Spinbox (lv_spinbox)¶
Overview¶
The Spinbox contains a number as text which can be increased or decreased by Keys or API functions. Under the hood the Spinbox is a modified Text area.
Parts and Styles¶
The Spinbox's main part is called LV_SPINBOX_PART_BG
which is a rectangle-like background using all the typical background style properties. It also describes the style of the label with its text style properties.
LV_SPINBOX_PART_CURSOR
is a virtual part describing the cursor. Read the Text area documentation for a detailed description.
Set format¶
lv_spinbox_set_digit_format(spinbox, digit_count, separator_position)
set the format of the number.
digit_count
sets the number of digits. Leading zeros are added to fill the space on the left.
separator_position
sets the number of digit before the decimal point. 0
means no decimal point.
lv_spinbox_set_padding_left(spinbox, cnt)
add cnt
"space" characters between the sign an the most left digit.
Value and ranges¶
lv_spinbox_set_range(spinbox, min, max)
sets the range of the Spinbox.
lv_spinbox_set_value(spinbox, num)
sets the Spinbox's value manually.
lv_spinbox_increment(spinbox)
and lv_spinbox_decrement(spinbox)
increments/decrements the value of the Spinbox.
lv_spinbox_set_step(spinbox, step)
sets the amount to increment decrement.
Events¶
Besides the Generic events the following Special events are sent by the Drop down lists:
LV_EVENT_VALUE_CHANGED sent when the value has changed. (the value is set as event data as
int32_t
)LV_EVENT_INSERT sent by the ancestor Text area but shouldn't be used.
Learn more about Events.
Keys¶
The following Keys are processed by the Buttons:
LV_KEY_LEFT/RIGHT With Keypad move the cursor left/right. With Encoder decrement/increment the selected digit.
LY_KEY_ENTER Apply the selected option (Send
LV_EVENT_VALUE_CHANGED
event and close the Drop down list)LV_KEY_ENTER With Encoder got the net digit. Jump to the first after the last.
Example¶
C¶
Simple Spinbox¶
code
#include "../../../lv_examples.h"
#include <stdio.h>
#if LV_USE_SPINBOX
static lv_obj_t * spinbox;
static void lv_spinbox_increment_event_cb(lv_obj_t * btn, lv_event_t e)
{
if(e == LV_EVENT_SHORT_CLICKED || e == LV_EVENT_LONG_PRESSED_REPEAT) {
lv_spinbox_increment(spinbox);
}
}
static void lv_spinbox_decrement_event_cb(lv_obj_t * btn, lv_event_t e)
{
if(e == LV_EVENT_SHORT_CLICKED || e == LV_EVENT_LONG_PRESSED_REPEAT) {
lv_spinbox_decrement(spinbox);
}
}
void lv_ex_spinbox_1(void)
{
spinbox = lv_spinbox_create(lv_scr_act(), NULL);
lv_spinbox_set_range(spinbox, -1000, 90000);
lv_spinbox_set_digit_format(spinbox, 5, 2);
lv_spinbox_step_prev(spinbox);
lv_obj_set_width(spinbox, 100);
lv_obj_align(spinbox, NULL, LV_ALIGN_CENTER, 0, 0);
lv_coord_t h = lv_obj_get_height(spinbox);
lv_obj_t * btn = lv_btn_create(lv_scr_act(), NULL);
lv_obj_set_size(btn, h, h);
lv_obj_align(btn, spinbox, LV_ALIGN_OUT_RIGHT_MID, 5, 0);
lv_theme_apply(btn, LV_THEME_SPINBOX_BTN);
lv_obj_set_style_local_value_str(btn, LV_BTN_PART_MAIN, LV_STATE_DEFAULT, LV_SYMBOL_PLUS);
lv_obj_set_event_cb(btn, lv_spinbox_increment_event_cb);
btn = lv_btn_create(lv_scr_act(), btn);
lv_obj_align(btn, spinbox, LV_ALIGN_OUT_LEFT_MID, -5, 0);
lv_obj_set_event_cb(btn, lv_spinbox_decrement_event_cb);
lv_obj_set_style_local_value_str(btn, LV_BTN_PART_MAIN, LV_STATE_DEFAULT, LV_SYMBOL_MINUS);
}
#endif
MicroPython¶
Click to try in the simulator!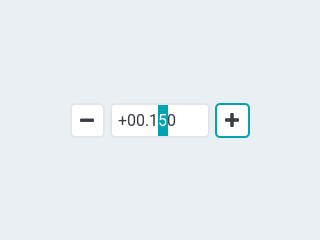
code
def increment_event_cb(source,evt):
if evt == lv.EVENT.SHORT_CLICKED or evt == lv.EVENT.LONG_PRESSED_REPEAT:
