Line (lv_line)¶
Overview¶
The Line object is capable of drawing straight lines between a set of points.
Parts and Styles¶
The Line has only a main part, called LV_LABEL_PART_MAIN
. It uses all the line style properties.
Usage¶
Set points¶
The points has to be stored in an lv_point_t
array and passed to the object by the lv_line_set_points(lines, point_array, point_cnt)
function.
Auto-size¶
It is possible to automatically set the size of the line object according to its points.
It can be enable with the lv_line_set_auto_size(line, true)
function.
If enabled then when the points are set the object's width and height will be changed according to the maximal x and y coordinates among the points. The auto size is enabled by default.
Invert y¶
By deafult, the y == 0 point is in the top of the object. It might be conter-intuitive in some cases so the y coordinates can be inverted with lv_line_set_y_invert(line, true)
. In this case, y == 0 will be the bottom of teh obejct.
The y invert is disabled by default.
Example¶
C¶
Simple Line¶
code
#include "../../../lv_examples.h"
#if LV_USE_LINE
void lv_ex_line_1(void)
{
/*Create an array for the points of the line*/
static lv_point_t line_points[] = { {5, 5}, {70, 70}, {120, 10}, {180, 60}, {240, 10} };
/*Create style*/
static lv_style_t style_line;
lv_style_init(&style_line);
lv_style_set_line_width(&style_line, LV_STATE_DEFAULT, 8);
lv_style_set_line_color(&style_line, LV_STATE_DEFAULT, LV_COLOR_BLUE);
lv_style_set_line_rounded(&style_line, LV_STATE_DEFAULT, true);
/*Create a line and apply the new style*/
lv_obj_t * line1;
line1 = lv_line_create(lv_scr_act(), NULL);
lv_line_set_points(line1, line_points, 5); /*Set the points*/
lv_obj_add_style(line1, LV_LINE_PART_MAIN, &style_line); /*Set the points*/
lv_obj_align(line1, NULL, LV_ALIGN_CENTER, 0, 0);
}
#endif
MicroPython¶
Simple Line¶
Click to try in the simulator!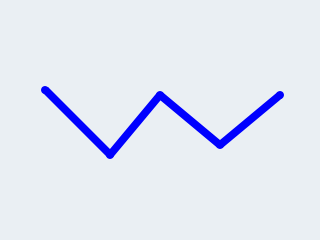
code
from lv_colors import lv_colors
# Create an array for the points of the line
line_points = [ {"x":5, "y":5},
{"x":70, "y":70},
{"x":120, "y":10},
{"x":180, "y":60},
{"x":240, "y":10}]
# Create new style (thick dark blue)
style_line = lv.style_t()
style_line.init()
style_line.set_line_width(lv.STATE.DEFAULT, 8)
style_line.set_line_color(lv.STATE.DEFAULT, lv_colors.BLUE)
style_line.set_line_rounded(lv.STATE.DEFAULT, True)
# Copy the previous line and apply the new style
line1 = lv.line(lv.scr_act())
line1.set_points(line_points, len(line_points)) # Set the points
line1.add_style(lv.line.PART.MAIN, style_line)
line1.align(None, lv.ALIGN.CENTER, 0, 0)
API¶
Typedefs
-
typedef uint8_t
lv_line_style_t
¶
Functions
-
lv_obj_t *
lv_line_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a line objects
- Parameters
par -- pointer to an object, it will be the parent of the new line
- Returns
pointer to the created line
-
void
lv_line_set_points
(lv_obj_t *line, const lv_point_t point_a[], uint16_t point_num)¶ Set an array of points. The line object will connect these points.
- Parameters
line -- pointer to a line object
point_a -- an array of points. Only the address is saved, so the array can NOT be a local variable which will be destroyed
point_num -- number of points in 'point_a'
-
void
lv_line_set_auto_size
(lv_obj_t *line, bool en)¶ Enable (or disable) the auto-size option. The size of the object will fit to its points. (set width to x max and height to y max)
- Parameters
line -- pointer to a line object
en -- true: auto size is enabled, false: auto size is disabled
-
void
lv_line_set_y_invert
(lv_obj_t *line, bool en)¶ Enable (or disable) the y coordinate inversion. If enabled then y will be subtracted from the height of the object, therefore the y=0 coordinate will be on the bottom.
- Parameters
line -- pointer to a line object
en -- true: enable the y inversion, false:disable the y inversion
-
struct
lv_line_ext_t
¶