List (lv_list)¶
Overview¶
The Lists are built from a background Page and Buttons on it. The Buttons contain an optional icon-like Image (which can be a symbol too) and a Label. When the list becomes long enough it can be scrolled.
Parts and Styles¶
The List has the same parts as the Page
LV_LIST_PART_BG
LV_LIST_PART_SCRL
LV_LIST_PART_SCRLBAR
LV_LIST_PART_EDGE_FLASH
Refer to the Page documentation for details.
The buttons on the list are treated as normal buttons and they only have a main part called LV_BTN_PART_MAIN
.
Usage¶
Add buttons¶
You can add new list elements (button) with lv_list_add_btn(list, &icon_img, "Text")
or with symbol lv_list_add_btn(list, SYMBOL_EDIT, "Edit text")
.
If you do not want to add image use NULL
as image source. The function returns with a pointer to the created button to allow further configurations.
The width of the buttons is set to maximum according to the object width. The height of the buttons are adjusted automatically according to the content. (content height + padding_top + padding_bottom).
The labels are created with LV_LABEL_LONG_SROLL_CIRC
long mode to automatically scroll the long labels circularly.
lv_list_get_btn_label(list_btn)
and lv_list_get_btn_img(list_btn)
can be used to get the label and the image of a list button.
The text can be et directly with lv_list_get_btn_text(list_btn)
.
Delete buttons¶
To delete a list element use lv_list_remove(list, btn_index)
. btn_index can be obtained by lv_list_get_btn_index(list, btn)
where btn is the return value of lv_list_add_btn()
.
To clean the list (remove all buttons) use lv_list_clean(list)
Layout¶
By default the list is vertical. To get a horizontal list use lv_list_set_layout(list, LV_LAYOUT_ROW_MID)
.
Edge flash¶
A circle-like effect can be shown when the list reaches the most top or bottom position.
lv_list_set_edge_flash(list, true)
enables this feature.
Keys¶
The following Keys are processed by the Lists:
LV_KEY_RIGHT/DOWN Select the next button
LV_KEY_LEFT/UP Select the previous button
Note that, as usual, the state of LV_KEY_ENTER
is translated to LV_EVENT_PRESSED/PRESSING/RELEASED
etc.
The Selected buttons are in LV_BTN_STATE_PR/TG_PR
state.
To manually select a button use lv_list_set_btn_selected(list, btn)
. When the list is defocused and focused again it will restore the last selected button.
Learn more about Keys.
Example¶
C¶
Simple List¶
code
#include "../../../lv_examples.h"
#include <stdio.h>
#if LV_USE_LIST
static void event_handler(lv_obj_t * obj, lv_event_t event)
{
if(event == LV_EVENT_CLICKED) {
printf("Clicked: %s\n", lv_list_get_btn_text(obj));
}
}
void lv_ex_list_1(void)
{
/*Create a list*/
lv_obj_t * list1 = lv_list_create(lv_scr_act(), NULL);
lv_obj_set_size(list1, 160, 200);
lv_obj_align(list1, NULL, LV_ALIGN_CENTER, 0, 0);
/*Add buttons to the list*/
lv_obj_t * list_btn;
list_btn = lv_list_add_btn(list1, LV_SYMBOL_FILE, "New");
lv_obj_set_event_cb(list_btn, event_handler);
list_btn = lv_list_add_btn(list1, LV_SYMBOL_DIRECTORY, "Open");
lv_obj_set_event_cb(list_btn, event_handler);
list_btn = lv_list_add_btn(list1, LV_SYMBOL_CLOSE, "Delete");
lv_obj_set_event_cb(list_btn, event_handler);
list_btn = lv_list_add_btn(list1, LV_SYMBOL_EDIT, "Edit");
lv_obj_set_event_cb(list_btn, event_handler);
list_btn = lv_list_add_btn(list1, LV_SYMBOL_SAVE, "Save");
lv_obj_set_event_cb(list_btn, event_handler);
