Base object (lv_obj)¶
Overview¶
The 'Base Object' implements the basic properties of widgets on a screen, such as:
coordinates
parent object
children
main style
attributes like Click enable, Drag enable, etc.
In object-oriented thinking, it is the base class from which all other objects in LVGL are inherited. This, among another things, helps reduce code duplication.
The functions and functionalities of Base object can be used with other widgets too. For example lv_obj_set_width(slider, 100)
The Base object can be directly used as a simple widgets. It nothing else then a rectangle.
Coordinates¶
Size¶
The object size can be modified on individual axes with lv_obj_set_width(obj, new_width)
and lv_obj_set_height(obj, new_height)
, or both axes can be modified at the same time with lv_obj_set_size(obj, new_width, new_height)
.
Styles can add Margin to the objects. Margin tells that "I want this space around me".
To set width or height reduced by the margin lv_obj_set_width_margin(obj, new_width)
or lv_obj_set_height_margin(obj, new_height)
.
In more exact way: new_width = left_margin + object_width + right_margin
.
To get the width or height which includes the margins use lv_obj_get_width/height_margin(obj)
.
Styles can add Padding to the object as well. Padding means "I don't want my children too close to my sides, so keep this space".
To set width or height reduced by the padding lv_obj_set_width_fit(obj, new_width)
or lv_obj_set_height_fit(obj, new_height)
.
In a more exact way: new_width = left_pad + object_width + right_pad
To get the width or height which is REDUCED by padding use lv_obj_get_width/height_fit(obj)
. It can be considered the "useful size of the object".
Margin and padding gets important when Layout or Auto-fit is used by other widgets.
Position¶
You can set the x and y coordinates relative to the parent with lv_obj_set_x(obj, new_x)
and lv_obj_set_y(obj, new_y)
, or both at the same time with lv_obj_set_pos(obj, new_x, new_y)
.
Alignment¶
You can align the object to another with lv_obj_align(obj, obj_ref, LV_ALIGN_..., x_ofs, y_ofs)
.
obj
is the object to align.obj_ref
is a reference object.obj
will be aligned to it. Ifobj_ref = NULL
, then the parent ofobj
will be used.The third argument is the type of alignment. These are the possible options:
The alignment types build like
LV_ALIGN_OUT_TOP_MID
.The last two arguments allow you to shift the object by a specified number of pixels after aligning it.
For example, to align a text below an image: lv_obj_align(text, image, LV_ALIGN_OUT_BOTTOM_MID, 0, 10)
.
Or to align a text in the middle of its parent: lv_obj_align(text, NULL, LV_ALIGN_CENTER, 0, 0)
.
lv_obj_align_origo
works similarly to lv_obj_align
but it aligns the center of the object.
For example, lv_obj_align_origo(btn, image, LV_ALIGN_OUT_BOTTOM_MID, 0, 0)
will align the center of the button the bottom of the image.
The parameters of the alignment will be saved in the object if LV_USE_OBJ_REALIGN
is enabled in lv_conf.h. You can then realign the objects simply by calling lv_obj_realign(obj)
.
It's equivalent to calling lv_obj_align
again with the same parameters.
If the alignment happened with lv_obj_align_origo
, then it will be used when the object is realigned.
The lv_obj_align_x/y
and lv_obj_align_origo_x/y
function can be used t align only on one axis.
If lv_obj_set_auto_realign(obj, true)
is used the object will be realigned automatically, if its size changes in lv_obj_set_width/height/size()
functions.
It's very useful when size animations are applied to the object and the original position needs to be kept.
Note that the coordinates of screens can't be changed. Attempting to use these functions on screens will result in undefined behavior.
Parents and children¶
You can set a new parent for an object with lv_obj_set_parent(obj, new_parent)
. To get the current parent, use lv_obj_get_parent(obj)
.
To get the children of an object, use lv_obj_get_child(obj, child_prev)
(from last to first) or lv_obj_get_child_back(obj, child_prev)
(from first to last).
To get the first child, pass NULL
as the second parameter and use the return value to iterate through the children. The function will return NULL
if there are no more children. For example:
lv_obj_t * child = lv_obj_get_child(parent, NULL);
while(child) {
/*Do something with "child" */
child = lv_obj_get_child(parent, child);
}
lv_obj_count_children(obj)
tells the number of children on an object. lv_obj_count_children_recursive(obj)
also tells the number of children but counts children of children recursively.
Screens¶
When you have created a screen like lv_obj_t * screen = lv_obj_create(NULL, NULL)
, you can load it with lv_scr_load(screen)
. The lv_scr_act()
function gives you a pointer to the current screen.
If you have more display then it's important to know that these functions operate on the lastly created or the explicitly selected (with lv_disp_set_default
) display.
To get an object's screen use the lv_obj_get_screen(obj)
function.
Layers¶
There are two automatically generated layers:
top layer
system layer
They are independent of the screens and they will be shown on every screen. The top layer is above every object on the screen and the system layer is above the top layer too.
You can add any pop-up windows to the top layer freely. But, the system layer is restricted to system-level things (e.g. mouse cursor will be placed here in lv_indev_set_cursor()
).
The lv_layer_top()
and lv_layer_sys()
functions gives a pointer to the top or system layer.
You can bring an object to the foreground or send it to the background with lv_obj_move_foreground(obj)
and lv_obj_move_background(obj)
.
Read the Layer overview section to learn more about layers.
Events¶
To set an event callback for an object, use lv_obj_set_event_cb(obj, event_cb)
,
To manually send an event to an object, use lv_event_send(obj, LV_EVENT_..., data)
Read the Event overview to learn more about the events.
Parts¶
The widgets can have multiple parts. For example a Button has only a main part but a Slider is built from a background, an indicator and a knob.
The name of the parts is constructed like LV_ + <TYPE> _PART_ <NAME>
. For example LV_BTN_PART_MAIN
or LV_SLIDER_PART_KNOB
. The parts are usually used when styles are add to the objects.
Using parts different styles can be assigned to the different parts of the objects.
To learn more about the parts read the related section of the Style overview.
States¶
The object can be in a combinations of the following states:
LV_STATE_DEFAULT Normal, released
LV_STATE_CHECKED Toggled or checked
LV_STATE_FOCUSED Focused via keypad or encoder or clicked via touchpad/mouse
LV_STATE_EDITED Edit by an encoder
LV_STATE_HOVERED Hovered by mouse (not supported now)
LV_STATE_PRESSED Pressed
LV_STATE_DISABLED Disabled or inactive
The states are usually automatically changed by the library as the user presses, releases, focuses etc an object.
However, the states can be changed manually too. To completely overwrite the current state use lv_obj_set_state(obj, part, LV_STATE...)
.
To set or clear given state (but leave to other states untouched) use lv_obj_add/clear_state(obj, part, LV_STATE_...)
In both cases ORed state values can be used as well. E.g. lv_obj_set_state(obj, part, LV_STATE_PRESSED | LV_PRESSED_CHECKED)
.
To learn more about the states read the related section of the Style overview.
Style¶
Be sure to read the Style overview first.
To add a style to an object use lv_obj_add_style(obj, part, &new_style)
function. The Base object use all the rectangle-like style properties.
To remove all styles from an object use lv_obj_reset_style_list(obj, part)
If you modify a style, which is already used by objects, in order to refresh the affected objects you can use either lv_obj_refresh_style(obj)
on each object using it or
to notify all objects with a given style use lv_obj_report_style_mod(&style)
. If the parameter of lv_obj_report_style_mod
is NULL
, all objects will be notified.
Attributes¶
There are some attributes which can be enabled/disabled by lv_obj_set_...(obj, true/false)
:
hidden - Hide the object. It will not be drawn and will be considered by input devices as if it doesn't exist., Its children will be hidden too.
click - Allows you to click the object via input devices. If disabled, then click events are passed to the object behind this one. (E.g. Labels are not clickable by default)
top - If enabled then when this object or any of its children is clicked then this object comes to the foreground.
drag - Enable dragging (moving by an input device)
drag_dir - Enable dragging only in specific directions. Can be
LV_DRAG_DIR_HOR/VER/ALL
.drag_throw - Enable "throwing" with dragging as if the object would have momentum
drag_parent - If enabled then the object's parent will be moved during dragging. It will look like as if the parent is dragged. Checked recursively, so can propagate to grandparents too.
parent_event - Propagate the events to the parents too. Checked recursively, so can propagate to grandparents too.
opa_scale_enable - Enable opacity scaling. See the [#opa-scale](Opa scale) section.
