Window (lv_win)¶
Overview¶
The Window is container-like objects built from a header with title and button and a content area.
Parts and Styles¶
The main part is LV_WIN_PART_BG
which holds the two other real parts:
LV_WIN_PART_HEADER
: a header Container on the top with a title and control buttonsLV_WIN_PART_CONTENT_SCRL
the scrollable part of a Page for the content below the header.
Besides these, LV_WIN_PART_CONTENT_SCRL
has a scrollbar part called LV_WIN_PART_CONTENT_SCRL
.
Read the documentation of Page for more details on the scrollbars.
All parts supports the typical background properties. The title uses the Text properties of the header part.
The height of the control buttons is: header height - header padding_top - header padding_bottom.
Title¶
On the header, there is a title which can be modified by: lv_win_set_title(win, "New title")
.
Control buttons¶
Control buttons can be added to the right of the window header with: lv_win_add_btn_right(win, LV_SYMBOL_CLOSE)
, to add a button to the left side of the window header use lv_win_add_btn_left(win, LV_SYMBOL_CLOSE)
instead.
The second parameter is an Image source so it can be a symbol, a pointer to an lv_img_dsc_t
variable or a path to file.
The width of the buttons can be set with lv_win_set_btn_width(win, w)
. If w == 0
the buttons will be square-shaped.
lv_win_close_event_cb
can be used as an event callback to close the Window.
Scrollbars¶
The scrollbar behavior can be set by lv_win_set_scrlbar_mode(win, LV_SCRLBAR_MODE_...)
.
See Page for details.
Manual scroll and focus¶
To scroll the Window directly you can use lv_win_scroll_hor(win, dist_px)
or lv_win_scroll_ver(win, dist_px)
.
To make the Window show an object on it use lv_win_focus(win, child, LV_ANIM_ON/OFF)
.
The time of scroll and focus animations can be adjusted with lv_win_set_anim_time(win, anim_time_ms)
Keys¶
The following Keys are processed by the Page:
LV_KEY_RIGHT/LEFT/UP/DOWN Scroll the page
Learn more about Keys.
Example¶
C¶
Simple window¶
code
#include "../../../lv_examples.h"
#if LV_USE_WIN
void lv_ex_win_1(void)
{
/*Create a window*/
lv_obj_t * win = lv_win_create(lv_scr_act(), NULL);
lv_win_set_title(win, "Window title"); /*Set the title*/
/*Add control button to the header*/
lv_obj_t * close_btn = lv_win_add_btn(win, LV_SYMBOL_CLOSE); /*Add close button and use built-in close action*/
lv_obj_set_event_cb(close_btn, lv_win_close_event_cb);
lv_win_add_btn(win, LV_SYMBOL_SETTINGS); /*Add a setup button*/
/*Add some dummy content*/
lv_obj_t * txt = lv_label_create(win, NULL);
lv_label_set_text(txt, "This is the content of the window\n\n"
"You can add control buttons to\n"
"the window header\n\n"
"The content area becomes\n"
"automatically scrollable is it's \n"
"large enough.\n\n"
" You can scroll the content\n"
"See the scroll bar on the right!");
}
#endif
MicroPython¶
Simple window¶
Click to try in the simulator!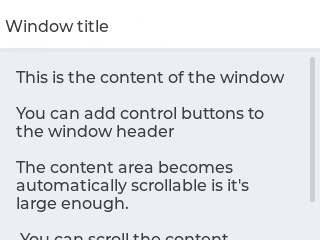
code
# create a window
win = lv.win(lv.scr_act(),None)
win.set_title("Window title") # Set the title
win_style = lv.style_t()
win_style.init()
win_style.set_margin_right(lv.STATE.DEFAULT, 50)
win.add_style(lv.win.PART.CONTENT_SCROLLABLE,win_style)
# Add control button to the header
close_btn = win.add_btn_right(lv.SYMBOL.CLOSE)
close_btn.set_event_cb(lambda obj,e: lv.win.close_event_cb(lv.win.__cast__(obj), e))
win.add_btn_right(lv.SYMBOL.SETTINGS) # Add a setup button
# Add some dummy content
txt = lv.label(win)
txt.set_text(
"""This is the content of the window
You can add control buttons to
the window header
The content area becomes automatically
scrollable if it's large enough.
You can scroll the content
See the scroll bar on the bottom!"""
)
API¶
Enums
-
enum [anonymous]¶
Window parts.
Values:
-
enumerator
LV_WIN_PART_BG
¶ Window object background style.
-
enumerator
_LV_WIN_PART_VIRTUAL_LAST
¶
-
enumerator
LV_WIN_PART_HEADER
¶ Window titlebar background style.
-
enumerator
LV_WIN_PART_CONTENT_SCROLLABLE
¶ Window content style.
-
enumerator
LV_WIN_PART_SCROLLBAR
¶ Window scrollbar style.
-
enumerator
_LV_WIN_PART_REAL_LAST
¶
-
enumerator
Functions
-
lv_obj_t *
lv_win_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a window objects
- Parameters
par -- pointer to an object, it will be the parent of the new window
copy -- pointer to a window object, if not NULL then the new object will be copied from it
- Returns
pointer to the created window
-
void
lv_win_clean
(lv_obj_t *win)¶ Delete all children of the scrl object, without deleting scrl child.
