Object mask (lv_objmask)¶
Overview¶
The Object mask is capable of add some mask to drawings when its children is drawn.
Parts and Styles¶
The Object mask has only a main part called LV_OBJMASK_PART_BG
and it uses the typical background style properties.
Usage¶
Adding mask¶
Before adding a mask to the Object mask the mask should be initialized:
lv_draw_mask_<type>_param_t mask_param;
lv_draw_mask_<type>_init(&mask_param, ...);
lv_objmask_mask_t * mask_p = lv_objmask_add_mask(objmask, &mask_param);
Lvgl supports the following mask types:
line clip the pixels on the top/bottom left/right of a line. Can be initialized from two points or a point and an angle:
angle keep the pixels only between a given start and end angle
radius keep the pixel only inside a rectangle which can have radius (can for a circle too). Can be inverted to keep the pixel outside of the rectangle.
fade fade vertically (change the pixels opacity according to their y position)
map use an alpha mask (a byte array) to describe the pixels opacity.
The coordinates in the mask are relative to the Object. That is if the object moves the masks move with it.
For the details of the mask init function see the API documentation below.
Update mask¶
AN existing mask can be updated with lv_objmask_update_mask(objmask, mask_p, new_param)
, where mask_p
is return value of lv_objmask_add_mask
.
Remove mask¶
A mask can be removed with lv_objmask_remove_mask(objmask, mask_p)
Example¶
C¶
Several object masks¶
code
#include "../../../lv_examples.h"
#if LV_USE_OBJMASK
void lv_ex_objmask_1(void)
{
/*Set a very visible color for the screen to clearly see what happens*/
lv_obj_set_style_local_bg_color(lv_scr_act(), LV_OBJ_PART_MAIN, LV_STATE_DEFAULT, lv_color_hex3(0xf33));
lv_obj_t * om = lv_objmask_create(lv_scr_act(), NULL);
lv_obj_set_size(om, 200, 200);
lv_obj_align(om, NULL, LV_ALIGN_CENTER, 0, 0);
lv_obj_t * label = lv_label_create(om, NULL);
lv_label_set_long_mode(label, LV_LABEL_LONG_BREAK);
lv_label_set_align(label, LV_LABEL_ALIGN_CENTER);
lv_obj_set_width(label, 180);
lv_label_set_text(label, "This label will be masked out. See how it works.");
lv_obj_align(label, NULL, LV_ALIGN_IN_TOP_MID, 0, 20);
lv_obj_t * cont = lv_cont_create(om, NULL);
lv_obj_set_size(cont, 180, 100);
lv_obj_set_drag(cont, true);
lv_obj_align(cont, NULL, LV_ALIGN_IN_BOTTOM_MID, 0, -10);
lv_obj_t * btn = lv_btn_create(cont, NULL);
lv_obj_align(btn, NULL, LV_ALIGN_CENTER, 0, 0);
lv_obj_set_style_local_value_str(btn, LV_BTN_PART_MAIN, LV_STATE_DEFAULT, "Button");
uint32_t t;
lv_refr_now(NULL);
t = lv_tick_get();
while(lv_tick_elaps(t) < 1000);
lv_area_t a;
lv_draw_mask_radius_param_t r1;
a.x1 = 10;
a.y1 = 10;
a.x2 = 190;
a.y2 = 190;
lv_draw_mask_radius_init(&r1, &a, LV_RADIUS_CIRCLE, false);
lv_objmask_add_mask(om, &r1);
lv_refr_now(NULL);
t = lv_tick_get();
while(lv_tick_elaps(t) < 1000);
a.x1 = 100;
a.y1 = 100;
a.x2 = 150;
a.y2 = 150;
lv_draw_mask_radius_init(&r1, &a, LV_RADIUS_CIRCLE, true);
lv_objmask_add_mask(om, &r1);