Protect¶
There are some specific actions which happen automatically in the library. To prevent one or more that kind of actions, you can protect the object against them. The following protections exists:
LV_PROTECT_NONE No protection
LV_PROTECT_POS Prevent automatic positioning (e.g. Layout in Containers)
LV_PROTECT_FOLLOW Prevent the object be followed (make a "line break") in automatic ordering (e.g. Layout in Containers)
LV_PROTECT_PARENT Prevent automatic parent change. (e.g. Page moves the children created on the background to the scrollable)
LV_PROTECT_PRESS_LOST Prevent losing press when the press is slid out of the objects. (E.g. a Button can be released out of it if it was being pressed)
LV_PROTECT_CLICK_FOCUS Prevent automatically focusing the object if it's in a Group and click focus is enabled.
LV_PROTECT_CHILD_CHG Disable the child change signal. Used internally by the library
The lv_obj_add/clear_protect(obj, LV_PROTECT_...)
sets/clears the protection. You can use 'OR'ed values of protection types too.
Groups¶
Once, an object is added to group with lv_group_add_obj(group, obj)
the object's current group can be get with lv_obj_get_group(obj)
.
lv_obj_is_focused(obj)
tells if the object is currently focused on its group or not. If the object is not added to a group, false
will be returned.
Read the Input devices overview to learn more about the Groups.
Extended click area¶
By default, the objects can be clicked only on their coordinates, however, this area can be extended with lv_obj_set_ext_click_area(obj, left, right, top, bottom)
.
left/right/top/bottom
describes how far the clickable area should extend past the default in each direction.
This feature needs to enabled in lv_conf.h with LV_USE_EXT_CLICK_AREA
. The possible values are:
LV_EXT_CLICK_AREA_FULL store all 4 coordinates as
lv_coord_t
LV_EXT_CLICK_AREA_TINY store only horizontal and vertical coordinates (use the greater value of left/right and top/bottom) as
uint8_t
LV_EXT_CLICK_AREA_OFF Disable this feature
Example¶
C¶
Base objects with custom styles¶
code
#include "../../../lv_examples.h"
void lv_ex_obj_1(void)
{
lv_obj_t * obj1;
obj1 = lv_obj_create(lv_scr_act(), NULL);
lv_obj_set_size(obj1, 100, 50);
lv_obj_align(obj1, NULL, LV_ALIGN_CENTER, -60, -30);
/*Copy the previous object and enable drag*/
lv_obj_t * obj2;
obj2 = lv_obj_create(lv_scr_act(), obj1);
lv_obj_align(obj2, NULL, LV_ALIGN_CENTER, 0, 0);
lv_obj_set_drag(obj2, true);
static lv_style_t style_shadow;
lv_style_init(&style_shadow);
lv_style_set_shadow_width(&style_shadow, LV_STATE_DEFAULT, 10);
lv_style_set_shadow_spread(&style_shadow, LV_STATE_DEFAULT, 5);
lv_style_set_shadow_color(&style_shadow, LV_STATE_DEFAULT, LV_COLOR_BLUE);
/*Copy the previous object (drag is already enabled)*/
lv_obj_t * obj3;
obj3 = lv_obj_create(lv_scr_act(), obj2);
lv_obj_add_style(obj3, LV_OBJ_PART_MAIN, &style_shadow);
lv_obj_align(obj3, NULL, LV_ALIGN_CENTER, 60, 30);
}
MicroPython¶
Base objects with custom styles¶
Click to try in the simulator!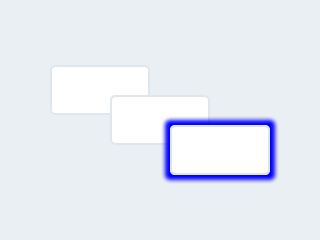
code
obj1 = lv.obj(lv.scr_act(),None)
obj1.set_size(100,50)
obj1.align(None,lv.ALIGN.CENTER, -60, -30)
# Copy the previous object and enable drag
obj2 = lv.obj(lv.scr_act(),obj1)
#obj2.set_size(100,50)
obj2.align(None,lv.ALIGN.CENTER, 0, 0)
obj2.set_drag(True)
# create style
style_shadow = lv.style_t()
style_shadow.init()
style_shadow.set_shadow_width(lv.STATE.DEFAULT, 10)
style_shadow.set_shadow_spread(lv.STATE.DEFAULT, 5)
LV_COLOR_BLUE=lv.color_hex3(0xF)
style_shadow.set_shadow_color(lv.STATE.DEFAULT,LV_COLOR_BLUE)
# Copy the previous object (drag is already enabled)
obj3 = lv.obj(lv.scr_act(),obj2)
obj3.add_style(obj3.PART.MAIN,style_shadow)
obj3.align(None,lv.ALIGN.CENTER, 60, 30)
API¶
Typedefs
-
typedef uint8_t
lv_design_mode_t
¶
-
typedef uint8_t
lv_design_res_t
¶
-
typedef lv_design_res_t (*
lv_design_cb_t
)(struct _lv_obj_t *obj, const lv_area_t *clip_area, lv_design_mode_t mode)¶ The design callback is used to draw the object on the screen. It accepts the object, a mask area, and the mode in which to draw the object.
-
typedef uint8_t
lv_event_t
¶ Type of event being sent to the object.
-
typedef void (*
lv_event_cb_t
)(struct _lv_obj_t *obj, lv_event_t event)¶ Event callback. Events are used to notify the user of some action being taken on the object. For details, see lv_event_t.
-
typedef uint8_t
lv_signal_t
¶
-
typedef lv_res_t (*
lv_signal_cb_t
)(struct _lv_obj_t *obj, lv_signal_t sign, void *param)¶
-
typedef uint8_t
lv_protect_t
¶
-
typedef uint8_t
lv_state_t
¶
-
typedef uint8_t
lv_obj_part_t
¶
Enums
-
enum [anonymous]¶
Design modes
Values:
-
enumerator
LV_DESIGN_DRAW_MAIN
¶ Draw the main portion of the object
-
enumerator
LV_DESIGN_DRAW_POST
¶ Draw extras on the object
-
enumerator
LV_DESIGN_COVER_CHK
¶ Check if the object fully covers the 'mask_p' area
-
enumerator
-
enum [anonymous]¶
Design results
Values:
-
enumerator
LV_DESIGN_RES_OK
¶ Draw ready
-
enumerator
LV_DESIGN_RES_COVER
¶ Returned on
LV_DESIGN_COVER_CHK
if the areas is fully covered
-
enumerator
LV_DESIGN_RES_NOT_COVER
¶ Returned on
LV_DESIGN_COVER_CHK
if the areas is not covered
-
enumerator
LV_DESIGN_RES_MASKED
¶ Returned on
LV_DESIGN_COVER_CHK
if the areas is masked out (children also not cover)
-
enumerator
-
enum [anonymous]¶
Values:
-
enumerator
LV_EVENT_PRESSED
¶ The object has been pressed
-
enumerator
LV_EVENT_PRESSING
¶ The object is being pressed (called continuously while pressing)
-
enumerator
LV_EVENT_PRESS_LOST
¶ User is still pressing but slid cursor/finger off of the object
-
enumerator
LV_EVENT_SHORT_CLICKED
¶ User pressed object for a short period of time, then released it. Not called if dragged.
-
enumerator
LV_EVENT_LONG_PRESSED
¶ Object has been pressed for at least
LV_INDEV_LONG_PRESS_TIME
. Not called if dragged.
-
enumerator
LV_EVENT_LONG_PRESSED_REPEAT
¶ Called after
LV_INDEV_LONG_PRESS_TIME
in everyLV_INDEV_LONG_PRESS_REP_TIME
ms. Not called if dragged.
