Gauge (lv_gauge)¶
Overview¶
The gauge is a meter with scale labels and one or more needles.
Parts and Styles¶
The Gauge's main part is called LV_GAUGE_PART_MAIN
. It draws a background using the typical background style properties and "minor" scale lines using the line and scale style properties.
It also uses the text properties to set the style of the scale labels. pad_inner is used to set space between the scale lines and the scale labels.
LV_GAUGE_PART_MAJOR
is a virtual part which describes the major scale lines (where labels are added) using the line and scale style properties.
LV_GAUGE_PART_NEEDLE
is also virtual part and it describes the needle(s) via the line style properties.
The size and the typical background properties are used to describe a rectangle (or circle) in the pivot point of the needle(s).
pad_inner is used to to make the needle(s) smaller than the outer radius of the scale lines.
Usage¶
Set value and needles¶
The gauge can show more than one needle.
Use the lv_gauge_set_needle_count(gauge, needle_num, color_array)
function to set the number of needles and an array with colors for each needle.
The array must be static or global variable because only its pointer is stored.
You can use lv_gauge_set_value(gauge, needle_id, value)
to set the value of a needle.
Scale¶
You can use the lv_gauge_set_scale(gauge, angle, line_num, label_cnt)
function to adjust the scale angle and the number of the scale lines and labels.
The default settings are 220 degrees, 6 scale labels, and 21 lines.
The scale of the Gauge can have offset. It can be adjusted with lv_gauge_set_angle_offset(gauge, angle)
.
Range¶
The range of the gauge can be specified by lv_gauge_set_range(gauge, min, max)
. The default range is 0..100.
Needle image¶
An images also can be used as needles. The image should point to the right (like ==>
). To set an image use lv_gauge_set_needle_img(gauge1, &img, pivot_x, pivot_y)
. pivot_x
and pivot_y
are offset of the rotation center from the top left corner. Images will be recolored to the needle's color with image_recolor_opa
intensity coming from the styles in LV_GAUGE_PART_NEEDLE
.
Critical value¶
To set a critical value, use lv_gauge_set_critical_value(gauge, value)
. The scale color will be changed to scale_end_color after this value. The default critical value is 80.
Example¶
C¶
Simple Gauge¶
code
#include "../../../lv_examples.h"
#if LV_USE_GAUGE
void lv_ex_gauge_1(void)
{
/*Describe the color for the needles*/
static lv_color_t needle_colors[3];
needle_colors[0] = LV_COLOR_BLUE;
needle_colors[1] = LV_COLOR_ORANGE;
needle_colors[2] = LV_COLOR_PURPLE;
/*Create a gauge*/
lv_obj_t * gauge1 = lv_gauge_create(lv_scr_act(), NULL);
lv_gauge_set_needle_count(gauge1, 3, needle_colors);
lv_obj_set_size(gauge1, 200, 200);
lv_obj_align(gauge1, NULL, LV_ALIGN_CENTER, 0, 0);
/*Set the values*/
lv_gauge_set_value(gauge1, 0, 10);
lv_gauge_set_value(gauge1, 1, 20);
lv_gauge_set_value(gauge1, 2, 30);
}
#endif
code
#include "../../../lv_examples.h"
#if LV_USE_GAUGE
void lv_ex_gauge_2(void)
{
/*Describe the color for the needles*/
static lv_color_t needle_colors[3];
needle_colors[0] = LV_COLOR_BLUE;
needle_colors[1] = LV_COLOR_ORANGE;
needle_colors[2] = LV_COLOR_PURPLE;
LV_IMG_DECLARE(img_hand);
/*Create a gauge*/
lv_obj_t * gauge1 = lv_gauge_create(lv_scr_act(), NULL);
lv_gauge_set_needle_count(gauge1, 3, needle_colors);
lv_obj_set_size(gauge1, 200, 200);
lv_obj_align(gauge1, NULL, LV_ALIGN_CENTER, 0, 0);
