Drop-down list (lv_dropdown)¶
Overview¶
The drop-down list allows the user to select one value from a list.
The drop-down list is closed by default and displays a single value or a predefined text. When activated (by click on the drop-down list), a list is created from which the user may select one option. When the user selects a new value, the list is deleted.
Parts and Styles¶
The drop-down list's main part is called LV_DROPDOWN_PART_MAIN
which is a simple lv_obj object.
It uses all the typical background properties. Pressed, Focused, Edited etc. stiles are also applied as usual.
The list, which is created when the main object is clicked, is an Page.
Its background part can be referenced with LV_DROPDOWN_PART_LIST
and uses all the typical background properties for the rectangle itself and text properties for the options.
To adjust the space between the options use the text_line_space style property.
Padding values can be used to make some space on the edges.
The scrollable part of the page is hidden and its styles are always empty (so transparent with no padding).
The scrollbar can be referenced with LV_DROPDOWN_PART_SCRLBAR
and uses all the typical background properties.
The selected option can be referenced with LV_DROPDOWN_PART_SELECTED
and uses all the typical background properties.
It will used in its default state to draw a rectangle on the selected option, and in pressed state to draw a rectangle on the being pressed option.
Usage¶
Overview¶
Set options¶
The options are passed to the drop-down list as a string with lv_dropdown_set_options(dropdown, options)
. The options should be separated by \n
. For example: "First\nSecond\nThird"
.
The string will be saved in the drop-down list, so it can in local variable too.
The lv_dropdown_add_option(dropdown, "New option", pos)
function inserts a new option to pos
index.
To save memory the options can set from a static(constant) string too with lv_dropdown_set_static_options(dropdown, options)
.
In this case the options string should be alive while the drop-down list exists and lv_dropdown_add_option
can't be used
You can select an option manually with lv_dropdown_set_selected(dropdown, id)
, where id is the index of an option.
Get selected option¶
The get the currently selected option, use lv_dropdown_get_selected(dropdown)
. It will return the index of the selected option.
lv_dropdown_get_selected_str(dropdown, buf, buf_size)
copies the name of the selected option to a buf
.
Direction¶
The list can be created on any side. The default LV_DROPDOWN_DOWN
can be modified by lv_dropdown_set_dir(dropdown, LV_DROPDOWN_DIR_LEFT/RIGHT/UP/DOWN)
function.
If the list would be vertically out of the screen, it will aligned to the edge.
Symbol¶
A symbol (typically an arrow) can be added to the drop down list with lv_dropdown_set_symbol(dropdown, LV_SYMBOL_...)
If the direction of the drop-down list is LV_DROPDOWN_DIR_LEFT
the symbol will be shown on the left, else on the right.
Maximum height¶
The maximum height of drop-down list can be set via lv_dropdown_set_max_height(dropdown, height)
. By default it's set to 3/4 vertical resolution.
Show selected¶
The main part can either show the selected option or a static text. It can controlled with lv_dropdown_set_show_selected(sropdown, true/false)
.
The static text can be set with lv_dropdown_set_text(dropdown, "Text")
. Only the pointer of the text is saved.
If you also don't want the selected option to be highlighted, a custom transparent style can be used for LV_DROPDOWN_PART_SELECTED
.
Animation time¶
The drop-down list's open/close animation time is adjusted by lv_dropdown_set_anim_time(ddlist, anim_time)
. Zero animation time means no animation.
Manually open/close¶
To manually open or close the drop-down list the lv_dropdown_open/close(dropdown, LV_ANIM_ON/OFF)
function can be used.
Events¶
Besides the Generic events, the following Special events are sent by the drop-down list:
LV_EVENT_VALUE_CHANGED - Sent when the new option is selected.
Learn more about Events.
Keys¶
The following Keys are processed by the Buttons:
LV_KEY_RIGHT/DOWN - Select the next option.
LV_KEY_LEFT/UP - Select the previous option.
LY_KEY_ENTER - Apply the selected option (Send
LV_EVENT_VALUE_CHANGED
event and close the drop-down list).
