Tabview (lv_tabview)¶
Overview¶
The Tab view object can be used to organize content in tabs.
Parts and Styles¶
The Tab view object has several parts. The main is LV_TABVIEW_PART_BG
. It a rectangle-like container which holds the other parts of the Tab view.
On the background 2 important real parts are created:
LV_TABVIEW_PART_BG_SCRL
: it's the scrollable part of Page. It holds the content of the tabs next to each other. The background of the Page is always transparent and can't be accessed externally.LV_TABVIEW_PART_TAB_BG
: The tab buttons which is a Button matrix. Clicking on a button will scrollLV_TABVIEW_PART_BG_SCRL
to the related tab's content. The tab buttons can be accessed viaLV_TABVIEW_PART_TAB_BTN
. When tabs are selected, the buttons are in the checked state, and can be styled usingLV_STATE_CHECKED
. The height of the tab's button matrix is calculated from the font height plus padding of the background's and the button's style.
All the listed parts supports the typical background style properties and padding.
LV_TABVIEW_PART_TAB_BG
has an additional real part, an indicator, called LV_TABVIEW_PART_INDIC
.
It's a thin rectangle-like object under the currently selected tab. When the tab view is animated to an other tab the indicator will be animated too.
It can be styles using the typical background style properties. The size style property will set the its thickness.
When a new tab is added a Page is create for them on LV_TABVIEW_PART_BG_SCRL
and a new button is added to LV_TABVIEW_PART_TAB_BG
Button matrix.
The created Pages can be used as normal Pages and they have the usual Page parts.
Usage¶
Adding tab¶
New tabs can be added with lv_tabview_add_tab(tabview, "Tab name")
. It will return with a pointer to a Page object where the tab's content can be created.
Change tab¶
To select a new tab you can:
Click on it on the Button matrix part
Slide
Use
lv_tabview_set_tab_act(tabview, id, LV_ANIM_ON/OFF)
function
Change tab's name¶
To change the name (shown text of the underlying button matrix) of tab id
during runtime the function lv_tabview_set_tab_name(tabview, id, name)
can be used.
Tab button's position¶
By default, the tab selector buttons are placed on the top of the Tab view. It can be changed with lv_tabview_set_btns_pos(tabview, LV_TABVIEW_TAB_POS_TOP/BOTTOM/LEFT/RIGHT/NONE)
LV_TABVIEW_TAB_POS_NONE
will hide the tabs.
Note that, you can't change the tab position from top or bottom to left or right when tabs are already added.
Animation time¶
The animation time is adjusted by lv_tabview_set_anim_time(tabview, anim_time_ms)
. It is used when the new tab is loaded.
Scroll propagation¶
As the tabs' content object is a Page it can receive scroll propagation from an other Page-like object.
For example, if a text area is created on the tab's content and that Text area is scrolled but it reached the end the scroll can be propagated to the content Page.
It can be enabled with lv_page/textarea_set_scroll_propagation(obj, true)
.
By default the tab's content Pages have enabled scroll propagation, therefore when they are scrolled horizontally the scroll is propagated to LV_TABVIEW_PART_BG_SCRL
and this way the Pages will be scrolled.
The manual sliding can be disabled with lv_page_set_scroll_propagation(tab_page, false)
.
Events¶
Besides the Generic events the following Special events are sent by the Slider:
LV_EVENT_VALUE_CHANGED Sent when a new tab is selected by sliding or clicking the tab button
Learn more about Events.
Keys¶
The following Keys are processed by the Tabview:
LV_KEY_RIGHT/LEFT Select a tab
LV_KEY_ENTER Change to the selected tab
Learn more about Keys.
