Label (lv_label)¶
Overview¶
A label is the basic object type that is used to display text.
Parts and Styles¶
The label has only a main part, called LV_LABEL_PART_MAIN
. It uses all the typical background properties and the text properties.
The padding values can be used to make the area for the text small in the related direction.
Usage¶
Set text¶
You can set the text on a label at runtime with lv_label_set_text(label, "New text")
.
It will allocate a buffer dynamically, and the provided string will be copied into that buffer.
Therefore, you don't need to keep the text you pass to lv_label_set_text
in scope after that function returns.
With lv_label_set_text_fmt(label, "Value: %d", 15)
printf formatting can be used to set the text.
Labels are able to show text from a static character buffer which is \0
-terminated.
To do so, use lv_label_set_text_static(label, "Text")
.
In this case, the text is not stored in the dynamic memory and the given buffer is used directly instead.
This means that the array can't be a local variable which goes out of scope when the function exits.
Constant strings are safe to use with lv_label_set_text_static
(except when used with LV_LABEL_LONG_DOT
, as it modifies the buffer in-place), as they are stored in ROM memory, which is always accessible.
Line break¶
Line breaks are handled automatically by the label object. You can use \n
to make a line break. For example: "line1\nline2\n\nline4"
Long modes¶
By default, the width of the label object automatically expands to the text size. Otherwise, the text can be manipulated according to several long mode policies:
LV_LABEL_LONG_EXPAND - Expand the object size to the text size (Default)
LV_LABEL_LONG_BREAK - Keep the object width, break (wrap) the too long lines and expand the object height
LV_LABEL_LONG_DOT - Keep the object size, break the text and write dots in the last line (not supported when using
lv_label_set_text_static
)LV_LABEL_LONG_SROLL - Keep the size and scroll the label back and forth
LV_LABEL_LONG_SROLL_CIRC - Keep the size and scroll the label circularly
LV_LABEL_LONG_CROP - Keep the size and crop the text out of it
You can specify the long mode with lv_label_set_long_mode(label, LV_LABEL_LONG_...)
It's important to note that, when a label is created and its text is set, the label's size already expanded to the text size.
In addition with the default LV_LABEL_LONG_EXPAND
, long mode lv_obj_set_width/height/size()
has no effect.
So you need to change the long mode first set the new long mode and then set the size with lv_obj_set_width/height/size()
.
Another important note is that LV_LABEL_LONG_DOT
manipulates the text buffer in-place in order to add/remove the dots.
When lv_label_set_text
or lv_label_set_array_text
are used, a separate buffer is allocated and this implementation detail is unnoticed.
This is not the case with lv_label_set_text_static
! The buffer you pass to lv_label_set_text_static
must be writable if you plan to use LV_LABEL_LONG_DOT
.
Text align¶
The lines of the text can be aligned to the left, right or center with lv_label_set_align(label, LV_LABEL_ALIGN_LEFT/RIGHT/CENTER)
. Note that, it will align only the lines, not the label object itself.
Vertical alignment is not supported by the label itself; you should place the label inside a larger container and align the whole label object instead.
Text recolor¶
In the text, you can use commands to recolor parts of the text. For example: "Write a #ff0000 red# word"
.
This feature can be enabled individually for each label by lv_label_set_recolor()
function.
Note that, recoloring work only in a single line. Therefore, \n
should not use in a recolored text or it should be wrapped by LV_LABEL_LONG_BREAK
else, the text in the new line won't be recolored.
Very long texts¶
Lvgl can efficiently handle very long (> 40k characters) by saving some extra data (~12 bytes) to speed up drawing. To enable this feature, set LV_LABEL_LONG_TXT_HINT 1
in lv_conf.h.