-
enumerator
LV_EVENT_CLICKED
¶ Called on release if not dragged (regardless to long press)
-
enumerator
LV_EVENT_RELEASED
¶ Called in every cases when the object has been released
-
enumerator
LV_EVENT_DRAG_BEGIN
¶
-
enumerator
LV_EVENT_DRAG_END
¶
-
enumerator
LV_EVENT_DRAG_THROW_BEGIN
¶
-
enumerator
LV_EVENT_GESTURE
¶ The object has been gesture
-
enumerator
LV_EVENT_KEY
¶
-
enumerator
LV_EVENT_FOCUSED
¶
-
enumerator
LV_EVENT_DEFOCUSED
¶
-
enumerator
LV_EVENT_LEAVE
¶
-
enumerator
LV_EVENT_VALUE_CHANGED
¶ The object's value has changed (i.e. slider moved)
-
enumerator
LV_EVENT_INSERT
¶
-
enumerator
LV_EVENT_REFRESH
¶
-
enumerator
LV_EVENT_APPLY
¶ "Ok", "Apply" or similar specific button has clicked
-
enumerator
LV_EVENT_CANCEL
¶ "Close", "Cancel" or similar specific button has clicked
-
enumerator
LV_EVENT_DELETE
¶ Object is being deleted
-
enumerator
_LV_EVENT_LAST
¶ Number of events
-
enumerator
-
enum [anonymous]¶
Signals are for use by the object itself or to extend the object's functionality. Applications should use lv_obj_set_event_cb to be notified of events that occur on the object.
Values:
-
enumerator
LV_SIGNAL_CLEANUP
¶ Object is being deleted
-
enumerator
LV_SIGNAL_CHILD_CHG
¶ Child was removed/added
-
enumerator
LV_SIGNAL_COORD_CHG
¶ Object coordinates/size have changed
-
enumerator
LV_SIGNAL_PARENT_SIZE_CHG
¶ Parent's size has changed
-
enumerator
LV_SIGNAL_STYLE_CHG
¶ Object's style has changed
-
enumerator
LV_SIGNAL_BASE_DIR_CHG
¶ The base dir has changed
-
enumerator
LV_SIGNAL_REFR_EXT_DRAW_PAD
¶ Object's extra padding has changed
-
enumerator
LV_SIGNAL_GET_TYPE
¶ LVGL needs to retrieve the object's type
-
enumerator
LV_SIGNAL_GET_STYLE
¶ Get the style of an object
-
enumerator
LV_SIGNAL_GET_STATE_DSC
¶ Get the state of the object
-
enumerator
LV_SIGNAL_HIT_TEST
¶ Advanced hit-testing
-
enumerator
LV_SIGNAL_PRESSED
¶ The object has been pressed
-
enumerator
LV_SIGNAL_PRESSING
¶ The object is being pressed (called continuously while pressing)
-
enumerator
LV_SIGNAL_PRESS_LOST
¶ User is still pressing but slid cursor/finger off of the object
-
enumerator
LV_SIGNAL_RELEASED
¶ User pressed object for a short period of time, then released it. Not called if dragged.
-
enumerator
LV_SIGNAL_LONG_PRESS
¶ Object has been pressed for at least
LV_INDEV_LONG_PRESS_TIME
. Not called if dragged.
-
enumerator
LV_SIGNAL_LONG_PRESS_REP
¶ Called after
LV_INDEV_LONG_PRESS_TIME
in everyLV_INDEV_LONG_PRESS_REP_TIME
ms. Not called if dragged.
-
enumerator
LV_SIGNAL_DRAG_BEGIN
¶
-
enumerator
LV_SIGNAL_DRAG_THROW_BEGIN
¶
-
enumerator
LV_SIGNAL_DRAG_END
¶
-
enumerator
LV_SIGNAL_GESTURE
¶ The object has been gesture
-
enumerator
LV_SIGNAL_LEAVE
¶ Another object is clicked or chosen via an input device
-
enumerator
LV_SIGNAL_FOCUS
¶
-
enumerator
LV_SIGNAL_DEFOCUS
¶
-
enumerator
LV_SIGNAL_CONTROL
¶
-
enumerator
LV_SIGNAL_GET_EDITABLE
¶
-
enumerator
-
enum [anonymous]¶
Values:
-
enumerator
LV_PROTECT_NONE
¶
-
enumerator
LV_PROTECT_CHILD_CHG
¶ Disable the child change signal. Used by the library
-
enumerator
LV_PROTECT_PARENT
¶ Prevent automatic parent change (e.g. in lv_page)
-
enumerator
LV_PROTECT_POS
¶ Prevent automatic positioning (e.g. in lv_cont layout)
-
enumerator
LV_PROTECT_FOLLOW
¶ Prevent the object be followed in automatic ordering (e.g. in lv_cont PRETTY layout)
-
enumerator
LV_PROTECT_PRESS_LOST
¶ If the
indev
was pressing this object but swiped out while pressing do not search other object.
-
enumerator
LV_PROTECT_CLICK_FOCUS
¶ Prevent focusing the object by clicking on it
-
enumerator
LV_PROTECT_EVENT_TO_DISABLED
¶ Pass events even to disabled objects
-
enumerator
Functions
-
void
lv_init
(void)¶ Init. the 'lv' library.
-
void
lv_deinit
(void)¶ Deinit the 'lv' library Currently only implemented when not using custom allocators, or GC is enabled.
-
lv_obj_t *
lv_obj_create
(lv_obj_t *parent, const lv_obj_t *copy)¶ Create a basic object
- Parameters
parent -- pointer to a parent object. If NULL then a screen will be created
copy -- pointer to a base object, if not NULL then the new object will be copied from it
- Returns
pointer to the new object
-
lv_res_t
lv_obj_del
(lv_obj_t *obj)¶ Delete 'obj' and all of its children
- Parameters
obj -- pointer to an object to delete
- Returns
LV_RES_INV because the object is deleted
-
void
lv_obj_del_anim_ready_cb
(lv_anim_t *a)¶ A function to be easily used in animation ready callback to delete an object when the animation is ready
- Parameters
a -- pointer to the animation
-
void
lv_obj_del_async
(struct _lv_obj_t *obj)¶ Helper function for asynchronously deleting objects. Useful for cases where you can't delete an object directly in an
LV_EVENT_DELETE
handler (i.e. parent).- Parameters
obj -- object to delete
-
void
lv_obj_clean
(lv_obj_t *obj)¶ Delete all children of an object
- Parameters
obj -- pointer to an object
-
void
lv_obj_invalidate_area
(const lv_obj_t *obj, const lv_area_t *area)¶ Mark an area of an object as invalid. This area will be redrawn by 'lv_refr_task'
- Parameters
obj -- pointer to an object
area -- the area to redraw
-
void
lv_obj_invalidate
(const lv_obj_t *obj)¶ Mark the object as invalid therefore its current position will be redrawn by 'lv_refr_task'
- Parameters
obj -- pointer to an object
-
bool
lv_obj_area_is_visible
(const lv_obj_t *obj, lv_area_t *area)¶ Tell whether an area of an object is visible (even partially) now or not
- Parameters
obj -- pointer to an object
area -- the are to check. The visible part of the area will be written back here.
- Returns
true: visible; false: not visible (hidden, out of parent, on other screen, etc)
-
bool
lv_obj_is_visible
(const lv_obj_t *obj)¶ Tell whether an object is visible (even partially) now or not
- Parameters
obj -- pointer to an object
- Returns
true: visible; false: not visible (hidden, out of parent, on other screen, etc)
-
void
lv_obj_set_parent
(lv_obj_t *obj, lv_obj_t *parent)¶ Set a new parent for an object. Its relative position will be the same.