lv_refr_now(NULL);
t = lv_tick_get();
while(lv_tick_elaps(t) < 1000);
lv_draw_mask_line_param_t l1;
lv_draw_mask_line_points_init(&l1, 0, 0, 100, 200, LV_DRAW_MASK_LINE_SIDE_TOP);
lv_objmask_add_mask(om, &l1);
lv_refr_now(NULL);
t = lv_tick_get();
while(lv_tick_elaps(t) < 1000);
lv_draw_mask_fade_param_t f1;
a.x1 = 100;
a.y1 = 0;
a.x2 = 200;
a.y2 = 200;
lv_draw_mask_fade_init(&f1, &a, LV_OPA_TRANSP, 0, LV_OPA_COVER, 150);
lv_objmask_add_mask(om, &f1);
}
#endif
Text mask¶
code
#include "../../../lv_examples.h"
#if LV_USE_OBJMASK
#define MASK_WIDTH 100
#define MASK_HEIGHT 50
void lv_ex_objmask_2(void)
{
/* Create the mask of a text by drawing it to a canvas*/
static lv_opa_t mask_map[MASK_WIDTH * MASK_HEIGHT];
/*Create a "8 bit alpha" canvas and clear it*/
lv_obj_t * canvas = lv_canvas_create(lv_scr_act(), NULL);
lv_canvas_set_buffer(canvas, mask_map, MASK_WIDTH, MASK_HEIGHT, LV_IMG_CF_ALPHA_8BIT);
lv_canvas_fill_bg(canvas, LV_COLOR_BLACK, LV_OPA_TRANSP);
/*Draw a label to the canvas. The result "image" will be used as mask*/
lv_draw_label_dsc_t label_dsc;
lv_draw_label_dsc_init(&label_dsc);
label_dsc.color = LV_COLOR_WHITE;
lv_canvas_draw_text(canvas, 5, 5, MASK_WIDTH, &label_dsc, "Text with gradient", LV_LABEL_ALIGN_CENTER);
/*The mask is reads the canvas is not required anymore*/
lv_obj_del(canvas);
/*Create an object mask which will use the created mask*/
lv_obj_t * om = lv_objmask_create(lv_scr_act(), NULL);
lv_obj_set_size(om, MASK_WIDTH, MASK_HEIGHT);
lv_obj_align(om, NULL, LV_ALIGN_CENTER, 0, 0);
/*Add the created mask map to the object mask*/
lv_draw_mask_map_param_t m;
lv_area_t a;
a.x1 = 0;
a.y1 = 0;
a.x2 = MASK_WIDTH - 1;
a.y2 = MASK_HEIGHT - 1;
lv_draw_mask_map_init(&m, &a, mask_map);
lv_objmask_add_mask(om, &m);
/*Create a style with gradient*/
static lv_style_t style_bg;
lv_style_init(&style_bg);
lv_style_set_bg_opa(&style_bg, LV_STATE_DEFAULT, LV_OPA_COVER);
lv_style_set_bg_color(&style_bg, LV_STATE_DEFAULT, LV_COLOR_RED);
lv_style_set_bg_grad_color(&style_bg, LV_STATE_DEFAULT, LV_COLOR_BLUE);
lv_style_set_bg_grad_dir(&style_bg, LV_STATE_DEFAULT, LV_GRAD_DIR_HOR);
/* Create and object with the gradient style on the object mask.
* The text will be masked from the gradient*/
lv_obj_t * bg = lv_obj_create(om, NULL);
lv_obj_reset_style_list(bg, LV_OBJ_PART_MAIN);
lv_obj_add_style(bg, LV_OBJ_PART_MAIN, &style_bg);
lv_obj_set_size(bg, MASK_WIDTH, MASK_HEIGHT);
}
#endif
MicroPython¶
Several object masks¶
Click to try in the simulator!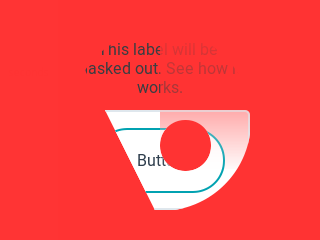
code
# Set a very visible color for the screen to clearly see what happens
lv.scr_act().set_style_local_bg_color(lv.obj.PART.MAIN, lv.STATE.DEFAULT, lv.color_hex3(0xf33))
om = lv.objmask(lv.scr_act(), None)
om.set_size(200, 200)
om.align(None, lv.ALIGN.CENTER, 0, 0)
label = lv.label(om, None)
label.set_long_mode(lv.label.LONG.BREAK)
label.set_align(lv.label.ALIGN.CENTER)
label.set_width(180)
label.set_text("This label will be masked out. See how it works.")
