Message box (lv_msgbox)¶
Overview¶
The Message boxes act as pop-ups. They are built from a background Container, a Label and a Button matrix for buttons.
The text will be broken into multiple lines automatically (has LV_LABEL_LONG_MODE_BREAK
) and the height will be set automatically to involve the text and the buttons (LV_FIT_TIGHT
fit vertically)-
Parts and Styles¶
The Message box's main part is called LV_MSGBOX_PART_MAIN
and it uses all the typical background style properties. Using padding will add space on the sides. pad_inner will add space between the text and the buttons.
The label style properties affect the style of text.
The buttons parts are the same as in case of Button matrix:
LV_MSGBOX_PART_BTN_BG
the background of the buttonsLV_MSGBOX_PART_BTN
the buttons
Usage¶
Set text¶
To set the text use the lv_msgbox_set_text(msgbox, "My text")
function. Not only the pointer of the text will be saved, so the the text can be in a local variable too.
Add buttons¶
To add buttons use the lv_msgbox_add_btns(msgbox, btn_str)
function. The button's text needs to be specified like const char * btn_str[] = {"Apply", "Close", ""}
.
For more information visit the Button matrix documentation.
The button matrix will be created only when lv_msgbox_add_btns()
is called for the first time.
Auto-close¶
With lv_msgbox_start_auto_close(mbox, delay)
the message box can be closed automatically after delay
milliseconds with an animation. The lv_mbox_stop_auto_close(mbox)
function stops a started auto close.
The duration of the close animation can be set by lv_mbox_set_anim_time(mbox, anim_time)
.
Events¶
Besides the Generic events the following Special events are sent by the Message boxes:
LV_EVENT_VALUE_CHANGED sent when the button is clicked. The event data is set to ID of the clicked button.
The Message box has a default event callback which closes itself when a button is clicked.
Learn more about Events.
##Keys
The following Keys are processed by the Buttons:
LV_KEY_RIGHT/DOWN Select the next button
LV_KEY_LEFT/TOP Select the previous button
LV_KEY_ENTER Clicks the selected button
Learn more about Keys.
Example¶
C¶
Simple Message box¶
code
#include "../../../lv_examples.h"
#include <stdio.h>
#if LV_USE_MSGBOX
static void event_handler(lv_obj_t * obj, lv_event_t event)
{
if(event == LV_EVENT_VALUE_CHANGED) {
printf("Button: %s\n", lv_msgbox_get_active_btn_text(obj));
}
}
void lv_ex_msgbox_1(void)
{
static const char * btns[] ={"Apply", "Close", ""};
lv_obj_t * mbox1 = lv_msgbox_create(lv_scr_act(), NULL);
lv_msgbox_set_text(mbox1, "A message box with two buttons.");
lv_msgbox_add_btns(mbox1, btns);
lv_obj_set_width(mbox1, 200);
lv_obj_set_event_cb(mbox1, event_handler);
lv_obj_align(mbox1, NULL, LV_ALIGN_CENTER, 0, 0); /*Align to the corner*/
}
#endif
Modal¶
code
#include "../../../lv_examples.h"
#if LV_USE_MSGBOX
static void mbox_event_cb(lv_obj_t *obj, lv_event_t evt);
static void btn_event_cb(lv_obj_t *btn, lv_event_t evt);
static void opa_anim(void * bg, lv_anim_value_t v);
static lv_obj_t *mbox, *info;
static lv_style_t style_modal;
static const char welcome_info[] = "Welcome to the modal message box demo!\n"
"Press the button to display a message box.";
static const char in_msg_info[] = "Notice that you cannot touch "
"the button again while the message box is open.";
void lv_ex_msgbox_2(void)
{
lv_style_init(&style_modal);
lv_style_set_bg_color(&style_modal, LV_STATE_DEFAULT, LV_COLOR_BLACK);
/* Create a button, then set its position and event callback */
lv_obj_t *btn = lv_btn_create(lv_scr_act(), NULL);
lv_obj_set_size(btn, 200, 60);
lv_obj_set_event_cb(btn, btn_event_cb);
lv_obj_align(btn, NULL, LV_ALIGN_IN_TOP_LEFT, 20, 20);
/* Create a label on the button */
lv_obj_t *label = lv_label_create(btn, NULL);