- Parameters
obj -- pointer to an object. Can't be a screen.
parent -- pointer to the new parent object. (Can't be NULL)
-
void
lv_obj_move_foreground
(lv_obj_t *obj)¶ Move and object to the foreground
- Parameters
obj -- pointer to an object
-
void
lv_obj_move_background
(lv_obj_t *obj)¶ Move and object to the background
- Parameters
obj -- pointer to an object
-
void
lv_obj_set_pos
(lv_obj_t *obj, lv_coord_t x, lv_coord_t y)¶ Set relative the position of an object (relative to the parent)
- Parameters
obj -- pointer to an object
x -- new distance from the left side of the parent
y -- new distance from the top of the parent
-
void
lv_obj_set_x
(lv_obj_t *obj, lv_coord_t x)¶ Set the x coordinate of a object
- Parameters
obj -- pointer to an object
x -- new distance from the left side from the parent
-
void
lv_obj_set_y
(lv_obj_t *obj, lv_coord_t y)¶ Set the y coordinate of a object
- Parameters
obj -- pointer to an object
y -- new distance from the top of the parent
-
void
lv_obj_set_size
(lv_obj_t *obj, lv_coord_t w, lv_coord_t h)¶ Set the size of an object
- Parameters
obj -- pointer to an object
w -- new width
h -- new height
-
void
lv_obj_set_width
(lv_obj_t *obj, lv_coord_t w)¶ Set the width of an object
- Parameters
obj -- pointer to an object
w -- new width
-
void
lv_obj_set_height
(lv_obj_t *obj, lv_coord_t h)¶ Set the height of an object
- Parameters
obj -- pointer to an object
h -- new height
-
void
lv_obj_set_width_fit
(lv_obj_t *obj, lv_coord_t w)¶ Set the width reduced by the left and right padding.
- Parameters
obj -- pointer to an object
w -- the width without paddings
-
void
lv_obj_set_height_fit
(lv_obj_t *obj, lv_coord_t h)¶ Set the height reduced by the top and bottom padding.
- Parameters
obj -- pointer to an object
h -- the height without paddings
-
void
lv_obj_set_width_margin
(lv_obj_t *obj, lv_coord_t w)¶ Set the width of an object by taking the left and right margin into account. The object width will be
obj_w = w - margin_left - margin_right
- Parameters
obj -- pointer to an object
w -- new height including margins
-
void
lv_obj_set_height_margin
(lv_obj_t *obj, lv_coord_t h)¶ Set the height of an object by taking the top and bottom margin into account. The object height will be
obj_h = h - margin_top - margin_bottom
- Parameters
obj -- pointer to an object
h -- new height including margins
-
void
lv_obj_align
(lv_obj_t *obj, const lv_obj_t *base, lv_align_t align, lv_coord_t x_ofs, lv_coord_t y_ofs)¶ Align an object to an other object.
- Parameters
obj -- pointer to an object to align
base -- pointer to an object (if NULL the parent is used). 'obj' will be aligned to it.
align -- type of alignment (see 'lv_align_t' enum)
x_ofs -- x coordinate offset after alignment
y_ofs -- y coordinate offset after alignment
-
void
lv_obj_align_x
(lv_obj_t *obj, const lv_obj_t *base, lv_align_t align, lv_coord_t x_ofs)¶ Align an object to an other object horizontally.
- Parameters
obj -- pointer to an object to align
base -- pointer to an object (if NULL the parent is used). 'obj' will be aligned to it.
align -- type of alignment (see 'lv_align_t' enum)
x_ofs -- x coordinate offset after alignment
-
void
lv_obj_align_y
(lv_obj_t *obj, const lv_obj_t *base, lv_align_t align, lv_coord_t y_ofs)¶ Align an object to an other object vertically.
- Parameters
obj -- pointer to an object to align
base -- pointer to an object (if NULL the parent is used). 'obj' will be aligned to it.
align -- type of alignment (see 'lv_align_t' enum)
y_ofs -- y coordinate offset after alignment
-
void
lv_obj_align_mid
(lv_obj_t *obj, const lv_obj_t *base, lv_align_t align, lv_coord_t x_ofs, lv_coord_t y_ofs)¶ Align an object to an other object.
- Parameters
obj -- pointer to an object to align
base -- pointer to an object (if NULL the parent is used). 'obj' will be aligned to it.
align -- type of alignment (see 'lv_align_t' enum)
x_ofs -- x coordinate offset after alignment
y_ofs -- y coordinate offset after alignment
-
void
lv_obj_align_mid_x
(lv_obj_t *obj, const lv_obj_t *base, lv_align_t align, lv_coord_t x_ofs)¶ Align an object's middle point to an other object horizontally.
- Parameters
obj -- pointer to an object to align
base -- pointer to an object (if NULL the parent is used). 'obj' will be aligned to it.
align -- type of alignment (see 'lv_align_t' enum)
x_ofs -- x coordinate offset after alignment
-
void
lv_obj_align_mid_y
(lv_obj_t *obj, const lv_obj_t *base, lv_align_t align, lv_coord_t y_ofs)¶ Align an object's middle point to an other object vertically.
- Parameters
obj -- pointer to an object to align
base -- pointer to an object (if NULL the parent is used). 'obj' will be aligned to it.
align -- type of alignment (see 'lv_align_t' enum)
y_ofs -- y coordinate offset after alignment
-
void
lv_obj_realign
(lv_obj_t *obj)¶ Realign the object based on the last
lv_obj_align
parameters.- Parameters
obj -- pointer to an object
-
void
lv_obj_set_auto_realign
(lv_obj_t *obj, bool en)¶ Enable the automatic realign of the object when its size has changed based on the last
lv_obj_align
parameters.- Parameters
obj -- pointer to an object
en -- true: enable auto realign; false: disable auto realign
-
void
lv_obj_set_ext_click_area
(lv_obj_t *obj, lv_coord_t left, lv_coord_t right, lv_coord_t top, lv_coord_t bottom)¶ Set the size of an extended clickable area
- Parameters
obj -- pointer to an object
left -- extended clickable are on the left [px]
right -- extended clickable are on the right [px]
top -- extended clickable are on the top [px]
bottom -- extended clickable are on the bottom [px]
-
void
lv_obj_add_style
(lv_obj_t *obj, uint8_t part, lv_style_t *style)¶ Add a new style to the style list of an object.
- Parameters
obj -- pointer to an object
part -- the part of the object which style property should be set. E.g.
LV_OBJ_PART_MAIN
,LV_BTN_PART_MAIN
,LV_SLIDER_PART_KNOB
style -- pointer to a style to add (Only its pointer will be saved)
-
void
lv_obj_remove_style
(lv_obj_t *obj, uint8_t part, lv_style_t *style)¶ Remove a style from the style list of an object.
- Parameters
obj -- pointer to an object
part -- the part of the object which style property should be set. E.g.
LV_OBJ_PART_MAIN
,LV_BTN_PART_MAIN
,LV_SLIDER_PART_KNOB
style -- pointer to a style to remove
-
void
lv_obj_clean_style_list
(lv_obj_t *obj, uint8_t part)¶ Reset a style to the default (empty) state. Release all used memories and cancel pending related transitions. Typically used in `LV_SIGN_CLEAN_UP.
- Parameters
obj -- pointer to an object
part -- the part of the object which style list should be reset. E.g.
LV_OBJ_PART_MAIN
,LV_BTN_PART_MAIN
,LV_SLIDER_PART_KNOB
-
void
lv_obj_reset_style_list
(lv_obj_t *obj, uint8_t part)¶ Reset a style to the default (empty) state. Release all used memories and cancel pending related transitions. Also notifies the object about the style change.
- Parameters
obj -- pointer to an object
part -- the part of the object which style list should be reset. E.g.
LV_OBJ_PART_MAIN
,LV_BTN_PART_MAIN
,LV_SLIDER_PART_KNOB
-
void
lv_obj_refresh_style
(lv_obj_t *obj, uint8_t part, lv_style_property_t prop)¶ Notify an object (and its children) about its style is modified
- Parameters
obj -- pointer to an object
prop --
LV_STYLE_PROP_ALL
or anLV_STYLE_...
property. It is used to optimize what needs to be refreshed.
-
void
lv_obj_report_style_mod
(lv_style_t *style)¶ Notify all object if a style is modified
- Parameters
style -- pointer to a style. Only the objects with this style will be notified (NULL to notify all objects)
-
void
_lv_obj_set_style_local_color
(lv_obj_t *obj, uint8_t type, lv_style_property_t prop, lv_color_t color)¶ Set a local style property of a part of an object in a given state.
