Button matrix (lv_btnmatrix)¶
Overview¶
The Button Matrix objects can display multiple buttons in rows and columns.
The main reasons for wanting to use a button matrix instead of a container and individual button objects are:
The button matrix is simpler to use for grid-based button layouts.
The button matrix consumes a lot less memory per button.
Parts and Styles¶
The Button matrix's main part is called LV_BTNMATRIX_PART_BG
. It draws a background using the typical background style properties.
LV_BTNMATRIX_PART_BTN
is virtual part and it refers to the buttons on the button matrix. It also uses all the typical background properties.
The top/bottom/left/right padding values from the background are used to keep some space on the sides. Inner padding is applied between the buttons.
Usage¶
Button's text¶
There is a text on each button. To specify them a descriptor string array, called map, needs to be used.
The map can be set with lv_btnmatrix_set_map(btnm, my_map)
.
The declaration of a map should look like const char * map[] = {"btn1", "btn2", "btn3", ""}
.
Note that the last element has to be an empty string!
Use "\n"
in the map to make line break. E.g. {"btn1", "btn2", "\n", "btn3", ""}
. Each line's buttons have their width calculated automatically.
Control buttons¶
The buttons width can be set relative to the other button in the same line with lv_btnmatrix_set_btn_width(btnm, btn_id, width)
E.g. in a line with two buttons: btnA, width = 1 and btnB, width = 2, btnA will have 33 % width and btnB will have 66 % width.
It's similar to how the flex-grow
property works in CSS.
In addition to width, each button can be customized with the following parameters:
LV_BTNMATRIX_CTRL_HIDDEN - make a button hidden (hidden buttons still take up space in the layout, they are just not visible or clickable)
LV_BTNMATRIX_CTRL_NO_REPEAT - disable repeating when the button is long pressed
LV_BTNMATRIX_CTRL_DISABLED - make a button disabled
LV_BTNMATRIX_CTRL_CHECKABLE - enable toggling of a button
LV_BTNMATRIX_CTRL_CHECK_STATE - set the toggle state
LV_BTNMATRIX_CTRL_CLICK_TRIG - if 0, the button will react on press, if 1, will react on release
The set or clear a button's control attribute, use lv_btnmatrix_set_btn_ctrl(btnm, btn_id, LV_BTNM_CTRL_...)
and
lv_btnmatrix_clear_btn_ctrl(btnm, btn_id, LV_BTNM_CTRL_...)
respectively. More LV_BTNM_CTRL_...
values can be Ored
The set/clear the same control attribute for all buttons of a button matrix, use lv_btnmatrix_set_btn_ctrl_all(btnm, btn_id, LV_BTNM_CTRL_...)
and
lv_btnmatrix_clear_btn_ctrl_all(btnm, btn_id, LV_BTNM_CTRL_...)
.
The set a control map for a button matrix (similarly to the map for the text), use lv_btnmatrix_set_ctrl_map(btnm, ctrl_map)
.
An element of ctrl_map
should look like ctrl_map[0] = width | LV_BTNM_CTRL_NO_REPEAT | LV_BTNM_CTRL_TGL_ENABLE
.
The number of elements should be equal to the number of buttons (excluding newlines characters).
One check¶
The "One check" feature can be enabled with lv_btnmatrix_set_one_check(btnm, true)
to allow only one button to be checked (toggled) at once.
Recolor¶
The texts on the button can be recolored similarly to the recolor feature for Label object. To enable it, use lv_btnmatrix_set_recolor(btnm, true)
.
After that a button with #FF0000 Red#
text will be red.
Aligning the button's text¶
To align the text on the buttons, use lv_btnmatrix_set_align(roller, LV_LABEL_ALIGN_LEFT/CENTER/RIGHT)
.
All text items in the button matrix will conform to the alignment proprty as it is set.
Notes¶
The Button matrix object is very light weighted because the buttons are not created just virtually drawn on the fly. This way, 1 button use only 8 extra bytes instead of the ~100-150 byte size of a normal Button object (plus the size of its container and a label for each button).