label.align(None, lv.ALIGN.IN_TOP_MID, 0, 20)
cont = lv.cont(om, None)
cont.set_size(180, 100)
cont.set_drag(True)
cont.align(None, lv.ALIGN.IN_BOTTOM_MID, 0, -10)
btn = lv.btn(cont, None)
btn.align(None, lv.ALIGN.CENTER, 0, 0)
btn.set_style_local_value_str(lv.btn.PART.MAIN, lv.STATE.DEFAULT, "Button")
lv.refr_now(None);
t = lv.tick_get();
while lv.tick_elaps(t) < 1000:
pass
a=lv.area_t()
r1=lv.draw_mask_radius_param_t()
a.x1 = 10
a.y1 = 10
a.x2 = 190
a.y2 = 190
r1.init(a, lv.RADIUS.CIRCLE, False)
om.add_mask(r1)
lv.refr_now(None);
t = lv.tick_get();
while lv.tick_elaps(t) < 1000:
pass
a.x1 = 100
a.y1 = 100
a.x2 = 150
a.y2 = 150
r1.init(a, lv.RADIUS.CIRCLE, True)
om.add_mask(r1)
lv.refr_now(None)
t = lv.tick_get()
while lv.tick_elaps(t) < 1000:
pass
l1=lv.draw_mask_line_param_t()
l1.points_init(0, 0, 100, 200, lv.DRAW_MASK_LINE_SIDE.TOP)
om.add_mask(l1)
lv.refr_now(None)
t = lv.tick_get()
while lv.tick_elaps(t) < 1000:
pass
f1= lv.draw_mask_fade_param_t()
a.x1 = 100
a.y1 = 0
a.x2 = 200
a.y2 = 200
f1.init(a, lv.OPA.TRANSP, 0, lv.OPA.COVER, 150)
om.add_mask(f1)
Text mask¶
Click to try in the simulator!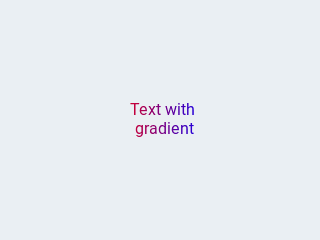
code
from lv_colors import lv_colors
MASK_WIDTH=100
MASK_HEIGHT=50
# Create the mask of a text by drawing it to a canvas
mask_map = bytearray (MASK_WIDTH * MASK_HEIGHT *4)
# Create a "8 bit alpha" canvas and clear it
canvas = lv.canvas(lv.scr_act(), None)
canvas.set_buffer(mask_map, MASK_WIDTH, MASK_HEIGHT, lv.img.CF.ALPHA_8BIT)
canvas.fill_bg(lv_colors.BLACK, lv.OPA.TRANSP)
# Draw a label to the canvas. The result "image" will be used as mask
label_dsc = lv.draw_label_dsc_t()
label_dsc.init()
label_dsc.color = lv_colors.WHITE
canvas.draw_text(5, 5, MASK_WIDTH, label_dsc, "Text with gradient", lv.label.ALIGN.CENTER)
# The mask is read, the canvas is not required anymore
canvas.delete()
# Create an object mask which will use the created mask
om = lv.objmask(lv.scr_act(), None)
om.set_size(MASK_WIDTH, MASK_HEIGHT)
om.align(None, lv.ALIGN.CENTER, 0, 0)
# Add the created mask map to the object mask
m = lv.draw_mask_map_param_t()
a=lv.area_t()
a.x1 = 0
a.y1 = 0
a.x2 = MASK_WIDTH - 1
a.y2 = MASK_HEIGHT - 1
m.init(a,mask_map)
om.add_mask(m)
# Create a style with gradient
style_bg= lv.style_t()
style_bg.init()
style_bg.set_bg_opa(lv.STATE.DEFAULT, lv.OPA.COVER)
style_bg.set_bg_color(lv.STATE.DEFAULT, lv_colors.RED)
style_bg.set_bg_grad_color(lv.STATE.DEFAULT, lv_colors.BLUE)
style_bg.set_bg_grad_dir(lv.STATE.DEFAULT, lv.GRAD_DIR.HOR)