Note
shouldn't be used directly. Use the specific property get functions instead. For example:
lv_obj_style_get_border_opa()
Note
for performance reasons it's not checked if the property really has color type
- Parameters
obj -- pointer to an object
part -- the part of the object which style property should be set. E.g.
LV_OBJ_PART_MAIN
,LV_BTN_PART_MAIN
,LV_SLIDER_PART_KNOB
prop -- a style property ORed with a state. E.g.
LV_STYLE_BORDER_COLOR | (LV_STATE_PRESSED << LV_STYLE_STATE_POS)
the -- value to set
-
void
_lv_obj_set_style_local_int
(lv_obj_t *obj, uint8_t type, lv_style_property_t prop, lv_style_int_t value)¶ Set a local style property of a part of an object in a given state.
Note
shouldn't be used directly. Use the specific property get functions instead. For example:
lv_obj_style_get_border_opa()
Note
for performance reasons it's not checked if the property really has integer type
- Parameters
obj -- pointer to an object
part -- the part of the object which style property should be set. E.g.
LV_OBJ_PART_MAIN
,LV_BTN_PART_MAIN
,LV_SLIDER_PART_KNOB
prop -- a style property ORed with a state. E.g.
LV_STYLE_BORDER_WIDTH | (LV_STATE_PRESSED << LV_STYLE_STATE_POS)
the -- value to set
-
void
_lv_obj_set_style_local_opa
(lv_obj_t *obj, uint8_t type, lv_style_property_t prop, lv_opa_t opa)¶ Set a local style property of a part of an object in a given state.
Note
shouldn't be used directly. Use the specific property get functions instead. For example:
lv_obj_style_get_border_opa()
Note
for performance reasons it's not checked if the property really has opacity type
- Parameters
obj -- pointer to an object
part -- the part of the object which style property should be set. E.g.
LV_OBJ_PART_MAIN
,LV_BTN_PART_MAIN
,LV_SLIDER_PART_KNOB
prop -- a style property ORed with a state. E.g.
LV_STYLE_BORDER_OPA | (LV_STATE_PRESSED << LV_STYLE_STATE_POS)
the -- value to set
-
void
_lv_obj_set_style_local_ptr
(lv_obj_t *obj, uint8_t type, lv_style_property_t prop, const void *value)¶ Set a local style property of a part of an object in a given state.
Note
shouldn't be used directly. Use the specific property get functions instead. For example:
lv_obj_style_get_border_opa()
Note
for performance reasons it's not checked if the property really has pointer type
- Parameters
obj -- pointer to an object
part -- the part of the object which style property should be set. E.g.
LV_OBJ_PART_MAIN
,LV_BTN_PART_MAIN
,LV_SLIDER_PART_KNOB
prop -- a style property ORed with a state. E.g.
LV_STYLE_TEXT_FONT | (LV_STATE_PRESSED << LV_STYLE_STATE_POS)
the -- value to set
-
bool
lv_obj_remove_style_local_prop
(lv_obj_t *obj, uint8_t part, lv_style_property_t prop)¶ Remove a local style property from a part of an object with a given state.
Note
shouldn't be used directly. Use the specific property remove functions instead. For example:
lv_obj_style_remove_border_opa()
- Parameters
obj -- pointer to an object
part -- the part of the object which style property should be removed. E.g.
LV_OBJ_PART_MAIN
,LV_BTN_PART_MAIN
,LV_SLIDER_PART_KNOB
prop -- a style property ORed with a state. E.g.
LV_STYLE_TEXT_FONT | (LV_STATE_PRESSED << LV_STYLE_STATE_POS)
- Returns
true: the property was found and removed; false: the property was not found
-
void
_lv_obj_disable_style_caching
(lv_obj_t *obj, bool dis)¶ Enable/disable the use of style cache for an object
- Parameters
obj -- pointer to an object
dis -- true: disable; false: enable (re-enable)
Hide an object. It won't be visible and clickable.
- Parameters
obj -- pointer to an object
en -- true: hide the object
-
void
lv_obj_set_adv_hittest
(lv_obj_t *obj, bool en)¶ Set whether advanced hit-testing is enabled on an object
- Parameters
obj -- pointer to an object
en -- true: advanced hit-testing is enabled
-
void
lv_obj_set_click
(lv_obj_t *obj, bool en)¶ Enable or disable the clicking of an object
- Parameters
obj -- pointer to an object
en -- true: make the object clickable
-
void
lv_obj_set_top
(lv_obj_t *obj, bool en)¶ Enable to bring this object to the foreground if it or any of its children is clicked
- Parameters
obj -- pointer to an object
en -- true: enable the auto top feature
-
void
lv_obj_set_drag
(lv_obj_t *obj, bool en)¶ Enable the dragging of an object
- Parameters
obj -- pointer to an object
en -- true: make the object draggable
-
void
lv_obj_set_drag_dir
(lv_obj_t *obj, lv_drag_dir_t drag_dir)¶ Set the directions an object can be dragged in
- Parameters
obj -- pointer to an object
drag_dir -- bitwise OR of allowed drag directions
-
void
lv_obj_set_drag_throw
(lv_obj_t *obj, bool en)¶ Enable the throwing of an object after is is dragged
- Parameters
obj -- pointer to an object
en -- true: enable the drag throw
-
void
lv_obj_set_drag_parent
(lv_obj_t *obj, bool en)¶ Enable to use parent for drag related operations. If trying to drag the object the parent will be moved instead
- Parameters
obj -- pointer to an object
en -- true: enable the 'drag parent' for the object
-
void
lv_obj_set_focus_parent
(lv_obj_t *obj, bool en)¶ Enable to use parent for focus state. When object is focused the parent will get the state instead (visual only)
- Parameters
obj -- pointer to an object
en -- true: enable the 'focus parent' for the object
-
void
lv_obj_set_gesture_parent
(lv_obj_t *obj, bool en)¶ Enable to use parent for gesture related operations. If trying to gesture the object the parent will be moved instead
- Parameters
obj -- pointer to an object
en -- true: enable the 'gesture parent' for the object
-
void
lv_obj_set_parent_event
(lv_obj_t *obj, bool en)¶ Propagate the events to the parent too
- Parameters
obj -- pointer to an object
en -- true: enable the event propagation
-
void
lv_obj_set_base_dir
(lv_obj_t *obj, lv_bidi_dir_t dir)¶ Set the base direction of the object
Note
This only works if LV_USE_BIDI is enabled.
- Parameters
obj -- pointer to an object
dir -- the new base direction.
LV_BIDI_DIR_LTR/RTL/AUTO/INHERIT
-
void
lv_obj_add_protect
(lv_obj_t *obj, uint8_t prot)¶ Set a bit or bits in the protect filed
- Parameters
obj -- pointer to an object
prot -- 'OR'-ed values from
lv_protect_t
-
void
lv_obj_clear_protect
(lv_obj_t *obj, uint8_t prot)¶ Clear a bit or bits in the protect filed
- Parameters
obj -- pointer to an object
prot -- 'OR'-ed values from
lv_protect_t
-
void
lv_obj_set_state
(lv_obj_t *obj, lv_state_t state)¶ Set the state (fully overwrite) of an object. If specified in the styles a transition animation will be started from the previous state to the current
- Parameters
obj -- pointer to an object
state -- the new state
-
void
lv_obj_add_state
(lv_obj_t *obj, lv_state_t state)¶ Add a given state or states to the object. The other state bits will remain unchanged. If specified in the styles a transition animation will be started from the previous state to the current
- Parameters
obj -- pointer to an object
state -- the state bits to add. E.g
LV_STATE_PRESSED | LV_STATE_FOCUSED
-
void
lv_obj_clear_state
(lv_obj_t *obj, lv_state_t state)¶ Remove a given state or states to the object. The other state bits will remain unchanged. If specified in the styles a transition animation will be started from the previous state to the current
- Parameters
obj -- pointer to an object
state -- the state bits to remove. E.g
LV_STATE_PRESSED | LV_STATE_FOCUSED
-
void
lv_obj_finish_transitions
(lv_obj_t *obj, uint8_t part)¶ Finish all pending transitions on a part of an object
- Parameters
obj -- pointer to an object
part -- part of the object, e.g
LV_BRN_PART_MAIN
orLV_OBJ_PART_ALL
for all parts
-
void
lv_obj_set_event_cb
(lv_obj_t *obj, lv_event_cb_t event_cb)¶ Set a an event handler function for an object. Used by the user to react on event which happens with the object.