The disadvantage of this setup is that the ability to style individual buttons to be different from others is limited (aside from the toggling feature). If you require that ability, using individual buttons is very likely to be a better approach.
Events¶
Besides the Generic events, the following Special events are sent by the button matrices:
LV_EVENT_VALUE_CHANGED - sent when the button is pressed/released or repeated after long press. The event data is set to the ID of the pressed/released button.
Learn more about Events.
Keys¶
The following Keys are processed by the Buttons:
LV_KEY_RIGHT/UP/LEFT/RIGHT - To navigate among the buttons to select one
LV_KEY_ENTER - To press/release the selected button
Learn more about Keys.
Example¶
C¶
Simple Button matrix¶
code
#include "../../../lv_examples.h"
#include <stdio.h>
#if LV_USE_BTNMATRIX
static void event_handler(lv_obj_t * obj, lv_event_t event)
{
if(event == LV_EVENT_VALUE_CHANGED) {
const char * txt = lv_btnmatrix_get_active_btn_text(obj);
printf("%s was pressed\n", txt);
}
}
static const char * btnm_map[] = {"1", "2", "3", "4", "5", "\n",
"6", "7", "8", "9", "0", "\n",
"Action1", "Action2", ""};
void lv_ex_btnmatrix_1(void)
{
lv_obj_t * btnm1 = lv_btnmatrix_create(lv_scr_act(), NULL);
lv_btnmatrix_set_map(btnm1, btnm_map);
lv_btnmatrix_set_btn_width(btnm1, 10, 2); /*Make "Action1" twice as wide as "Action2"*/
lv_btnmatrix_set_btn_ctrl(btnm1, 10, LV_BTNMATRIX_CTRL_CHECKABLE);
lv_btnmatrix_set_btn_ctrl(btnm1, 11, LV_BTNMATRIX_CTRL_CHECK_STATE);
lv_obj_align(btnm1, NULL, LV_ALIGN_CENTER, 0, 0);
lv_obj_set_event_cb(btnm1, event_handler);
}
#endif
MicroPython¶
Simple Button matrix¶
Click to try in the simulator!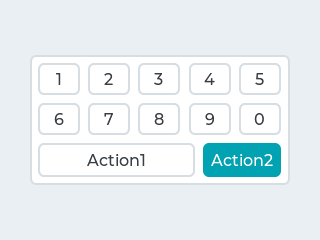
code
def event_handler(source,evt):
if evt == lv.EVENT.VALUE_CHANGED:
print("Toggled")
txt = source.get_active_btn_text()
print("%s was pressed"%txt)
# Create a screen and load it
scr = lv.obj()
lv.scr_load(scr)
btnm_map = ["1","2", "3", "4", "5", "\n",
"6", "7", "8", "9", "0", "\n",
"Action1", "Action2", ""]
btnm_ctlr_map = [46|lv.btnmatrix.CTRL.NO_REPEAT,46,46,46,46,
46,46,46,46,46,
115,115]
# create a button matrix
btnm1 = lv.btnmatrix(lv.scr_act(),None)
btnm1.set_map(btnm_map)
#btnm1.set_ctrl_map(btnm_ctlr_map)
btnm1.set_btn_ctrl(10,lv.btnmatrix.CTRL.CHECKABLE)
btnm1.set_btn_ctrl(11,lv.btnmatrix.CTRL.CHECK_STATE)
btnm1.set_width(230)
btnm1.align(None,lv.ALIGN.CENTER,0,0)
# attach the callback
btnm1.set_event_cb(event_handler)
API¶
Enums
-
enum [anonymous]¶
Type to store button control bits (disabled, hidden etc.) The first 3 bits are used to store the width
Values:
-
enumerator
LV_BTNMATRIX_CTRL_HIDDEN
¶ Button hidden
-
enumerator
LV_BTNMATRIX_CTRL_NO_REPEAT
¶ Do not repeat press this button.
-
enumerator
LV_BTNMATRIX_CTRL_DISABLED
¶ Disable this button.
-
enumerator
LV_BTNMATRIX_CTRL_CHECKABLE
¶ Button can be toggled.