Example¶
C¶
Simple Drop down list¶
code
#include "../../../lv_examples.h"
#include <stdio.h>
#if LV_USE_DROPDOWN
static void event_handler(lv_obj_t * obj, lv_event_t event)
{
if(event == LV_EVENT_VALUE_CHANGED) {
char buf[32];
lv_dropdown_get_selected_str(obj, buf, sizeof(buf));
printf("Option: %s\n", buf);
}
}
void lv_ex_dropdown_1(void)
{
/*Create a normal drop down list*/
lv_obj_t * ddlist = lv_dropdown_create(lv_scr_act(), NULL);
lv_dropdown_set_options(ddlist, "Apple\n"
"Banana\n"
"Orange\n"
"Melon\n"
"Grape\n"
"Raspberry");
lv_obj_align(ddlist, NULL, LV_ALIGN_IN_TOP_MID, 0, 20);
lv_obj_set_event_cb(ddlist, event_handler);
}
#endif
Drop "up" list¶
code
#include "../../../lv_examples.h"
#include <stdio.h>
#if LV_USE_DROPDOWN
/**
* Create a drop LEFT menu
*/
void lv_ex_dropdown_2(void)
{
/*Create a drop down list*/
lv_obj_t * ddlist = lv_dropdown_create(lv_scr_act(), NULL);
lv_dropdown_set_options(ddlist, "Apple\n"
"Banana\n"
"Orange\n"
"Melon\n"
"Grape\n"
"Raspberry");
lv_dropdown_set_dir(ddlist, LV_DROPDOWN_DIR_LEFT);
lv_dropdown_set_symbol(ddlist, NULL);
lv_dropdown_set_show_selected(ddlist, false);
lv_dropdown_set_text(ddlist, "Fruits");
/*It will be called automatically when the size changes*/
lv_obj_align(ddlist, NULL, LV_ALIGN_IN_TOP_RIGHT, 0, 20);
/*Copy the drop LEFT list*/
ddlist = lv_dropdown_create(lv_scr_act(), ddlist);
lv_obj_align(ddlist, NULL, LV_ALIGN_IN_TOP_RIGHT, 0, 100);
}
#endif
MicroPython¶
Simple Drop down list¶
Click to try in the simulator!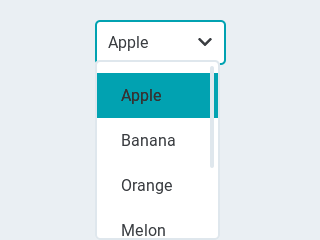
code
def event_handler(obj, event):
if event == lv.EVENT.VALUE_CHANGED:
option = " "*10 # should be large enough to store the option
obj.get_selected_str(option, len(option))
# .strip() removes trailing spaces
print("Option: \"%s\"" % option.strip())
# Create a drop down list
ddlist = lv.dropdown(lv.scr_act())
ddlist.set_options("\n".join([
"Apple",
"Banana",
"Orange",
"Melon",
"Grape",
"Raspberry"]))
# ddlist.set_fix_width(150)
# ddlist.set_draw_arrow(True)
ddlist.align(None, lv.ALIGN.IN_TOP_MID, 0, 20)
ddlist.set_event_cb(event_handler)
Drop "up" list¶
Click to try in the simulator!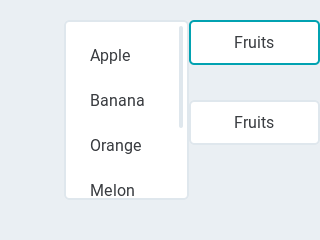
code
# Create a drop down list
ddlist = lv.dropdown(lv.scr_act())
ddlist.set_options("\n".join([
"Apple",
"Banana",
"Orange",
"Melon",
"Grape",
"Raspberry"]))
ddlist.set_dir(lv.dropdown.DIR.LEFT);
ddlist.set_symbol(None)
ddlist.set_show_selected(False)
ddlist.set_text("Fruits")
# It will be called automatically when the size changes
ddlist.align(None, lv.ALIGN.IN_TOP_RIGHT, 0, 20)
# Copy the drop LEFT list
ddlist = lv.dropdown(lv.scr_act(), ddlist)
ddlist.align(None, lv.ALIGN.IN_TOP_RIGHT, 0, 100)
API¶
Enums
Functions
-
lv_obj_t *
lv_dropdown_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a drop down list objects
- Parameters
par -- pointer to an object, it will be the parent of the new drop down list
copy -- pointer to a drop down list object, if not NULL then the new object will be copied from it
- Returns
pointer to the created drop down list
-
void
lv_dropdown_set_text
(lv_obj_t *ddlist, const char *txt)¶ Set text of the ddlist (Displayed on the button if
show_selected = false
)- Parameters
ddlist -- pointer to a drop down list object
txt -- the text as a string (Only it's pointer is saved)
-
void
lv_dropdown_clear_options
(lv_obj_t *ddlist)¶ Clear any options in a drop down list. Static or dynamic.
- Parameters
ddlist -- pointer to drop down list object
-
void
lv_dropdown_set_options
(lv_obj_t *ddlist, const char *options)¶ Set the options in a drop down list from a string
- Parameters
ddlist -- pointer to drop down list object
options --
a string with '
' separated options. E.g. "One\nTwo\nThree" The options string can be destroyed after calling this function
-
void
lv_dropdown_set_options_static
(lv_obj_t *ddlist, const char *options)¶ Set the options in a drop down list from a string
- Parameters
ddlist -- pointer to drop down list object
options --
a static string with '
' separated options. E.g. "One\nTwo\nThree"
-
void
lv_dropdown_add_option
(lv_obj_t *ddlist, const char *option, uint32_t pos)¶ Add an options to a drop down list from a string. Only works for dynamic options.