- Parameters
obj -- pointer to an object
event_cb -- the new event function
-
lv_res_t
lv_event_send
(lv_obj_t *obj, lv_event_t event, const void *data)¶ Send an event to the object
- Parameters
obj -- pointer to an object
event -- the type of the event from
lv_event_t
.data -- arbitrary data depending on the object type and the event. (Usually
NULL
)
- Returns
LV_RES_OK:
obj
was not deleted in the event; LV_RES_INV:obj
was deleted in the event
-
lv_res_t
lv_event_send_refresh
(lv_obj_t *obj)¶ Send LV_EVENT_REFRESH event to an object
- Parameters
obj -- point to an object. (Can NOT be NULL)
- Returns
LV_RES_OK: success, LV_RES_INV: to object become invalid (e.g. deleted) due to this event.
-
void
lv_event_send_refresh_recursive
(lv_obj_t *obj)¶ Send LV_EVENT_REFRESH event to an object and all of its children
- Parameters
obj -- pointer to an object or NULL to refresh all objects of all displays
-
lv_res_t
lv_event_send_func
(lv_event_cb_t event_xcb, lv_obj_t *obj, lv_event_t event, const void *data)¶ Call an event function with an object, event, and data.
- Parameters
event_xcb -- an event callback function. If
NULL
LV_RES_OK
will return without any actions. (the 'x' in the argument name indicates that its not a fully generic function because it not follows thefunc_name(object, callback, ...)
convention)obj -- pointer to an object to associate with the event (can be
NULL
to simply call theevent_cb
)event -- an event
data -- pointer to a custom data
- Returns
LV_RES_OK:
obj
was not deleted in the event; LV_RES_INV:obj
was deleted in the event
-
const void *
lv_event_get_data
(void)¶ Get the
data
parameter of the current event- Returns
the
data
parameter
-
void
lv_obj_set_signal_cb
(lv_obj_t *obj, lv_signal_cb_t signal_cb)¶ Set the a signal function of an object. Used internally by the library. Always call the previous signal function in the new.
- Parameters
obj -- pointer to an object
signal_cb -- the new signal function
-
lv_res_t
lv_signal_send
(lv_obj_t *obj, lv_signal_t signal, void *param)¶ Send an event to the object
- Parameters
obj -- pointer to an object
event -- the type of the event from
lv_event_t
.
- Returns
LV_RES_OK or LV_RES_INV
-
void
lv_obj_set_design_cb
(lv_obj_t *obj, lv_design_cb_t design_cb)¶ Set a new design function for an object
- Parameters
obj -- pointer to an object
design_cb -- the new design function
-
void *
lv_obj_allocate_ext_attr
(lv_obj_t *obj, uint16_t ext_size)¶ Allocate a new ext. data for an object
- Parameters
obj -- pointer to an object
ext_size -- the size of the new ext. data
- Returns
pointer to the allocated ext
-
void
lv_obj_refresh_ext_draw_pad
(lv_obj_t *obj)¶ Send a 'LV_SIGNAL_REFR_EXT_SIZE' signal to the object to refresh the extended draw area. he object needs to be invalidated by
lv_obj_invalidate(obj)
manually after this function.- Parameters
obj -- pointer to an object
-
lv_obj_t *
lv_obj_get_screen
(const lv_obj_t *obj)¶ Return with the screen of an object
- Parameters
obj -- pointer to an object
- Returns
pointer to a screen
-
lv_disp_t *
lv_obj_get_disp
(const lv_obj_t *obj)¶ Get the display of an object
- Returns
pointer the object's display
-
lv_obj_t *
lv_obj_get_parent
(const lv_obj_t *obj)¶ Returns with the parent of an object
- Parameters
obj -- pointer to an object
- Returns
pointer to the parent of 'obj'
-
lv_obj_t *
lv_obj_get_child
(const lv_obj_t *obj, const lv_obj_t *child)¶ Iterate through the children of an object (start from the "youngest, lastly created")
- Parameters
obj -- pointer to an object
child -- NULL at first call to get the next children and the previous return value later
- Returns
the child after 'act_child' or NULL if no more child
-
lv_obj_t *
lv_obj_get_child_back
(const lv_obj_t *obj, const lv_obj_t *child)¶ Iterate through the children of an object (start from the "oldest", firstly created)
- Parameters
obj -- pointer to an object
child -- NULL at first call to get the next children and the previous return value later
- Returns
the child after 'act_child' or NULL if no more child
-
uint16_t
lv_obj_count_children
(const lv_obj_t *obj)¶ Count the children of an object (only children directly on 'obj')
- Parameters
obj -- pointer to an object
- Returns
children number of 'obj'
-
uint16_t
lv_obj_count_children_recursive
(const lv_obj_t *obj)¶ Recursively count the children of an object
- Parameters
obj -- pointer to an object
- Returns
children number of 'obj'
-
void
lv_obj_get_coords
(const lv_obj_t *obj, lv_area_t *cords_p)¶ Copy the coordinates of an object to an area
- Parameters
obj -- pointer to an object
cords_p -- pointer to an area to store the coordinates
-
void
lv_obj_get_inner_coords
(const lv_obj_t *obj, lv_area_t *coords_p)¶ Reduce area retried by
lv_obj_get_coords()
the get graphically usable area of an object. (Without the size of the border or other extra graphical elements)- Parameters
coords_p -- store the result area here
-
lv_coord_t
lv_obj_get_x
(const lv_obj_t *obj)¶ Get the x coordinate of object
- Parameters
obj -- pointer to an object
- Returns
distance of 'obj' from the left side of its parent
-
lv_coord_t
lv_obj_get_y
(const lv_obj_t *obj)¶ Get the y coordinate of object
- Parameters
obj -- pointer to an object
- Returns
distance of 'obj' from the top of its parent
-
lv_coord_t
lv_obj_get_width
(const lv_obj_t *obj)¶ Get the width of an object
- Parameters
obj -- pointer to an object
- Returns
the width
-
lv_coord_t
lv_obj_get_height
(const lv_obj_t *obj)¶ Get the height of an object
- Parameters
obj -- pointer to an object
- Returns
the height
-
lv_coord_t
lv_obj_get_width_fit
(const lv_obj_t *obj)¶ Get that width reduced by the left and right padding.
- Parameters
obj -- pointer to an object
- Returns
the width which still fits into the container
-
lv_coord_t
lv_obj_get_height_fit
(const lv_obj_t *obj)¶ Get that height reduced by the top an bottom padding.
- Parameters
obj -- pointer to an object
- Returns
the height which still fits into the container
-
lv_coord_t
lv_obj_get_height_margin
(lv_obj_t *obj)¶ Get the height of an object by taking the top and bottom margin into account. The returned height will be
obj_h + margin_top + margin_bottom
- Parameters
obj -- pointer to an object
- Returns
the height including thee margins
-
lv_coord_t
lv_obj_get_width_margin
(lv_obj_t *obj)¶ Get the width of an object by taking the left and right margin into account. The returned width will be
obj_w + margin_left + margin_right
- Parameters
obj -- pointer to an object
- Returns
the height including thee margins
-
lv_coord_t
lv_obj_get_width_grid
(lv_obj_t *obj, uint8_t div, uint8_t span)¶ Divide the width of the object and get the width of a given number of columns. Take paddings into account.
- Parameters
obj -- pointer to an object
div -- indicates how many columns are assumed. If 1 the width will be set the parent's width If 2 only half parent width - inner padding of the parent If 3 only third parent width - 2 * inner padding of the parent
span -- how many columns are combined
- Returns
the width according to the given parameters
-
lv_coord_t
lv_obj_get_height_grid
(lv_obj_t *obj, uint8_t div, uint8_t span)¶ Divide the height of the object and get the width of a given number of columns. Take paddings into account.