lv_gauge_set_needle_img(gauge1, &img_hand, 4, 4);
/*Allow recoloring of the images according to the needles' color*/
lv_obj_set_style_local_image_recolor_opa(gauge1, LV_GAUGE_PART_NEEDLE, LV_STATE_DEFAULT, LV_OPA_COVER);
/*Set the values*/
lv_gauge_set_value(gauge1, 0, 10);
lv_gauge_set_value(gauge1, 1, 20);
lv_gauge_set_value(gauge1, 2, 30);
}
#endif
MicroPython¶
Click to try in the simulator!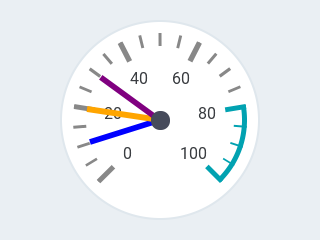
code
from lv_colors import lv_colors
# needle colors
needle_colors=[lv_colors.BLUE,lv_colors.ORANGE,lv_colors.PURPLE]
# create the gauge
gauge1=lv.gauge(lv.scr_act(),None)
gauge1.set_needle_count(3, needle_colors)
gauge1.set_size(200,200)
gauge1.align(None,lv.ALIGN.CENTER,0,0)
# Set the values
gauge1.set_value(0, 10)
gauge1.set_value(1, 20)
gauge1.set_value(2, 50)
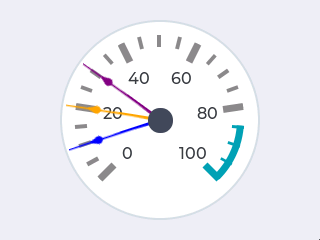
code
import usys as sys
from lv_colors import lv_colors
try:
with open('../../../assets/img_hand_argb.bin','rb') as f:
hand_img_data = f.read()
except:
try:
with open('images/img_hand_rgb565.bin','rb') as f:
hand_img_data = f.read()
except:
print("Could not find img_hand file")
sys.exit()
# create the hands image data
img_hand_dsc = lv.img_dsc_t(
{
"header": {"always_zero": 0, "w": 100, "h": 9, "cf": lv.img.CF.TRUE_COLOR_ALPHA},
"data": hand_img_data,
"data_size": len(hand_img_data),
}
)
# needle colors
needle_colors=[lv_colors.BLUE,lv_colors.ORANGE,lv_colors.PURPLE]
# create the gauge
gauge1=lv.gauge(lv.scr_act(),None)
gauge1.set_needle_count(3, needle_colors)
gauge1.set_size(200,200)
gauge1.align(None,lv.ALIGN.CENTER,0,0)
gauge1.set_needle_img(img_hand_dsc, 4, 4)
gauge1.set_style_local_image_recolor_opa(lv.gauge.PART.NEEDLE, lv.STATE.DEFAULT, lv.OPA.COVER)
# Set the values
gauge1.set_value(0, 10)
gauge1.set_value(1, 20)
gauge1.set_value(2, 30)
API¶
Typedefs
-
typedef uint8_t
lv_gauge_style_t
¶
Enums
Functions
-
lv_obj_t *
lv_gauge_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a gauge objects
- Parameters
par -- pointer to an object, it will be the parent of the new gauge
copy -- pointer to a gauge object, if not NULL then the new object will be copied from it
- Returns
pointer to the created gauge
-
void
lv_gauge_set_needle_count
(lv_obj_t *gauge, uint8_t needle_cnt, const lv_color_t colors[])¶ Set the number of needles
- Parameters
gauge -- pointer to gauge object
needle_cnt -- new count of needles
colors -- an array of colors for needles (with 'num' elements)
-
void
lv_gauge_set_value
(lv_obj_t *gauge, uint8_t needle_id, int32_t value)¶ Set the value of a needle
- Parameters
gauge -- pointer to a gauge
needle_id -- the id of the needle
value -- the new value
-
static inline void
lv_gauge_set_range
(lv_obj_t *gauge, int32_t min, int32_t max)¶ Set minimum and the maximum values of a gauge
- Parameters
gauge -- pointer to he gauge object
min -- minimum value
max -- maximum value
-
static inline void
lv_gauge_set_critical_value
(lv_obj_t *gauge, int32_t value)¶ Set a critical value on the scale. After this value 'line.color' scale lines will be drawn
- Parameters
gauge -- pointer to a gauge object
value -- the critical value
-
void
lv_gauge_set_scale
(lv_obj_t *gauge, uint16_t angle, uint8_t line_cnt, uint8_t label_cnt)¶ Set the scale settings of a gauge
- Parameters
gauge -- pointer to a gauge object
angle -- angle of the scale (0..360)
line_cnt -- count of scale lines. To get a given "subdivision" lines between labels:
line_cnt = (sub_div + 1) * (label_cnt - 1) + 1
label_cnt -- count of scale labels.
