Slider (lv_slider)¶
Overview¶
The Slider object looks like a Bar supplemented with a knob. The knob can be dragged to set a value. The Slider also can be vertical or horizontal.
Parts and Styles¶
The Slider's main part is called LV_SLIDER_PART_BG
and it uses the typical background style properties.
LV_SLIDER_PART_INDIC
is a virtual part which also uses all the typical background properties.
By default, the indicator maximal size is the same as the background's size but setting positive padding values in LV_SLIDER_PART_BG
will make the indicator smaller. (negative values will make it larger)
If the value style property is used on the indicator the alignment will be calculated based on the current size of the indicator.
For example a center aligned value is always shown in the middle of the indicator regardless it's current size.
LV_SLIDER_PART_KNOB
is a virtual part using all the typical background properties to describe the knob(s). Similarly to the indicator the value text is also aligned to the current position and size of the knob.
By default the knob is square (with a radius) with side length equal to the smaller side of the slider. The knob can be made larger with the padding values. Padding values can be asymmetric too.
Usage¶
Value and range¶
To set an initial value use lv_slider_set_value(slider, new_value, LV_ANIM_ON/OFF)
.
lv_slider_set_anim_time(slider, anim_time)
sets the animation time in milliseconds.
To specify the range (min, max values) the lv_slider_set_range(slider, min , max)
can be used.
Symmetrical and Range¶
Besides the normal type the Slider can be configured in two additional types:
LV_SLIDER_TYPE_NORMAL
normal typeLV_SLIDER_TYPE_SYMMETRICAL
draw the indicator symmetrical to zero (drawn from zero, left to right)LV_SLIDER_TYPE_RANGE
allow the use of an additional knob for the left (start) value. (Can be used withlv_slider_set/get_left_value()
)
The type can be changed with lv_slider_set_type(slider, LV_SLIDER_TYPE_...)
Knob-only mode¶
Normally, the slider can be adjusted either by dragging the knob, or clicking on the slider bar. In the latter case the knob moves to the point clicked and slider value changes accordingly. In some cases it is desirable to set the slider to react on dragging the knob only.
This feature is enabled by calling lv_obj_set_adv_hittest(slider, true);
.
Events¶
Besides the Generic events the following Special events are sent by the Slider:
LV_EVENT_VALUE_CHANGED Sent while the slider is being dragged or changed with keys. The event is sent continuously while the slider is dragged and only when it is released. Use
lv_slider_is_dragged
to decide whether is slider is being dragged or just released.
Keys¶
LV_KEY_UP, LV_KEY_RIGHT Increment the slider's value by 1
LV_KEY_DOWN, LV_KEY_LEFT Decrement the slider's value by 1
Learn more about Keys.
Example¶
C¶
Slider with custom style¶
code
#include "../../../lv_examples.h"
#include <stdio.h>
#if LV_USE_SLIDER
static void event_handler(lv_obj_t * obj, lv_event_t event)
{
if(event == LV_EVENT_VALUE_CHANGED) {
printf("Value: %d\n", lv_slider_get_value(obj));
}
}
void lv_ex_slider_1(void)
{
/*Create a slider*/
lv_obj_t * slider = lv_slider_create(lv_scr_act(), NULL);
lv_obj_align(slider, NULL, LV_ALIGN_CENTER, 0, 0);
lv_obj_set_event_cb(slider, event_handler);
}
#endif
Set value with slider¶
code
#include "../../../lv_examples.h"
#include <stdio.h>
#if LV_USE_SLIDER
static void slider_event_cb(lv_obj_t * slider, lv_event_t event);
static lv_obj_t * slider_label;
void lv_ex_slider_2(void)
{
/* Create a slider in the center of the display */
lv_obj_t * slider = lv_slider_create(lv_scr_act(), NULL);
lv_obj_set_width(slider, LV_DPI * 2);
lv_obj_align(slider, NULL, LV_ALIGN_CENTER, 0, 0);
lv_obj_set_event_cb(slider, slider_event_cb);
lv_slider_set_range(slider, 0, 100);
/* Create a label below the slider */
slider_label = lv_label_create(lv_scr_act(), NULL);
lv_label_set_text(slider_label, "0");
lv_obj_set_auto_realign(slider_label, true);
lv_obj_align(slider_label, slider, LV_ALIGN_OUT_BOTTOM_MID, 0, 10);
/* Create an informative label */
lv_obj_t * info = lv_label_create(lv_scr_act(), NULL);
lv_label_set_text(info, "Welcome to the slider+label demo!\n"
"Move the slider and see that the label\n"
"updates to match it.");
lv_obj_align(info, NULL, LV_ALIGN_IN_TOP_LEFT, 10, 10);
}
static void slider_event_cb(lv_obj_t * slider, lv_event_t event)
{
if(event == LV_EVENT_VALUE_CHANGED) {
static char buf[4]; /* max 3 bytes for number plus 1 null terminating byte */
snprintf(buf, 4, "%u", lv_slider_get_value(slider));
lv_label_set_text(slider_label, buf);
}
}
#endif
MicroPython¶
Slider with custom style¶
Click to try in the simulator!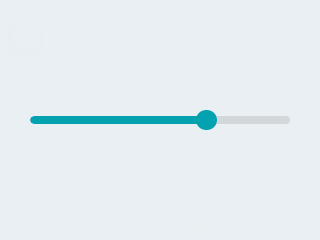
code
def event_handler(source,evt):
if evt == lv.EVENT.VALUE_CHANGED:
print("Value:",slider.get_value())
# create a slider
slider = lv.slider(lv.scr_act(),None)
slider.set_width(200)
slider.align(None,lv.ALIGN.CENTER,0,0)
slider.set_event_cb(event_handler)
Set value with slider¶
Click to try in the simulator!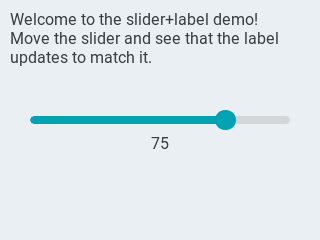
code
def event_handler(source,evt):
if evt == lv.EVENT.VALUE_CHANGED:
# print(slider.get_value())
slider_label.set_text(str(slider.get_value()))
# Create a slider in the center of the display
slider = lv.slider(lv.scr_act(),None)
slider.set_width(200)
slider.align(None,lv.ALIGN.CENTER,0,0)
slider.set_event_cb(event_handler)
slider.set_range(0, 100)
# Create a label below the slider
slider_label=lv.label(lv.scr_act(), None)
slider_label.set_text("0")
slider_label.set_auto_realign(True)
slider_label.align(slider,lv.ALIGN.OUT_BOTTOM_MID,0,10)
# Create an informative label
info_label=lv.label(lv.scr_act(), None)
info_label.set_text(
"""Welcome to the slider +
label demo!