- Parameters
obj -- pointer to an object
div -- indicates how many rows are assumed. If 1 the height will be set the parent's height If 2 only half parent height - inner padding of the parent If 3 only third parent height - 2 * inner padding of the parent
span -- how many rows are combined
- Returns
the height according to the given parameters
-
bool
lv_obj_get_auto_realign
(const lv_obj_t *obj)¶ Get the automatic realign property of the object.
- Parameters
obj -- pointer to an object
- Returns
true: auto realign is enabled; false: auto realign is disabled
-
lv_coord_t
lv_obj_get_ext_click_pad_left
(const lv_obj_t *obj)¶ Get the left padding of extended clickable area
- Parameters
obj -- pointer to an object
- Returns
the extended left padding
-
lv_coord_t
lv_obj_get_ext_click_pad_right
(const lv_obj_t *obj)¶ Get the right padding of extended clickable area
- Parameters
obj -- pointer to an object
- Returns
the extended right padding
-
lv_coord_t
lv_obj_get_ext_click_pad_top
(const lv_obj_t *obj)¶ Get the top padding of extended clickable area
- Parameters
obj -- pointer to an object
- Returns
the extended top padding
-
lv_coord_t
lv_obj_get_ext_click_pad_bottom
(const lv_obj_t *obj)¶ Get the bottom padding of extended clickable area
- Parameters
obj -- pointer to an object
- Returns
the extended bottom padding
-
lv_coord_t
lv_obj_get_ext_draw_pad
(const lv_obj_t *obj)¶ Get the extended size attribute of an object
- Parameters
obj -- pointer to an object
- Returns
the extended size attribute
-
lv_style_list_t *
lv_obj_get_style_list
(const lv_obj_t *obj, uint8_t part)¶ Get the style list of an object's part.
- Parameters
obj -- pointer to an object.
part -- part the part of the object which style list should be get. E.g.
LV_OBJ_PART_MAIN
,LV_BTN_PART_MAIN
,LV_SLIDER_PART_KNOB
- Returns
pointer to the style list. (Can be
NULL
)
-
lv_style_int_t
_lv_obj_get_style_int
(const lv_obj_t *obj, uint8_t part, lv_style_property_t prop)¶ Get a style property of a part of an object in the object's current state. If there is a running transitions it is taken into account
Note
shouldn't be used directly. Use the specific property get functions instead. For example:
lv_obj_style_get_border_width()
Note
for performance reasons it's not checked if the property really has integer type
- Parameters
obj -- pointer to an object
part -- the part of the object which style property should be get. E.g.
LV_OBJ_PART_MAIN
,LV_BTN_PART_MAIN
,LV_SLIDER_PART_KNOB
prop -- the property to get. E.g.
LV_STYLE_BORDER_WIDTH
. The state of the object will be added internally
- Returns
the value of the property of the given part in the current state. If the property is not found a default value will be returned.
-
lv_color_t
_lv_obj_get_style_color
(const lv_obj_t *obj, uint8_t part, lv_style_property_t prop)¶ Get a style property of a part of an object in the object's current state. If there is a running transitions it is taken into account
Note
shouldn't be used directly. Use the specific property get functions instead. For example:
lv_obj_style_get_border_color()
Note
for performance reasons it's not checked if the property really has color type
- Parameters
obj -- pointer to an object
part -- the part of the object which style property should be get. E.g.
LV_OBJ_PART_MAIN
,LV_BTN_PART_MAIN
,LV_SLIDER_PART_KNOB
prop -- the property to get. E.g.
LV_STYLE_BORDER_COLOR
. The state of the object will be added internally
- Returns
the value of the property of the given part in the current state. If the property is not found a default value will be returned.
-
lv_opa_t
_lv_obj_get_style_opa
(const lv_obj_t *obj, uint8_t part, lv_style_property_t prop)¶ Get a style property of a part of an object in the object's current state. If there is a running transitions it is taken into account
Note
shouldn't be used directly. Use the specific property get functions instead. For example:
lv_obj_style_get_border_opa()
Note
for performance reasons it's not checked if the property really has opacity type
- Parameters
obj -- pointer to an object
part -- the part of the object which style property should be get. E.g.
LV_OBJ_PART_MAIN
,LV_BTN_PART_MAIN
,LV_SLIDER_PART_KNOB
prop -- the property to get. E.g.
LV_STYLE_BORDER_OPA
. The state of the object will be added internally
- Returns
the value of the property of the given part in the current state. If the property is not found a default value will be returned.
-
const void *
_lv_obj_get_style_ptr
(const lv_obj_t *obj, uint8_t part, lv_style_property_t prop)¶ Get a style property of a part of an object in the object's current state. If there is a running transitions it is taken into account
Note
shouldn't be used directly. Use the specific property get functions instead. For example:
lv_obj_style_get_border_opa()
Note
for performance reasons it's not checked if the property really has pointer type
- Parameters
obj -- pointer to an object
part -- the part of the object which style property should be get. E.g.
LV_OBJ_PART_MAIN
,LV_BTN_PART_MAIN
,LV_SLIDER_PART_KNOB
prop -- the property to get. E.g.
LV_STYLE_TEXT_FONT
. The state of the object will be added internally
- Returns
the value of the property of the given part in the current state. If the property is not found a default value will be returned.
-
lv_style_t *
lv_obj_get_local_style
(lv_obj_t *obj, uint8_t part)¶ Get the local style of a part of an object.
- Parameters
obj -- pointer to an object
part -- the part of the object which style property should be set. E.g.
LV_OBJ_PART_MAIN
,LV_BTN_PART_MAIN
,LV_SLIDER_PART_KNOB
- Returns
pointer to the local style if exists else
NULL
.
Get the hidden attribute of an object
- Parameters
obj -- pointer to an object
- Returns
true: the object is hidden
-
bool
lv_obj_get_adv_hittest
(const lv_obj_t *obj)¶ Get whether advanced hit-testing is enabled on an object
- Parameters
obj -- pointer to an object
- Returns
true: advanced hit-testing is enabled
-
bool
lv_obj_get_click
(const lv_obj_t *obj)¶ Get the click enable attribute of an object
- Parameters
obj -- pointer to an object
- Returns
true: the object is clickable
-
bool
lv_obj_get_top
(const lv_obj_t *obj)¶ Get the top enable attribute of an object
- Parameters
obj -- pointer to an object
- Returns
true: the auto top feature is enabled
-
bool
lv_obj_get_drag
(const lv_obj_t *obj)¶ Get the drag enable attribute of an object
- Parameters
obj -- pointer to an object
- Returns
true: the object is draggable
-
lv_drag_dir_t
lv_obj_get_drag_dir
(const lv_obj_t *obj)¶ Get the directions an object can be dragged
- Parameters
obj -- pointer to an object
- Returns
bitwise OR of allowed directions an object can be dragged in
-
bool
lv_obj_get_drag_throw
(const lv_obj_t *obj)¶ Get the drag throw enable attribute of an object
- Parameters
obj -- pointer to an object
- Returns
true: drag throw is enabled
-
bool
lv_obj_get_drag_parent
(const lv_obj_t *obj)¶ Get the drag parent attribute of an object
- Parameters
obj -- pointer to an object
- Returns
true: drag parent is enabled
-
bool
lv_obj_get_focus_parent
(const lv_obj_t *obj)¶ Get the focus parent attribute of an object
- Parameters
obj -- pointer to an object
- Returns
true: focus parent is enabled
-
bool
lv_obj_get_parent_event
(const lv_obj_t *obj)¶ Get the drag parent attribute of an object
- Parameters
obj -- pointer to an object
- Returns
true: drag parent is enabled
-
bool
lv_obj_get_gesture_parent
(const lv_obj_t *obj)¶ Get the gesture parent attribute of an object
- Parameters
obj -- pointer to an object
- Returns
true: gesture parent is enabled
-
uint8_t
lv_obj_get_protect
(const lv_obj_t *obj)¶ Get the protect field of an object
- Parameters
obj -- pointer to an object
- Returns
protect field ('OR'ed values of
lv_protect_t
)
-
bool
lv_obj_is_protected
(const lv_obj_t *obj, uint8_t prot)¶ Check at least one bit of a given protect bitfield is set
- Parameters
obj -- pointer to an object
prot -- protect bits to test ('OR'ed values of
lv_protect_t
)
- Returns
false: none of the given bits are set, true: at least one bit is set
-
lv_state_t
lv_obj_get_state
(const lv_obj_t *obj, uint8_t part)¶
-
lv_signal_cb_t
lv_obj_get_signal_cb
(const lv_obj_t *obj)¶ Get the signal function of an object
- Parameters
obj -- pointer to an object
- Returns
the signal function
-
lv_design_cb_t
lv_obj_get_design_cb
(const lv_obj_t *obj)¶ Get the design function of an object
- Parameters
obj -- pointer to an object
- Returns
the design function
-
lv_event_cb_t
lv_obj_get_event_cb
(const lv_obj_t *obj)¶ Get the event function of an object
- Parameters
obj -- pointer to an object
- Returns
the event function
-
bool
lv_obj_is_point_on_coords
(lv_obj_t *obj, const lv_point_t *point)¶ Check if a given screen-space point is on an object's coordinates.