-
static inline void
lv_gauge_set_angle_offset
(lv_obj_t *gauge, uint16_t angle)¶ Set the set an offset for the gauge's angles to rotate it.
- Parameters
gauge -- pointer to a line meter object
angle -- angle offset (0..360), rotates clockwise
-
void
lv_gauge_set_needle_img
(lv_obj_t *gauge, const void *img, lv_coord_t pivot_x, lv_coord_t pivot_y)¶ Set an image to display as needle(s). The needle image should be horizontal and pointing to the right (
--->
).- Parameters
gauge -- pointer to a gauge object
img_src -- pointer to an
lv_img_dsc_t
variable or a path to an image (not anlv_img
object)pivot_x -- the X coordinate of rotation center of the image
pivot_y -- the Y coordinate of rotation center of the image
-
void
lv_gauge_set_formatter_cb
(lv_obj_t *gauge, lv_gauge_format_cb_t format_cb)¶ Assign a function to format gauge values
- Parameters
gauge -- pointer to a gauge object
format_cb -- pointer to function of lv_gauge_format_cb_t
-
int32_t
lv_gauge_get_value
(const lv_obj_t *gauge, uint8_t needle)¶ Get the value of a needle
- Parameters
gauge -- pointer to gauge object
needle -- the id of the needle
- Returns
the value of the needle [min,max]
-
uint8_t
lv_gauge_get_needle_count
(const lv_obj_t *gauge)¶ Get the count of needles on a gauge
- Parameters
gauge -- pointer to gauge
- Returns
count of needles
-
static inline int32_t
lv_gauge_get_min_value
(const lv_obj_t *lmeter)¶ Get the minimum value of a gauge
- Parameters
gauge -- pointer to a gauge object
- Returns
the minimum value of the gauge
-
static inline int32_t
lv_gauge_get_max_value
(const lv_obj_t *lmeter)¶ Get the maximum value of a gauge
- Parameters
gauge -- pointer to a gauge object
- Returns
the maximum value of the gauge
-
static inline int32_t
lv_gauge_get_critical_value
(const lv_obj_t *gauge)¶ Get a critical value on the scale.
- Parameters
gauge -- pointer to a gauge object
- Returns
the critical value
-
uint8_t
lv_gauge_get_label_count
(const lv_obj_t *gauge)¶ Set the number of labels (and the thicker lines too)
- Parameters
gauge -- pointer to a gauge object
- Returns
count of labels
-
static inline uint16_t
lv_gauge_get_line_count
(const lv_obj_t *gauge)¶ Get the scale number of a gauge
- Parameters
gauge -- pointer to a gauge object
- Returns
number of the scale units
-
static inline uint16_t
lv_gauge_get_scale_angle
(const lv_obj_t *gauge)¶ Get the scale angle of a gauge
- Parameters
gauge -- pointer to a gauge object
- Returns
angle of the scale
-
static inline uint16_t
lv_gauge_get_angle_offset
(lv_obj_t *gauge)¶ Get the offset for the gauge.
- Parameters
gauge -- pointer to a gauge object
- Returns
angle offset (0..360)
-
const void *
lv_gauge_get_needle_img
(lv_obj_t *gauge)¶ Get an image to display as needle(s).
- Parameters
gauge -- pointer to a gauge object
- Returns
pointer to an
lv_img_dsc_t
variable or a path to an image (not anlv_img
object).NULL
if not used.
-
struct
lv_gauge_ext_t
¶ Public Members
-
lv_linemeter_ext_t
lmeter
¶
-
int32_t *
values
¶
-
const lv_color_t *
needle_colors
¶
-
const void *
needle_img
¶
-
lv_point_t
needle_img_pivot
¶
-
lv_style_list_t
style_needle
¶
-
lv_style_list_t
style_strong
¶
-
uint8_t
needle_count
¶
-
uint8_t
label_count
¶
-
lv_gauge_format_cb_t
format_cb
¶
-
lv_linemeter_ext_t