Line meter (lv_lmeter)¶
Overview¶
The Line meter object consists of some radial lines which draw a scale. Setting a value for the Line meter will change the color of the scale lines proportionally.
Parts and Styles¶
The Line meter has only a main part, called LV_LINEMETER_PART_MAIN
. It uses all the typical background properties the draw a rectangle-like or circle background and the line and scale properties to draw the scale lines.
The active lines (which are related to smaller values the the current value) are colored from line_color to scale_grad_color. The lines in the end (after the current value) are set to scale_end_color color.
Usage¶
Set value¶
When setting a new value with lv_linemeter_set_value(linemeter, new_value)
the proportional part of the scale will be recolored.
Range and Angles¶
The lv_linemeter_set_range(linemeter, min, max)
function sets the range of the line meter.
You can set the angle of the scale and the number of the lines by: lv_linemeter_set_scale(linemeter, angle, line_num)
.
The default angle is 240 and the default line number is 31.
Angle offset¶
By default the scale angle is interpreted symmetrically to the y axis. It results in "standing" line meter. With lv_linemeter_set_angle_offset
an offset can be added the scale angle.
It can used e.g to put a quarter line meter into a corner or a half line meter to the right or left side.
Mirror¶
By default the Line meter's lines are activated clock-wise. It can be changed using lv_linemeter_set_mirror(linemeter, true/false)
.
Example¶
C¶
Simple Line meter¶
code
#include "../../../lv_examples.h"
#if LV_USE_LINEMETER
void lv_ex_linemeter_1(void)
{
/*Create a line meter */
lv_obj_t * lmeter;
lmeter = lv_linemeter_create(lv_scr_act(), NULL);
lv_linemeter_set_range(lmeter, 0, 100); /*Set the range*/
lv_linemeter_set_value(lmeter, 80); /*Set the current value*/
lv_linemeter_set_scale(lmeter, 240, 21); /*Set the angle and number of lines*/
lv_obj_set_size(lmeter, 150, 150);
lv_obj_align(lmeter, NULL, LV_ALIGN_CENTER, 0, 0);
}
#endif
MicroPython¶
Simple Line meter¶
Click to try in the simulator!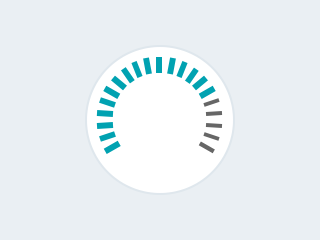
code
lmeter = lv.linemeter(lv.scr_act(),None)
lmeter.set_range(0,100) # Set the range
lmeter.set_value(80) # Set the current value
lmeter.set_scale(240,21) # Set the angle and number of lines
lmeter.set_size(150,150)
lmeter.align(None,lv.ALIGN.CENTER,0,0)
API¶
Typedefs
-
typedef uint8_t
lv_linemeter_part_t
¶
Enums
Functions
-
lv_obj_t *
lv_linemeter_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a line meter objects
- Parameters
par -- pointer to an object, it will be the parent of the new line meter
copy -- pointer to a line meter object, if not NULL then the new object will be copied from it
- Returns
pointer to the created line meter
-
void
lv_linemeter_set_value
(lv_obj_t *lmeter, int32_t value)¶ Set a new value on the line meter
- Parameters
lmeter -- pointer to a line meter object
value -- new value
-
void
lv_linemeter_set_range
(lv_obj_t *lmeter, int32_t min, int32_t max)¶ Set minimum and the maximum values of a line meter
- Parameters
lmeter -- pointer to he line meter object
min -- minimum value
max -- maximum value
-
void
lv_linemeter_set_scale
(lv_obj_t *lmeter, uint16_t angle, uint16_t line_cnt)¶ Set the scale settings of a line meter
- Parameters
lmeter -- pointer to a line meter object
angle -- angle of the scale (0..360)
line_cnt -- number of lines
-
void
lv_linemeter_set_angle_offset
(lv_obj_t *lmeter, uint16_t angle)¶ Set the set an offset for the line meter's angles to rotate it.
- Parameters
lmeter -- pointer to a line meter object
angle -- angle offset (0..360), rotates clockwise
-
void
lv_linemeter_set_mirror
(lv_obj_t *lmeter, bool mirror)¶ Set the orientation of the meter growth, clockwise or counterclockwise (mirrored)
- Parameters
lmeter -- pointer to a line meter object
mirror -- mirror setting
-
int32_t
lv_linemeter_get_value
(const lv_obj_t *lmeter)¶ Get the value of a line meter
- Parameters
lmeter -- pointer to a line meter object
- Returns
the value of the line meter
-
int32_t
lv_linemeter_get_min_value
(const lv_obj_t *lmeter)¶ Get the minimum value of a line meter
- Parameters
lmeter -- pointer to a line meter object
- Returns
the minimum value of the line meter
-
int32_t
lv_linemeter_get_max_value
(const lv_obj_t *lmeter)¶ Get the maximum value of a line meter
- Parameters
lmeter -- pointer to a line meter object
- Returns
the maximum value of the line meter
-
uint16_t
lv_linemeter_get_line_count
(const lv_obj_t *lmeter)¶ Get the scale number of a line meter
- Parameters
lmeter -- pointer to a line meter object
- Returns
number of the scale units
-
uint16_t
lv_linemeter_get_scale_angle
(const lv_obj_t *lmeter)¶ Get the scale angle of a line meter
- Parameters
lmeter -- pointer to a line meter object
- Returns
angle of the scale
-
struct
lv_linemeter_ext_t
¶