This method is intended to be used mainly by advanced hit testing algorithms to check whether the point is even within the object (as an optimization).
- Parameters
obj -- object to check
point -- screen-space point
-
bool
lv_obj_hittest
(lv_obj_t *obj, lv_point_t *point)¶ Hit-test an object given a particular point in screen space.
- Parameters
obj -- object to hit-test
point -- screen-space point
- Returns
true if the object is considered under the point
-
void *
lv_obj_get_ext_attr
(const lv_obj_t *obj)¶ Get the ext pointer
- Parameters
obj -- pointer to an object
- Returns
the ext pointer but not the dynamic version Use it as ext->data1, and NOT da(ext)->data1
-
void
lv_obj_get_type
(const lv_obj_t *obj, lv_obj_type_t *buf)¶ Get object's and its ancestors type. Put their name in
type_buf
starting with the current type. E.g. buf.type[0]="lv_btn", buf.type[1]="lv_cont", buf.type[2]="lv_obj"- Parameters
obj -- pointer to an object which type should be get
buf -- pointer to an
lv_obj_type_t
buffer to store the types
-
lv_obj_user_data_t
lv_obj_get_user_data
(const lv_obj_t *obj)¶ Get the object's user data
- Parameters
obj -- pointer to an object
- Returns
user data
-
lv_obj_user_data_t *
lv_obj_get_user_data_ptr
(const lv_obj_t *obj)¶ Get a pointer to the object's user data
- Parameters
obj -- pointer to an object
- Returns
pointer to the user data
-
void
lv_obj_set_user_data
(lv_obj_t *obj, lv_obj_user_data_t data)¶ Set the object's user data. The data will be copied.
- Parameters
obj -- pointer to an object
data -- user data
-
void *
lv_obj_get_group
(const lv_obj_t *obj)¶ Get the group of the object
- Parameters
obj -- pointer to an object
- Returns
the pointer to group of the object
-
bool
lv_obj_is_focused
(const lv_obj_t *obj)¶ Tell whether the object is the focused object of a group or not.
- Parameters
obj -- pointer to an object
- Returns
true: the object is focused, false: the object is not focused or not in a group
-
lv_obj_t *
lv_obj_get_focused_obj
(const lv_obj_t *obj)¶ Get the really focused object by taking
focus_parent
into account.- Parameters
obj -- the start object
- Returns
the object to really focus
-
lv_res_t
lv_obj_handle_get_type_signal
(lv_obj_type_t *buf, const char *name)¶ Used in the signal callback to handle
LV_SIGNAL_GET_TYPE
signal- Parameters
buf -- pointer to
lv_obj_type_t
. (param
in the signal callback)name -- name of the object. E.g. "lv_btn". (Only the pointer is saved)
- Returns
LV_RES_OK
-
void
lv_obj_init_draw_rect_dsc
(lv_obj_t *obj, uint8_t type, lv_draw_rect_dsc_t *draw_dsc)¶ Initialize a rectangle descriptor from an object's styles
Note
Only the relevant fields will be set. E.g. if
border width == 0
the other border properties won't be evaluated.- Parameters
obj -- pointer to an object
type -- type of style. E.g.
LV_OBJ_PART_MAIN
,LV_BTN_SLIDER_KOB
draw_dsc -- the descriptor the initialize
-
void
lv_obj_init_draw_img_dsc
(lv_obj_t *obj, uint8_t part, lv_draw_img_dsc_t *draw_dsc)¶
-
lv_coord_t
lv_obj_get_draw_rect_ext_pad_size
(lv_obj_t *obj, uint8_t part)¶ Get the required extra size (around the object's part) to draw shadow, outline, value etc.
- Parameters
obj -- pointer to an object
part -- part of the object
-
void
lv_obj_fade_in
(lv_obj_t *obj, uint32_t time, uint32_t delay)¶ Fade in (from transparent to fully cover) an object and all its children using an
opa_scale
animation.- Parameters
obj -- the object to fade in
time -- duration of the animation [ms]
delay -- wait before the animation starts [ms]
-
void
lv_obj_fade_out
(lv_obj_t *obj, uint32_t time, uint32_t delay)¶ Fade out (from fully cover to transparent) an object and all its children using an
opa_scale
animation.- Parameters
obj -- the object to fade in
time -- duration of the animation [ms]
delay -- wait before the animation starts [ms]
-
struct
lv_realign_t
¶
-
struct
_lv_obj_t
¶ Public Members
-
lv_ll_t
child_ll
¶ Linked list to store the children objects
-
lv_area_t
coords
¶ Coordinates of the object (x1, y1, x2, y2)
-
lv_event_cb_t
event_cb
¶ Event callback function
-
lv_signal_cb_t
signal_cb
¶ Object type specific signal function
-
lv_design_cb_t
design_cb
¶ Object type specific design function
-
void *
ext_attr
¶ Object type specific extended data
-
lv_style_list_t
style_list
¶
-
uint8_t
ext_click_pad_hor
¶ Extra click padding in horizontal direction
-
uint8_t
ext_click_pad_ver
¶ Extra click padding in vertical direction
-
lv_area_t
ext_click_pad
¶ Extra click padding area.
-
lv_coord_t
ext_draw_pad
¶ EXTend the size in every direction for drawing.
-
uint8_t
click
¶ 1: Can be pressed by an input device
-
uint8_t
drag
¶ 1: Enable the dragging
-
uint8_t
drag_throw
¶ 1: Enable throwing with drag
-
uint8_t
drag_parent
¶ 1: Parent will be dragged instead
1: Object is hidden
-
uint8_t
top
¶ 1: If the object or its children is clicked it goes to the foreground
-
uint8_t
parent_event
¶ 1: Send the object's events to the parent too.
-
uint8_t
adv_hittest
¶ 1: Use advanced hit-testing (slower)
-
uint8_t
gesture_parent
¶ 1: Parent will be gesture instead
-
uint8_t
focus_parent
¶ 1: Parent will be focused instead
-
lv_drag_dir_t
drag_dir
¶ Which directions the object can be dragged in
-
lv_bidi_dir_t
base_dir
¶ Base direction of texts related to this object
-
void *
group_p
¶
-
uint8_t
protect
¶ Automatically happening actions can be prevented. 'OR'ed values from
lv_protect_t
-
lv_state_t
state
¶
-
lv_realign_t
realign
¶ Information about the last call to lv_obj_align.
-
lv_obj_user_data_t
user_data
¶ Custom user data for object.
-
lv_ll_t
-
struct
lv_obj_type_t
¶ - #include <lv_obj.h>
Used by
lv_obj_get_type()
. The object's and its ancestor types are stored herePublic Members
-
const char *
type
[LV_MAX_ANCESTOR_NUM
]¶ [0]: the actual type, [1]: ancestor, [2] #1's ancestor ... [x]: "lv_obj"
-
const char *
-
struct
lv_hit_test_info_t
¶
-
struct
lv_get_style_info_t
¶
-
struct
lv_get_state_info_t
¶