Example¶
C¶
Label recoloring and scrolling¶
code
#include "../../../lv_examples.h"
#if LV_USE_LABEL
void lv_ex_label_1(void)
{
lv_obj_t * label1 = lv_label_create(lv_scr_act(), NULL);
lv_label_set_long_mode(label1, LV_LABEL_LONG_BREAK); /*Break the long lines*/
lv_label_set_recolor(label1, true); /*Enable re-coloring by commands in the text*/
lv_label_set_align(label1, LV_LABEL_ALIGN_CENTER); /*Center aligned lines*/
lv_label_set_text(label1, "#0000ff Re-color# #ff00ff words# #ff0000 of a# label "
"and wrap long text automatically.");
lv_obj_set_width(label1, 150);
lv_obj_align(label1, NULL, LV_ALIGN_CENTER, 0, -30);
lv_obj_t * label2 = lv_label_create(lv_scr_act(), NULL);
lv_label_set_long_mode(label2, LV_LABEL_LONG_SROLL_CIRC); /*Circular scroll*/
lv_obj_set_width(label2, 150);
lv_label_set_text(label2, "It is a circularly scrolling text. ");
lv_obj_align(label2, NULL, LV_ALIGN_CENTER, 0, 30);
}
#endif
Text shadow¶
code
#include "../../../lv_examples.h"
#if LV_USE_LABEL
void lv_ex_label_2(void)
{
/* Create a style for the shadow*/
static lv_style_t label_shadow_style;
lv_style_init(&label_shadow_style);
lv_style_set_text_opa(&label_shadow_style, LV_STATE_DEFAULT, LV_OPA_50);
lv_style_set_text_color(&label_shadow_style, LV_STATE_DEFAULT, LV_COLOR_RED);
/*Create a label for the shadow first (it's in the background) */
lv_obj_t * shadow_label = lv_label_create(lv_scr_act(), NULL);
lv_obj_add_style(shadow_label, LV_LABEL_PART_MAIN, &label_shadow_style);
/* Create the main label */
lv_obj_t * main_label = lv_label_create(lv_scr_act(), NULL);
lv_label_set_text(main_label, "A simple method to create\n"
"shadows on text\n"
"It even works with\n\n"
"newlines and spaces.");
/*Set the same text for the shadow label*/
lv_label_set_text(shadow_label, lv_label_get_text(main_label));
/* Position the main label */
lv_obj_align(main_label, NULL, LV_ALIGN_CENTER, 0, 0);
/* Shift the second label down and to the right by 2 pixel */
lv_obj_align(shadow_label, main_label, LV_ALIGN_IN_TOP_LEFT, 1, 1);
}
#endif
Align labels¶
code
#include "../../../lv_examples.h"
#if LV_USE_LABEL
static void text_changer(lv_task_t * t);
lv_obj_t * labels[3];
/**
* Create three labels to demonstrate the alignments.
*/
void lv_ex_label_3(void)
{
/*`lv_label_set_align` is not required to align the object itslef.
* It's used only when the text has multiple lines*/
/* Create a label on the top.
* No additional alignment so it will be the reference*/
labels[0] = lv_label_create(lv_scr_act(), NULL);
lv_obj_align(labels[0], NULL, LV_ALIGN_IN_TOP_MID, 0, 5);
lv_label_set_align(labels[0], LV_LABEL_ALIGN_CENTER);
/* Create a label in the middle.
* `lv_obj_align` will be called every time the text changes
* to keep the middle position */
labels[1] = lv_label_create(lv_scr_act(), NULL);
lv_obj_align(labels[1], NULL, LV_ALIGN_CENTER, 0, 0);
lv_label_set_align(labels[1], LV_LABEL_ALIGN_CENTER);
/* Create a label in the bottom.
* Enable auto realign. */
labels[2] = lv_label_create(lv_scr_act(), NULL);
lv_obj_set_auto_realign(labels[2], true);
lv_obj_align(labels[2], NULL, LV_ALIGN_IN_BOTTOM_MID, 0, -5);
lv_label_set_align(labels[2], LV_LABEL_ALIGN_CENTER);
lv_task_t * t = lv_task_create(text_changer, 1000, LV_TASK_PRIO_MID, NULL);
lv_task_ready(t);
}
static void text_changer(lv_task_t * t)
{
const char * texts[] = {"Text", "A very long text", "A text with\nmultiple\nlines", NULL};
static uint8_t i = 0;
lv_label_set_text(labels[0], texts[i]);
lv_label_set_text(labels[1], texts[i]);
lv_label_set_text(labels[2], texts[i]);
/*Manually realaign `labels[1]`*/
lv_obj_align(labels[1], NULL, LV_ALIGN_CENTER, 0, 0);
i++;
if(texts[i] == NULL) i = 0;
}
#endif
MicroPython¶
Label recoloring and scrolling¶
Click to try in the simulator!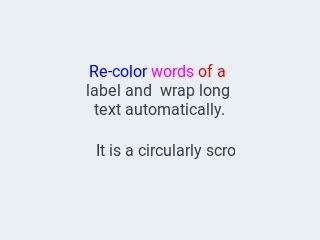
code
label1 = lv.label(lv.scr_act(),None)
label1.set_long_mode(lv.label.LONG.BREAK) # Break the long lines
label1.set_recolor(True) # Enable re-coloring by commands in the text
label1.set_align(lv.label.ALIGN.CENTER) # Center aligned lines
label1.set_text(
"""#0000ff Re-color# #ff00ff words# #ff0000 of a# label
and wrap long text automatically.""")
