Bar (lv_bar)¶
Overview¶
The bar object has a background and an indicator on it. The width of the indicator is set according to the current value of the bar.
Vertical bars can be created if the width of the object is smaller than its height.
Not only end, but the start value of the bar can be set which changes the start position of the indicator.
Parts and Styles¶
The Bar's main part is called LV_BAR_PART_BG
and it uses the typical background style properties.
LV_BAR_PART_INDIC
is a virtual part which also uses all the typical background properties.
By default the indicator maximal size is the same as the background's size but setting positive padding values in LV_BAR_PART_BG
will make the indicator smaller. (negative values will make it larger)
If the value style property is used on the indicator the alignment will be calculated based on the current size of the indicator.
For example a center aligned value is always shown in the middle of the indicator regardless it's current size.
Usage¶
Value and range¶
A new value can be set by lv_bar_set_value(bar, new_value, LV_ANIM_ON/OFF)
.
The value is interpreted in a range (minimum and maximum values) which can be modified with lv_bar_set_range(bar, min, max)
.
The default range is 1..100.
The new value in lv_bar_set_value
can be set with or without an animation depending on the last parameter (LV_ANIM_ON/OFF
).
The time of the animation can be adjusted by lv_bar_set_anim_time(bar, 100)
. The time is in milliseconds unit.
It's also possible to set the start value of the bar using lv_bar_set_start_value(bar, new_value, LV_ANIM_ON/OFF)
Modes¶
The bar can be drawn symmetrical to zero (drawn from zero, left to right), if it's enabled with lv_bar_set_type(bar, LV_BAR_TYPE_SYMMETRICAL)
.
Example¶
C¶
Simple Bar¶
code
#include "../../../lv_examples.h"
#if LV_USE_BAR
void lv_ex_bar_1(void)
{
lv_obj_t * bar1 = lv_bar_create(lv_scr_act(), NULL);
lv_obj_set_size(bar1, 200, 20);
lv_obj_align(bar1, NULL, LV_ALIGN_CENTER, 0, 0);
lv_bar_set_anim_time(bar1, 2000);
lv_bar_set_value(bar1, 100, LV_ANIM_ON);
}
#endif
MicroPython¶
Click to try in the simulator!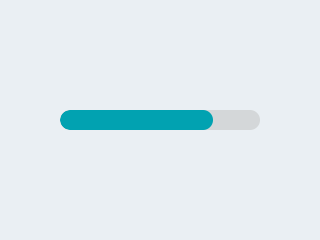
code
def event_handler(source,evt):
if evt == lv.EVENT.VALUE_CHANGED:
if source.is_checked():
print("State: checked")
else:
print("State: unchecked")
# create a simple bar
bar1 = lv.bar(lv.scr_act(),None)
bar1.set_size(200,20)
bar1.align(None,lv.ALIGN.CENTER,0,0)
bar1.set_anim_time(2000)
bar1.set_value(100,lv.ANIM.ON)
API¶
Enums
Functions
-
lv_obj_t *
lv_bar_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a bar objects
- Parameters
par -- pointer to an object, it will be the parent of the new bar
copy -- pointer to a bar object, if not NULL then the new object will be copied from it
- Returns
pointer to the created bar
-
void
lv_bar_set_value
(lv_obj_t *bar, int16_t value, lv_anim_enable_t anim)¶ Set a new value on the bar
- Parameters
bar -- pointer to a bar object
value -- new value
anim -- LV_ANIM_ON: set the value with an animation; LV_ANIM_OFF: change the value immediately
-
void
lv_bar_set_start_value
(lv_obj_t *bar, int16_t start_value, lv_anim_enable_t anim)¶ Set a new start value on the bar
- Parameters
bar -- pointer to a bar object
value -- new start value
anim -- LV_ANIM_ON: set the value with an animation; LV_ANIM_OFF: change the value immediately
-
void
lv_bar_set_range
(lv_obj_t *bar, int16_t min, int16_t max)¶ Set minimum and the maximum values of a bar
- Parameters
bar -- pointer to the bar object
min -- minimum value
max -- maximum value
-
void
lv_bar_set_type
(lv_obj_t *bar, lv_bar_type_t type)¶ Set the type of bar.
- Parameters
bar -- pointer to bar object
type -- bar type
-
void
lv_bar_set_anim_time
(lv_obj_t *bar, uint16_t anim_time)¶ Set the animation time of the bar
- Parameters
bar -- pointer to a bar object
anim_time -- the animation time in milliseconds.
-
int16_t
lv_bar_get_value
(const lv_obj_t *bar)¶ Get the value of a bar
- Parameters
bar -- pointer to a bar object
- Returns
the value of the bar
-
int16_t
lv_bar_get_start_value
(const lv_obj_t *bar)¶ Get the start value of a bar
- Parameters
bar -- pointer to a bar object
- Returns
the start value of the bar
-
int16_t
lv_bar_get_min_value
(const lv_obj_t *bar)¶ Get the minimum value of a bar
- Parameters
bar -- pointer to a bar object
- Returns
the minimum value of the bar
-
int16_t
lv_bar_get_max_value
(const lv_obj_t *bar)¶ Get the maximum value of a bar
- Parameters
bar -- pointer to a bar object
- Returns
the maximum value of the bar
-
lv_bar_type_t
lv_bar_get_type
(lv_obj_t *bar)¶ Get the type of bar.
- Parameters
bar -- pointer to bar object
- Returns
bar type
-
struct
lv_bar_anim_t
¶
-
struct
lv_bar_ext_t
¶ - #include <lv_bar.h>
Data of bar
Public Members
-
int16_t
cur_value
¶
-
int16_t
min_value
¶
-
int16_t
max_value
¶
-
int16_t
start_value
¶
-
lv_area_t
indic_area
¶
-
lv_anim_value_t
anim_time
¶
-
lv_bar_anim_t
cur_value_anim
¶
-
lv_bar_anim_t
start_value_anim
¶
-
uint8_t
type
¶
-
lv_style_list_t
style_indic
¶
-
int16_t