Checkbox (lv_cb)¶
Overview¶
The Checkbox objects are built from a Button background which contains an also Button bullet and a Label to realize a classical checkbox.
Parts and Styles¶
The Check box's main part is called LV_CHECKBOX_PART_BG
. It's a container for a "bullet" and a text next to it. The background uses all the typical background style properties.
The bullet is real lv_obj object and can be referred with LV_CHECKBOX_PART_BULLET
.
The bullet automatically inherits the state of the background. So the background is pressed the bullet goes to pressed state as well.
The bullet also uses all the typical background style properties.
There is not dedicated part for the label. Its styles can be set in the background's styles because the text styles properties are always inherited.
Usage¶
Text¶
The text can be modified by the lv_checkbox_set_text(cb, "New text")
function. It will dynamically allocate the text.
To set a static text, use lv_checkbox_set_static_text(cb, txt)
. This way, only a pointer of txt
will be stored and it shouldn't be deallocated while the checkbox exists.
Check/Uncheck¶
You can manually check / un-check the Checkbox via lv_checkbox_set_checked(cb, true/false)
. Setting true
will check the checkbox and false
will un-check the checkbox.
Disabled¶
To make the Checkbox disabled, use lv_checkbox_set_disabled(cb, true)
.
Get/Set Checkbox State¶
You can get the current state of the Checkbox with the lv_checkbox_get_state(cb)
function which returns the current state.
You can set the current state of the Checkbox with the lv_checkbox_set_state(cb, state)
.
The available states as defined by the enum lv_btn_state_t
are:
LV_BTN_STATE_RELEASED
LV_BTN_STATE_PRESSED
LV_BTN_STATE_DISABLED
LV_BTN_STATE_CHECKED_RELEASED
LV_BTN_STATE_CHECKED_PRESSED
LV_BTN_STATE_CHECKED_DISABLED
Events¶
Besides the Generic events the following Special events are sent by the Checkboxes:
LV_EVENT_VALUE_CHANGED - sent when the checkbox is toggled.
Note that, the generic input device-related events (like LV_EVENT_PRESSED
) are sent in the inactive state too. You need to check the state with lv_cb_is_inactive(cb)
to ignore the events from inactive Checkboxes.
Learn more about Events.
Keys¶
The following Keys are processed by the 'Buttons':
LV_KEY_RIGHT/UP - Go to toggled state if toggling is enabled
LV_KEY_LEFT/DOWN - Go to non-toggled state if toggling is enabled
Note that, as usual, the state of LV_KEY_ENTER
is translated to LV_EVENT_PRESSED/PRESSING/RELEASED
etc.
Learn more about Keys.
Example¶
C¶
Simple Checkbox¶
code
#include "../../../lv_examples.h"
#include <stdio.h>
#if LV_USE_CHECKBOX
static void event_handler(lv_obj_t * obj, lv_event_t event)
{
if(event == LV_EVENT_VALUE_CHANGED) {
printf("State: %s\n", lv_checkbox_is_checked(obj) ? "Checked" : "Unchecked");
}
}
void lv_ex_checkbox_1(void)
{
lv_obj_t * cb = lv_checkbox_create(lv_scr_act(), NULL);
lv_checkbox_set_text(cb, "I agree to terms and conditions.");
lv_obj_align(cb, NULL, LV_ALIGN_CENTER, 0, 0);
lv_obj_set_event_cb(cb, event_handler);
}
#endif
MicroPython¶
Simple Checkbox¶
Click to try in the simulator!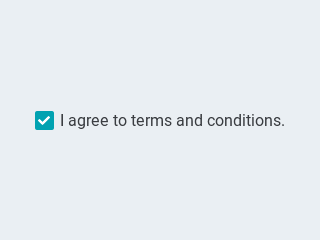
code
def event_handler(source,evt):
if evt == lv.EVENT.VALUE_CHANGED:
if source.is_checked():
print("State: checked")
else:
print("State: unchecked")
# create a checkbox
cb = lv.checkbox(lv.scr_act(),None)
cb.set_text("I agree to terms\nand conditions.")
cb.align(None,lv.ALIGN.CENTER, 0, 0)
# attach the callback
cb.set_event_cb(event_handler)
API¶
Typedefs
-
typedef uint8_t
lv_checkbox_style_t
¶
Enums
Functions
-
lv_obj_t *
lv_checkbox_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a check box objects
- Parameters
par -- pointer to an object, it will be the parent of the new check box
copy -- pointer to a check box object, if not NULL then the new object will be copied from it
- Returns
pointer to the created check box
-
void
lv_checkbox_set_text
(lv_obj_t *cb, const char *txt)¶ Set the text of a check box.
txt
will be copied and may be deallocated after this function returns.- Parameters
cb -- pointer to a check box
txt -- the text of the check box. NULL to refresh with the current text.
-
void
lv_checkbox_set_text_static
(lv_obj_t *cb, const char *txt)¶ Set the text of a check box.
txt
must not be deallocated during the life of this checkbox.- Parameters
cb -- pointer to a check box
txt -- the text of the check box. NULL to refresh with the current text.
-
void
lv_checkbox_set_checked
(lv_obj_t *cb, bool checked)¶ Set the state of the check box
- Parameters
cb -- pointer to a check box object
checked -- true: make the check box checked; false: make it unchecked
-
void
lv_checkbox_set_disabled
(lv_obj_t *cb)¶ Make the check box inactive (disabled)
- Parameters
cb -- pointer to a check box object
-
void
lv_checkbox_set_state
(lv_obj_t *cb, lv_btn_state_t state)¶ Set the state of a check box
- Parameters
cb -- pointer to a check box object
state -- the new state of the check box (from lv_btn_state_t enum)
-
const char *
lv_checkbox_get_text
(const lv_obj_t *cb)¶ Get the text of a check box
- Parameters
cb -- pointer to check box object
- Returns
pointer to the text of the check box
-
static inline bool
lv_checkbox_is_checked
(const lv_obj_t *cb)¶ Get the current state of the check box
- Parameters
cb -- pointer to a check box object
- Returns
true: checked; false: not checked
-
static inline bool
lv_checkbox_is_inactive
(const lv_obj_t *cb)¶ Get whether the check box is inactive or not.
- Parameters
cb -- pointer to a check box object
- Returns
true: inactive; false: not inactive
-
static inline lv_btn_state_t
lv_checkbox_get_state
(const lv_obj_t *cb)¶ Get the current state of a check box
- Parameters
cb -- pointer to a check box object
- Returns
the state of the check box (from lv_btn_state_t enum)
-
struct
lv_checkbox_ext_t
¶