Roller (lv_roller)¶
Overview¶
Roller allows you to simply select one option from more with scrolling.
Parts and Styles¶
The Roller's main part is called LV_ROLLER_PART_BG
. It's a rectangle and uses all the typical background properties.
The style of the Roller's label is inherited from the text style properties of the background.
To adjust the space between the options use the text_line_space style property.
The padding style properties set the space on the sides.
The selected option in the middle can be referenced with LV_ROLLER_PART_SELECTED
virtual part. Besides the typical background properties it uses the text properties to change the appearance of the text in the selected area.
Usage¶
Set options¶
The options are passed to the Roller as a string with lv_roller_set_options(roller, options, LV_ROLLER_MODE_NORMAL/INFINITE)
. The options should be separated by \n
. For example: "First\nSecond\nThird"
.
LV_ROLLER_MODE_INFINITE
make the roller circular.
You can select an option manually with lv_roller_set_selected(roller, id, LV_ANIM_ON/OFF)
, where id is the index of an option.
Get selected option¶
The get the currently selected option use lv_roller_get_selected(roller)
it will return the index of the selected option.
lv_roller_get_selected_str(roller, buf, buf_size)
copy the name of the selected option to buf
.
Align the options¶
To align the label horizontally use lv_roller_set_align(roller, LV_LABEL_ALIGN_LEFT/CENTER/RIGHT)
.
Visible rows¶
The number of visible rows can be adjusted with lv_roller_set_visible_row_count(roller, num)
Animation time¶
When the Roller is scrolled and doesn't stop exactly on an option it will scroll to the nearest valid option automatically.
The time of this scroll animation can be changed by lv_roller_set_anim_time(roller, anim_time)
. Zero animation time means no animation.
Events¶
Besides, the Generic events the following Special events are sent by the Drop down lists:
LV_EVENT_VALUE_CHANGED sent when a new option is selected
Learn more about Events.
Keys¶
The following Keys are processed by the Buttons:
LV_KEY_RIGHT/DOWN Select the next option
LV_KEY_LEFT/UP Select the previous option
LY_KEY_ENTER Apply the selected option (Send
LV_EVENT_VALUE_CHANGED
event)
Example¶
C¶
Simple Roller¶
code
#include "../../../lv_examples.h"
#include <stdio.h>
#if LV_USE_ROLLER
static void event_handler(lv_obj_t * obj, lv_event_t event)
{
if(event == LV_EVENT_VALUE_CHANGED) {
char buf[32];
lv_roller_get_selected_str(obj, buf, sizeof(buf));
printf("Selected month: %s\n", buf);
}
}
void lv_ex_roller_1(void)
{
lv_obj_t *roller1 = lv_roller_create(lv_scr_act(), NULL);
lv_roller_set_options(roller1,
"January\n"
"February\n"
"March\n"
"April\n"
"May\n"
"June\n"
"July\n"
"August\n"
"September\n"
"October\n"
"November\n"
"December",
LV_ROLLER_MODE_INFINITE);
lv_roller_set_visible_row_count(roller1, 4);
lv_obj_align(roller1, NULL, LV_ALIGN_CENTER, 0, 0);
lv_obj_set_event_cb(roller1, event_handler);
}
#endif
MicroPython¶
Simple Roller¶
Click to try in the simulator!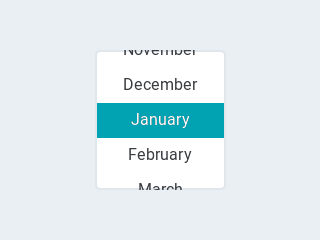
code
def event_handler(source,evt):
if evt == lv.EVENT.VALUE_CHANGED:
month=" "*10
roller.get_selected_str(month,len(month))
print("Selected month: ",month)
roller = lv.roller(lv.scr_act(),None)
roller.set_options("January\n"
"February\n"
"March\n"
"April\n"
"May\n"
"June\n"
"July\n"
"August\n"
"September\n"