# Create and object with the gradient style on the object mask.
# The text will be masked from the gradient
bg = lv.obj(om, None)
bg.reset_style_list(lv.obj.PART.MAIN)
bg.add_style(lv.obj.PART.MAIN, style_bg)
bg.set_size(MASK_WIDTH, MASK_HEIGHT)
API¶
Typedefs
-
typedef uint8_t
lv_objmask_part_t
¶
Functions
-
lv_obj_t *
lv_objmask_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a object mask objects
- Parameters
par -- pointer to an object, it will be the parent of the new object mask
copy -- pointer to a object mask object, if not NULL then the new object will be copied from it
- Returns
pointer to the created object mask
-
lv_objmask_mask_t *
lv_objmask_add_mask
(lv_obj_t *objmask, void *param)¶ Add a mask
- Parameters
objmask -- pointer to an Object mask object
param -- an initialized mask parameter
- Returns
pointer to the added mask
-
void
lv_objmask_update_mask
(lv_obj_t *objmask, lv_objmask_mask_t *mask, void *param)¶ Update an already created mask
- Parameters
objmask -- pointer to an Object mask object
mask -- pointer to created mask (returned by
lv_objmask_add_mask
)param -- an initialized mask parameter (initialized by
lv_draw_mask_line/angle/.../_init
)
-
void
lv_objmask_remove_mask
(lv_obj_t *objmask, lv_objmask_mask_t *mask)¶ Remove a mask
- Parameters
objmask -- pointer to an Object mask object
mask -- pointer to created mask (returned by
lv_objmask_add_mask
) IfNULL
passed all masks will be deleted.
-
struct
lv_objmask_ext_t
¶
Typedefs
-
typedef uint8_t
lv_draw_mask_res_t
¶
-
typedef uint8_t
lv_draw_mask_type_t
¶
-
typedef lv_draw_mask_res_t (*
lv_draw_mask_xcb_t
)(lv_opa_t *mask_buf, lv_coord_t abs_x, lv_coord_t abs_y, lv_coord_t len, void *p)¶ A common callback type for every mask type. Used internally by the library.
-
typedef uint8_t
lv_draw_mask_line_side_t
¶
-
typedef struct _lv_draw_mask_map_param_t
lv_draw_mask_map_param_t
¶
-
typedef _lv_draw_mask_saved_t
_lv_draw_mask_saved_arr_t
[_LV_MASK_MAX_NUM
]¶
Enums
-
enum [anonymous]¶
Values:
-
enumerator
LV_DRAW_MASK_RES_TRANSP
¶
-
enumerator
LV_DRAW_MASK_RES_FULL_COVER
¶
-
enumerator
LV_DRAW_MASK_RES_CHANGED
¶
-
enumerator
LV_DRAW_MASK_RES_UNKNOWN
¶
-
enumerator
Functions
-
int16_t
lv_draw_mask_add
(void *param, void *custom_id)¶ Add a draw mask. Everything drawn after it (until removing the mask) will be affected by the mask.
- Parameters
param -- an initialized mask parameter. Only the pointer is saved.
custom_id -- a custom pointer to identify the mask. Used in
lv_draw_mask_remove_custom
.
- Returns
the an integer, the ID of the mask. Can be used in
lv_draw_mask_remove_id
.
-
void *
lv_draw_mask_remove_id
(int16_t id)¶ Remove a mask with a given ID
- Parameters
id -- the ID of the mask. Returned by
lv_draw_mask_add
- Returns
the parameter of the removed mask. If more masks have
custom_id
ID then the last mask's parameter will be returned
-
void *
lv_draw_mask_remove_custom
(void *custom_id)¶ Remove all mask with a given custom ID
- Parameters
custom_id -- a pointer used in
lv_draw_mask_add
- Returns
return the parameter of the removed mask. If more masks have
custom_id
ID then the last mask's parameter will be returned
-
void
lv_draw_mask_line_points_init
(lv_draw_mask_line_param_t *param, lv_coord_t p1x, lv_coord_t p1y, lv_coord_t p2x, lv_coord_t p2y, lv_draw_mask_line_side_t side)¶ Initialize a line mask from two points.
