API¶
Typedefs
-
typedef uint8_t
lv_scrollbar_mode_t
¶
-
typedef uint8_t
lv_page_edge_t
¶
-
typedef uint8_t
lv_part_style_t
¶
Enums
-
enum [anonymous]¶
Scrollbar modes: shows when should the scrollbars be visible
Values:
-
enumerator
LV_SCROLLBAR_MODE_OFF
¶ Never show scroll bars
-
enumerator
LV_SCROLLBAR_MODE_ON
¶ Always show scroll bars
-
enumerator
LV_SCROLLBAR_MODE_DRAG
¶ Show scroll bars when page is being dragged
-
enumerator
LV_SCROLLBAR_MODE_AUTO
¶ Show scroll bars when the scrollable container is large enough to be scrolled
-
enumerator
LV_SCROLLBAR_MODE_HIDE
¶ Hide the scroll bar temporally
-
enumerator
LV_SCROLLBAR_MODE_UNHIDE
¶ Unhide the previously hidden scroll bar. Recover original mode too
-
enumerator
Functions
-
lv_obj_t *
lv_page_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a page objects
- Parameters
par -- pointer to an object, it will be the parent of the new page
copy -- pointer to a page object, if not NULL then the new object will be copied from it
- Returns
pointer to the created page
-
void
lv_page_clean
(lv_obj_t *page)¶ Delete all children of the scrl object, without deleting scrl child.
- Parameters
page -- pointer to an object
-
lv_obj_t *
lv_page_get_scrollable
(const lv_obj_t *page)¶ Get the scrollable object of a page
- Parameters
page -- pointer to a page object
- Returns
pointer to a container which is the scrollable part of the page
-
uint16_t
lv_page_get_anim_time
(const lv_obj_t *page)¶ Get the animation time
- Parameters
page -- pointer to a page object
- Returns
the animation time in milliseconds
-
void
lv_page_set_scrollbar_mode
(lv_obj_t *page, lv_scrollbar_mode_t sb_mode)¶ Set the scroll bar mode on a page
- Parameters
page -- pointer to a page object
sb_mode -- the new mode from 'lv_page_sb.mode_t' enum
-
void
lv_page_set_anim_time
(lv_obj_t *page, uint16_t anim_time)¶ Set the animation time for the page
- Parameters
page -- pointer to a page object
anim_time -- animation time in milliseconds
-
void
lv_page_set_scroll_propagation
(lv_obj_t *page, bool en)¶ Enable the scroll propagation feature. If enabled then the page will move its parent if there is no more space to scroll. The page needs to have a page-like parent (e.g.
lv_page
,lv_tabview
tab,lv_win
content area etc) If enabled drag direction will be changedLV_DRAG_DIR_ONE
automatically to allow scrolling only in one direction at one time.- Parameters
page -- pointer to a Page
en -- true or false to enable/disable scroll propagation
-
void
lv_page_set_edge_flash
(lv_obj_t *page, bool en)¶ Enable the edge flash effect. (Show an arc when the an edge is reached)
- Parameters
page -- pointer to a Page
en -- true or false to enable/disable end flash
-
static inline void
lv_page_set_scrollable_fit4
(lv_obj_t *page, lv_fit_t left, lv_fit_t right, lv_fit_t top, lv_fit_t bottom)¶ Set the fit policy in all 4 directions separately. It tell how to change the page size automatically.
- Parameters
page -- pointer to a page object
left -- left fit policy from
lv_fit_t
right -- right fit policy from
lv_fit_t
top -- bottom fit policy from
lv_fit_t
bottom -- bottom fit policy from
lv_fit_t
-
static inline void
lv_page_set_scrollable_fit2
(lv_obj_t *page, lv_fit_t hor, lv_fit_t ver)¶ Set the fit policy horizontally and vertically separately. It tell how to change the page size automatically.
- Parameters
page -- pointer to a page object
hot -- horizontal fit policy from
lv_fit_t
ver -- vertical fit policy from
lv_fit_t
-
static inline void
lv_page_set_scrollable_fit
(lv_obj_t *page, lv_fit_t fit)¶ Set the fit policy in all 4 direction at once. It tell how to change the page size automatically.
- Parameters
page -- pointer to a button object
fit -- fit policy from
lv_fit_t
-
static inline void
lv_page_set_scrl_width
(lv_obj_t *page, lv_coord_t w)¶ Set width of the scrollable part of a page
- Parameters
page -- pointer to a page object
w -- the new width of the scrollable (it ha no effect is horizontal fit is enabled)
-
static inline void
lv_page_set_scrl_height
(lv_obj_t *page, lv_coord_t h)¶ Set height of the scrollable part of a page
- Parameters
page -- pointer to a page object
h -- the new height of the scrollable (it ha no effect is vertical fit is enabled)
-
static inline void
lv_page_set_scrl_layout
(lv_obj_t *page, lv_layout_t layout)¶ Set the layout of the scrollable part of the page
- Parameters
page -- pointer to a page object
layout -- a layout from 'lv_cont_layout_t'
-
lv_scrollbar_mode_t
lv_page_get_scrollbar_mode
(const lv_obj_t *page)¶ Set the scroll bar mode on a page
- Parameters
page -- pointer to a page object
- Returns
the mode from 'lv_page_sb.mode_t' enum
-
bool
lv_page_get_scroll_propagation
(lv_obj_t *page)¶ Get the scroll propagation property
- Parameters
page -- pointer to a Page
- Returns
true or false
-
bool
lv_page_get_edge_flash
(lv_obj_t *page)¶ Get the edge flash effect property.
