Switch (lv_switch)¶
Overview¶
The Switch can be used to turn on/off something. It looks like a little slider.
Parts and Styles¶
The Switch uses the the following parts:
LV_SWITCH_PART_BG
: main partLV_SWITCH_PART_INDIC
: the indicator (virtual part)LV_SWITCH_PART_KNOB
: the knob (virtual part)
The parts and style works the same as in case of Slider. Read its documentation for a details description.
##Usage
Change state¶
The state of the Switch can be changed by clicking on it or by lv_switch_on(switch, LV_ANIM_ON/OFF)
, lv_switch_off(switch, LV_ANIM_ON/OFF)
or lv_switch_toggle(switch, LV_ANOM_ON/OFF)
functions
Animation time¶
The time of animations, when the switch changes state, can be adjusted with lv_switch_set_anim_time(switch, anim_time)
.
Events¶
Besides the Generic events the following Special events are sent by the Switch:
LV_EVENT_VALUE_CHANGED Sent when the switch changes state.
Keys¶
LV_KEY_UP, LV_KEY_RIGHT Turn on the slider
LV_KEY_DOWN, LV_KEY_LEFT Turn off the slider
Learn more about Keys.
Example¶
C¶
Simple Switch¶
code
#include "../../../lv_examples.h"
#include <stdio.h>
#if LV_USE_SWITCH
static void event_handler(lv_obj_t * obj, lv_event_t event)
{
if(event == LV_EVENT_VALUE_CHANGED) {
printf("State: %s\n", lv_switch_get_state(obj) ? "On" : "Off");
}
}
void lv_ex_switch_1(void)
{
/*Create a switch and apply the styles*/
lv_obj_t *sw1 = lv_switch_create(lv_scr_act(), NULL);
lv_obj_align(sw1, NULL, LV_ALIGN_CENTER, 0, -50);
lv_obj_set_event_cb(sw1, event_handler);
/*Copy the first switch and turn it ON*/
lv_obj_t *sw2 = lv_switch_create(lv_scr_act(), sw1);
lv_switch_on(sw2, LV_ANIM_ON);
lv_obj_align(sw2, NULL, LV_ALIGN_CENTER, 0, 50);
}
#endif
MicroPython¶
Simple Switch¶
Click to try in the simulator!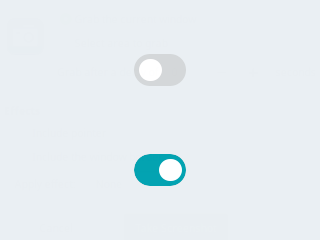
code
def event_handler(obj,evt):
if evt == lv.EVENT.VALUE_CHANGED:
state = obj.get_state()
if state:
print("State: On")
else:
print("State: Off")
#Create a switch and apply the styles
sw1 = lv.switch(lv.scr_act(), None)
sw1.align(None, lv.ALIGN.CENTER, 0, -50)
sw1.set_event_cb(event_handler)
# Copy the first switch and turn it ON
sw2=lv.switch(lv.scr_act(),sw1)
sw2.on(lv.ANIM.ON)
sw2.set_event_cb(event_handler)
sw2.align(None, lv.ALIGN.CENTER, 0, 50)
API¶
Typedefs
-
typedef uint8_t
lv_switch_part_t
¶
Enums
Functions
-
lv_obj_t *
lv_switch_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a switch objects
- Parameters
par -- pointer to an object, it will be the parent of the new switch
copy -- pointer to a switch object, if not NULL then the new object will be copied from it
- Returns
pointer to the created switch
-
void
lv_switch_on
(lv_obj_t *sw, lv_anim_enable_t anim)¶ Turn ON the switch
- Parameters
sw -- pointer to a switch object
anim -- LV_ANIM_ON: set the value with an animation; LV_ANIM_OFF: change the value immediately
-
void
lv_switch_off
(lv_obj_t *sw, lv_anim_enable_t anim)¶ Turn OFF the switch
- Parameters
sw -- pointer to a switch object
anim -- LV_ANIM_ON: set the value with an animation; LV_ANIM_OFF: change the value immediately
-
bool
lv_switch_toggle
(lv_obj_t *sw, lv_anim_enable_t anim)¶ Toggle the position of the switch
- Parameters
sw -- pointer to a switch object
anim -- LV_ANIM_ON: set the value with an animation; LV_ANIM_OFF: change the value immediately
- Returns
resulting state of the switch.
-
static inline void
lv_switch_set_anim_time
(lv_obj_t *sw, uint16_t anim_time)¶ Set the animation time of the switch
- Parameters
sw -- pointer to a switch object
anim_time -- animation time
- Returns
style pointer to a style
-
struct
lv_switch_ext_t
¶