lv_label_set_text(label, "Display a message box!");
/* Create an informative label on the screen */
info = lv_label_create(lv_scr_act(), NULL);
lv_label_set_text(info, welcome_info);
lv_label_set_long_mode(info, LV_LABEL_LONG_BREAK); /* Make sure text will wrap */
lv_obj_set_width(info, LV_HOR_RES - 10);
lv_obj_align(info, NULL, LV_ALIGN_IN_BOTTOM_LEFT, 5, -5);
}
static void mbox_event_cb(lv_obj_t *obj, lv_event_t evt)
{
if(evt == LV_EVENT_DELETE && obj == mbox) {
/* Delete the parent modal background */
lv_obj_del_async(lv_obj_get_parent(mbox));
mbox = NULL; /* happens before object is actually deleted! */
lv_label_set_text(info, welcome_info);
} else if(evt == LV_EVENT_VALUE_CHANGED) {
/* A button was clicked */
lv_msgbox_start_auto_close(mbox, 0);
}
}
static void btn_event_cb(lv_obj_t *btn, lv_event_t evt)
{
if(evt == LV_EVENT_CLICKED) {
/* Create a full-screen background */
/* Create a base object for the modal background */
lv_obj_t *obj = lv_obj_create(lv_scr_act(), NULL);
lv_obj_reset_style_list(obj, LV_OBJ_PART_MAIN);
lv_obj_add_style(obj, LV_OBJ_PART_MAIN, &style_modal);
lv_obj_set_pos(obj, 0, 0);
lv_obj_set_size(obj, LV_HOR_RES, LV_VER_RES);
static const char * btns2[] = {"Ok", "Cancel", ""};
/* Create the message box as a child of the modal background */
mbox = lv_msgbox_create(obj, NULL);
lv_msgbox_add_btns(mbox, btns2);
lv_msgbox_set_text(mbox, "Hello world!");
lv_obj_align(mbox, NULL, LV_ALIGN_CENTER, 0, 0);
lv_obj_set_event_cb(mbox, mbox_event_cb);
/* Fade the message box in with an animation */
lv_anim_t a;
lv_anim_init(&a);
lv_anim_set_var(&a, obj);
lv_anim_set_time(&a, 500);
lv_anim_set_values(&a, LV_OPA_TRANSP, LV_OPA_50);
lv_anim_set_exec_cb(&a, (lv_anim_exec_xcb_t)opa_anim);
lv_anim_start(&a);
lv_label_set_text(info, in_msg_info);
lv_obj_align(info, NULL, LV_ALIGN_IN_BOTTOM_LEFT, 5, -5);
}
}
static void opa_anim(void * bg, lv_anim_value_t v)
{
lv_obj_set_style_local_bg_opa(bg, LV_OBJ_PART_MAIN, LV_STATE_DEFAULT, v);
}
#endif
MicroPython¶
Simple Message box¶
Click to try in the simulator!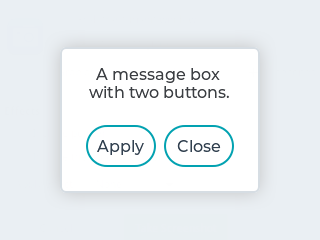
code
def event_handler(obj, event):
if event == lv.EVENT.VALUE_CHANGED:
print("Button: %s" % obj.get_active_btn_text())
btns = ["Apply", "Close", ""]
mbox1 = lv.msgbox(lv.scr_act())
mbox1.set_text("A message box with two buttons.");
mbox1.add_btns(btns)
mbox1.set_width(200)
mbox1.set_event_cb(event_handler)
mbox1.align(None, lv.ALIGN.CENTER, 0, 0) # Align to the corner
Modal¶
Click to try in the simulator!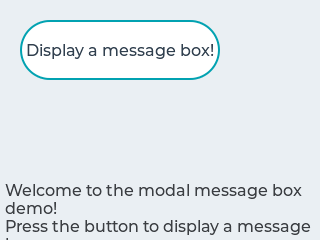
code
from lv_colors import lv_colors
welcome_info = "Welcome to the modal message box demo!\nPress the button to display a message box."
in_msg_info = "Notice that you cannot touch the button again while the message box is open."
LV_HOR_RES=240
LV_VER_RES=240
def opa_anim(mbox,v):
bg = lv.obj.__cast__(mbox)
# print("v: ",v)
mbox.get_parent().set_style_local_bg_opa(lv.obj.PART.MAIN, lv.STATE.DEFAULT, v)
def mbox_event_cb(obj, evt):
if evt == lv.EVENT.DELETE:
# Delete the parent modal background
lv.obj.del_async(obj.get_parent())
info.set_text(welcome_info)
info.align(None, lv.ALIGN.IN_BOTTOM_LEFT, 5, -15)
elif evt == lv.EVENT.VALUE_CHANGED:
# a button was clicked
obj.start_auto_close(0)
def btn_event_cb(btn,evt):
if evt == lv.EVENT.CLICKED:
# Create a full-screen background
#Create a base object for the modal background
obj = lv.obj(lv.scr_act(), None)
obj.reset_style_list(lv.obj.PART.MAIN)
obj.add_style(lv.obj.PART.MAIN, style_modal)
obj.set_pos(0, 0)
obj.set_size(LV_HOR_RES, LV_VER_RES)
#obj.set_style_local_bg_opa(lv.obj.PART.MAIN, lv.STATE.DEFAULT, lv.OPA._80)
btns2 = ["Ok", "Cancel", ""]
# Create the message box as a child of the modal background
mbox = lv.msgbox(obj, None)
mbox.add_btns(btns2);
mbox.set_text("Hello world!")