label1.set_width(150)
label1.align(None,lv.ALIGN.CENTER, 0, -30)
label2 = lv.label(lv.scr_act(), None)
label2.set_long_mode(lv.label.LONG.SROLL_CIRC)
label2.set_width(150)
label2.set_text("It is a circularly scrolling text. ")
label2.align(None, lv.ALIGN.CENTER, 0, 30)
Text shadow¶
Click to try in the simulator!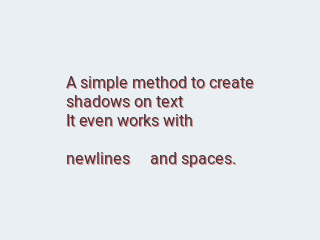
code
from lv_colors import lv_colors
# Create a style for the shadow
label_shadow_style = lv.style_t()
label_shadow_style.init()
label_shadow_style.set_text_opa(lv.STATE.DEFAULT,lv.OPA._50)
label_shadow_style.set_text_color(lv.STATE.DEFAULT, lv_colors.RED);
# Create a label for the shadow first (it's in the background)
shadow_label = lv.label(lv.scr_act())
shadow_label.add_style(lv.label.PART.MAIN, label_shadow_style)
# Create the main label
main_label = lv.label(lv.scr_act())
main_label.set_text("A simple method to create\n" +
"shadows on text\n" +
"It even works with\n\n" +
"newlines and spaces.")
# Set the same text for the shadow label
shadow_label.set_text(main_label.get_text())
# Position the main label
main_label.align(None, lv.ALIGN.CENTER, 0, 0)
# Shift the second label down and to the right by 1 pixel
shadow_label.align(main_label, lv.ALIGN.IN_TOP_LEFT, 1, 1)
while True:
lv.task_handler()
time.sleep_ms(5)
Align labels¶
Click to try in the simulator!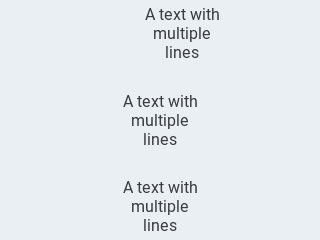
code
# Create three labels to demonstrate the alignments.
labels = []
# `label.set_align` is not required to align the object itslef.
# It's used only when the text has multiple lines
# Create a label on the top.
# No additional alignment so it will be the reference
label = lv.label(lv.scr_act())
label.align(None, lv.ALIGN.IN_TOP_MID, 0, 5)
label.set_align(lv.label.ALIGN.CENTER)
labels.append(label)
# Create a label in the middle.
# `obj.align` will be called every time the text changes
# to keep the middle position
label = lv.label(lv.scr_act())
label.align(None, lv.ALIGN.CENTER, 0, 0)
label.set_align(lv.label.ALIGN.CENTER)
labels.append(label)