list_btn = lv_list_add_btn(list1, LV_SYMBOL_BELL, "Notify");
lv_obj_set_event_cb(list_btn, event_handler);
list_btn = lv_list_add_btn(list1, LV_SYMBOL_BATTERY_FULL, "Battery");
lv_obj_set_event_cb(list_btn, event_handler);
}
#endif
MicroPython¶
Simple List¶
Click to try in the simulator!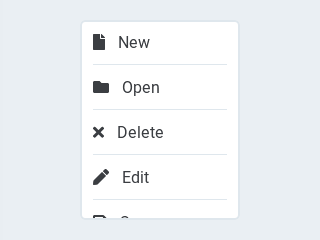
code
def event_handler(obj, event):
list_btn = lv.list.__cast__(obj)
if event == lv.EVENT.CLICKED:
print("Clicked: %s" % list_btn.get_btn_text())
# Create a list
list1 = lv.list(lv.scr_act())
list1.set_size(160, 200)
list1.align(None, lv.ALIGN.CENTER, 0, 0)
# Add buttons to the list
list_btn = list1.add_btn(lv.SYMBOL.FILE, "New")
list_btn.set_event_cb(event_handler)
list_btn = list1.add_btn(lv.SYMBOL.DIRECTORY, "Open")
list_btn.set_event_cb(event_handler)
list_btn = list1.add_btn(lv.SYMBOL.CLOSE, "Delete")
list_btn.set_event_cb(event_handler)
list_btn = list1.add_btn(lv.SYMBOL.EDIT, "Edit")
list_btn.set_event_cb(event_handler)
list_btn = list1.add_btn(lv.SYMBOL.SAVE, "Save")
list_btn.set_event_cb(event_handler)
list_btn = list1.add_btn(lv.SYMBOL.BELL, "Notify")
list_btn.set_event_cb(event_handler)
list_btn = list1.add_btn(lv.SYMBOL.BATTERY_FULL, "Battery")
list_btn.set_event_cb(event_handler)
API¶
Typedefs
-
typedef uint8_t
lv_list_style_t
¶
Enums
-
enum [anonymous]¶
List styles.
Values:
-
enumerator
LV_LIST_PART_BG
¶ List background style
-
enumerator
LV_LIST_PART_SCROLLBAR
¶ List scrollbar style.
-
enumerator
LV_LIST_PART_EDGE_FLASH
¶ List edge flash style.
-
enumerator
_LV_LIST_PART_VIRTUAL_LAST
¶
-
enumerator
LV_LIST_PART_SCROLLABLE
¶ List scrollable area style.
-
enumerator
_LV_LIST_PART_REAL_LAST
¶
-
enumerator
Functions
-
lv_obj_t *
lv_list_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a list objects
- Parameters
par -- pointer to an object, it will be the parent of the new list
copy -- pointer to a list object, if not NULL then the new object will be copied from it
- Returns
pointer to the created list
-
void
lv_list_clean
(lv_obj_t *list)¶ Delete all children of the scrl object, without deleting scrl child.
- Parameters
list -- pointer to an object
-
lv_obj_t *
lv_list_add_btn
(lv_obj_t *list, const void *img_src, const char *txt)¶ Add a list element to the list
- Parameters
list -- pointer to list object
img_fn -- file name of an image before the text (NULL if unused)
txt -- text of the list element (NULL if unused)
- Returns
pointer to the new list element which can be customized (a button)
-
bool
lv_list_remove
(const lv_obj_t *list, uint16_t index)¶ Remove the index of the button in the list
- Parameters
list -- pointer to a list object
index -- pointer to a the button's index in the list, index must be 0 <= index < lv_list_ext_t.size
- Returns
true: successfully deleted
-
void
lv_list_focus_btn
(lv_obj_t *list, lv_obj_t *btn)¶ Make a button selected
- Parameters
list -- pointer to a list object
btn -- pointer to a button to select NULL to not select any buttons
-
static inline void
lv_list_set_scrollbar_mode
(lv_obj_t *list, lv_scrollbar_mode_t mode)¶ Set the scroll bar mode of a list
- Parameters
list -- pointer to a list object
sb_mode -- the new mode from 'lv_page_sb_mode_t' enum
-
static inline void
lv_list_set_scroll_propagation
(lv_obj_t *list, bool en)¶ Enable the scroll propagation feature. If enabled then the List will move its parent if there is no more space to scroll.