"October\n"
"November\n"
"December",
lv.roller.MODE.INFINITE)
roller.set_visible_row_count(4)
roller.align(None,lv.ALIGN.CENTER,0,0)
roller.set_event_cb(event_handler)
API¶
Enums
Functions
-
lv_obj_t *
lv_roller_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a roller object
- Parameters
par -- pointer to an object, it will be the parent of the new roller
copy -- pointer to a roller object, if not NULL then the new object will be copied from it
- Returns
pointer to the created roller
-
void
lv_roller_set_options
(lv_obj_t *roller, const char *options, lv_roller_mode_t mode)¶ Set the options on a roller
- Parameters
roller -- pointer to roller object
options --
a string with '
' separated options. E.g. "One\nTwo\nThree"
mode --
LV_ROLLER_MODE_NORMAL
orLV_ROLLER_MODE_INFINITE
-
void
lv_roller_set_align
(lv_obj_t *roller, lv_label_align_t align)¶ Set the align of the roller's options (left, right or center[default])
- Parameters
roller -- - pointer to a roller object
align -- - one of lv_label_align_t values (left, right, center)
-
void
lv_roller_set_selected
(lv_obj_t *roller, uint16_t sel_opt, lv_anim_enable_t anim)¶ Set the selected option
- Parameters
roller -- pointer to a roller object
sel_opt -- id of the selected option (0 ... number of option - 1);
anim -- LV_ANIM_ON: set with animation; LV_ANIM_OFF set immediately
-
void
lv_roller_set_visible_row_count
(lv_obj_t *roller, uint8_t row_cnt)¶ Set the height to show the given number of rows (options)
- Parameters
roller -- pointer to a roller object
row_cnt -- number of desired visible rows
-
void
lv_roller_set_auto_fit
(lv_obj_t *roller, bool auto_fit)¶ Allow automatically setting the width of roller according to it's content.
- Parameters
roller -- pointer to a roller object
auto_fit -- true: enable auto fit
-
static inline void
lv_roller_set_anim_time
(lv_obj_t *roller, uint16_t anim_time)¶ Set the open/close animation time.
- Parameters
roller -- pointer to a roller object
anim_time -- open/close animation time [ms]
-
uint16_t
lv_roller_get_selected
(const lv_obj_t *roller)¶ Get the id of the selected option
- Parameters
roller -- pointer to a roller object
- Returns
id of the selected option (0 ... number of option - 1);
-
uint16_t
lv_roller_get_option_cnt
(const lv_obj_t *roller)¶ Get the total number of options
- Parameters
roller -- pointer to a roller object
- Returns
the total number of options in the list
-
void
lv_roller_get_selected_str
(const lv_obj_t *roller, char *buf, uint32_t buf_size)¶ Get the current selected option as a string
- Parameters
roller -- pointer to roller object
buf -- pointer to an array to store the string
buf_size -- size of
buf
in bytes. 0: to ignore it.
-
lv_label_align_t
lv_roller_get_align
(const lv_obj_t *roller)¶ Get the align attribute. Default alignment after _create is LV_LABEL_ALIGN_CENTER
- Parameters
roller -- pointer to a roller object
- Returns
LV_LABEL_ALIGN_LEFT, LV_LABEL_ALIGN_RIGHT or LV_LABEL_ALIGN_CENTER
-
bool
lv_roller_get_auto_fit
(lv_obj_t *roller)¶ Get whether the auto fit option is enabled or not.
- Parameters
roller -- pointer to a roller object
- Returns
true: auto fit is enabled
-
struct
lv_roller_ext_t
¶ Public Members
-
lv_page_ext_t
page
¶
-
lv_style_list_t
style_sel
¶
-
uint16_t
option_cnt
¶
-
uint16_t
sel_opt_id
¶
-
uint16_t
sel_opt_id_ori
¶
-
lv_roller_mode_t
mode
¶
-
uint8_t
auto_fit
¶
-
lv_page_ext_t