# Create a label in the bottom.
# Enable auto realign.
label = lv.label(lv.scr_act())
label.set_auto_realign(True)
label.align(None, lv.ALIGN.IN_BOTTOM_MID, 0, -5)
label.set_align(lv.label.ALIGN.CENTER)
labels.append(label)
class TextChanger:
"""Changes texts of all labels every second"""
def __init__(self, labels,
texts=["Text", "A very long text", "A text with\nmultiple\nlines"],
rate=1000):
self.texts = texts
self.labels = labels
self.rate = rate
self.counter = 0
def start(self):
lv.task_create(self.task_cb, self.rate, lv.TASK_PRIO.LOWEST, None)
def task_cb(self, task):
for label in labels:
label.set_text(self.texts[self.counter])
# Manually realaign `labels[1]`
if len(self.labels) > 1:
self.labels[1].align(None, lv.ALIGN.CENTER, 0, 0)
self.counter = (self.counter + 1) % len(self.texts)
text_changer = TextChanger(labels)
text_changer.start()
API¶
Typedefs
-
typedef uint8_t
lv_label_long_mode_t
¶
-
typedef uint8_t
lv_label_align_t
¶
-
typedef uint8_t
lv_label_part_t
¶
Enums
-
enum [anonymous]¶
Long mode behaviors. Used in 'lv_label_ext_t'
Values:
-
enumerator
LV_LABEL_LONG_EXPAND
¶ Expand the object size to the text size
-
enumerator
LV_LABEL_LONG_BREAK
¶ Keep the object width, break the too long lines and expand the object height
-
enumerator
LV_LABEL_LONG_DOT
¶ Keep the size and write dots at the end if the text is too long
-
enumerator
LV_LABEL_LONG_SROLL
¶ Keep the size and roll the text back and forth
-
enumerator
LV_LABEL_LONG_SROLL_CIRC
¶ Keep the size and roll the text circularly
-
enumerator
LV_LABEL_LONG_CROP
¶ Keep the size and crop the text out of it
-
enumerator
-
enum [anonymous]¶
Label align policy
Values:
-
enumerator
LV_LABEL_ALIGN_LEFT
¶ Align text to left
-
enumerator
LV_LABEL_ALIGN_CENTER
¶ Align text to center
-
enumerator
LV_LABEL_ALIGN_RIGHT
¶ Align text to right
-
enumerator
LV_LABEL_ALIGN_AUTO
¶ Use LEFT or RIGHT depending on the direction of the text (LTR/RTL)
-
enumerator
Functions
-
LV_EXPORT_CONST_INT
(LV_LABEL_DOT_NUM)¶
-
LV_EXPORT_CONST_INT
(LV_LABEL_POS_LAST)¶
-
LV_EXPORT_CONST_INT
(LV_LABEL_TEXT_SEL_OFF)¶
-
lv_obj_t *
lv_label_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a label objects
- Parameters
par -- pointer to an object, it will be the parent of the new label
copy -- pointer to a button object, if not NULL then the new object will be copied from it
- Returns
pointer to the created button
-
void
lv_label_set_text
(lv_obj_t *label, const char *text)¶ Set a new text for a label. Memory will be allocated to store the text by the label.
- Parameters
label -- pointer to a label object
text -- '\0' terminated character string. NULL to refresh with the current text.
-
void
lv_label_set_text_fmt
(lv_obj_t *label, const char *fmt, ...)¶ Set a new formatted text for a label. Memory will be allocated to store the text by the label.
- Parameters
label -- pointer to a label object
fmt --
printf
-like format
-
void
lv_label_set_text_static
(lv_obj_t *label, const char *text)¶ Set a static text. It will not be saved by the label so the 'text' variable has to be 'alive' while the label exist.
- Parameters
label -- pointer to a label object
text -- pointer to a text. NULL to refresh with the current text.
-
void
lv_label_set_long_mode
(lv_obj_t *label, lv_label_long_mode_t long_mode)¶ Set the behavior of the label with longer text then the object size
- Parameters
label -- pointer to a label object
long_mode -- the new mode from 'lv_label_long_mode' enum. In LV_LONG_BREAK/LONG/ROLL the size of the label should be set AFTER this function
-
void
lv_label_set_align
(lv_obj_t *label, lv_label_align_t align)¶ Set the align of the label (left or center)
- Parameters
label -- pointer to a label object
align -- 'LV_LABEL_ALIGN_LEFT' or 'LV_LABEL_ALIGN_LEFT'
-
void
lv_label_set_recolor
(lv_obj_t *label, bool en)¶ Enable the recoloring by in-line commands
- Parameters
label -- pointer to a label object
en -- true: enable recoloring, false: disable
-
void
lv_label_set_anim_speed
(lv_obj_t *label, uint16_t anim_speed)¶ Set the label's animation speed in LV_LABEL_LONG_SROLL/SROLL_CIRC modes
- Parameters
label -- pointer to a label object
anim_speed -- speed of animation in px/sec unit
-
void
lv_label_set_text_sel_start
(lv_obj_t *label, uint32_t index)¶ Set the selection start index.
- Parameters
label -- pointer to a label object.
index -- index to set.
LV_LABEL_TXT_SEL_OFF
to select nothing.
-
void
lv_label_set_text_sel_end
(lv_obj_t *label, uint32_t index)¶ Set the selection end index.
- Parameters
label -- pointer to a label object.
index -- index to set.