- Parameters
win -- pointer to an object
-
lv_obj_t *
lv_win_add_btn_right
(lv_obj_t *win, const void *img_src)¶ Add control button on the right side of the window header
- Parameters
win -- pointer to a window object
img_src -- an image source ('lv_img_t' variable, path to file or a symbol)
- Returns
pointer to the created button object
-
lv_obj_t *
lv_win_add_btn_left
(lv_obj_t *win, const void *img_src)¶ Add control button on the left side of the window header
- Parameters
win -- pointer to a window object
img_src -- an image source ('lv_img_t' variable, path to file or a symbol)
- Returns
pointer to the created button object
-
void
lv_win_close_event_cb
(lv_obj_t *btn, lv_event_t event)¶ Can be assigned to a window control button to close the window
- Parameters
btn -- pointer to the control button on the widows header
event -- the event type
-
void
lv_win_set_title
(lv_obj_t *win, const char *title)¶ Set the title of a window
- Parameters
win -- pointer to a window object
title -- string of the new title
-
void
lv_win_set_header_height
(lv_obj_t *win, lv_coord_t size)¶ Set the control button size of a window
- Parameters
win -- pointer to a window object
- Returns
control button size
-
void
lv_win_set_btn_width
(lv_obj_t *win, lv_coord_t width)¶ Set the width of the control buttons on the header
- Parameters
win -- pointer to a window object
width -- width of the control button. 0: to make them square automatically.
-
void
lv_win_set_content_size
(lv_obj_t *win, lv_coord_t w, lv_coord_t h)¶ Set the size of the content area.
- Parameters
win -- pointer to a window object
w -- width
h -- height (the window will be higher with the height of the header)
-
void
lv_win_set_layout
(lv_obj_t *win, lv_layout_t layout)¶ Set the layout of the window
- Parameters
win -- pointer to a window object
layout -- the layout from 'lv_layout_t'
-
void
lv_win_set_scrollbar_mode
(lv_obj_t *win, lv_scrollbar_mode_t sb_mode)¶ Set the scroll bar mode of a window
- Parameters
win -- pointer to a window object
sb_mode -- the new scroll bar mode from 'lv_scrollbar_mode_t'
-
void
lv_win_set_anim_time
(lv_obj_t *win, uint16_t anim_time)¶ Set focus animation duration on
lv_win_focus()
- Parameters
win -- pointer to a window object
anim_time -- duration of animation [ms]
-
void
lv_win_set_drag
(lv_obj_t *win, bool en)¶ Set drag status of a window. If set to 'true' window can be dragged like on a PC.
- Parameters
win -- pointer to a window object
en -- whether dragging is enabled
-
void
lv_win_title_set_alignment
(lv_obj_t *win, uint8_t alignment)¶ Set alignment of title text in window header.
- Parameters
win -- pointer to a window object
alignment -- set the type of alignment with LV_TXT_FLAGS
-
const char *
lv_win_get_title
(const lv_obj_t *win)¶ Get the title of a window
- Parameters
win -- pointer to a window object
- Returns
title string of the window
-
lv_obj_t *
lv_win_get_content
(const lv_obj_t *win)¶ Get the content holder object of window (
lv_page
) to allow additional customization- Parameters
win -- pointer to a window object
- Returns
the Page object where the window's content is
-
lv_coord_t
lv_win_get_header_height
(const lv_obj_t *win)¶ Get the header height
- Parameters
win -- pointer to a window object
- Returns
header height
-
lv_coord_t
lv_win_get_btn_width
(lv_obj_t *win)¶ Get the width of the control buttons on the header
- Parameters
win -- pointer to a window object
- Returns
width of the control button. 0: square.
-
lv_obj_t *
lv_win_get_from_btn
(const lv_obj_t *ctrl_btn)¶ Get the pointer of a widow from one of its control button. It is useful in the action of the control buttons where only button is known.
- Parameters
ctrl_btn -- pointer to a control button of a window
- Returns
pointer to the window of 'ctrl_btn'
-
lv_layout_t
lv_win_get_layout
(lv_obj_t *win)¶ Get the layout of a window
- Parameters
win -- pointer to a window object
- Returns
the layout of the window (from 'lv_layout_t')
-
lv_scrollbar_mode_t
lv_win_get_sb_mode
(lv_obj_t *win)¶ Get the scroll bar mode of a window
- Parameters
win -- pointer to a window object
- Returns
the scroll bar mode of the window (from 'lv_sb_mode_t')
-
uint16_t
lv_win_get_anim_time
(const lv_obj_t *win)¶ Get focus animation duration
- Parameters
win -- pointer to a window object
- Returns
duration of animation [ms]
-
lv_coord_t
lv_win_get_width
(lv_obj_t *win)¶ Get width of the content area (page scrollable) of the window
- Parameters
win -- pointer to a window object
- Returns
the width of the content area
-
static inline bool
lv_win_get_drag
(const lv_obj_t *win)¶ Get drag status of a window. If set to 'true' window can be dragged like on a PC.
- Parameters
win -- pointer to a window object
- Returns
whether window is draggable
-
uint8_t
lv_win_title_get_alignment
(lv_obj_t *win)¶ Get the current alignment of title text in window header.
- Parameters
win -- pointer to a window object
-
void
lv_win_focus
(lv_obj_t *win, lv_obj_t *obj, lv_anim_enable_t anim_en)¶ Focus on an object. It ensures that the object will be visible in the window.
- Parameters
win -- pointer to a window object
obj -- pointer to an object to focus (must be in the window)
anim_en -- LV_ANIM_ON focus with an animation; LV_ANIM_OFF focus without animation
-
struct
lv_win_ext_t
¶