Example¶
C¶
Simple Tabview¶
code
#include "../../../lv_examples.h"
#if LV_USE_TABVIEW
void lv_ex_tabview_1(void)
{
/*Create a Tab view object*/
lv_obj_t *tabview;
tabview = lv_tabview_create(lv_scr_act(), NULL);
/*Add 3 tabs (the tabs are page (lv_page) and can be scrolled*/
lv_obj_t *tab1 = lv_tabview_add_tab(tabview, "Tab 1");
lv_obj_t *tab2 = lv_tabview_add_tab(tabview, "Tab 2");
lv_obj_t *tab3 = lv_tabview_add_tab(tabview, "Tab 3");
/*Add content to the tabs*/
lv_obj_t * label = lv_label_create(tab1, NULL);
lv_label_set_text(label, "This the first tab\n\n"
"If the content\n"
"of a tab\n"
"become too long\n"
"the it \n"
"automatically\n"
"become\n"
"scrollable.");
label = lv_label_create(tab2, NULL);
lv_label_set_text(label, "Second tab");
label = lv_label_create(tab3, NULL);
lv_label_set_text(label, "Third tab");
}
#endif
MicroPython¶
Simple Tabview¶
Click to try in the simulator!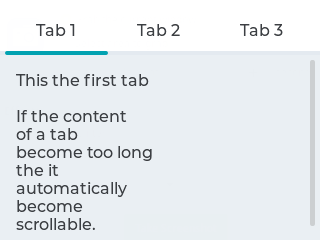
code
# Create a Tab view object
tabview = lv.tabview(lv.scr_act(), None)
# Add 3 tabs (the tabs are page (lv_page) and can be scrolled
tab1=tabview.add_tab("Tab 1")
tab2=tabview.add_tab("Tab 2")
tab3=tabview.add_tab("Tab 3")
# Add content to the tabs
label = lv.label(tab1,None)
label.set_text('''
This the first tab\n
If the content
of a tab
become too long
then it
automatically
becomes
scrollable.
''')
label = lv.label(tab2,None)
label.set_text("Second tab")
label = lv.label(tab3, None)
label.set_text("Third tab");
API¶
Enums
Functions
-
lv_obj_t *
lv_tabview_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a Tab view object
- Parameters
par -- pointer to an object, it will be the parent of the new tab
copy -- pointer to a tab object, if not NULL then the new object will be copied from it
- Returns
pointer to the created tab
-
lv_obj_t *
lv_tabview_add_tab
(lv_obj_t *tabview, const char *name)¶ Add a new tab with the given name
- Parameters
tabview -- pointer to Tab view object where to ass the new tab
name -- the text on the tab button
- Returns
pointer to the created page object (lv_page). You can create your content here
-
void
lv_tabview_clean_tab
(lv_obj_t *tab)¶ Delete all children of a tab created by
lv_tabview_add_tab
.- Parameters
tab -- pointer to a tab
-
void
lv_tabview_set_tab_act
(lv_obj_t *tabview, uint16_t id, lv_anim_enable_t anim)¶ Set a new tab
- Parameters
tabview -- pointer to Tab view object
id -- index of a tab to load
anim -- LV_ANIM_ON: set the value with an animation; LV_ANIM_OFF: change the value immediately
-
void
lv_tabview_set_tab_name
(lv_obj_t *tabview, uint16_t id, char *name)¶ Set the name of a tab.
- Parameters
tabview -- pointer to Tab view object
id -- index of the tab the name should be set
name -- new tab name
-
void
lv_tabview_set_anim_time
(lv_obj_t *tabview, uint16_t anim_time)¶ Set the animation time of tab view when a new tab is loaded
- Parameters
tabview -- pointer to Tab view object
anim_time -- time of animation in milliseconds
-
void
lv_tabview_set_btns_pos
(lv_obj_t *tabview, lv_tabview_btns_pos_t btns_pos)¶ Set the position of tab select buttons
- Parameters
tabview -- pointer to a tab view object
btns_pos -- which button position
-
uint16_t
lv_tabview_get_tab_act
(const lv_obj_t *tabview)¶ Get the index of the currently active tab
- Parameters
tabview -- pointer to Tab view object
- Returns
the active tab index
-
uint16_t
lv_tabview_get_tab_count
(const lv_obj_t *tabview)¶ Get the number of tabs
- Parameters
tabview -- pointer to Tab view object
- Returns
tab count
-
lv_obj_t *
lv_tabview_get_tab
(const lv_obj_t *tabview, uint16_t id)¶ Get the page (content area) of a tab
- Parameters
tabview -- pointer to Tab view object
id -- index of the tab (>= 0)
- Returns
pointer to page (lv_page) object
-
uint16_t
lv_tabview_get_anim_time
(const lv_obj_t *tabview)¶ Get the animation time of tab view when a new tab is loaded
- Parameters
tabview -- pointer to Tab view object
- Returns
time of animation in milliseconds
-
lv_tabview_btns_pos_t
lv_tabview_get_btns_pos
(const lv_obj_t *tabview)¶ Get position of tab select buttons
- Parameters
tabview -- pointer to a ab view object
-
struct
lv_tabview_ext_t
¶