- Parameters
ddlist -- pointer to drop down list object
option --
a string without '
'. E.g. "Four"
pos -- the insert position, indexed from 0, LV_DROPDOWN_POS_LAST = end of string
-
void
lv_dropdown_set_selected
(lv_obj_t *ddlist, uint16_t sel_opt)¶ Set the selected option
- Parameters
ddlist -- pointer to drop down list object
sel_opt -- id of the selected option (0 ... number of option - 1);
-
void
lv_dropdown_set_dir
(lv_obj_t *ddlist, lv_dropdown_dir_t dir)¶ Set the direction of the a drop down list
- Parameters
ddlist -- pointer to a drop down list object
dir -- LV_DROPDOWN_DIR_LEF/RIGHT/TOP/BOTTOM
-
void
lv_dropdown_set_max_height
(lv_obj_t *ddlist, lv_coord_t h)¶ Set the maximal height for the drop down list
- Parameters
ddlist -- pointer to a drop down list
h -- the maximal height
-
void
lv_dropdown_set_symbol
(lv_obj_t *ddlist, const char *symbol)¶ Set an arrow or other symbol to display when the drop-down list is closed.
- Parameters
ddlist -- pointer to drop down list object
symbol -- a text like
LV_SYMBOL_DOWN
or NULL to not draw icon
-
void
lv_dropdown_set_show_selected
(lv_obj_t *ddlist, bool show)¶ Set whether the ddlist highlight the last selected option and display its text or not
- Parameters
ddlist -- pointer to a drop down list object
show -- true/false
-
const char *
lv_dropdown_get_text
(lv_obj_t *ddlist)¶ Get text of the ddlist (Displayed on the button if
show_selected = false
)- Parameters
ddlist -- pointer to a drop down list object
- Returns
the text string
-
const char *
lv_dropdown_get_options
(const lv_obj_t *ddlist)¶ Get the options of a drop down list
- Parameters
ddlist -- pointer to drop down list object
- Returns
the options separated by '
'-s (E.g. "Option1\nOption2\nOption3")
-
uint16_t
lv_dropdown_get_selected
(const lv_obj_t *ddlist)¶ Get the selected option
- Parameters
ddlist -- pointer to drop down list object
- Returns
id of the selected option (0 ... number of option - 1);
-
uint16_t
lv_dropdown_get_option_cnt
(const lv_obj_t *ddlist)¶ Get the total number of options
- Parameters
ddlist -- pointer to drop down list object
- Returns
the total number of options in the list
-
void
lv_dropdown_get_selected_str
(const lv_obj_t *ddlist, char *buf, uint32_t buf_size)¶ Get the current selected option as a string
- Parameters
ddlist -- pointer to ddlist object
buf -- pointer to an array to store the string
buf_size -- size of
buf
in bytes. 0: to ignore it.
-
lv_coord_t
lv_dropdown_get_max_height
(const lv_obj_t *ddlist)¶ Get the fix height value.
- Parameters
ddlist -- pointer to a drop down list object
- Returns
the height if the ddlist is opened (0: auto size)
-
const char *
lv_dropdown_get_symbol
(lv_obj_t *ddlist)¶ Get the symbol to draw when the drop-down list is closed
- Parameters
ddlist -- pointer to drop down list object
- Returns
the symbol or NULL if not enabled
-
lv_dropdown_dir_t
lv_dropdown_get_dir
(const lv_obj_t *ddlist)¶ Get the symbol to draw when the drop-down list is closed
- Parameters
ddlist -- pointer to drop down list object
- Returns
the symbol or NULL if not enabled
-
bool
lv_dropdown_get_show_selected
(lv_obj_t *ddlist)¶ Get whether the ddlist highlight the last selected option and display its text or not
- Parameters
ddlist -- pointer to a drop down list object
- Returns
true/false
-
struct
lv_dropdown_ext_t
¶ Public Members
-
const char *
text
¶
-
const char *
symbol
¶
-
char *
options
¶
-
lv_style_list_t
style_selected
¶
-
lv_style_list_t
style_page
¶
-
lv_style_list_t
style_scrlbar
¶
-
lv_coord_t
max_height
¶
-
uint16_t
option_cnt
¶
-
uint16_t
sel_opt_id
¶
-
uint16_t
sel_opt_id_orig
¶
-
uint16_t
pr_opt_id
¶
-
uint8_t
show_selected
¶
-
uint8_t
static_txt
¶
-
const char *