LED (lv_led)¶
Overview¶
The LEDs are rectangle-like (or circle) object. It's brightness can be adjusted. With lower brightness the the colors of the LED become darker.
Parts and Styles¶
The LEDs have only one main part, called LV_LED_PART_MAIN
and it uses all the typical background style properties.
Usage¶
Brightness¶
You can set their brightness with lv_led_set_bright(led, bright)
. The brightness should be between 0 (darkest) and 255 (lightest).
Toggle¶
Use lv_led_on(led)
and lv_led_off(led)
to set the brightness to a predefined ON or OFF value. The lv_led_toggle(led)
toggles between the ON and OFF state.
Example¶
C¶
LED with custom style¶
code
#include "../../../lv_examples.h"
#if LV_USE_LED
void lv_ex_led_1(void)
{
/*Create a LED and switch it OFF*/
lv_obj_t * led1 = lv_led_create(lv_scr_act(), NULL);
lv_obj_align(led1, NULL, LV_ALIGN_CENTER, -80, 0);
lv_led_off(led1);
/*Copy the previous LED and set a brightness*/
lv_obj_t * led2 = lv_led_create(lv_scr_act(), led1);
lv_obj_align(led2, NULL, LV_ALIGN_CENTER, 0, 0);
lv_led_set_bright(led2, 190);
/*Copy the previous LED and switch it ON*/
lv_obj_t * led3 = lv_led_create(lv_scr_act(), led1);
lv_obj_align(led3, NULL, LV_ALIGN_CENTER, 80, 0);
lv_led_on(led3);
}
#endif
MicroPython¶
LED with custom style¶
Click to try in the simulator!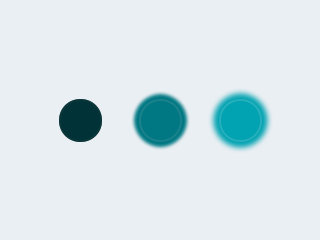
code
# Create a LED and switch it OFF
led1 = lv.led(lv.scr_act(),None)
led1.align(None,lv.ALIGN.CENTER,-80,0)
led1.off()
# Copy the previous LED and set a brightness
led2=lv.led(lv.scr_act(), led1)
led2.align(None,lv.ALIGN.CENTER,0,0)
led2.set_bright(190)
# Copy the previous LED and switch it ON
led3=lv.led(lv.scr_act(), led1)
led3.align(None,lv.ALIGN.CENTER,80,0)
led3.on()
API¶
Typedefs
-
typedef uint8_t
lv_led_part_t
¶
Functions
-
lv_obj_t *
lv_led_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a led objects
- Parameters
par -- pointer to an object, it will be the parent of the new led
copy -- pointer to a led object, if not NULL then the new object will be copied from it
- Returns
pointer to the created led
-
void
lv_led_set_bright
(lv_obj_t *led, uint8_t bright)¶ Set the brightness of a LED object
- Parameters
led -- pointer to a LED object
bright -- LV_LED_BRIGHT_MIN (max. dark) ... LV_LED_BRIGHT_MAX (max. light)