Container (lv_cont)¶
Overview¶
The containers are essentially a basic object with layout and automatic sizing features.
Parts and Styles¶
The containers has only a main style called LV_CONT_PART_MAIN
and it can use all the typicaly bacground properties and padding for layout auto sizing.
Usage¶
Layout¶
You can apply a layout on the containers to automatically order their children. The layout spacing comes from the style's pad
properties. The possible layout options:
LV_LAYOUT_OFF - Do not align the children.
LV_LAYOUT_CENTER - Align children to the center in column and keep
pad_inner
space between them.LV_LAYOUT_COLUMN_LEFT - Align children in a left-justified column. Keep
pad_left
space on the left,pad_top
space on the top andpad_inner
space between the children.LV_LAYOUT_COLUMN_MID - Align children in centered column. Keep
pad_top
space on the top andpad_inner
space between the children.LV_LAYOUT_COLUMN_RIGHT - Align children in a right-justified column. Keep
pad_right
space on the right,pad_top
space on the top andpad_inner
space between the children.LV_LAYOUT_ROW_TOP - Align children in a top justified row. Keep
pad_left
space on the left,pad_top
space on the top andpad_inner
space between the children.LV_LAYOUT_ROW_MID - Align children in centered row. Keep
pad_left
space on the left andpad_inner
space between the children.LV_LAYOUT_ROW_BOTTOM - Align children in a bottom justified row. Keep
pad_left
space on the left,pad_bottom
space on the bottom andpad_inner
space between the children.LV_LAYOUT_PRETTY_TOP - Put as many objects as possible in a row (with at least
pad_inner
space andpad_left/right
space on the sides). Divide the space in each line equally between the children. If here are children with different height in a row align their top edge.LV_LAYOUT_PRETTY_MID - Same as
LV_LAYOUT_PRETTY_TOP
but if here are children with different height in a row align their middle line.LV_LAYOUT_PRETTY_BOTTOM - Same as
LV_LAYOUT_PRETTY_TOP
but if here are children with different height in a row align their bottom line.LV_LAYOUT_GRID - Similar to
LV_LAYOUT_PRETTY
but not divide horizontal space equally just letpad_left/right
on the edges andpad_inner
space between the elements.
Autofit¶
Container have an autofit feature which can automatically change the size of the container according to its children and/or its parent. The following options exist:
LV_FIT_NONE - Do not change the size automatically.
LV_FIT_TIGHT - Shrink-wrap the container around all of its children, while keeping
pad_top/bottom/left/right
space on the edges.LV_FIT_PARENT - Set the size to the parent's size minus
pad_top/bottom/left/right
(from the parent's style) space.LV_FIT_MAX - Use
LV_FIT_PARENT
while smaller than the parent andLV_FIT_TIGHT
when larger. It will ensure that the container is, at minimum, the size of its parent.
To set the auto fit mode for all directions, use lv_cont_set_fit(cont, LV_FIT_...)
.
To use different auto fit horizontally and vertically, use lv_cont_set_fit2(cont, hor_fit_type, ver_fit_type)
.
To use different auto fit in all 4 directions, use lv_cont_set_fit4(cont, left_fit_type, right_fit_type, top_fit_type, bottom_fit_type)
.
Example¶
C¶
Container with auto-fit¶
code
#include "../../../lv_examples.h"
#if LV_USE_CONT
void lv_ex_cont_1(void)
{
lv_obj_t * cont;
cont = lv_cont_create(lv_scr_act(), NULL);
lv_obj_set_auto_realign(cont, true); /*Auto realign when the size changes*/
lv_obj_align_origo(cont, NULL, LV_ALIGN_CENTER, 0, 0); /*This parametrs will be sued when realigned*/
lv_cont_set_fit(cont, LV_FIT_TIGHT);
lv_cont_set_layout(cont, LV_LAYOUT_COLUMN_MID);
lv_obj_t * label;
label = lv_label_create(cont, NULL);
lv_label_set_text(label, "Short text");
/*Refresh and pause here for a while to see how `fit` works*/
uint32_t t;
lv_refr_now(NULL);
t = lv_tick_get();
while(lv_tick_elaps(t) < 500);
label = lv_label_create(cont, NULL);
lv_label_set_text(label, "It is a long text");
/*Wait here too*/
lv_refr_now(NULL);
t = lv_tick_get();
while(lv_tick_elaps(t) < 500);
label = lv_label_create(cont, NULL);
lv_label_set_text(label, "Here is an even longer text");
}
#endif
MicroPython¶
Container with auto-fit¶
Click to try in the simulator!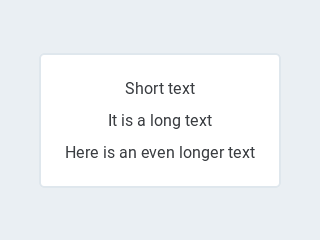
code
# create a container
cont = lv.cont(lv.scr_act(),None)
cont.set_auto_realign(True) # Auto realign when the size changes
cont.align_mid(None,lv.ALIGN.CENTER,0,0) # This parameters will be sued when realigned
cont.set_fit(lv.FIT.TIGHT)
cont.set_layout(lv.LAYOUT.COLUMN_MID);
label = lv.label(cont)