LV_LABEL_TXT_SEL_OFF
to select nothing.
-
char *
lv_label_get_text
(const lv_obj_t *label)¶ Get the text of a label
- Parameters
label -- pointer to a label object
- Returns
the text of the label
-
lv_label_long_mode_t
lv_label_get_long_mode
(const lv_obj_t *label)¶ Get the long mode of a label
- Parameters
label -- pointer to a label object
- Returns
the long mode
-
lv_label_align_t
lv_label_get_align
(const lv_obj_t *label)¶ Get the align attribute
- Parameters
label -- pointer to a label object
- Returns
LV_LABEL_ALIGN_LEFT or LV_LABEL_ALIGN_CENTER
-
bool
lv_label_get_recolor
(const lv_obj_t *label)¶ Get the recoloring attribute
- Parameters
label -- pointer to a label object
- Returns
true: recoloring is enabled, false: disable
-
uint16_t
lv_label_get_anim_speed
(const lv_obj_t *label)¶ Get the label's animation speed in LV_LABEL_LONG_ROLL and SCROLL modes
- Parameters
label -- pointer to a label object
- Returns
speed of animation in px/sec unit
-
void
lv_label_get_letter_pos
(const lv_obj_t *label, uint32_t index, lv_point_t *pos)¶ Get the relative x and y coordinates of a letter
- Parameters
label -- pointer to a label object
index -- index of the letter [0 ... text length]. Expressed in character index, not byte index (different in UTF-8)
pos -- store the result here (E.g. index = 0 gives 0;0 coordinates)
-
uint32_t
lv_label_get_letter_on
(const lv_obj_t *label, lv_point_t *pos)¶ Get the index of letter on a relative point of a label
- Parameters
label -- pointer to label object
pos -- pointer to point with coordinates on a the label
- Returns
the index of the letter on the 'pos_p' point (E.g. on 0;0 is the 0. letter) Expressed in character index and not byte index (different in UTF-8)
-
bool
lv_label_is_char_under_pos
(const lv_obj_t *label, lv_point_t *pos)¶ Check if a character is drawn under a point.
- Parameters
label -- Label object
pos -- Point to check for character under
- Returns
whether a character is drawn under the point
-
uint32_t
lv_label_get_text_sel_start
(const lv_obj_t *label)¶ Get the selection start index.
- Parameters
label -- pointer to a label object.
- Returns
selection start index.
LV_LABEL_TXT_SEL_OFF
if nothing is selected.
-
uint32_t
lv_label_get_text_sel_end
(const lv_obj_t *label)¶ Get the selection end index.
- Parameters
label -- pointer to a label object.
- Returns
selection end index.
LV_LABEL_TXT_SEL_OFF
if nothing is selected.
-
lv_style_list_t *
lv_label_get_style
(lv_obj_t *label, uint8_t type)¶
-
void
lv_label_ins_text
(lv_obj_t *label, uint32_t pos, const char *txt)¶ Insert a text to the label. The label text can not be static.
- Parameters
label -- pointer to a label object
pos -- character index to insert. Expressed in character index and not byte index (Different in UTF-8) 0: before first char. LV_LABEL_POS_LAST: after last char.
txt -- pointer to the text to insert
-
void
lv_label_cut_text
(lv_obj_t *label, uint32_t pos, uint32_t cnt)¶ Delete characters from a label. The label text can not be static.
- Parameters
label -- pointer to a label object
pos -- character index to insert. Expressed in character index and not byte index (Different in UTF-8) 0: before first char.
cnt -- number of characters to cut
-
struct
lv_label_ext_t
¶ - #include <lv_label.h>
Data of label
Public Members
-
char *
text
¶
-
char *
tmp_ptr
¶
-
char
tmp
[LV_LABEL_DOT_NUM
+ 1]¶
-
union lv_label_ext_t::[anonymous]
dot
¶
-
uint32_t
dot_end
¶
-
uint16_t
anim_speed
¶
-
lv_point_t
offset
¶
-
lv_draw_label_hint_t
hint
¶
-
uint32_t
sel_start
¶
-
uint32_t
sel_end
¶
-
lv_label_long_mode_t
long_mode
¶
-
uint8_t
static_txt
¶
-
uint8_t
align
¶
-
uint8_t
recolor
¶
-
uint8_t
expand
¶
-
uint8_t
dot_tmp_alloc
¶
-
char *