spinbox.increment()
def decrement_event_cb(source,evt):
if evt == lv.EVENT.SHORT_CLICKED or evt == lv.EVENT.LONG_PRESSED_REPEAT:
spinbox.decrement()
spinbox = lv.spinbox(lv.scr_act(),None)
spinbox.set_range(-1000,90000)
spinbox.set_digit_format(5,2)
spinbox.step_prev()
spinbox.set_width(100)
spinbox.align(None,lv.ALIGN.CENTER,0,0)
h = spinbox.get_height()
btn = lv.btn(lv.scr_act(),None)
btn.set_size(h,h)
btn.align(spinbox,lv.ALIGN.OUT_RIGHT_MID, 5, 0)
lv.theme_apply(btn,lv.THEME.SPINBOX_BTN)
btn.set_style_local_value_str(lv.btn.PART.MAIN, lv.STATE.DEFAULT, lv.SYMBOL.PLUS)
btn.set_event_cb(increment_event_cb)
btn = lv.btn(lv.scr_act(), btn)
btn.align(spinbox, lv.ALIGN.OUT_LEFT_MID, -5, 0)
btn.set_event_cb(decrement_event_cb)
btn.set_style_local_value_str(lv.btn.PART.MAIN, lv.STATE.DEFAULT, lv.SYMBOL.MINUS)
API¶
Typedefs
-
typedef uint8_t
lv_spinbox_part_t
¶
Enums
Functions
-
lv_obj_t *
lv_spinbox_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a spinbox objects
- Parameters
par -- pointer to an object, it will be the parent of the new spinbox
copy -- pointer to a spinbox object, if not NULL then the new object will be copied from it
- Returns
pointer to the created spinbox
-
void
lv_spinbox_set_rollover
(lv_obj_t *spinbox, bool b)¶ Set spinbox rollover function
- Parameters
spinbox -- pointer to spinbox
b -- true or false to enable or disable (default)
-
void
lv_spinbox_set_value
(lv_obj_t *spinbox, int32_t i)¶ Set spinbox value
- Parameters
spinbox -- pointer to spinbox
i -- value to be set
-
void
lv_spinbox_set_digit_format
(lv_obj_t *spinbox, uint8_t digit_count, uint8_t separator_position)¶ Set spinbox digit format (digit count and decimal format)
- Parameters
spinbox -- pointer to spinbox
digit_count -- number of digit excluding the decimal separator and the sign
separator_position -- number of digit before the decimal point. If 0, decimal point is not shown
-
void
lv_spinbox_set_step
(lv_obj_t *spinbox, uint32_t step)¶ Set spinbox step
- Parameters
spinbox -- pointer to spinbox
step -- steps on increment/decrement
-
void
lv_spinbox_set_range
(lv_obj_t *spinbox, int32_t range_min, int32_t range_max)¶ Set spinbox value range
- Parameters
spinbox -- pointer to spinbox
range_min -- maximum value, inclusive
range_max -- minimum value, inclusive
-
void
lv_spinbox_set_padding_left
(lv_obj_t *spinbox, uint8_t padding)¶ Set spinbox left padding in digits count (added between sign and first digit)
- Parameters
spinbox -- pointer to spinbox
cb -- Callback function called on value change event
-
bool
lv_spinbox_get_rollover
(lv_obj_t *spinbox)¶ Get spinbox rollover function status
- Parameters
spinbox -- pointer to spinbox
-
int32_t
lv_spinbox_get_value
(lv_obj_t *spinbox)¶ Get the spinbox numeral value (user has to convert to float according to its digit format)
- Parameters
spinbox -- pointer to spinbox
- Returns
value integer value of the spinbox
-
static inline int32_t
lv_spinbox_get_step
(lv_obj_t *spinbox)¶ Get the spinbox step value (user has to convert to float according to its digit format)
- Parameters
spinbox -- pointer to spinbox
- Returns
value integer step value of the spinbox
-
void
lv_spinbox_step_next
(lv_obj_t *spinbox)¶ Select next lower digit for edition by dividing the step by 10
- Parameters
spinbox -- pointer to spinbox
-
void
lv_spinbox_step_prev
(lv_obj_t *spinbox)¶ Select next higher digit for edition by multiplying the step by 10
- Parameters
spinbox -- pointer to spinbox
-
struct
lv_spinbox_ext_t
¶