mbox.align(None, lv.ALIGN.CENTER, 0, 0)
mbox.set_event_cb(mbox_event_cb)
# Fade the message box in with an animation
a=lv.anim_t()
a.init()
a.set_var(obj)
a.set_time(500)
a.set_values(lv.OPA.TRANSP, lv.OPA._70)
a.set_custom_exec_cb(lambda a, val: opa_anim(mbox,val))
lv.anim_t.start(a)
info.set_text(in_msg_info)
info.align(None, lv.ALIGN.IN_BOTTOM_LEFT, 5, -5)
style_modal = lv.style_t()
style_modal.init()
style_modal.set_bg_color(lv.STATE.DEFAULT, lv_colors.BLACK)
# Create a button, then set its position and event callback */
btn = lv.btn(lv.scr_act(), None)
btn.set_size(200, 60)
btn.set_event_cb(btn_event_cb)
btn.align(None, lv.ALIGN.IN_TOP_LEFT, 20, 20)
# Create a label on the button
label = lv.label(btn,None)
label.set_text("Display a message box!")
# Create an informative label on the screen
info = lv.label(lv.scr_act(), None)
info.set_text(welcome_info)
info.set_long_mode(lv.label.LONG.BREAK) # Make sure text will wrap
info.set_width(LV_HOR_RES - 10)
info.align(None, lv.ALIGN.IN_BOTTOM_LEFT, 5, -15)
API¶
Typedefs
-
typedef uint8_t
lv_msgbox_style_t
¶
Enums
Functions
-
lv_obj_t *
lv_msgbox_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a message box objects
- Parameters
par -- pointer to an object, it will be the parent of the new message box
copy -- pointer to a message box object, if not NULL then the new object will be copied from it
- Returns
pointer to the created message box
-
void
lv_msgbox_add_btns
(lv_obj_t *mbox, const char *btn_mapaction[])¶ Add button to the message box
- Parameters
mbox -- pointer to message box object
btn_map -- button descriptor (button matrix map). E.g. a const char *txt[] = {"ok", "close", ""} (Can not be local variable)
-
void
lv_msgbox_set_text
(lv_obj_t *mbox, const char *txt)¶ Set the text of the message box
- Parameters
mbox -- pointer to a message box
txt -- a '\0' terminated character string which will be the message box text
-
void
lv_msgbox_set_text_fmt
(lv_obj_t *mbox, const char *fmt, ...)¶ Set a formatted text for the message box
- Parameters
mbox -- pointer to a message box
fmt --
printf
-like format
-
void
lv_msgbox_set_anim_time
(lv_obj_t *mbox, uint16_t anim_time)¶ Set animation duration
- Parameters
mbox -- pointer to a message box object
anim_time -- animation length in milliseconds (0: no animation)
-
void
lv_msgbox_start_auto_close
(lv_obj_t *mbox, uint16_t delay)¶ Automatically delete the message box after a given time
- Parameters
mbox -- pointer to a message box object
delay -- a time (in milliseconds) to wait before delete the message box
-
void
lv_msgbox_stop_auto_close
(lv_obj_t *mbox)¶ Stop the auto. closing of message box
- Parameters
mbox -- pointer to a message box object
-
void
lv_msgbox_set_recolor
(lv_obj_t *mbox, bool en)¶ Set whether recoloring is enabled. Must be called after
lv_msgbox_add_btns
.- Parameters
mbox -- pointer to message box object
en -- whether recoloring is enabled
-
const char *
lv_msgbox_get_text
(const lv_obj_t *mbox)¶ Get the text of the message box
- Parameters
mbox -- pointer to a message box object
- Returns
pointer to the text of the message box
-
uint16_t
lv_msgbox_get_active_btn
(lv_obj_t *mbox)¶ Get the index of the lastly "activated" button by the user (pressed, released etc) Useful in the
event_cb
.- Parameters
mbox -- pointer to message box object
- Returns
index of the last released button (LV_BTNMATRIX_BTN_NONE: if unset)
-
const char *
lv_msgbox_get_active_btn_text
(lv_obj_t *mbox)¶ Get the text of the lastly "activated" button by the user (pressed, released etc) Useful in the
event_cb
.- Parameters
mbox -- pointer to message box object
- Returns
text of the last released button (NULL: if unset)
-
uint16_t
lv_msgbox_get_anim_time
(const lv_obj_t *mbox)¶ Get the animation duration (close animation time)
- Parameters
mbox -- pointer to a message box object
- Returns
animation length in milliseconds (0: no animation)
-
struct
lv_msgbox_ext_t
¶