Move the slider
and see that the label
updates to match it."""
)
info_label.align(None, lv.ALIGN.IN_TOP_LEFT, 10, 10)
API¶
Typedefs
-
typedef uint8_t
lv_slider_type_t
¶
Enums
Functions
-
lv_obj_t *
lv_slider_create
(lv_obj_t *par, const lv_obj_t *copy)¶ Create a slider objects
- Parameters
par -- pointer to an object, it will be the parent of the new slider
copy -- pointer to a slider object, if not NULL then the new object will be copied from it
- Returns
pointer to the created slider
-
static inline void
lv_slider_set_value
(lv_obj_t *slider, int16_t value, lv_anim_enable_t anim)¶ Set a new value on the slider
- Parameters
slider -- pointer to a slider object
value -- new value
anim -- LV_ANIM_ON: set the value with an animation; LV_ANIM_OFF: change the value immediately
-
static inline void
lv_slider_set_left_value
(lv_obj_t *slider, int16_t left_value, lv_anim_enable_t anim)¶ Set a new value for the left knob of a slider
- Parameters
slider -- pointer to a slider object
left_value -- new value
anim -- LV_ANIM_ON: set the value with an animation; LV_ANIM_OFF: change the value immediately
-
static inline void
lv_slider_set_range
(lv_obj_t *slider, int16_t min, int16_t max)¶ Set minimum and the maximum values of a bar
- Parameters
slider -- pointer to the slider object
min -- minimum value
max -- maximum value
-
static inline void
lv_slider_set_anim_time
(lv_obj_t *slider, uint16_t anim_time)¶ Set the animation time of the slider
- Parameters
slider -- pointer to a bar object
anim_time -- the animation time in milliseconds.
-
static inline void
lv_slider_set_type
(lv_obj_t *slider, lv_slider_type_t type)¶ Make the slider symmetric to zero. The indicator will grow from zero instead of the minimum position.
- Parameters
slider -- pointer to a slider object
en -- true: enable disable symmetric behavior; false: disable
-
int16_t
lv_slider_get_value
(const lv_obj_t *slider)¶ Get the value of the main knob of a slider
- Parameters
slider -- pointer to a slider object
- Returns
the value of the main knob of the slider
-
static inline int16_t
lv_slider_get_left_value
(const lv_obj_t *slider)¶ Get the value of the left knob of a slider
- Parameters
slider -- pointer to a slider object
- Returns
the value of the left knob of the slider
-
static inline int16_t
lv_slider_get_min_value
(const lv_obj_t *slider)¶ Get the minimum value of a slider
- Parameters
slider -- pointer to a slider object
- Returns
the minimum value of the slider
-
static inline int16_t
lv_slider_get_max_value
(const lv_obj_t *slider)¶ Get the maximum value of a slider
- Parameters
slider -- pointer to a slider object
- Returns
the maximum value of the slider
-
bool
lv_slider_is_dragged
(const lv_obj_t *slider)¶ Give the slider is being dragged or not
- Parameters
slider -- pointer to a slider object
- Returns
true: drag in progress false: not dragged
-
static inline uint16_t
lv_slider_get_anim_time
(lv_obj_t *slider)¶ Get the animation time of the slider
- Parameters
slider -- pointer to a slider object
- Returns
the animation time in milliseconds.
-
static inline lv_slider_type_t
lv_slider_get_type
(lv_obj_t *slider)¶ Get whether the slider is symmetric or not.
- Parameters
slider -- pointer to a bar object
- Returns
true: symmetric is enabled; false: disable
-
struct
lv_slider_ext_t
¶ Public Members
-
lv_bar_ext_t
bar
¶
-
lv_style_list_t
style_knob
¶
-
lv_area_t
left_knob_area
¶
-
lv_area_t
right_knob_area
¶
-
int16_t *
value_to_set
¶
-
uint8_t
dragging
¶
-
uint8_t
left_knob_focus
¶
-
lv_bar_ext_t