label.set_text("Short text")
# Refresh and pause here for a while to see how `fit` works
time.sleep(1)
label = lv.label(cont)
label.set_text("It is a long text")
# Wait here too
time.sleep(1)
label = lv.label(cont)
label.set_text("Here an even longer text")
API¶
Enums
-
enum [anonymous]¶
Container layout options
Values:
-
enumerator
LV_LAYOUT_OFF
¶ No layout
-
enumerator
LV_LAYOUT_CENTER
¶ Center objects
-
enumerator
LV_LAYOUT_COLUMN_LEFT
¶ COLUMN:
Place the object below each other
Keep
pad_top
space on the topKeep
pad_inner
space between the objectsColumn left align
-
enumerator
LV_LAYOUT_COLUMN_MID
¶ Column middle align
-
enumerator
LV_LAYOUT_COLUMN_RIGHT
¶ Column right align
-
enumerator
LV_LAYOUT_ROW_TOP
¶ ROW:
Place the object next to each other
Keep
pad_left
space on the leftKeep
pad_inner
space between the objectsIf the object which applies the layout has
base_dir == LV_BIDI_DIR_RTL
the row will start from the right applyingpad.right
spaceRow top align
-
enumerator
LV_LAYOUT_ROW_MID
¶ Row middle align
-
enumerator
LV_LAYOUT_ROW_BOTTOM
¶ Row bottom align
-
enumerator
LV_LAYOUT_PRETTY_TOP
¶ PRETTY:
Place the object next to each other
If there is no more space start a new row
Respect
pad_left
andpad_right
when determining the available space in a rowKeep
pad_inner
space between the objects in the same rowKeep
pad_inner
space between the objects in rowsDivide the remaining horizontal space equallyRow top align
-
enumerator
LV_LAYOUT_PRETTY_MID
¶ Row middle align
-
enumerator
LV_LAYOUT_PRETTY_BOTTOM
¶ Row bottom align
-
enumerator
LV_LAYOUT_GRID
¶ GRID
Place the object next to each other
If there is no more space start a new row
Respect
pad_left
andpad_right
when determining the available space in a rowKeep
pad_inner
space between the objects in the same rowKeep
pad_inner
space between the objects in rowsUnlike
PRETTY
,GRID
always keeppad_inner
space horizontally between objects so it doesn't divide the remaining horizontal space equallyAlign same-sized object into a grid
-
enumerator
_LV_LAYOUT_LAST
¶
-
enumerator
-
enum [anonymous]¶
How to resize the container around the children.
Values:
-
enumerator
LV_FIT_NONE
¶ Do not change the size automatically
-
enumerator
LV_FIT_TIGHT
¶ Shrink wrap around the children
-
enumerator
LV_FIT_PARENT
¶ Align the size to the parent's edge
-
enumerator
LV_FIT_MAX
¶ Align the size to the parent's edge first but if there is an object out of it then get larger
-
enumerator
_LV_FIT_LAST
¶
-
enumerator
Functions
-
lv_obj_t *
lv_cont_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a container objects
- Parameters
par -- pointer to an object, it will be the parent of the new container
copy -- pointer to a container object, if not NULL then the new object will be copied from it
- Returns
pointer to the created container
-
void
lv_cont_set_layout
(lv_obj_t *cont, lv_layout_t layout)¶ Set a layout on a container
- Parameters
cont -- pointer to a container object
layout -- a layout from 'lv_cont_layout_t'
-
void
lv_cont_set_fit4
(lv_obj_t *cont, lv_fit_t left, lv_fit_t right, lv_fit_t top, lv_fit_t bottom)¶ Set the fit policy in all 4 directions separately. It tell how to change the container's size automatically.
- Parameters
cont -- pointer to a container object
left -- left fit policy from
lv_fit_t
right -- right fit policy from
lv_fit_t
top -- top fit policy from
lv_fit_t
bottom -- bottom fit policy from
lv_fit_t
-
static inline void
lv_cont_set_fit2
(lv_obj_t *cont, lv_fit_t hor, lv_fit_t ver)¶ Set the fit policy horizontally and vertically separately. It tells how to change the container's size automatically.
- Parameters
cont -- pointer to a container object
hor -- horizontal fit policy from
lv_fit_t
ver -- vertical fit policy from
lv_fit_t
-
static inline void
lv_cont_set_fit
(lv_obj_t *cont, lv_fit_t fit)¶ Set the fit policy in all 4 direction at once. It tells how to change the container's size automatically.
- Parameters
cont -- pointer to a container object
fit -- fit policy from
lv_fit_t
-
lv_layout_t
lv_cont_get_layout
(const lv_obj_t *cont)¶ Get the layout of a container
- Parameters
cont -- pointer to container object
- Returns
the layout from 'lv_cont_layout_t'
-
lv_fit_t
lv_cont_get_fit_left
(const lv_obj_t *cont)¶ Get left fit mode of a container
- Parameters
cont -- pointer to a container object
- Returns
an element of
lv_fit_t
-
lv_fit_t
lv_cont_get_fit_right
(const lv_obj_t *cont)¶ Get right fit mode of a container
- Parameters
cont -- pointer to a container object
- Returns
an element of
lv_fit_t
-
struct
lv_cont_ext_t
¶