- Parameters
list -- pointer to a List
en -- true or false to enable/disable scroll propagation
-
static inline void
lv_list_set_edge_flash
(lv_obj_t *list, bool en)¶ Enable the edge flash effect. (Show an arc when the an edge is reached)
- Parameters
list -- pointer to a List
en -- true or false to enable/disable end flash
-
static inline void
lv_list_set_anim_time
(lv_obj_t *list, uint16_t anim_time)¶ Set scroll animation duration on 'list_up()' 'list_down()' 'list_focus()'
- Parameters
list -- pointer to a list object
anim_time -- duration of animation [ms]
-
void
lv_list_set_layout
(lv_obj_t *list, lv_layout_t layout)¶ Set layout of a list
- Parameters
list -- pointer to a list object
layout -- which layout should be used
-
const char *
lv_list_get_btn_text
(const lv_obj_t *btn)¶ Get the text of a list element
- Parameters
btn -- pointer to list element
- Returns
pointer to the text
-
lv_obj_t *
lv_list_get_btn_label
(const lv_obj_t *btn)¶ Get the label object from a list element
- Parameters
btn -- pointer to a list element (button)
- Returns
pointer to the label from the list element or NULL if not found
-
lv_obj_t *
lv_list_get_btn_img
(const lv_obj_t *btn)¶ Get the image object from a list element
- Parameters
btn -- pointer to a list element (button)
- Returns
pointer to the image from the list element or NULL if not found
-
lv_obj_t *
lv_list_get_prev_btn
(const lv_obj_t *list, lv_obj_t *prev_btn)¶ Get the next button from list. (Starts from the bottom button)
- Parameters
list -- pointer to a list object
prev_btn -- pointer to button. Search the next after it.
- Returns
pointer to the next button or NULL when no more buttons
-
lv_obj_t *
lv_list_get_next_btn
(const lv_obj_t *list, lv_obj_t *prev_btn)¶ Get the previous button from list. (Starts from the top button)
- Parameters
list -- pointer to a list object
prev_btn -- pointer to button. Search the previous before it.
- Returns
pointer to the previous button or NULL when no more buttons
-
int32_t
lv_list_get_btn_index
(const lv_obj_t *list, const lv_obj_t *btn)¶ Get the index of the button in the list
- Parameters
list -- pointer to a list object. If NULL, assumes btn is part of a list.
btn -- pointer to a list element (button)
- Returns
the index of the button in the list, or -1 of the button not in this list
-
uint16_t
lv_list_get_size
(const lv_obj_t *list)¶ Get the number of buttons in the list
- Parameters
list -- pointer to a list object
- Returns
the number of buttons in the list
-
lv_obj_t *
lv_list_get_btn_selected
(const lv_obj_t *list)¶ Get the currently selected button. Can be used while navigating in the list with a keypad.
- Parameters
list -- pointer to a list object
- Returns
pointer to the selected button
-
lv_layout_t
lv_list_get_layout
(lv_obj_t *list)¶ Get layout of a list
- Parameters
list -- pointer to a list object
- Returns
layout of the list object
-
static inline lv_scrollbar_mode_t
lv_list_get_scrollbar_mode
(const lv_obj_t *list)¶ Get the scroll bar mode of a list
- Parameters
list -- pointer to a list object
- Returns
scrollbar mode from 'lv_scrollbar_mode_t' enum
-
static inline bool
lv_list_get_scroll_propagation
(lv_obj_t *list)¶ Get the scroll propagation property
- Parameters
list -- pointer to a List
- Returns
true or false
-
static inline bool
lv_list_get_edge_flash
(lv_obj_t *list)¶ Get the scroll propagation property
- Parameters
list -- pointer to a List
- Returns
true or false
-
static inline uint16_t
lv_list_get_anim_time
(const lv_obj_t *list)¶ Get scroll animation duration
- Parameters
list -- pointer to a list object
- Returns
duration of animation [ms]
-
void
lv_list_up
(const lv_obj_t *list)¶ Move the list elements up by one
- Parameters
list -- pointer a to list object
-
void
lv_list_down
(const lv_obj_t *list)¶ Move the list elements down by one
- Parameters
list -- pointer to a list object
-
void
lv_list_focus
(const lv_obj_t *btn, lv_anim_enable_t anim)¶ Focus on a list button. It ensures that the button will be visible on the list.
- Parameters
btn -- pointer to a list button to focus
anim -- LV_ANIM_ON: scroll with animation, LV_ANIM_OFF: without animation
-
struct
lv_list_ext_t
¶