- Parameters
param -- pointer to a
lv_draw_mask_param_t
to initializep1x -- X coordinate of the first point of the line
p1y -- Y coordinate of the first point of the line
p2x -- X coordinate of the second point of the line
p2y -- y coordinate of the second point of the line
side -- and element of
lv_draw_mask_line_side_t
to describe which side to keep. WithLV_DRAW_MASK_LINE_SIDE_LEFT/RIGHT
and horizontal line all pixels are kept WithLV_DRAW_MASK_LINE_SIDE_TOP/BOTTOM
and vertical line all pixels are kept
-
void
lv_draw_mask_line_angle_init
(lv_draw_mask_line_param_t *param, lv_coord_t p1x, lv_coord_t py, int16_t angle, lv_draw_mask_line_side_t side)¶ Initialize a line mask from a point and an angle.
- Parameters
param -- pointer to a
lv_draw_mask_param_t
to initializepx -- X coordinate of a point of the line
py -- X coordinate of a point of the line
angle -- right 0 deg, bottom: 90
side -- and element of
lv_draw_mask_line_side_t
to describe which side to keep. WithLV_DRAW_MASK_LINE_SIDE_LEFT/RIGHT
and horizontal line all pixels are kept WithLV_DRAW_MASK_LINE_SIDE_TOP/BOTTOM
and vertical line all pixels are kept
-
void
lv_draw_mask_angle_init
(lv_draw_mask_angle_param_t *param, lv_coord_t vertex_x, lv_coord_t vertex_y, lv_coord_t start_angle, lv_coord_t end_angle)¶ Initialize an angle mask.
- Parameters
param -- pointer to a
lv_draw_mask_param_t
to initializevertex_x -- X coordinate of the angle vertex (absolute coordinates)
vertex_y -- Y coordinate of the angle vertex (absolute coordinates)
start_angle -- start angle in degrees. 0 deg on the right, 90 deg, on the bottom
end_angle -- end angle
-
void
lv_draw_mask_radius_init
(lv_draw_mask_radius_param_t *param, const lv_area_t *rect, lv_coord_t radius, bool inv)¶ Initialize a fade mask.
- Parameters
param -- param pointer to a
lv_draw_mask_param_t
to initializerect -- coordinates of the rectangle to affect (absolute coordinates)
radius -- radius of the rectangle
inv -- true: keep the pixels inside the rectangle; keep the pixels outside of the rectangle
-
void
lv_draw_mask_fade_init
(lv_draw_mask_fade_param_t *param, const lv_area_t *coords, lv_opa_t opa_top, lv_coord_t y_top, lv_opa_t opa_bottom, lv_coord_t y_bottom)¶ Initialize a fade mask.
- Parameters
param -- pointer to a
lv_draw_mask_param_t
to initializecoords -- coordinates of the area to affect (absolute coordinates)
opa_top -- opacity on the top
y_top -- at which coordinate start to change to opacity to
opa_bottom
opa_bottom -- opacity at the bottom
y_bottom -- at which coordinate reach
opa_bottom
.
-
void
lv_draw_mask_map_init
(lv_draw_mask_map_param_t *param, const lv_area_t *coords, const lv_opa_t *map)¶ Initialize a map mask.
- Parameters
param -- pointer to a
lv_draw_mask_param_t
to initializecoords -- coordinates of the map (absolute coordinates)
map -- array of bytes with the mask values
-
struct
lv_draw_mask_common_dsc_t
¶
-
struct
lv_draw_mask_line_param_t
¶
-
struct
lv_draw_mask_angle_param_t
¶ Public Members
-
lv_point_t
vertex_p
¶
-
lv_coord_t
start_angle
¶
-
lv_coord_t
end_angle
¶
-
struct lv_draw_mask_angle_param_t::[anonymous]
cfg
¶
-
lv_draw_mask_line_param_t
start_line
¶
-
lv_draw_mask_line_param_t
end_line
¶
-
uint16_t
delta_deg
¶
-
lv_point_t
-
struct
lv_draw_mask_radius_param_t
¶
-
struct
lv_draw_mask_fade_param_t
¶
-
struct
_lv_draw_mask_map_param_t
¶ Public Members
-
lv_area_t
coords
¶
-
const lv_opa_t *
map
¶
-
struct _lv_draw_mask_map_param_t::[anonymous]
cfg
¶
-
lv_area_t
-
struct
_lv_draw_mask_saved_t
¶