- Parameters
page -- pointer to a Page return true or false
-
lv_coord_t
lv_page_get_width_fit
(lv_obj_t *page)¶ Get that width which can be set to the children to still not cause overflow (show scrollbars)
- Parameters
page -- pointer to a page object
- Returns
the width which still fits into the page
-
lv_coord_t
lv_page_get_height_fit
(lv_obj_t *page)¶ Get that height which can be set to the children to still not cause overflow (show scrollbars)
- Parameters
page -- pointer to a page object
- Returns
the height which still fits into the page
-
lv_coord_t
lv_page_get_width_grid
(lv_obj_t *page, uint8_t div, uint8_t span)¶ Divide the width of the object and get the width of a given number of columns. Take into account the paddings of the background and scrollable too.
- Parameters
page -- pointer to an object
div -- indicates how many columns are assumed. If 1 the width will be set the parent's width If 2 only half parent width - inner padding of the parent If 3 only third parent width - 2 * inner padding of the parent
span -- how many columns are combined
- Returns
the width according to the given parameters
-
lv_coord_t
lv_page_get_height_grid
(lv_obj_t *page, uint8_t div, uint8_t span)¶ Divide the height of the object and get the width of a given number of columns. Take into account the paddings of the background and scrollable too.
- Parameters
page -- pointer to an object
div -- indicates how many rows are assumed. If 1 the height will be set the parent's height If 2 only half parent height - inner padding of the parent If 3 only third parent height - 2 * inner padding of the parent
span -- how many rows are combined
- Returns
the height according to the given parameters
-
static inline lv_coord_t
lv_page_get_scrl_width
(const lv_obj_t *page)¶ Get width of the scrollable part of a page
- Parameters
page -- pointer to a page object
- Returns
the width of the scrollable
-
static inline lv_coord_t
lv_page_get_scrl_height
(const lv_obj_t *page)¶ Get height of the scrollable part of a page
- Parameters
page -- pointer to a page object
- Returns
the height of the scrollable
-
static inline lv_layout_t
lv_page_get_scrl_layout
(const lv_obj_t *page)¶ Get the layout of the scrollable part of a page
- Parameters
page -- pointer to page object
- Returns
the layout from 'lv_cont_layout_t'
-
static inline lv_fit_t
lv_page_get_scrl_fit_left
(const lv_obj_t *page)¶ Get the left fit mode
- Parameters
page -- pointer to a page object
- Returns
an element of
lv_fit_t
-
static inline lv_fit_t
lv_page_get_scrl_fit_right
(const lv_obj_t *page)¶ Get the right fit mode
- Parameters
page -- pointer to a page object
- Returns
an element of
lv_fit_t
-
static inline lv_fit_t
lv_page_get_scrl_fit_top
(const lv_obj_t *page)¶ Get the top fit mode
- Parameters
page -- pointer to a page object
- Returns
an element of
lv_fit_t
-
static inline lv_fit_t
lv_page_get_scrl_fit_bottom
(const lv_obj_t *page)¶ Get the bottom fit mode
- Parameters
page -- pointer to a page object
- Returns
an element of
lv_fit_t
-
bool
lv_page_on_edge
(lv_obj_t *page, lv_page_edge_t edge)¶ Find whether the page has been scrolled to a certain edge.
- Parameters
page -- Page object
edge -- Edge to check
- Returns
true if the page is on the specified edge
-
void
lv_page_glue_obj
(lv_obj_t *obj, bool glue)¶ Glue the object to the page. After it the page can be moved (dragged) with this object too.
- Parameters
obj -- pointer to an object on a page
glue -- true: enable glue, false: disable glue
-
void
lv_page_focus
(lv_obj_t *page, const lv_obj_t *obj, lv_anim_enable_t anim_en)¶ Focus on an object. It ensures that the object will be visible on the page.
- Parameters
page -- pointer to a page object
obj -- pointer to an object to focus (must be on the page)
anim_en -- LV_ANIM_ON to focus with animation; LV_ANIM_OFF to focus without animation
-
void
lv_page_scroll_hor
(lv_obj_t *page, lv_coord_t dist)¶ Scroll the page horizontally
- Parameters
page -- pointer to a page object
dist -- the distance to scroll (< 0: scroll left; > 0 scroll right)
-
void
lv_page_scroll_ver
(lv_obj_t *page, lv_coord_t dist)¶ Scroll the page vertically
- Parameters
page -- pointer to a page object
dist -- the distance to scroll (< 0: scroll down; > 0 scroll up)
-
void
lv_page_start_edge_flash
(lv_obj_t *page, lv_page_edge_t edge)¶ Not intended to use directly by the user but by other object types internally. Start an edge flash animation.
- Parameters
page --
edge -- the edge to flash. Can be
LV_PAGE_EDGE_LEFT/RIGHT/TOP/BOTTOM
-
struct
lv_page_ext_t
¶ Public Members
-
lv_style_list_t
style
¶
-
lv_area_t
hor_area
¶
-
lv_area_t
ver_area
¶
-
uint8_t
hor_draw
¶
-
uint8_t
ver_draw
¶
-
lv_scrollbar_mode_t
mode
¶
-
struct lv_page_ext_t::[anonymous]
scrlbar
¶
-
lv_anim_value_t
state
¶
-
uint8_t
enabled
¶
-
uint8_t
top_ip
¶
-
uint8_t
bottom_ip
¶
-
uint8_t
right_ip
¶
-
uint8_t
left_ip
¶
-
struct lv_page_ext_t::[anonymous]
edge_flash
¶
-
uint16_t
anim_time
¶
-
uint8_t
scroll_prop
¶
-
lv_style_list_t