-
enumerator
LV_BTNMATRIX_CTRL_CHECK_STATE
¶ Button is currently toggled (e.g. checked).
-
enumerator
LV_BTNMATRIX_CTRL_CLICK_TRIG
¶ 1: Send LV_EVENT_SELECTED on CLICK, 0: Send LV_EVENT_SELECTED on PRESS
-
enumerator
Functions
-
LV_EXPORT_CONST_INT
(LV_BTNMATRIX_BTN_NONE)¶
-
lv_obj_t *
lv_btnmatrix_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a button matrix objects
- Parameters
par -- pointer to an object, it will be the parent of the new button matrix
copy -- pointer to a button matrix object, if not NULL then the new object will be copied from it
- Returns
pointer to the created button matrix
-
void
lv_btnmatrix_set_map
(lv_obj_t *btnm, const char *map[])¶ Set a new map. Buttons will be created/deleted according to the map. The button matrix keeps a reference to the map and so the string array must not be deallocated during the life of the matrix.
- Parameters
btnm -- pointer to a button matrix object
map -- pointer a string array. The last string has to be: "". Use "\n" to make a line break.
-
void
lv_btnmatrix_set_ctrl_map
(lv_obj_t *btnm, const lv_btnmatrix_ctrl_t ctrl_map[])¶ Set the button control map (hidden, disabled etc.) for a button matrix. The control map array will be copied and so may be deallocated after this function returns.
- Parameters
btnm -- pointer to a button matrix object
ctrl_map -- pointer to an array of
lv_btn_ctrl_t
control bytes. The length of the array and position of the elements must match the number and order of the individual buttons (i.e. excludes newline entries). An element of the map should look like e.g.:ctrl_map[0] = width | LV_BTNMATRIX_CTRL_NO_REPEAT | LV_BTNMATRIX_CTRL_TGL_ENABLE
-
void
lv_btnmatrix_set_focused_btn
(lv_obj_t *btnm, uint16_t id)¶ Set the focused button i.e. visually highlight it.
- Parameters
btnm -- pointer to button matrix object
id -- index of the button to focus(
LV_BTNMATRIX_BTN_NONE
to remove focus)
-
void
lv_btnmatrix_set_recolor
(const lv_obj_t *btnm, bool en)¶ Enable recoloring of button's texts
- Parameters
btnm -- pointer to button matrix object
en -- true: enable recoloring; false: disable
-
void
lv_btnmatrix_set_btn_ctrl
(lv_obj_t *btnm, uint16_t btn_id, lv_btnmatrix_ctrl_t ctrl)¶ Set the attributes of a button of the button matrix
- Parameters
btnm -- pointer to button matrix object
btn_id -- 0 based index of the button to modify. (Not counting new lines)
-
void
lv_btnmatrix_clear_btn_ctrl
(const lv_obj_t *btnm, uint16_t btn_id, lv_btnmatrix_ctrl_t ctrl)¶ Clear the attributes of a button of the button matrix
- Parameters
btnm -- pointer to button matrix object
btn_id -- 0 based index of the button to modify. (Not counting new lines)
-
void
lv_btnmatrix_set_btn_ctrl_all
(lv_obj_t *btnm, lv_btnmatrix_ctrl_t ctrl)¶ Set the attributes of all buttons of a button matrix
- Parameters
btnm -- pointer to a button matrix object
ctrl -- attribute(s) to set from
lv_btnmatrix_ctrl_t
. Values can be ORed.
-
void
lv_btnmatrix_clear_btn_ctrl_all
(lv_obj_t *btnm, lv_btnmatrix_ctrl_t ctrl)¶ Clear the attributes of all buttons of a button matrix
- Parameters
btnm -- pointer to a button matrix object
ctrl -- attribute(s) to set from
lv_btnmatrix_ctrl_t
. Values can be ORed.en -- true: set the attributes; false: clear the attributes
-
void
lv_btnmatrix_set_btn_width
(lv_obj_t *btnm, uint16_t btn_id, uint8_t width)¶ Set a single buttons relative width. This method will cause the matrix be regenerated and is a relatively expensive operation. It is recommended that initial width be specified using
lv_btnmatrix_set_ctrl_map
and this method only be used for dynamic changes.- Parameters
btnm -- pointer to button matrix object
btn_id -- 0 based index of the button to modify.
width -- Relative width compared to the buttons in the same row. [1..7]
-
void
lv_btnmatrix_set_one_check
(lv_obj_t *btnm, bool one_chk)¶ Make the button matrix like a selector widget (only one button may be toggled at a time).
Checkable
must be enabled on the buttons you want to be selected withlv_btnmatrix_set_ctrl
orlv_btnmatrix_set_btn_ctrl_all
.- Parameters
btnm -- Button matrix object
one_chk -- Whether "one check" mode is enabled
-
void
lv_btnmatrix_set_align
(lv_obj_t *btnm, lv_label_align_t align)¶ Set the align of the map text (left, right or center)
- Parameters
btnm -- pointer to a btnmatrix object
align -- LV_LABEL_ALIGN_LEFT, LV_LABEL_ALIGN_RIGHT or LV_LABEL_ALIGN_CENTER
-
const char **
lv_btnmatrix_get_map_array
(const lv_obj_t *btnm)¶ Get the current map of a button matrix
- Parameters
btnm -- pointer to a button matrix object
- Returns
the current map
-
bool
lv_btnmatrix_get_recolor
(const lv_obj_t *btnm)¶ Check whether the button's text can use recolor or not
- Parameters
btnm -- pointer to button matrix object
- Returns
true: text recolor enable; false: disabled
-
uint16_t
lv_btnmatrix_get_active_btn
(const lv_obj_t *btnm)¶ Get the index of the lastly "activated" button by the user (pressed, released etc) Useful in the
event_cb
to get the text of the button, check if hidden etc.- Parameters
btnm -- pointer to button matrix object
- Returns
index of the last released button (LV_BTNMATRIX_BTN_NONE: if unset)
-
const char *
lv_btnmatrix_get_active_btn_text
(const lv_obj_t *btnm)¶ Get the text of the lastly "activated" button by the user (pressed, released etc) Useful in the
event_cb
- Parameters
btnm -- pointer to button matrix object
- Returns
text of the last released button (NULL: if unset)
-
uint16_t
lv_btnmatrix_get_focused_btn
(const lv_obj_t *btnm)¶ Get the focused button's index.
- Parameters
btnm -- pointer to button matrix object
- Returns
index of the focused button (LV_BTNMATRIX_BTN_NONE: if unset)
-
const char *
lv_btnmatrix_get_btn_text
(const lv_obj_t *btnm, uint16_t btn_id)¶ Get the button's text
- Parameters
btnm -- pointer to button matrix object
btn_id -- the index a button not counting new line characters. (The return value of lv_btnmatrix_get_pressed/released)
- Returns
text of btn_index` button
-
bool
lv_btnmatrix_get_btn_ctrl
(lv_obj_t *btnm, uint16_t btn_id, lv_btnmatrix_ctrl_t ctrl)¶ Get the whether a control value is enabled or disabled for button of a button matrix
- Parameters
btnm -- pointer to a button matrix object
btn_id -- the index a button not counting new line characters. (E.g. the return value of lv_btnmatrix_get_pressed/released)
ctrl -- control values to check (ORed value can be used)
- Returns
true: long press repeat is disabled; false: long press repeat enabled
-
bool
lv_btnmatrix_get_one_check
(const lv_obj_t *btnm)¶ Find whether "one toggle" mode is enabled.
- Parameters
btnm -- Button matrix object
- Returns
whether "one toggle" mode is enabled
-
lv_label_align_t
lv_btnmatrix_get_align
(const lv_obj_t *btnm)¶ Get the align attribute
- Parameters
btnm -- pointer to a btnmatrix object
- Returns
LV_LABEL_ALIGN_LEFT, LV_LABEL_ALIGN_RIGHT or LV_LABEL_ALIGN_CENTER
-
struct
lv_btnmatrix_ext_t
¶